今天是一次做Go的笔记,一开始直接打开Github上的Go项目然后跑到Wiki位置,然后作者列出了一堆学习Go的资料,这里我
以第一个学习资料https://tour.golang.org/作为Go学习到入门。然后为了训练我的终端运用
能力还有Vim下的编码能力这里我使用到了tmux
和Vim
编辑器,然后之前已经在VIM里面安装了Vim-go
插件了,所以在之前
的Go项目文件目录下可以直接使用命令模式使用:Go
来执行相应的操作。如果项目报错都话你可能是没有按照
Vim-go
的要求现在项目里面执行:GoInstallBinaries
.
You will also need to install all the necessary binaries. vim-go makes it easy to install all of them by providing a command, :GoInstallBinaries, which will go get all the required binaries.
但是由于但由于go的代码很多在github和golang.org上,涉及到墙的问题。自动安装可能会失败。当然你有梯子的话除外;我们可以手工安装,进入到GOPATH的SRC目录下,运行命令 git clone https://github.com/golang/tools golang.org/x/tools
,再接着上一步:GoInstallBinaries
即可
我的解决方式是先让终端邹代理,至于如何让终端走代理呢,无非就是
export http_proxy=http://127.0.0.1:12333
export http_proxy=https://127.0.0.1:12333
然后在通过命令打开vim,然后再:GoInstallBinaries
,这样子就能下载Go所需要的文件了
最后出现如图所示:
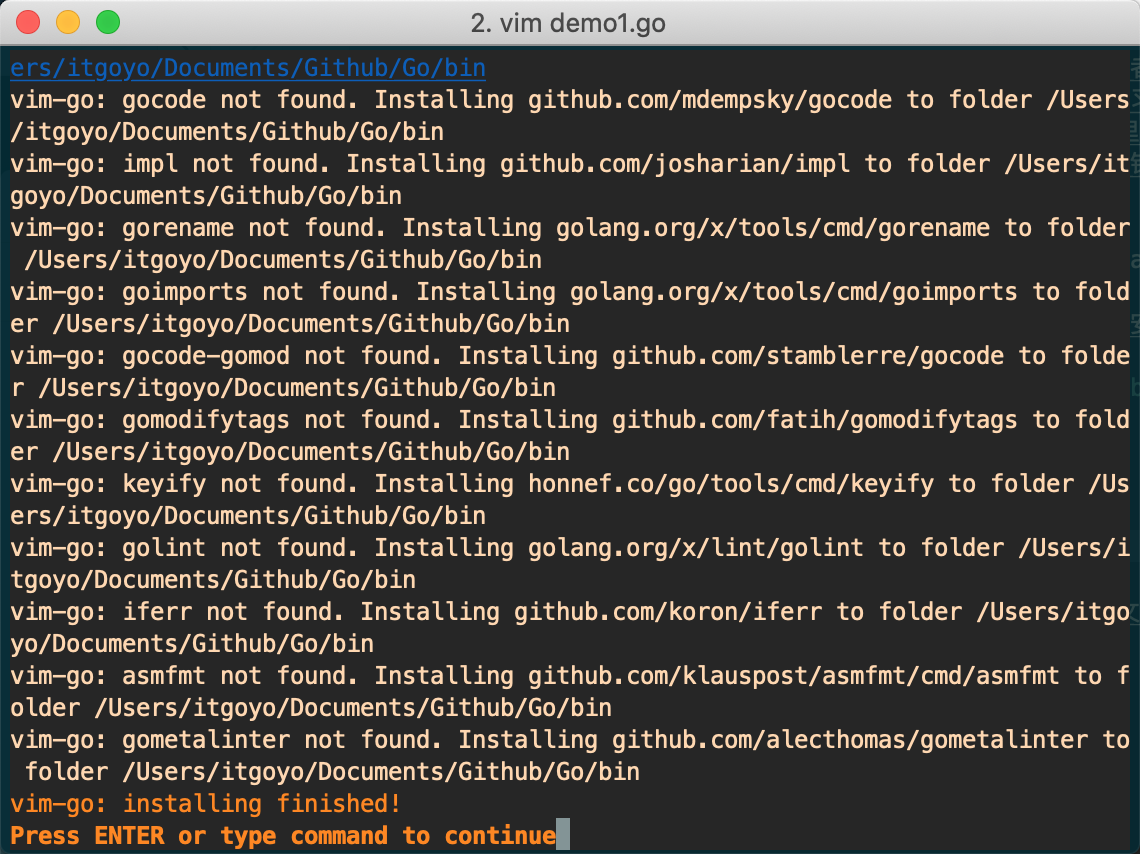
项目一 Packages
package main
import (
"fmt"
"math/rand"
)
func main() {
fmt.Println("My favorite number is", rand.Intn(10))
}
然后在Vim下面执行:Go Run
即可输出
My favorite number is 1
项目二 Imports
package main
import (
"fmt"
"math"
)
func main() {
fmt.Printf("Now you have %g problems.\n", math.Sqrt(7))
}
输出
Now you have 2.6457513110645907 problems.
项目三 Exported names
In Go, a name is exported if it begins with a capital letter. For example, Pizza
is an exported name, as is Pi
, which is exported from the math
package.
pizza
and pi
do not start with a capital letter, so they are not exported.
When importing a package, you can refer only to its exported names. Any "unexported" names are not accessible from outside the package.
Run the code. Notice the error message.
To fix the error, rename math.pi
to math.Pi
and try it again.
package main
import (
"fmt"
"math"
)
func main() {
fmt.Println(math.pi)
}
输出
3.141592653589793
项目四 Functions
A function can take zero or more arguments.
In this example, add
takes two parameters of type int
.
Notice that the type comes after the variable name.
(For more about why types look the way they do, see the article on Go's declaration syntax.)
package main
import "fmt"
func add(x int, y int) int {
return x + y
}
func main() {
fmt.Println(add(42, 13))
}
输出
55
项目五 Functions continued
When two or more consecutive named function parameters share a type, you can omit the type from all but the last.
In this example, we shortened
x int, y int
to
x, y int
package main
import "fmt"
func add(x, y int) int {
return x + y
}
func main() {
fmt.Println(add(42, 13))
}
输出
55
项目六 Multiple results
A function can return any number of results.
The swap
function returns two strings.
package main
import "fmt"
func swap(x, y string) (string, string) {
return y, x
}
func main() {
a, b := swap("hello", "world")
fmt.Println(a, b)
}
输出
world hello
项目七 Named return values
Go's return values may be named. If so, they are treated as variables defined at the top of the function.
These names should be used to document the meaning of the return values.
A return statement without arguments returns the named return values. This is known as a "naked" return.
Naked return statements should be used only in short functions, as with the example shown here. They can harm readability in longer functions.
package main
import "fmt"
func split(sum int) (x, y int) {
x = sum * 4 / 9
y = sum - x
return
}
func main() {
fmt.Println(split(17))
}
输出
7 10
项目八 Variables
The var
statement declares a list of variables; as in function argument lists, the type is last.
A var
statement can be at package or function level. We see both in this example.
package main
import "fmt"
var c, python, java bool
func main() {
var i int
fmt.Println(i, c, python, java)
}
输出
0 false false false
项目九 Variables with initializers
A var declaration can include initializers, one per variable.
If an initializer is present, the type can be omitted; the variable will take the type of the initializer.
package main
import "fmt"
var i, j int = 1, 2
func main() {
var c, python, java = true, false, "no!"
fmt.Println(i, j, c, python, java)
}
输出
1 2 true false no!
项目十 Short variable declarations
Inside a function, the :=
short assignment statement can be used in place of a var
declaration with implicit type.
Outside a function, every statement begins with a keyword (var
, func
, and so on) and so the :=
construct is not available.
package main
import "fmt"
func main() {
var i, j int = 1, 2
k := 3
c, python, java := true, false, "no!"
fmt.Println(i, j, k, c, python, java)
}
输出
1 2 3 true false no!
项目十一Basic types
Go's basic types are
bool
string
int int8 int16 int32 int64
uint uint8 uint16 uint32 uint64 uintptr
byte // alias for uint8
rune // alias for int32
// represents a Unicode code point
float32 float64
complex64 complex128
The example shows variables of several types, and also that variable declarations may be "factored" into blocks, as with import statements.
The int
, uint
, and uintptr
types are usually 32 bits wide on 32-bit systems and 64 bits wide on 64-bit systems. When you need an integer value you should use int
unless you have a specific reason to use a sized or unsigned integer type.
package main
import (
"fmt"
"math/cmplx"
)
var (
ToBe bool = false
MaxInt uint64 = 1<<64 - 1
z complex128 = cmplx.Sqrt(-5 + 12i)
)
func main() {
fmt.Printf("Type: %T Value: %v\n", ToBe, ToBe)
fmt.Printf("Type: %T Value: %v\n", MaxInt, MaxInt)
fmt.Printf("Type: %T Value: %v\n", z, z)
}
输出
Type: bool Value: false
Type: uint64 Value: 18446744073709551615
Type: complex128 Value: (2+3i)
项目十二 Zero values
Variables declared without an explicit initial value are given their zero value.
The zero value is:
0
for numeric types,false
for the boolean type, and""
(the empty string) for strings.
package main
import "fmt"
func main() {
var i int
var f float64
var b bool
var s string
fmt.Printf("%v %v %v %q\n", i, f, b, s)
}
输出
0 0 false ""
项目十三 Type conversions
The expression T(v)
converts the value v
to the type T
.
Some numeric conversions:
var i int = 42
var f float64 = float64(i)
var u uint = uint(f)
Or, put more simply:
i := 42
f := float64(i)
u := uint(f)
Unlike in C, in Go assignment between items of different type requires an explicit conversion. Try removing the float64
or uint
conversions in the example and see what happens.
package main
import (
"fmt"
"math"
)
func main() {
var x, y int = 3, 4
var f float64 = math.Sqrt(float64(x*x + y*y))
var z uint = uint(f)
fmt.Println(x, y, z)
}
输出
3 4 5
项目十四 Type inference
When declaring a variable without specifying an explicit type (either by using the :=
syntax or var =
expression syntax), the variable's type is inferred from the value on the right hand side.
When the right hand side of the declaration is typed, the new variable is of that same type:
var i int
j := i // j is an int
But when the right hand side contains an untyped numeric constant, the new variable may be an int
, float64
, or complex128
depending on the precision of the constant:
i := 42 // int
f := 3.142 // float64
g := 0.867 + 0.5i // complex128
Try changing the initial value of v
in the example code and observe how its type is affected.
package main
import "fmt"
func main() {
v := 42 // change me!
fmt.Printf("v is of type %T\n", v)
}
输出
v is of type int
项目十五 Constants
Constants are declared like variables, but with the const
keyword.
Constants can be character, string, boolean, or numeric values.
Constants cannot be declared using the :=
syntax.
package main
import "fmt"
const Pi = 3.14
func main() {
const World = "世界"
fmt.Println("Hello", World)
fmt.Println("Happy", Pi, "Day")
const Truth = true
fmt.Println("Go rules?", Truth)
}
输出
Hello 世界
Happy 3.14 Day
Go rules? true
项目十六 Numeric Constants
Numeric constants are high-precision values.
An untyped constant takes the type needed by its context.
Try printing needInt(Big)
too.
(An int
can store at maximum a 64-bit integer, and sometimes less.)
package main
import "fmt"
const (
// Create a huge number by shifting a 1 bit left 100 places.
// In other words, the binary number that is 1 followed by 100 zeroes.
Big = 1 << 100
// Shift it right again 99 places, so we end up with 1<<1, or 2.
Small = Big >> 99
)
func needInt(x int) int { return x*10 + 1 }
func needFloat(x float64) float64 {
return x * 0.1
}
func main() {
fmt.Println(needInt(Small))
fmt.Println(needFloat(Small))
fmt.Println(needFloat(Big))
}
输出
21
0.2
1.2676506002282295e+29
Congratulations!
You finished this lesson!
You can go back to the list of modules to find what to learn next, or continue with the next lesson
<Br>
<Br>
<Br>
发现更多更好玩的,欢迎关注我的微信公众号:<span style='color:red;'> FullStacker </span><br />
<Br>
<Br>
<Br>
<img src="https://raw.githubusercontent.com/itgoyo/PicGoRes/master/img/%E6%A0%BC%E9%B2%81%E7%89%B9toolpool.jpg"
height="400px" width="400px" />
</div>