request.js
import axios from 'axios'
// config:就是请求对象,打印出来,可以看到包括 headers 等项
export function request(config) {
// 1.创建axios的实例
const instance = axios.create({
baseURL: 'http://123.207.32.32:8000',
timeout: 5000
})
// 2.axios的拦截器
// 2.1.请求拦截的作用
instance.interceptors.request.use(config => {
// console.log(config);
// 1.比如config中的一些信息不符合服务器的要求
// 2.比如每次发送网络请求时, 都希望在界面中显示一个请求的图标
// 3.某些网络请求(比如登录(token)), 必须携带一些特殊的信息
return config // 必须要把config返回给调用者
}, err => {
// console.log(err);
})
// 2.2.响应拦截
instance.interceptors.response.use(res => {
// console.log(res);
return res.data // 必须要把res.data返回给调用者
}, err => {
console.log(err);
})
// 3.发送真正的网络请求 【instance是promise实例,执行instance函数,返回的是promise】
return instance(config)
}
// export function request(config) {
// return new Promise((resolve, reject) => {
// // 1.创建axios的实例
// const instance = axios.create({
// baseURL: 'http://123.207.32.32:8000',
// timeout: 5000
// })
//
// // 发送真正的网络请求
// instance(config)
// .then(res => {
// resolve(res)
// })
// .catch(err => {
// reject(err)
// })
// })
// }
// export function request(config) {
// // 1.创建axios的实例
// const instance = axios.create({
// baseURL: 'http://123.207.32.32:8000',
// timeout: 5000
// })
//
// // 发送真正的网络请求
// instance(config.baseConfig)
// .then(res => {
// // console.log(res);
// config.success(res);
// })
// .catch(err => {
// // console.log(err);
// config.failure(err)
// })
// }
// export function request(config, success, failure) {
// // 1.创建axios的实例
// const instance = axios.create({
// baseURL: 'http://123.207.32.32:8000',
// timeout: 5000
// })
//
// // 发送真正的网络请求
// instance(config)
// .then(res => {
// // console.log(res);
// success(res);
// })
// .catch(err => {
// // console.log(err);
// failure(err)
// })
// }
// function test(aaa, bbb) {
// // aaa('Hello World')
// bbb('err message')
// }
//
// test(function (res) {
// console.log(res);
// }, function (err) {
// console.log(err);
// })
mian.js
import Vue from 'vue'
import App from './App'
import axios from 'axios'
Vue.config.productionTip = false
new Vue({
el: '#app',
render: h => h(App)
})
// const obj = {
// name: 'kobe',
// age: 30
// }
//
// const {name, age} = obj;
//
// const names = ['why', 'kobe', 'james']
// // const name1 = names[0]
// // const name2 = names[1]
// // const name3 = names[2]
// const [name1, name2, name3] = names;
// 1.axios的基本使用
axios({
url: 'http://123.207.32.32:8000/home/multidata',
// method: 'post'
}).then(res => {
console.log(res);
})
axios({
url: 'http://123.207.32.32:8000/home/data',
// 专门针对get请求的参数拼接
params: {
type: 'pop',
page: 1
}
}).then(res => {
console.log(res);
})
// ------------------------
// 2.axios发送并发请求
axios.all([axios({
url: 'http://123.207.32.32:8000/home/multidata'
}), axios({
url: 'http://123.207.32.32:8000/home/data',
params: {
type: 'sell',
page: 5
}
})]).then(results => {
console.log(results);
console.log(results[0]);
console.log(results[1]);
})
// ------------------------
// 3.使用全局的axios 和 对应的配置在进行网络请求
axios.defaults.baseURL = 'http://123.207.32.32:8000'
axios.defaults.timeout = 5000
axios.all([axios({
url: '/home/multidata'
}), axios({
url: '/home/data',
params: {
type: 'sell',
page: 5
}
})]).then(axios.spread((res1, res2) => {
console.log(res1);
console.log(res2);
}))
// axios.defaults.baseURL = 'http://222.111.33.33:8000'
// axios.defaults.timeout = 10000
axios({
url: 'http://123.207.32.32:8000/category'
})
// ------------------------
// 4.创建对应的axios的实例
const instance1 = axios.create({
baseURL: 'http://123.207.32.32:8000',
timeout: 5000
})
instance1({
url: '/home/multidata'
}).then(res => {
console.log(res);
})
instance1({
url: '/home/data',
params: {
type: 'pop',
page: 1
}
}).then(res => {
console.log(res);
})
const instance2 = axios.create({
baseURL: 'http://222.111.33.33:8000',
timeout: 10000,
// headers: {}
})
// ------------------------
// 5.封装request模块
import { request } from "./network/request";
request({
url: '/home/multidata'
}, res => {
console.log(res);
}, err => {
console.log(err);
})
request({
baseConfig: {},
success: function (res) { },
failure: function (err) { }
})
request({
url: '/home/multidata'
}).then(res => {
console.log(res);
}).catch(err => {
// console.log(err);
})
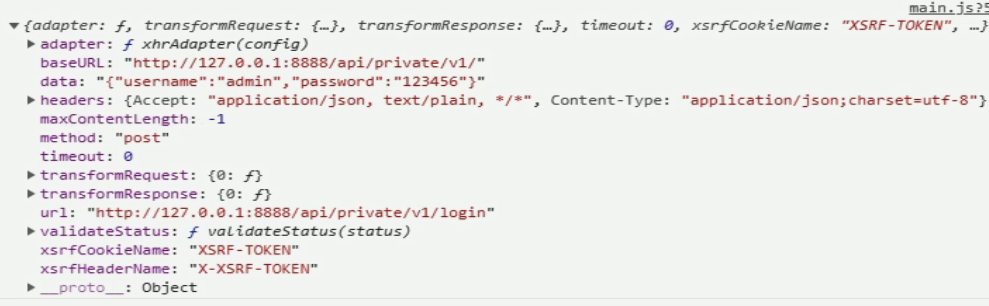
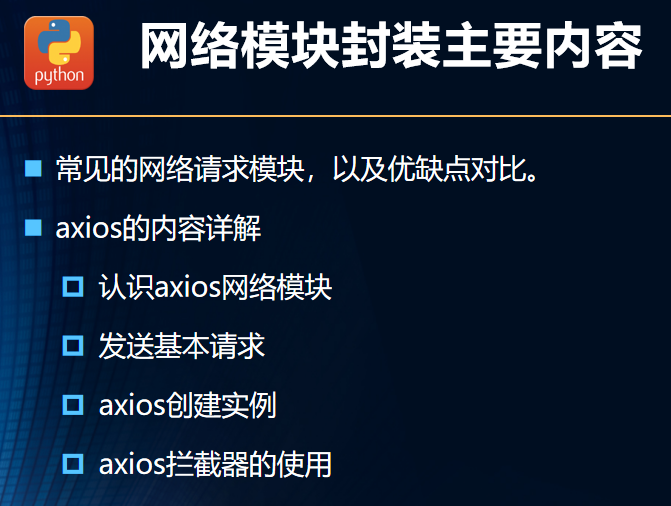
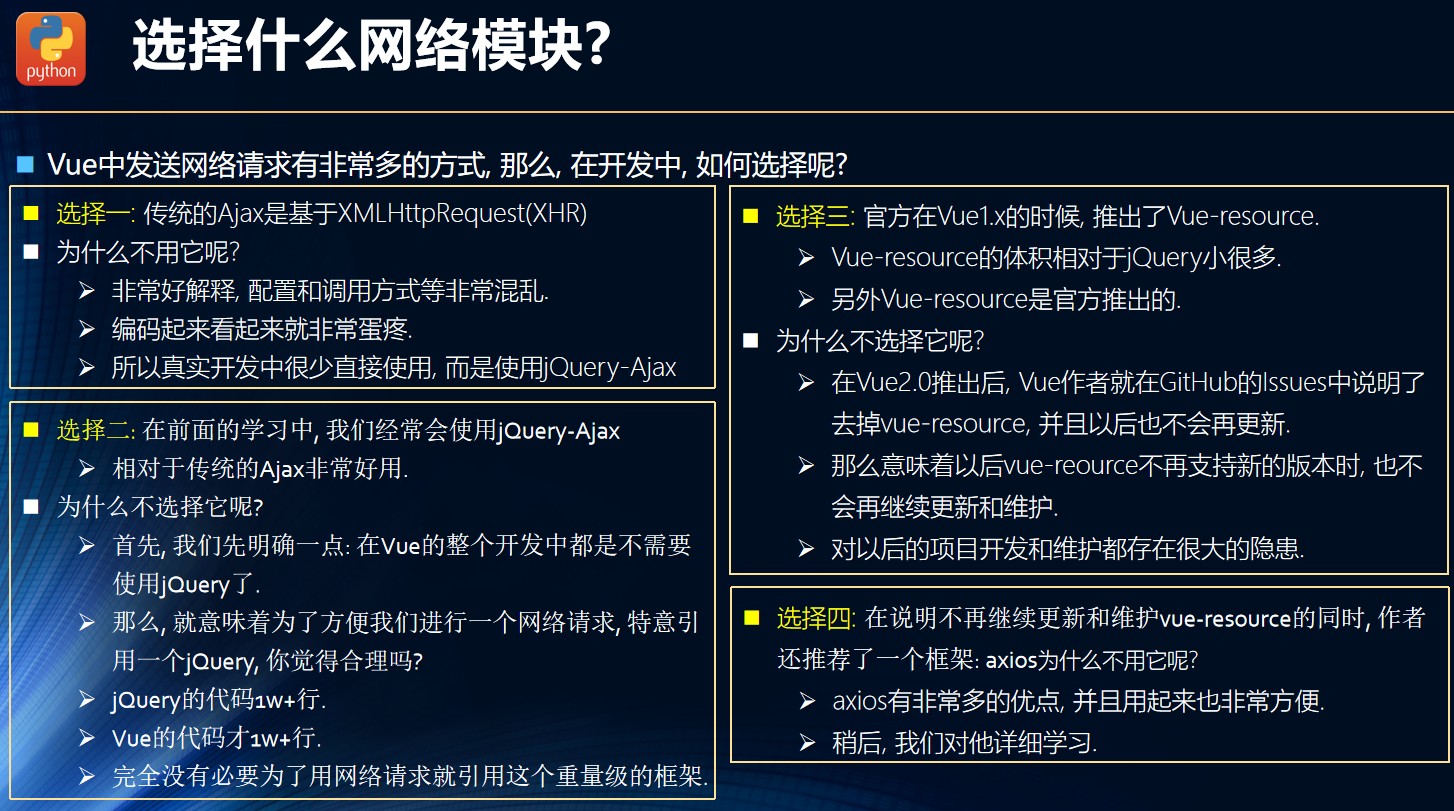
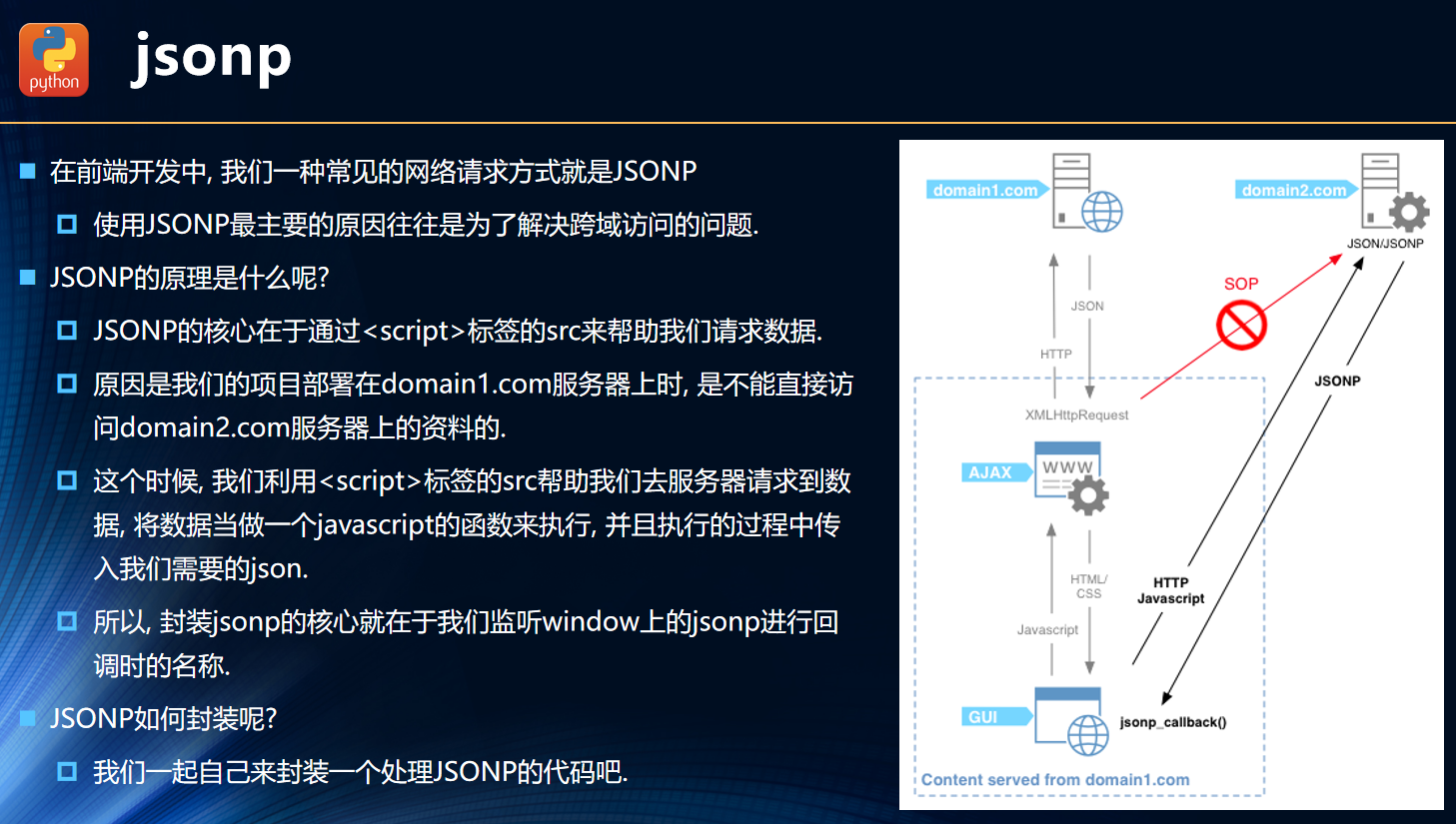
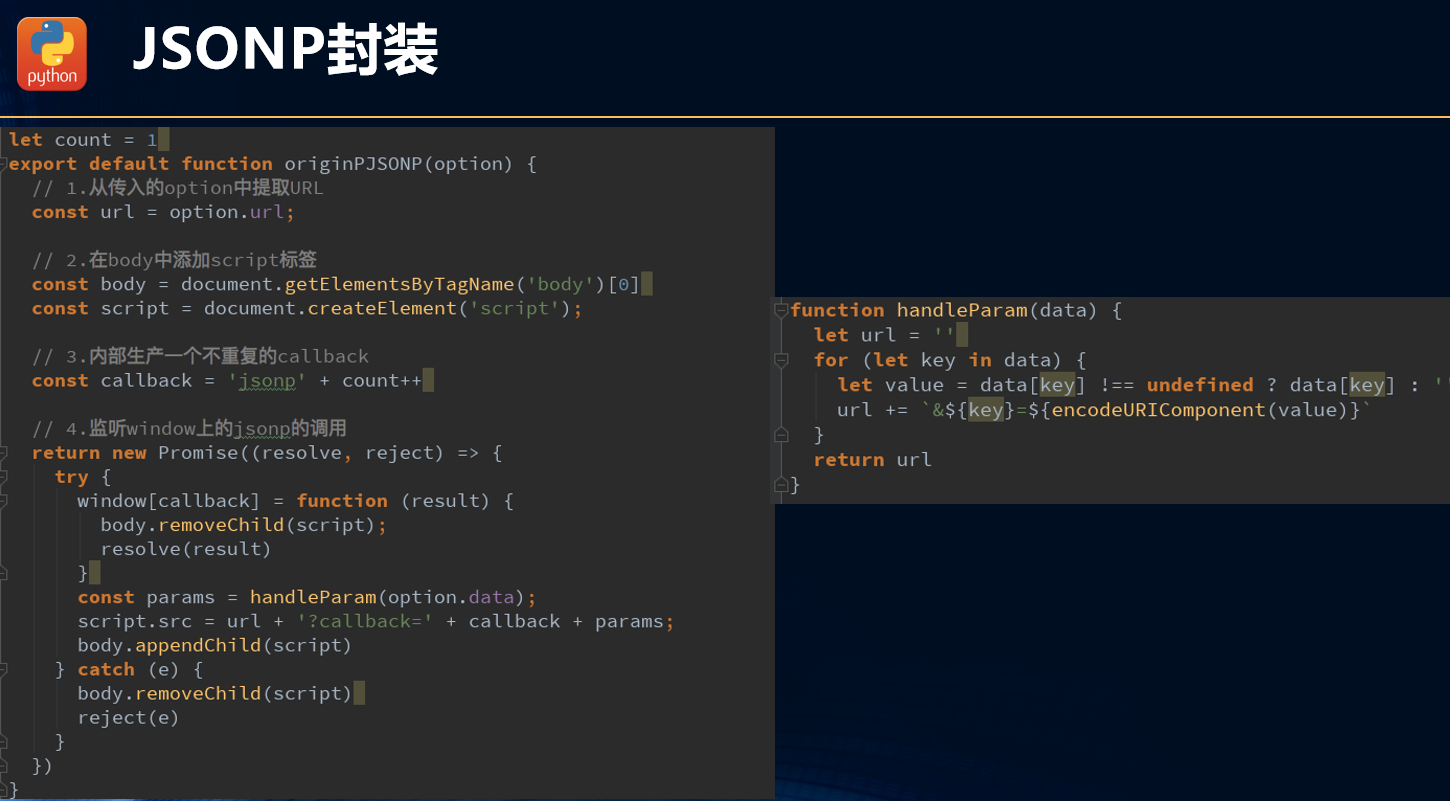
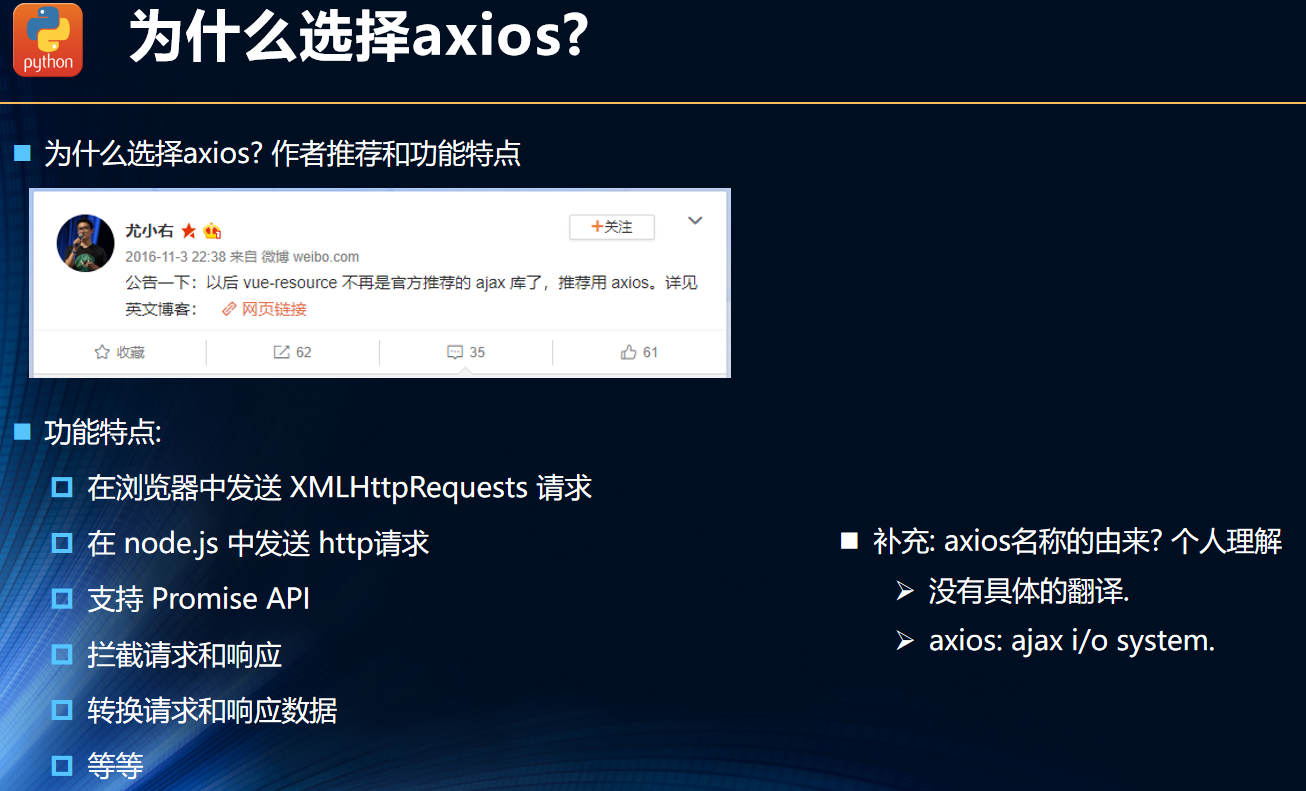

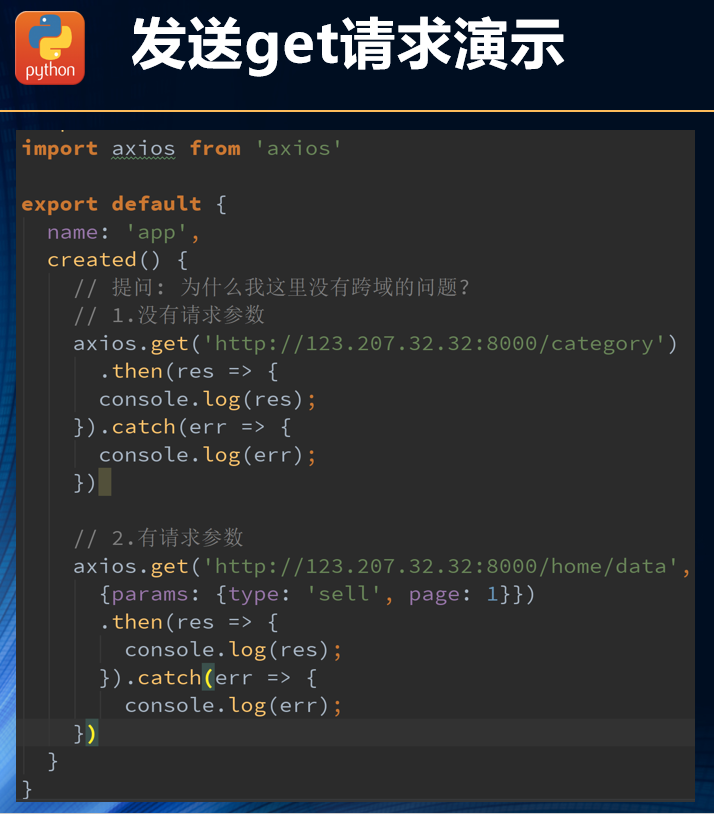
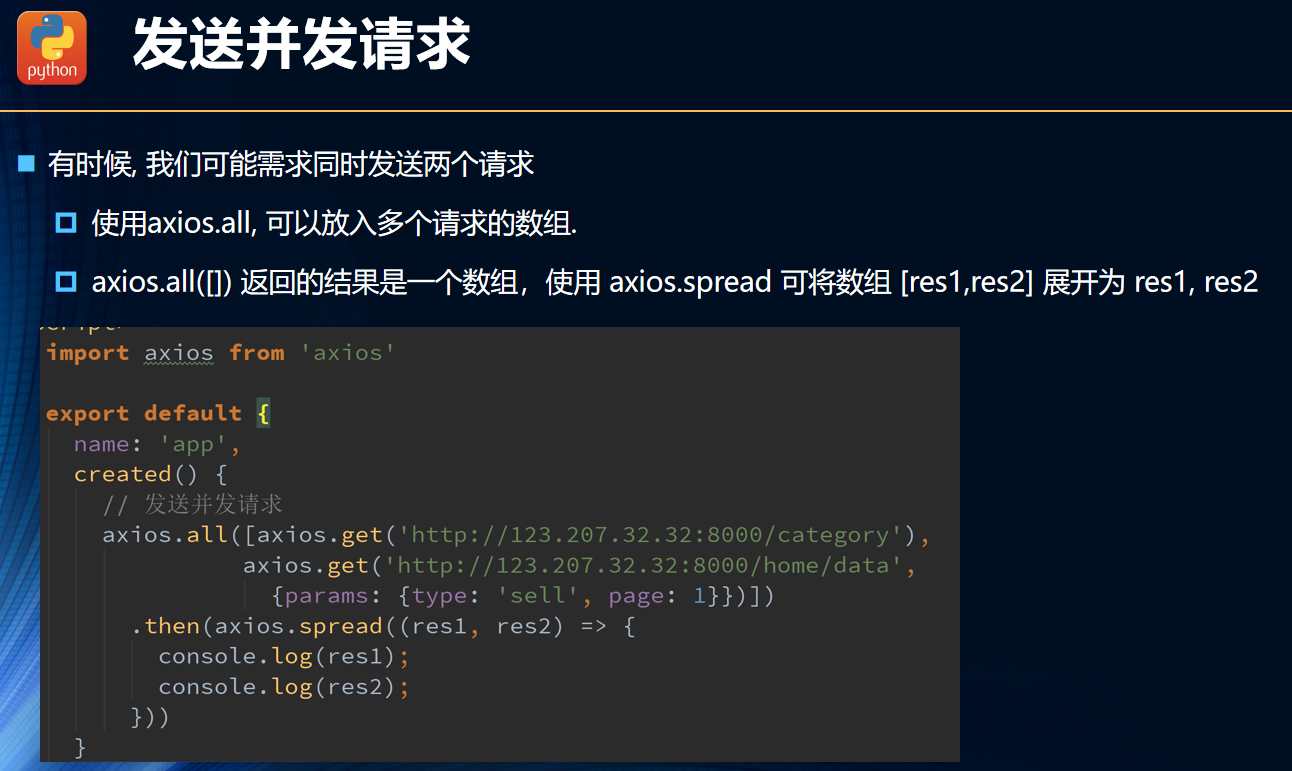
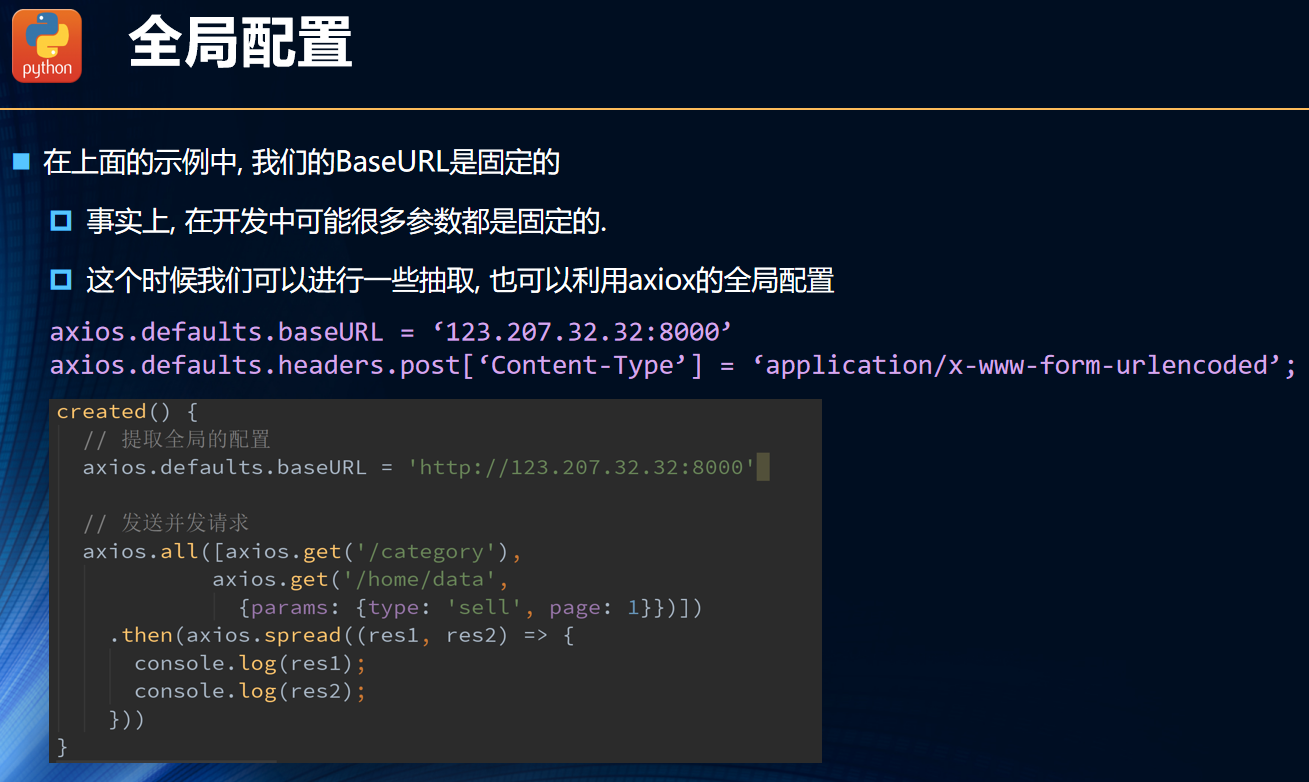
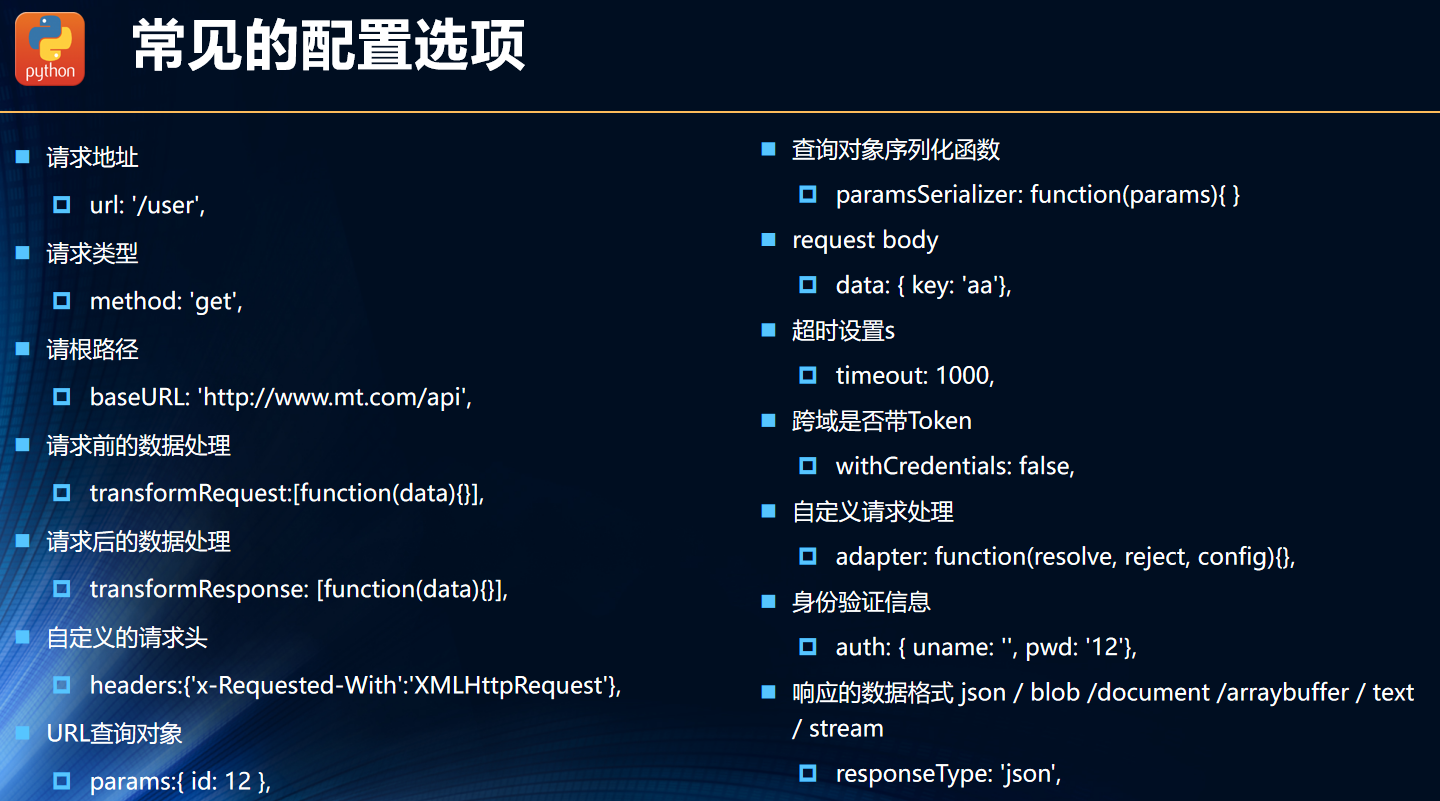
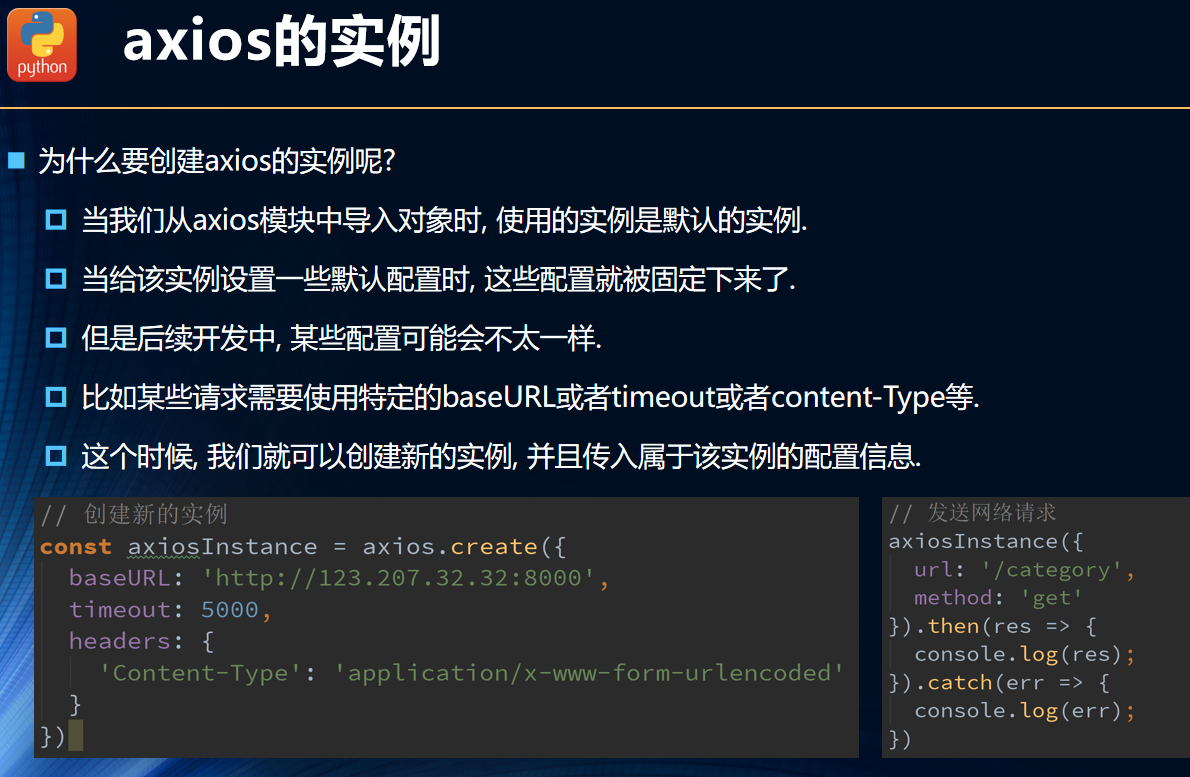
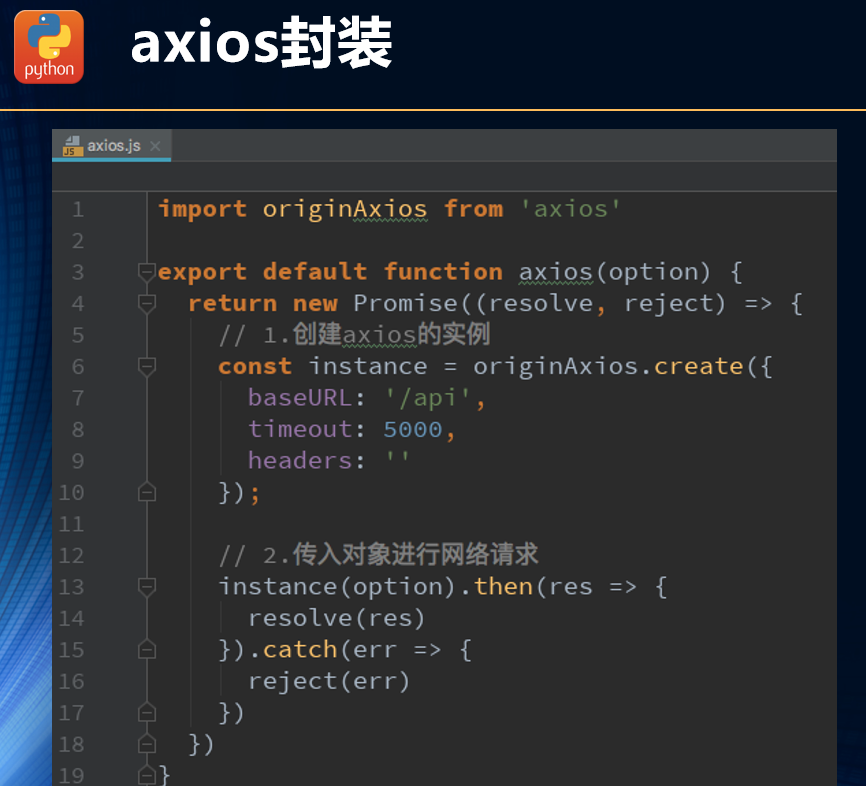
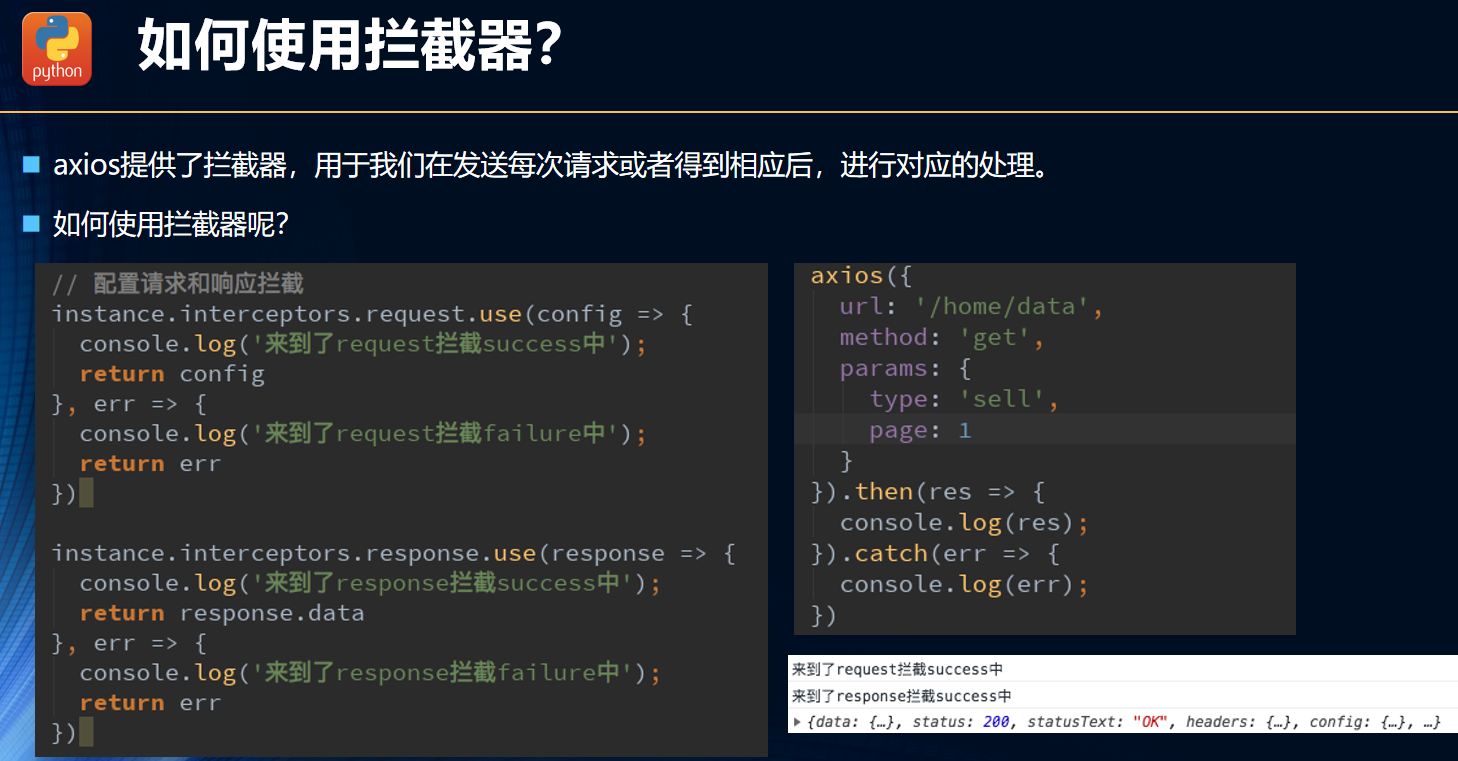
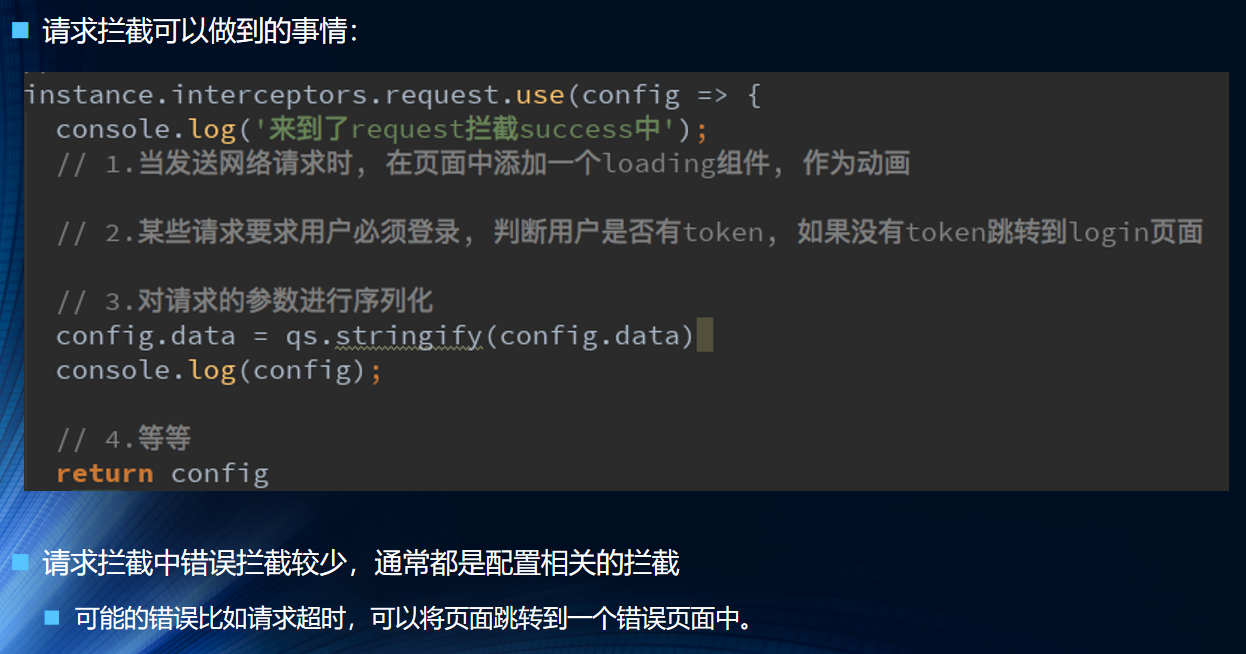
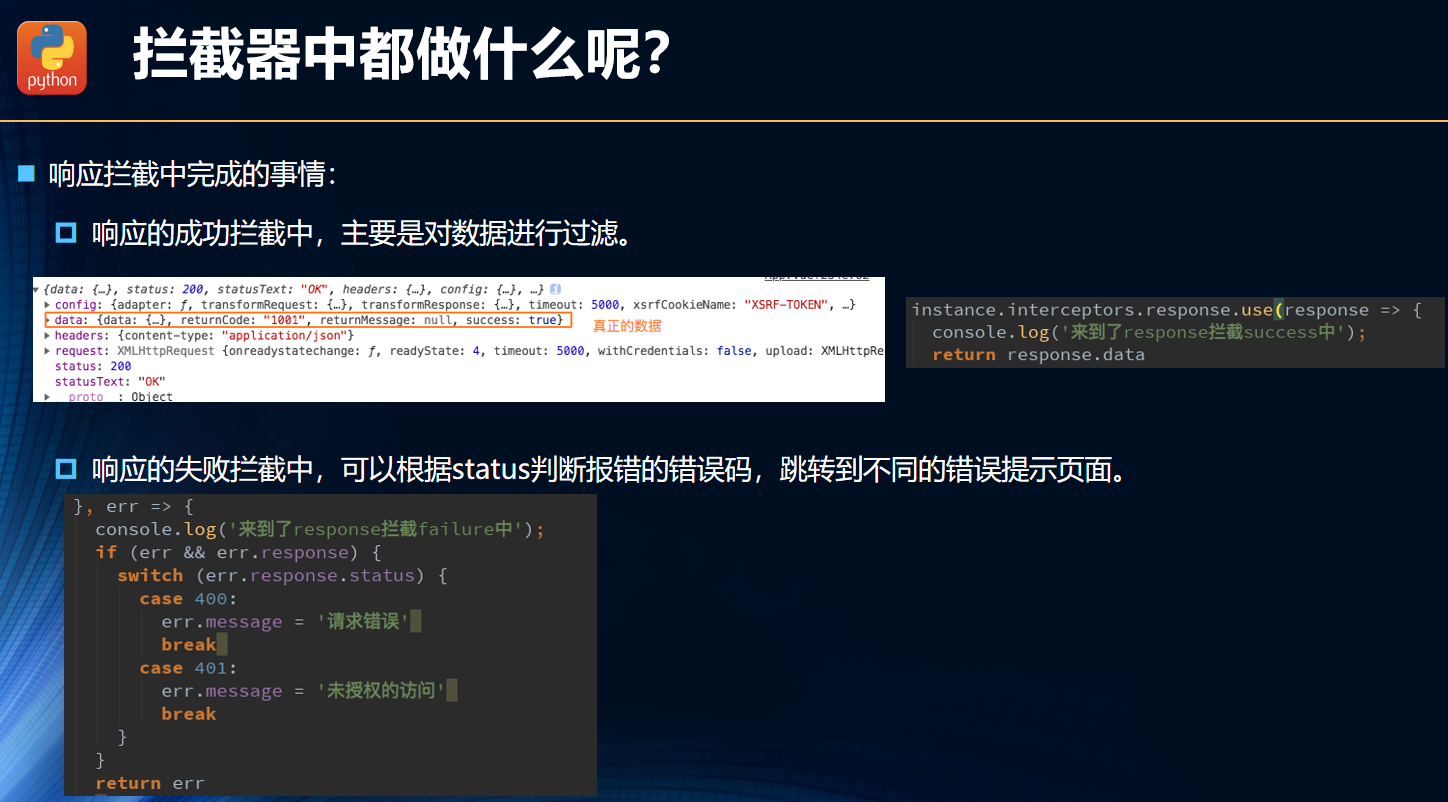