1.新建项目
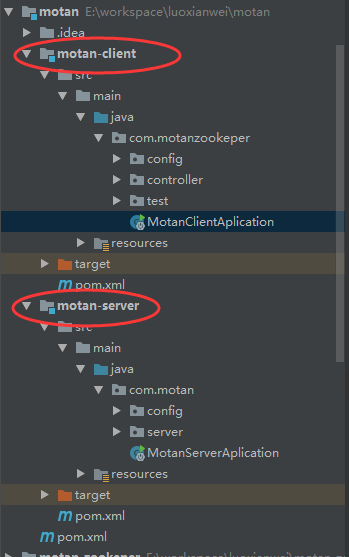
2.motan pom.xml
1 <?xml version="1.0" encoding="UTF-8"?>
2 <project xmlns="http://maven.apache.org/POM/4.0.0"
3 xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
4 xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
5 <modelVersion>4.0.0</modelVersion>
6
7 <groupId>com.motan</groupId>
8 <artifactId>motan</artifactId>
9 <packaging>pom</packaging>
10 <version>1.0-SNAPSHOT</version>
11 <modules>
12 <module>motan-server</module>
13 <module>motan-client</module>
14 </modules>
15
16 <parent>
17 <groupId>org.springframework.boot</groupId>
18 <artifactId>spring-boot-starter-parent</artifactId>
19 <version>1.5.7.RELEASE</version>
20 </parent>
21
22 <build>
23 <plugins>
24 <plugin>
25 <groupId>org.springframework.boot</groupId>
26 <artifactId>spring-boot-maven-plugin</artifactId>
27 <executions>
28 <execution>
29 <goals>
30 <goal>repackage</goal>
31 </goals>
32 </execution>
33 </executions>
34 <configuration>
35 <executable>true</executable>
36 </configuration>
37 </plugin>
38 </plugins>
39 </build>
40
41 </project>
3.motan-server pom.xml
1 <?xml version="1.0" encoding="UTF-8"?>
2 <project xmlns="http://maven.apache.org/POM/4.0.0"
3 xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
4 xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
5 <parent>
6 <artifactId>motan</artifactId>
7 <groupId>com.motan</groupId>
8 <version>1.0-SNAPSHOT</version>
9 </parent>
10 <modelVersion>4.0.0</modelVersion>
11
12 <artifactId>motan-server</artifactId>
13
14 <dependencies>
15 <!--springboot自动配置 不需要web依赖-->
16 <dependency>
17 <groupId>org.springframework.boot</groupId>
18 <artifactId>spring-boot-starter</artifactId>
19 </dependency>
20 <!--motan核心依赖-->
21 <dependency>
22 <groupId>com.weibo</groupId>
23 <artifactId>motan-core</artifactId>
24 <version>0.2.2</version>
25 </dependency>
26 <!--motan传输层 netty实现-->
27 <dependency>
28 <groupId>com.weibo</groupId>
29 <artifactId>motan-transport-netty</artifactId>
30 <version>0.2.2</version>
31 </dependency>
32 <!--motan与springboot整合-->
33 <dependency>
34 <groupId>com.weibo</groupId>
35 <artifactId>motan-springsupport</artifactId>
36 <version>0.2.1</version>
37 </dependency>
38 <!--motan使用zookeeper作为注册中心-->
39 <dependency>
40 <groupId>com.weibo</groupId>
41 <artifactId>motan-registry-zookeeper</artifactId>
42 <version>0.2.1</version>
43 </dependency>
44 </dependencies>
45
46 </project>
4.motan-client pom.xml
1 <?xml version="1.0" encoding="UTF-8"?>
2 <project xmlns="http://maven.apache.org/POM/4.0.0"
3 xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
4 xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
5 <parent>
6 <artifactId>motan</artifactId>
7 <groupId>com.motan</groupId>
8 <version>1.0-SNAPSHOT</version>
9 </parent>
10 <modelVersion>4.0.0</modelVersion>
11
12 <artifactId>motan-client</artifactId>
13
14 <properties>
15 <motan.version>0.2.1</motan.version>
16 </properties>
17
18 <dependencies>
19 <!--springboot自动配置+web mvc自动配置-->
20 <dependency>
21 <groupId>org.springframework.boot</groupId>
22 <artifactId>spring-boot-starter-web</artifactId>
23 </dependency>
24 <!--motan核心依赖-->
25 <dependency>
26 <groupId>com.weibo</groupId>
27 <artifactId>motan-core</artifactId>
28 <version>0.2.2</version>
29 </dependency>
30 <!--motan传输层 netty实现-->
31 <dependency>
32 <groupId>com.weibo</groupId>
33 <artifactId>motan-transport-netty</artifactId>
34 <version>0.2.2</version>
35 </dependency>
36 <!--motan与springboot整合-->
37 <dependency>
38 <groupId>com.weibo</groupId>
39 <artifactId>motan-springsupport</artifactId>
40 <version>0.2.1</version>
41 </dependency>
42 <!--motan使用zookeeper作为注册中心-->
43 <dependency>
44 <groupId>com.weibo</groupId>
45 <artifactId>motan-registry-zookeeper</artifactId>
46 <version>0.2.1</version>
47 </dependency>
48
49 <!--依赖关系-->
50 <dependency>
51 <groupId>com.motan</groupId>
52 <artifactId>motan-server</artifactId>
53 <version>1.0-SNAPSHOT</version>
54 </dependency>
55
56 <dependency>
57 <groupId>org.springframework.boot</groupId>
58 <artifactId>spring-boot-starter-test</artifactId>
59 </dependency>
60 </dependencies>
61 </project>
5.motan-server 结构
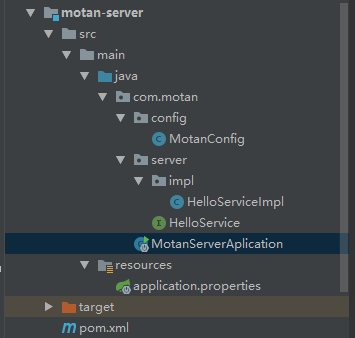
5.1 MotanConfig.class
1 package com.motan.config;
2
3 import com.weibo.api.motan.config.springsupport.*;
4 import org.springframework.beans.factory.annotation.Value;
5 import org.springframework.context.annotation.Bean;
6 import org.springframework.context.annotation.Configuration;
7
8 @Configuration
9 public class MotanConfig {
10
11 @Bean
12 public AnnotationBean motanAnnotationBean(@Value("${motan.annotation.package}") String packageName) {
13 AnnotationBean motanAnnotationBean = new AnnotationBean();
14 motanAnnotationBean.setPackage(packageName);
15 return motanAnnotationBean;
16 }
17
18 @Bean(name = "motan")
19 public ProtocolConfigBean protocolConfig() {
20 ProtocolConfigBean config = new ProtocolConfigBean();
21 config.setDefault(true);
22 config.setSerialization("hessian2");
23 config.setName("motan");
24 config.setMaxContentLength(1548576);
25 config.setRequestTimeout(50000000);
26
27 return config;
28 }
29
30 @Bean(name = "registry")
31 public RegistryConfigBean registryConfigSit(@Value("${motan.zookeeper.host}") String zookeeperAddress) {
32 RegistryConfigBean config = new RegistryConfigBean();
33 config.setDefault(true);
34 config.setRegProtocol("zookeeper");
35 config.setAddress(zookeeperAddress);
36 return config;
37 }
38
39 /**
40 * 服务端配置
41 */
42 @Bean(name = "motanServerBasicConfig")
43 public BasicServiceConfigBean baseServiceConfig(@Value("${motan.export.port}") String port) {
44 BasicServiceConfigBean config = new BasicServiceConfigBean();
45 config.setDefault(true);
46 config.setExport("motan:" + port);
47 config.setGroup("sinomall");
48 config.setAccessLog(false);
49 config.setShareChannel(true);
50 config.setRequestTimeout(9000000);
51 config.setRegistry("registry");
52 return config;
53 }
54
55 /**
56 * 客户端配置
57 */
58 @Bean(name = "motanClientBasicConfig")
59 public BasicRefererConfigBean baseRefererConfig() {
60 BasicRefererConfigBean config = new BasicRefererConfigBean();
61 config.setProtocol("motan");
62 config.setGroup("sinomall");
63 config.setAccessLog(false);
64 config.setRegistry("registry");
65 config.setCheck(false);
66 config.setRetries(0);
67 config.setThrowException(true);
68 config.setRequestTimeout(9000);
69 config.setDefault(true);
70 return config;
71 }
72 }
5.2 application.properties
1 # motan配置
2 motan.annotation.package=com.motan
3 motan.export.port=${random.int[5001,9999]}
4 motan.zookeeper.host=127.0.0.1:2181
5.3 HelloService.class
1 package com.motan.server;
2
3 /**
4 * @date 2018/5/28
5 */
6 public interface HelloService {
7 String hello();
8 }
5.4 HelloServiceImpl.class
1 package com.motan.server.impl;
2
3 import com.motan.server.HelloService;
4 import com.weibo.api.motan.config.springsupport.annotation.MotanService;
5
6 /**
7 * @date 2018/5/28
8 */
9 @MotanService
10 public class HelloServiceImpl implements HelloService {
11
12 @Override
13 public String hello() {
14 return "hello motan";
15 }
16 }
5.5 MotanServerAplication.class
1 package com.motan;
2
3 import com.weibo.api.motan.common.MotanConstants;
4 import com.weibo.api.motan.util.MotanSwitcherUtil;
5 import org.springframework.boot.SpringApplication;
6 import org.springframework.boot.autoconfigure.SpringBootApplication;
7
8 /**
9 * @date 2018/5/28
10 */
11 @SpringBootApplication
12 public class MotanServerAplication {
13 public static void main(String[] args) {
14 SpringApplication.run(MotanServerAplication.class, args);
15 //服务提供方需要开启心跳检测
16 MotanSwitcherUtil.setSwitcherValue(MotanConstants.REGISTRY_HEARTBEAT_SWITCHER, true);
17 System.out.println("服务端启动完成......");
18 }
19 }
6. motan-client 结构
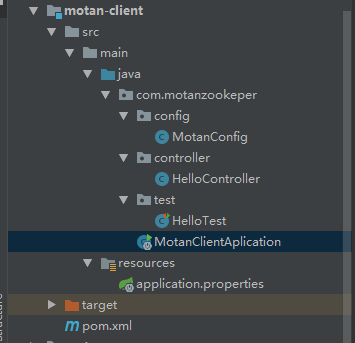
6.1 MotanConfig.class
1 package com.motanzookeper.config;
2
3 import com.weibo.api.motan.config.springsupport.*;
4 import org.springframework.beans.factory.annotation.Value;
5 import org.springframework.context.annotation.Bean;
6 import org.springframework.context.annotation.Configuration;
7
8 @Configuration
9 public class MotanConfig {
10
11 @Bean
12 public AnnotationBean motanAnnotationBean(@Value("${motan.annotation.package}") String packageName) {
13 AnnotationBean motanAnnotationBean = new AnnotationBean();
14 motanAnnotationBean.setPackage(packageName);
15 return motanAnnotationBean;
16 }
17
18 @Bean(name = "motan")
19 public ProtocolConfigBean protocolConfig() {
20 ProtocolConfigBean config = new ProtocolConfigBean();
21 config.setDefault(true);
22 config.setSerialization("hessian2");
23 config.setName("motan");
24 config.setMaxContentLength(1548576);
25 config.setRequestTimeout(50000000);
26
27 return config;
28 }
29
30 @Bean(name = "registry")
31 public RegistryConfigBean registryConfigSit(@Value("${motan.zookeeper.host}") String zookeeperAddress) {
32 RegistryConfigBean config = new RegistryConfigBean();
33 config.setDefault(true);
34 config.setRegProtocol("zookeeper");
35 config.setAddress(zookeeperAddress);
36 return config;
37 }
38
39 /**
40 * 服务端配置
41 */
42 @Bean(name = "motanServerBasicConfig")
43 public BasicServiceConfigBean baseServiceConfig(@Value("${motan.export.port}") String port) {
44 BasicServiceConfigBean config = new BasicServiceConfigBean();
45 config.setDefault(true);
46 config.setExport("motan:" + port);
47 config.setGroup("sinomall");
48 config.setAccessLog(false);
49 config.setShareChannel(true);
50 config.setRequestTimeout(9000000);
51 config.setRegistry("registry");
52 return config;
53 }
54
55 /**
56 * 客户端配置
57 */
58 @Bean(name = "motanClientBasicConfig")
59 public BasicRefererConfigBean baseRefererConfig() {
60 BasicRefererConfigBean config = new BasicRefererConfigBean();
61 config.setProtocol("motan");
62 config.setGroup("sinomall");
63 config.setAccessLog(false);
64 config.setRegistry("registry");
65 config.setCheck(false);
66 config.setRetries(0);
67 config.setThrowException(true);
68 config.setRequestTimeout(9000);
69 config.setDefault(true);
70 return config;
71 }
72 }
6.2 application.properties
1 server.port=7081
2 # motan配置
3 motan.annotation.package=com.motanzookeper
4 motan.export.port=${random.int[5001,9999]}
5 motan.zookeeper.host=127.0.0.1:2181
6.3 HelloTest.class
1 package com.motanzookeper.test;
2
3 import com.motan.server.HelloService;
4 import com.motanzookeper.MotanClientAplication;
5 import com.weibo.api.motan.config.springsupport.annotation.MotanReferer;
6 import org.junit.Test;
7 import org.junit.runner.RunWith;
8 import org.springframework.boot.test.context.SpringBootTest;
9 import org.springframework.test.context.junit4.SpringRunner;
10
11 @SpringBootTest(classes = MotanClientAplication.class)
12 @RunWith(SpringRunner.class)
13 public class HelloTest {
14
15 @MotanReferer
16 private HelloService helloService;
17
18 @Test
19 public void addUsers() {
20 System.out.println("helloService:" + helloService);
21 System.out.println(helloService.hello());
22 }
23 }
6.4 MotanClientAplication.class
1 package com.motanzookeper;
2
3 import org.springframework.boot.SpringApplication;
4 import org.springframework.boot.autoconfigure.SpringBootApplication;
5
6 /**
7 * @date 2018/5/28
8 */
9 @SpringBootApplication
10 public class MotanClientAplication {
11 public static void main(String[] args) {
12 SpringApplication.run(MotanClientAplication.class,args);
13 System.out.println("客户端启动完成......");
14 }
15 }
7.使用步骤
① 先在本地启动zookeper
② 启动MotanServerAplication.class
③ 启动MotanClientAplication.class
测试结果
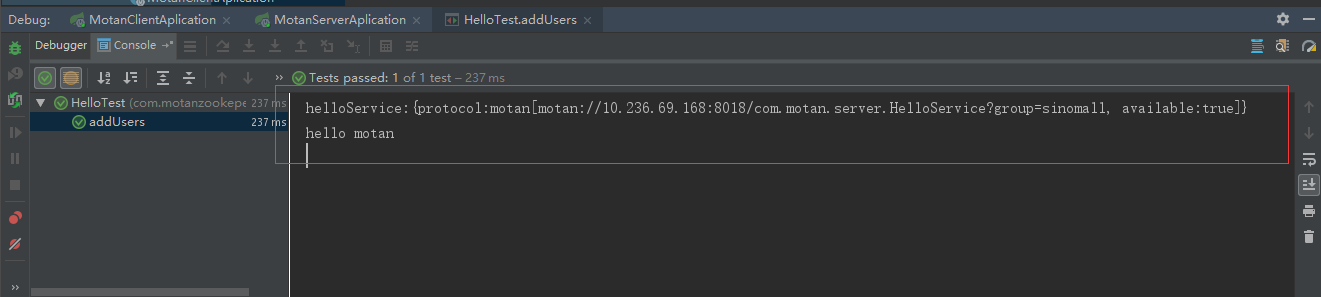