android popup
android popup 有两种类型:
1. popup window
2. popup menu
POPUP WINDOW
popup window 和 popup menu 都是显示在其他的空间的上面(z轴)。
下面举例实现的例子;
例子:
package com.hualu.popup; import java.util.ArrayList; import java.util.List; import android.app.Activity; import android.content.Context; import android.content.Intent; import android.os.Bundle; import android.view.Gravity; import android.view.Menu; import android.view.View; import android.view.View.OnClickListener; import android.view.WindowManager; import android.widget.AdapterView; import android.widget.AdapterView.OnItemClickListener; import android.widget.ArrayAdapter; import android.widget.Button; import android.widget.ListView; import android.widget.PopupWindow; import android.widget.TextView; import android.widget.Toast; public class MainActivity extends Activity { private Button Left , right , above, below; private PopupWindow popupWLeft,popupWRight,popupWAbove,popupWBelow ; private Context mContext ; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); mContext = this.getApplicationContext() ; Left = (Button)this.findViewById(R.id.Left) ; right = (Button)this.findViewById(R.id.right) ; above = (Button)this.findViewById(R.id.above) ; below = (Button)this.findViewById(R.id.below) ; Button b = (Button)this.findViewById(R.id.button1) ; b.setOnClickListener(new OnClickListener() { @Override public void onClick(View v) { startActivity(new Intent("com.hualu.popup.POPUP_MENU")) ; } }); Left.setOnClickListener(new OnClickListener(){ @Override public void onClick(View v) { if(null != popupWLeft ) if(!popupWLeft.isShowing()) { showLeftPopupWindow(); }else{ dimissionLeftPopupWindow() ; } }}) ; right.setOnClickListener(new OnClickListener(){ @Override public void onClick(View v) { if(null != popupWRight ) if(!popupWRight.isShowing()) { showRightPopupWindow(); }else{ dimissionRightPopupWindow() ; } }}) ; below.setOnClickListener(new OnClickListener(){ @Override public void onClick(View v) { if(null != popupWBelow ) if(!popupWBelow.isShowing()) { showBelowPopupWindow(); }else{ dimissionBelowPopupWindow() ; } }}) ; above.setOnClickListener(new OnClickListener(){ @Override public void onClick(View v) { if(null != popupWAbove) if(!popupWAbove.isShowing()) { showAbovePopupWindow(); }else{ dimissionAbovePopupWindow() ; } }}) ; initLeftPopupWindow(); initRightPopupWindow(); initAbovePopupWindow(); initBelowPopupWindow(); } void initLeftPopupWindow(){ popupWLeft = new PopupWindow(this) ; popupWLeft.setWidth(100) ; popupWLeft.setHeight(200) ; popupWLeft.setAnimationStyle(R.style.AnimationFade) ; popupWLeft.setFocusable(true) ; //设置为false 界面上除了popup window上面可以点击,其他控件都不可以点击(点击了会无效), 并且popup window会消失 //默认是true. 其他控件都可以点击(点击会产生响应的效果), 并且popup window会消失 popupWLeft.setOutsideTouchable(true); popupWLeft.setClippingEnabled(true); popupWLeft.setIgnoreCheekPress(); popupWLeft.setInputMethodMode(PopupWindow.INPUT_METHOD_FROM_FOCUSABLE) ; popupWLeft.setBackgroundDrawable(this.getResources().getDrawable(R.drawable.balloon_r_pressed)) ; View view = this.getLayoutInflater().inflate(R.layout.layout_popupwindow_view_listview,null); popupWLeft.setContentView(view) ; ListView list = (ListView)view.findViewById(R.id.listView1); List<String> objects = new ArrayList<String>() ; objects.add("one") ; objects.add("two") ; objects.add("three") ; objects.add("four") ; ArrayAdapter<String> adapter = new ArrayAdapter<String>(this, android.R.layout.simple_list_item_1, objects) ; list.setSelector(R.drawable.task_list_selector); list.setAdapter(adapter) ; list.setOnItemClickListener(new OnItemClickListener() { @Override public void onItemClick(AdapterView<?> parent, View view, int position, long id) { Toast.makeText(mContext, ((TextView)view).getText().toString(), Toast.LENGTH_SHORT).show() ; //dimissionLeftPopupWindow() ; } }) ; } void initRightPopupWindow(){ popupWRight = new PopupWindow(this) ; popupWRight.setWidth(100) ; popupWRight.setHeight(200) ; popupWRight.setAnimationStyle(R.style.AnimationFade) ; popupWRight.setBackgroundDrawable(this.getResources().getDrawable(R.drawable.balloon_l_pressed)) ; popupWRight.setContentView(this.getLayoutInflater().inflate(R.layout.layout_popupwindow_view,null)) ; } void initAbovePopupWindow(){ popupWAbove = new PopupWindow(this) ; popupWAbove.setWidth(100) ; popupWAbove.setHeight(200) ; popupWAbove.setAnimationStyle(R.style.AnimationFade) ; //当输入法弹起来的时候,popupView不会向上移动。 popupWAbove.setSoftInputMode(WindowManager.LayoutParams.SOFT_INPUT_ADJUST_PAN); popupWAbove.setFocusable(true); //单独设定这句:下面这个两个效果达不到 //1. 點擊PopupWindow以外的地方。 //2. 點擊左下的Back按鈕。 //需要加popupWAbove.setFocusable(true); popupWAbove.setBackgroundDrawable(/*new BitmapDrawable());*/this.getResources().getDrawable(R.drawable.billd_selected)) ; popupWAbove.setContentView(this.getLayoutInflater().inflate(R.layout.layout_popupwindow_view,null)) ; } void initBelowPopupWindow(){ popupWBelow = new PopupWindow(this) ; popupWBelow.setWidth(100) ; popupWBelow.setHeight(200) ; popupWBelow.setAnimationStyle(R.style.AnimationFade) ; popupWBelow.setBackgroundDrawable(this.getResources().getDrawable(R.drawable.near_filter_popup)) ; popupWBelow.setContentView(this.getLayoutInflater().inflate(R.layout.layout_popupwindow_view,null)) ; } void showLeftPopupWindow(){ popupWLeft.showAtLocation(Left, Gravity.LEFT|Gravity.BOTTOM, 60, 200); } void dimissionLeftPopupWindow(){ popupWLeft.dismiss(); } void showRightPopupWindow(){ popupWRight.showAtLocation(right, Gravity.RIGHT|Gravity.BOTTOM, 50, 140); } void dimissionRightPopupWindow(){ popupWRight.dismiss(); } void showAbovePopupWindow(){ popupWAbove.showAtLocation(above, Gravity.TOP, 50, 185); } void dimissionAbovePopupWindow(){ popupWAbove.dismiss(); } void showBelowPopupWindow(){ popupWBelow.showAsDropDown(below); } void dimissionBelowPopupWindow(){ popupWBelow.dismiss(); } @Override public boolean onCreateOptionsMenu(Menu menu) { getMenuInflater().inflate(R.menu.activity_main, menu); return true; } }
activity_main.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity" > <Button android:id="@+id/Left" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerInParent="true" android:textColor="@drawable/button_selector" android:background="@drawable/button_drawable_selector" android:text="Left" /> <Button android:id="@+id/above" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignBaseline="@+id/Left" android:layout_alignBottom="@+id/Left" android:layout_toRightOf="@+id/Left" android:text="above" /> <Button android:id="@+id/below" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignLeft="@+id/Left" android:layout_below="@+id/Left" android:text="below" /> <Button android:id="@+id/right" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_below="@+id/above" android:layout_toRightOf="@+id/below" android:text="right" /> <Button android:id="@+id/button1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentTop="true" android:layout_centerHorizontal="true" android:text="@string/popup_menu" /> </RelativeLayout>layout_popupwindow_view.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" > <TextView android:id="@+id/textView1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="TextView" /> <TextView android:id="@+id/textView2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="TextView" /> <TextView android:id="@+id/textView3" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="TextView" /> <TextView android:id="@+id/textView4" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="TextView" /> </LinearLayout>
layout_popupwindow_view_listview.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" > <TextView android:id="@+id/textView1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Header" /> <ListView android:id="@+id/listView1" android:layout_width="match_parent" android:layout_height="wrap_content" > </ListView> </LinearLayout>style.xml
<resources> <!-- Base application theme, dependent on API level. This theme is replaced by AppBaseTheme from res/values-vXX/styles.xml on newer devices. --> <style name="AppBaseTheme" parent="android:Theme.Light"> <!-- Theme customizations available in newer API levels can go in res/values-vXX/styles.xml, while customizations related to backward-compatibility can go here. --> </style> <!-- Application theme. --> <style name="AppTheme" parent="AppBaseTheme"> <!-- All customizations that are NOT specific to a particular API-level can go here. --> </style> <style name="AnimationFade"> <item name="android:windowEnterAnimation">@anim/fade_in</item> <item name="android:windowExitAnimation">@anim/fade_out</item> </style> </resources>fade_in.xml
<?xml version="1.0" encoding="utf-8"?> <alpha xmlns:android="http://schemas.android.com/apk/res/android" android:interpolator="@android:anim/accelerate_interpolator" android:fromAlpha="0" android:toAlpha="1" android:duration="1000"> </alpha>fade_out.xml
<?xml version="1.0" encoding="utf-8"?> <alpha xmlns:android="http://schemas.android.com/apk/res/android" android:interpolator="@android:anim/accelerate_interpolator" android:fromAlpha="1" android:toAlpha="0" android:duration="1000"> </alpha>
效果:
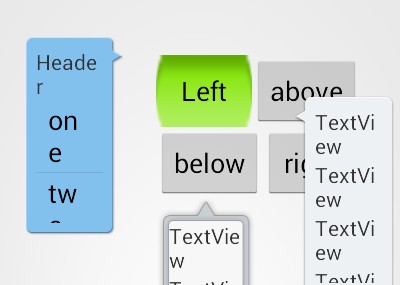
POPUP MENU
package com.hualu.popup; import android.annotation.SuppressLint; import android.app.Activity; import android.os.Bundle; import android.view.MenuItem; import android.view.View; import android.view.View.OnClickListener; import android.widget.Button; import android.widget.PopupMenu; import android.widget.PopupMenu.OnMenuItemClickListener; import android.widget.Toast; public class PopupMenuActivity extends Activity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.layout_popup_menu) ; Button btn = (Button) findViewById(R.id.btn); OnClickListener listener = new OnClickListener() { @SuppressLint("NewApi") @Override public void onClick(View v) { /** Instantiating PopupMenu class */ PopupMenu popup = new PopupMenu(getBaseContext(), v); /** Adding menu items to the popumenu */ popup.getMenuInflater().inflate(R.menu.popup, popup.getMenu()); /** Defining menu item click listener for the popup menu */ popup.setOnMenuItemClickListener(new OnMenuItemClickListener() { @Override public boolean onMenuItemClick(MenuItem item) { Toast.makeText(getBaseContext(), "You selected the action : " + item.getTitle(), Toast.LENGTH_SHORT).show(); return true; } }); /** Showing the popup menu */ popup.show(); } }; btn.setOnClickListener(listener); } }layout_popup_menu.xml
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" > <Button android:id="@+id/btn" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerHorizontal="true" android:layout_centerVertical="true" android:text="@string/str_btn" /> </RelativeLayout>strings.xml
<string name="str_btn">Open Popup Menu</string> <string name="action1">Action1</string> <string name="action2">Action2</string> <string name="action3">Action3</string> <string name="popup_menu">popup menu</string>
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.hualu.popup" android:versionCode="1" android:versionName="1.0" > <uses-sdk android:minSdkVersion="8" android:targetSdkVersion="17" /> <application android:allowBackup="true" android:icon="@drawable/ic_launcher" android:label="@string/app_name" android:theme="@style/AppTheme" > <activity android:name="com.hualu.popup.MainActivity" android:label="@string/app_name" > <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> <activity android:name=".PopupMenuActivity" > <intent-filter> <action android:name="com.hualu.popup.POPUP_MENU" /> <category android:name="android.intent.category.DEFAULT"/> </intent-filter> </activity> </application> </manifest>
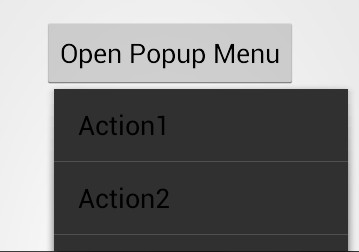