1. 新建项目
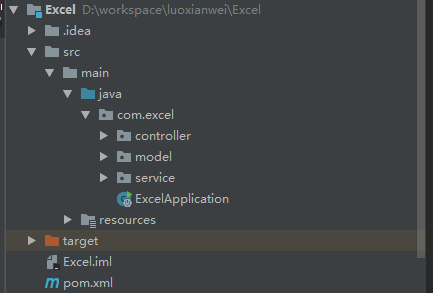
2. pom.xml
1 <?xml version="1.0" encoding="UTF-8"?>
2 <project xmlns="http://maven.apache.org/POM/4.0.0"
3 xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
4 xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
5 <modelVersion>4.0.0</modelVersion>
6
7 <groupId>com.excel</groupId>
8 <artifactId>Excel</artifactId>
9 <version>1.0-SNAPSHOT</version>
10 <!-- Spring boot 父引用-->
11 <parent>
12 <groupId>org.springframework.boot</groupId>
13 <artifactId>spring-boot-starter-parent</artifactId>
14 <version>1.4.1.RELEASE</version>
15 </parent>
16 <dependencies>
17
18 <!-- Spring boot 核心web-->
19 <dependency>
20 <groupId>org.springframework.boot</groupId>
21 <artifactId>spring-boot-starter-web</artifactId>
22 </dependency>
23
24 <!-- https://mvnrepository.com/artifact/org.apache.poi/poi -->
25 <dependency>
26 <groupId>org.apache.poi</groupId>
27 <artifactId>poi</artifactId>
28 <version>3.14</version>
29 </dependency>
30
31 <!-- https://mvnrepository.com/artifact/org.apache.poi/poi-ooxml -->
32 <dependency>
33 <groupId>org.apache.poi</groupId>
34 <artifactId>poi-ooxml</artifactId>
35 <version>3.14</version>
36 </dependency>
37
38 <!-- https://mvnrepository.com/artifact/org.apache.poi/poi-scratchpad -->
39 <dependency>
40 <groupId>org.apache.poi</groupId>
41 <artifactId>poi-scratchpad</artifactId>
42 <version>3.14</version>
43 </dependency>
44
45 <!-- https://mvnrepository.com/artifact/org.apache.poi/poi-ooxml-schemas -->
46 <dependency>
47 <groupId>org.apache.poi</groupId>
48 <artifactId>poi-ooxml-schemas</artifactId>
49 <version>3.14</version>
50 </dependency>
51 <dependency>
52 <groupId>org.projectlombok</groupId>
53 <artifactId>lombok</artifactId>
54 <version>1.16.18</version>
55 </dependency>
56
57 </dependencies>
58
59 <build>
60 <plugins>
61 <plugin>
62 <groupId>org.springframework.boot</groupId>
63 <artifactId>spring-boot-maven-plugin</artifactId>
64 <executions>
65 <execution>
66 <goals>
67 <goal>repackage</goal>
68 </goals>
69 </execution>
70 </executions>
71 <configuration>
72 <executable>true</executable>
73 </configuration>
74 </plugin>
75 </plugins>
76 </build>
77
78 </project>
3. Student.java
1 package com.excel.model;
2
3 import lombok.Data;
4
5 import java.util.Date;
6
7 @Data
8 public class Student {
9 private long id;
10 private String name;
11 private int age;
12 private String sex;
13 private Date birthday;
14
15 public Student() {
16 }
17
18 public Student(long id, String name, int age, String sex, Date birthday) {
19 this.id = id;
20 this.name = name;
21 this.age = age;
22 this.sex = sex;
23 this.birthday = birthday;
24 }
25
26 }
4. TestController.java
1 package com.excel.controller;
2
3 import com.excel.model.Student;
4 import com.excel.service.ExcelService;
5 import org.apache.poi.xssf.usermodel.XSSFWorkbook;
6 import org.springframework.beans.factory.annotation.Autowired;
7 import org.springframework.http.HttpHeaders;
8 import org.springframework.http.HttpStatus;
9 import org.springframework.http.MediaType;
10 import org.springframework.http.ResponseEntity;
11 import org.springframework.stereotype.Controller;
12 import org.springframework.web.bind.annotation.RequestMapping;
13 import java.io.ByteArrayOutputStream;
14 import java.io.OutputStream;
15 import java.io.UnsupportedEncodingException;
16 import java.text.SimpleDateFormat;
17 import java.util.ArrayList;
18 import java.util.Arrays;
19 import java.util.Date;
20 import java.util.List;
21
22 /**
23 * @date 2018/5/10
24 */
25 @Controller
26 public class TestController {
27
28 @Autowired
29 private ExcelService excelService;
30
31 @RequestMapping("/hello")
32 public String hello() {
33 return "/pages/index";
34 }
35
36 @RequestMapping("/downloadExcel")
37 public ResponseEntity<byte[]> downloadExcel() {
38 ResponseEntity<byte[]> responseEntity = null;
39 try {
40 ByteArrayOutputStream byteArrayOutputStream = new ByteArrayOutputStream();
41 String fileName = java.net.URLEncoder.encode("学生信息表.xlsx", "UTF-8");
42
43 List<Student> students = new ArrayList<Student>();
44 students.add(new Student(10000001, "张三", 20, "男", new Date()));
45 students.add(new Student(20000002, "李四", 24, "男", new Date()));
46 students.add(new Student(30000003, "王五", 22, "男", new Date()));
47 //生成EXCEL XLSX格式
48 this.exportData(students, byteArrayOutputStream);
49
50 //设置响应头让浏览器正确显示下载
51 HttpHeaders headers = new HttpHeaders();
52 headers.setContentType(MediaType.APPLICATION_OCTET_STREAM);
53 headers.setContentDispositionFormData("attachment", fileName);
54 responseEntity = new ResponseEntity<byte[]>(byteArrayOutputStream.toByteArray(), headers, HttpStatus.OK);
55 } catch (UnsupportedEncodingException e) {
56 e.printStackTrace();
57 }
58 return responseEntity;
59 }
60
61
62 public void exportData(List<Student> students, OutputStream outputStream) {
63 String header = "学生信息表";
64 //EXCEL标题
65 List<String> titles = Arrays.asList(new String[]{"学生id","姓名", "年龄", "性别", "生日"});
66 //EXCEL列
67 List<List<Object>> rows = new ArrayList<List<Object>>();
68
69 SimpleDateFormat sdf = new SimpleDateFormat("yyyy/MM/dd");
70 //从给定数据获取指定列作为EXCEL列数据
71 for (Student student : students) {
72 List<Object> row = new ArrayList<Object>();
73 //学生id
74 row.add(student.getId());
75 //姓名
76 row.add(student.getName());
77 //年龄
78 row.add(student.getAge());
79 //性别
80 row.add(student.getSex());
81 //生日
82 row.add(sdf.format(student.getBirthday()));
83 rows.add(row);
84 }
85
86 XSSFWorkbook xwb = excelService.excelForXLSX(header,titles, rows, "信息表");
87 try {
88 xwb.write(outputStream);
89 outputStream.flush();
90 outputStream.close();
91 } catch (Exception e) {
92 e.printStackTrace();
93 } finally {
94 try {
95 xwb.close();
96 }catch (Exception e) {
97 e.printStackTrace();
98 }
99 }
100 }
101
102 }
5. ExcelService.java
1 package com.excel.service;
2 import org.apache.poi.ss.util.CellRangeAddress;
3 import org.apache.poi.xssf.usermodel.*;
4 import org.springframework.stereotype.Service;
5 import java.util.List;
6
7 /**
8 * @date 2018/5/10
9 */
10 @Service
11 public class ExcelService {
12
13 /**
14 * excel
15 * @param titles 表头
16 * @param rows 数据行
17 * @param sheetName 工作表名
18 * @return
19 */
20 public XSSFWorkbook excelForXLSX(String header, List<String> titles, List<List<Object>> rows, String sheetName) {
21 XSSFWorkbook xwb = new XSSFWorkbook();
22 XSSFSheet sheet = xwb.createSheet(sheetName==null? "sheet1" : sheetName);
23
24 //创建第一行头部并设置行高及合并单元格样式
25 sheet.addMergedRegion(new CellRangeAddress(0,0,0,titles.size()-1));
26 XSSFRow row1 = sheet.createRow(0);
27 row1.setHeight((short)600);
28 /**
29 * 头部样式设置
30 */
31 //设置字体
32 XSSFFont fontHeader = xwb.createFont();
33 fontHeader.setFontName("宋体");
34 fontHeader.setFontHeightInPoints((short)16);
35 fontHeader.setBoldweight(XSSFFont.BOLDWEIGHT_BOLD);// 字体加粗
36 XSSFDataFormat formatHeader = xwb.createDataFormat();
37 //设置单元格格式
38 XSSFCellStyle styleHeader = xwb.createCellStyle();
39 styleHeader.setFont(fontHeader);
40 styleHeader.setDataFormat(formatHeader.getFormat("@")); //设置输入格式为文本格式
41 styleHeader.setAlignment(XSSFCellStyle.ALIGN_CENTER);
42 styleHeader.setVerticalAlignment(XSSFCellStyle.VERTICAL_CENTER);
43 styleHeader.setWrapText(true);
44 //给单元格赋内容
45 XSSFCell cell1 = row1.createCell(0);
46 cell1.setCellStyle(styleHeader);
47 cell1.setCellValue(new XSSFRichTextString(header));
48
49
50 //创建第二行标题并设置行高
51 XSSFRow row = sheet.createRow(1);
52 row.setHeight((short)400);
53 /**
54 * 标题样式设置
55 */
56 //设置字体
57 XSSFFont fontTitle = xwb.createFont();
58 fontTitle.setFontName("宋体");
59 fontTitle.setBoldweight(XSSFFont.BOLDWEIGHT_BOLD);// 字体加粗
60 fontTitle.setFontHeightInPoints((short)10);
61 XSSFDataFormat format = xwb.createDataFormat();
62 //设置单元格格式
63 XSSFCellStyle styleTitle = xwb.createCellStyle();
64 styleTitle.setFont(fontTitle);
65 styleTitle.setDataFormat(format.getFormat("@")); //设置输入格式为文本格式
66 styleTitle.setAlignment(XSSFCellStyle.ALIGN_CENTER);
67 styleTitle.setVerticalAlignment(XSSFCellStyle.VERTICAL_CENTER);
68 styleTitle.setWrapText(true);
69
70 /**
71 * 主体样式设置
72 */
73 //设置字体
74 XSSFFont font = xwb.createFont();
75 font.setFontName("宋体");
76 font.setFontHeightInPoints((short)10);
77 //设置单元格格式
78 XSSFCellStyle style = xwb.createCellStyle();
79 style.setFont(font);
80 style.setDataFormat(format.getFormat("@")); //设置输入格式为文本格式
81 style.setAlignment(XSSFCellStyle.ALIGN_CENTER);
82 style.setVerticalAlignment(XSSFCellStyle.VERTICAL_CENTER);
83 style.setWrapText(true);
84
85 //设置标题单元格内容
86 for(int i = 0; i < titles.size(); i++){
87 XSSFCell cell = row.createCell(i);
88 cell.setCellStyle(styleTitle);
89 cell.setCellValue(new XSSFRichTextString(titles.get(i)));
90 sheet.setColumnWidth(i, 5000);
91 sheet.setDefaultColumnStyle(i, style);
92 }
93 //设置主体单元格内容
94 XSSFCell cell;
95 int rowIdx = 2;
96 for(List<Object> dr : rows) {
97 row = sheet.createRow(rowIdx);
98 for(int di=0; di < dr.size(); di++) {
99 cell = row.createCell(di);
100 cell.setCellStyle(style);
101 String cellValue = "";
102 if(dr.get(di)==null){
103 cellValue = "";
104 }else{
105 cellValue = dr.get(di)+"";
106 }
107 cell.setCellValue(new XSSFRichTextString(cellValue));
108 }
109 rowIdx ++;
110 }
111 return xwb;
112 }
113
114 }
6. ExcelApplication.java
1 package com.excel;
2
3 import org.springframework.boot.SpringApplication;
4 import org.springframework.boot.autoconfigure.SpringBootApplication;
5
6 /**
7 * @date 2018/5/10
8 */
9 @SpringBootApplication
10 public class ExcelApplication {
11
12 public static void main(String[] args) {
13 SpringApplication.run(ExcelApplication.class,args);
14 }
15 }
7. index.html
1 <!DOCTYPE html>
2 <html lang="en">
3 <head>
4 <meta charset="UTF-8">
5 <title>Title</title>
6 </head>
7 <body>
8
9 <h2><a href="/downloadExcel">导出Excel</a></h2>
10 </body>
11 </html>
8. 页面
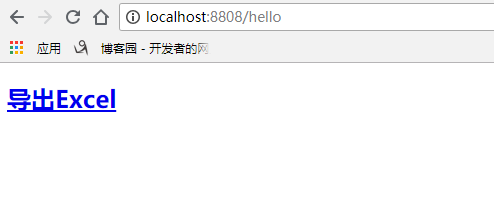
9. excel.xlsx
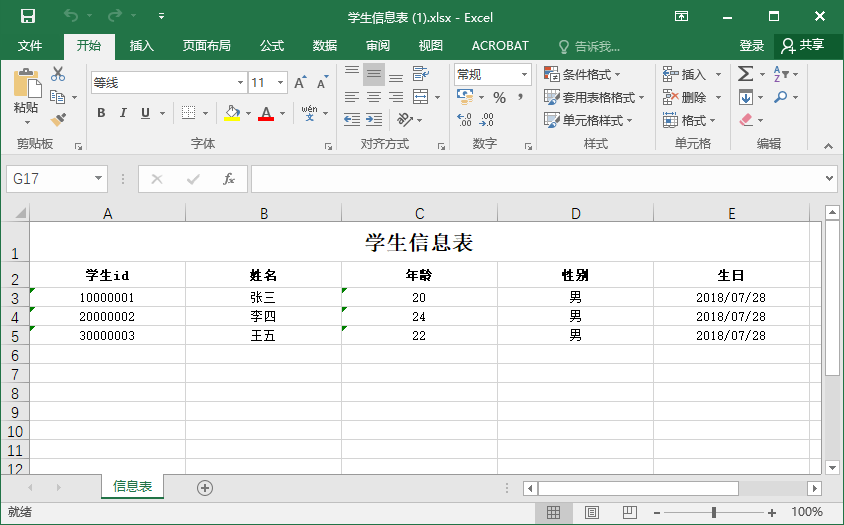