本文笔者在深圳吃饭的时候突然想到的...这段时间就有想写几篇关于线段覆盖的笔记,所以回家到之后就奋笔疾书的写出来发表了
Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions: 9733 | Accepted: 2566 |
Description
You are to write a program that has to decide whether a given line segment intersects a given rectangle.
An example:
line: start point: (4,9)
end point: (11,2)
rectangle: left-top: (1,5)
right-bottom: (7,1)
Figure 1: Line segment does not intersect rectangle
The line is said to intersect the rectangle if the line and the rectangle have at least one point in common. The rectangle consists of four straight lines and the area in between. Although all input values are integer numbers, valid intersection points do not have to lay on the integer grid.
An example:
line: start point: (4,9)
end point: (11,2)
rectangle: left-top: (1,5)
right-bottom: (7,1)
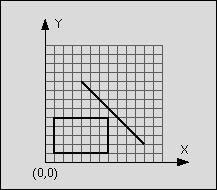
Figure 1: Line segment does not intersect rectangle
The line is said to intersect the rectangle if the line and the rectangle have at least one point in common. The rectangle consists of four straight lines and the area in between. Although all input values are integer numbers, valid intersection points do not have to lay on the integer grid.
Input
The input consists of n test cases. The first line of the input file contains the number n. Each following line contains one test case of the format:
xstart ystart xend yend xleft ytop xright ybottom
where (xstart, ystart) is the start and (xend, yend) the end point of the line and (xleft, ytop) the top left and (xright, ybottom) the bottom right corner of the rectangle. The eight numbers are separated by a blank. The terms top left and bottom right do not imply any ordering of coordinates.
xstart ystart xend yend xleft ytop xright ybottom
where (xstart, ystart) is the start and (xend, yend) the end point of the line and (xleft, ytop) the top left and (xright, ybottom) the bottom right corner of the rectangle. The eight numbers are separated by a blank. The terms top left and bottom right do not imply any ordering of coordinates.
Output
For each test case in the input file, the output file should contain a line consisting either of the letter "T" if the line segment intersects the rectangle or the letter "F" if the line segment does not intersect the rectangle.
Sample Input
1 4 9 11 2 1 5 7 1
Sample Output
F
Source
Southwestern European Regional Contest 1995
考虑:线段在矩形内也算订交,开始忘考虑一直情况,两个线段订交,也可能其中一条线段完整覆盖另一个线段,所以判断的时候要互相的判断点时候在线段内。
考虑:线段在矩形内也算订交,开始忘考虑一直情况,两个线段订交,也可能其中一条线段完整覆盖另一个线段,所以判断的时候要互相的判断点时候在线段内。
#include <iostream> #include <cstdio> #include <cstdlib> #include <algorithm> #include <vector> #include <cmath> using namespace std; const double eps = 1e-8; struct point { int x; int y; }; struct line { point start; point endx; }; int dcmp(double x) { if(fabs(x)<eps) return 0; else return x<0 ? -1 : 1; } double det(const point p1, const point p2, const point p0) { return (p1.x-p0.x)*(p2.y-p0.y)-(p2.x-p0.x)*(p1.y-p0.y); } bool PointOnSegment(const line a, point p) { if((dcmp(det(a.start, a.endx, p))==0) && ((a.start.x-p.x)*(a.endx.x-p.x)+(a.start.y-p.y)*(a.endx.y-p.y))<=0) return true; return false; } bool Point_in_rectangle(int x1, int x2, int y1, int y2, line a) { if((a.start.x>=x1 && a.start.x <=x2 && a.start.y>=y1 && a.start.y<=y2)&& a.endx.x>=x1 && a.endx.x<=x2 && a.endx.y>=y1 && a.endx.y<=y2) return true; return false; } bool ok(const line a, const line b) { int flag1 = 0, flag2 = 0; if(max(a.start.x, a.endx.x)>=min(b.start.x, b.endx.x) && max(b.start.x, b.endx.x)>=min(a.start.x, a.endx.x) && max(a.start.y, a.endx.y)>=min(b.start.y, b.endx.y) && max(b.start.y, b.endx.y)>=min(a.start.y, a.endx.y) && det(b.start, a.endx, a.start)*det(a.endx, b.endx,a.start)> 0 && det(a.start, b.endx, b.start)*det(b.endx, a.endx, b.start)> 0 ) flag1 = 1; if(PointOnSegment(a, b.endx)||PointOnSegment(a, b.start) ||PointOnSegment(b, a.endx) || PointOnSegment(b, a.start) ) flag2 = 1; if(flag1 || flag2) return true; return false; } int main() { //freopen("d:\\in.txt","r",stdin); //freopen("d:\\out.txt","w",stdout); int n; int xstart, ystart, xend, yend; int xleft, ytop, xright, ybottom; line a, b, c, d; line t; bool flag = false; scanf("%d", &n); int tmp1, tmp2, tmp3, tmp4; while(n--) { flag = false; scanf("%d%d%d%d", &xstart, &ystart, &xend, ¥d); scanf("%d%d%d%d", &xleft, &ytop, &xright, &ybottom); t.start.x = xstart; t.start.y = ystart; t.endx.x = xend; t.endx.y = yend; tmp1 = min(xleft, xright); tmp2 = max(xleft, xright); tmp3 = min(ytop, ybottom); tmp4 = max(ytop, ybottom); a.start.x = a.endx.x = c.start.x = b.endx.x = tmp1; //xleft; a.start.y = b.start.y = b.endx.y = d.endx.y = tmp4;//ytop; a.endx.y = d.start.y = c.start.y = c.endx.y = tmp3;//ybottom; b.start.x = c.endx.x = d.start.x = d.endx.x = tmp2;//xright; if(Point_in_rectangle(tmp1, tmp2, tmp3, tmp4, t)) flag = true; if(ok(a,t)) flag = true; if(ok(b,t)) flag = true; if(ok(c,t)) flag = true; if(ok(d,t)) flag = true; if(flag){ printf("T\n"); } else { printf("F\n"); } } return 0; }
文章结束给大家分享下程序员的一些笑话语录:
女人篇
有的女人就是Windows虽然很优秀,但是安全隐患太大。
有的女人就是MFC她条件很好,然而不是谁都能玩的起。
有的女人就是C#长的很漂亮,但是家务活不行。
有的女人就是C++,她会默默的为你做很多的事情。
有的女人就是汇编虽然很麻烦,但是有的时候还得求它。
有的女人就是SQL,她会为你的发展带来莫大的帮助。
---------------------------------
原创文章 By
线段和覆盖
---------------------------------