认识类的使用
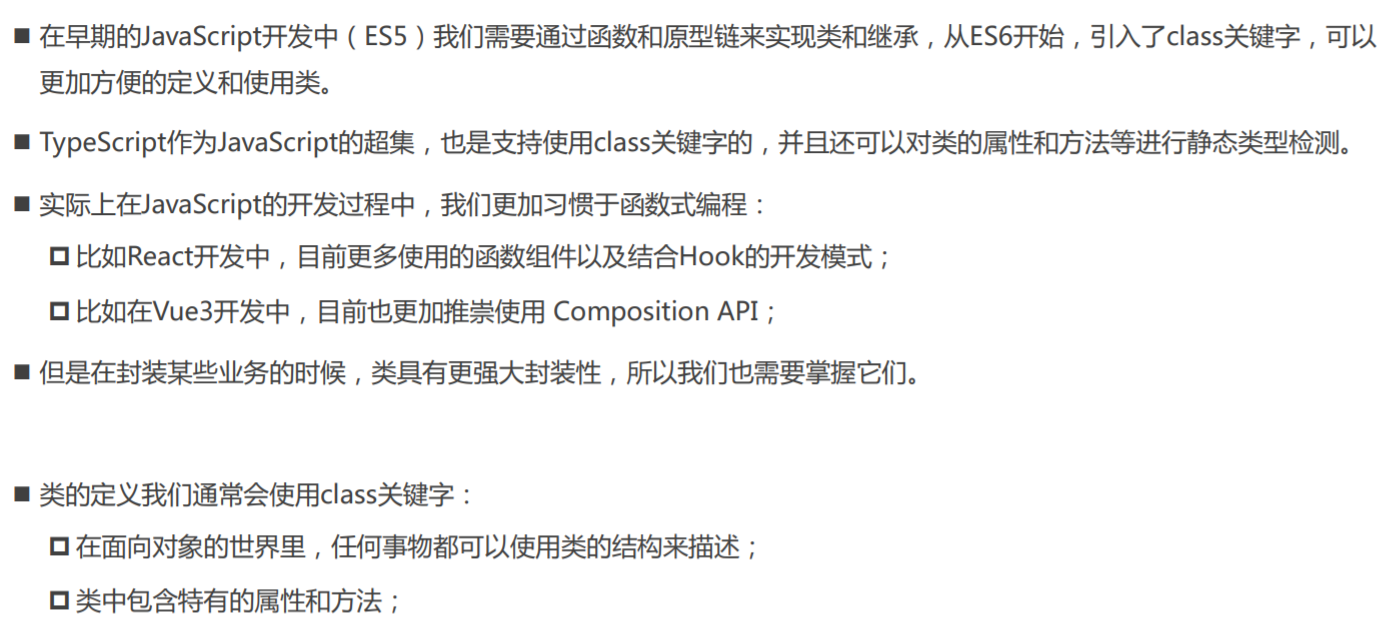
类的定义
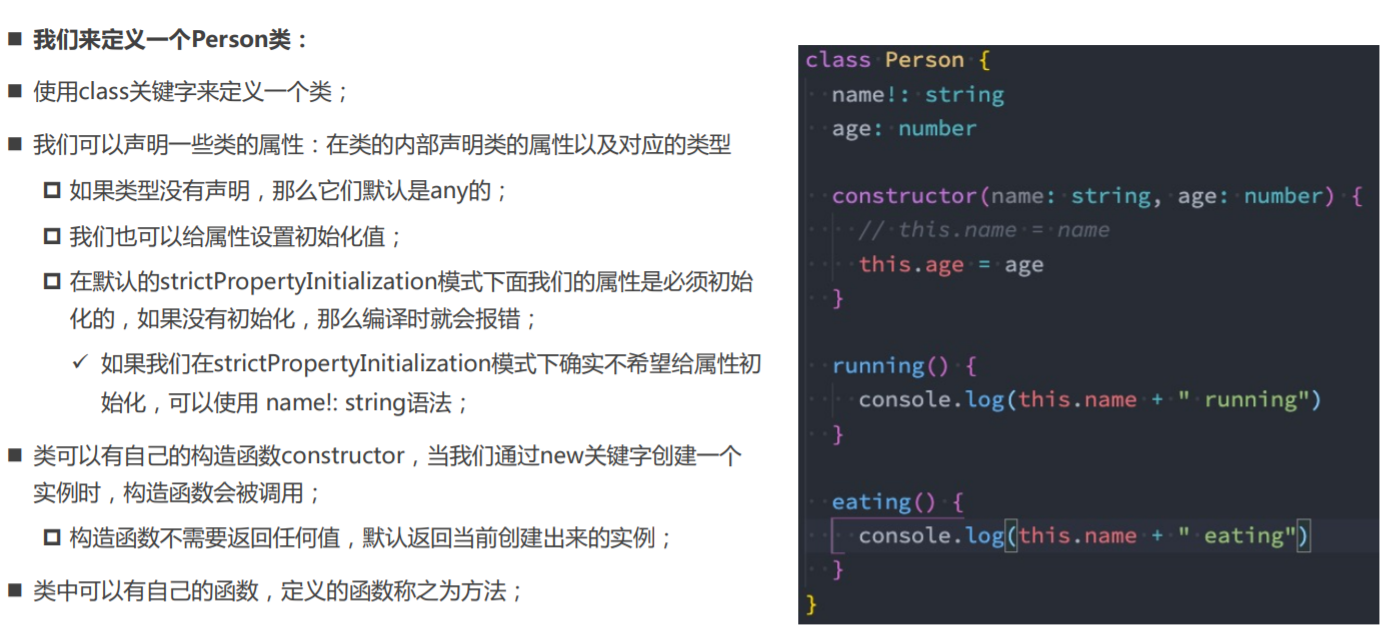
类的继承
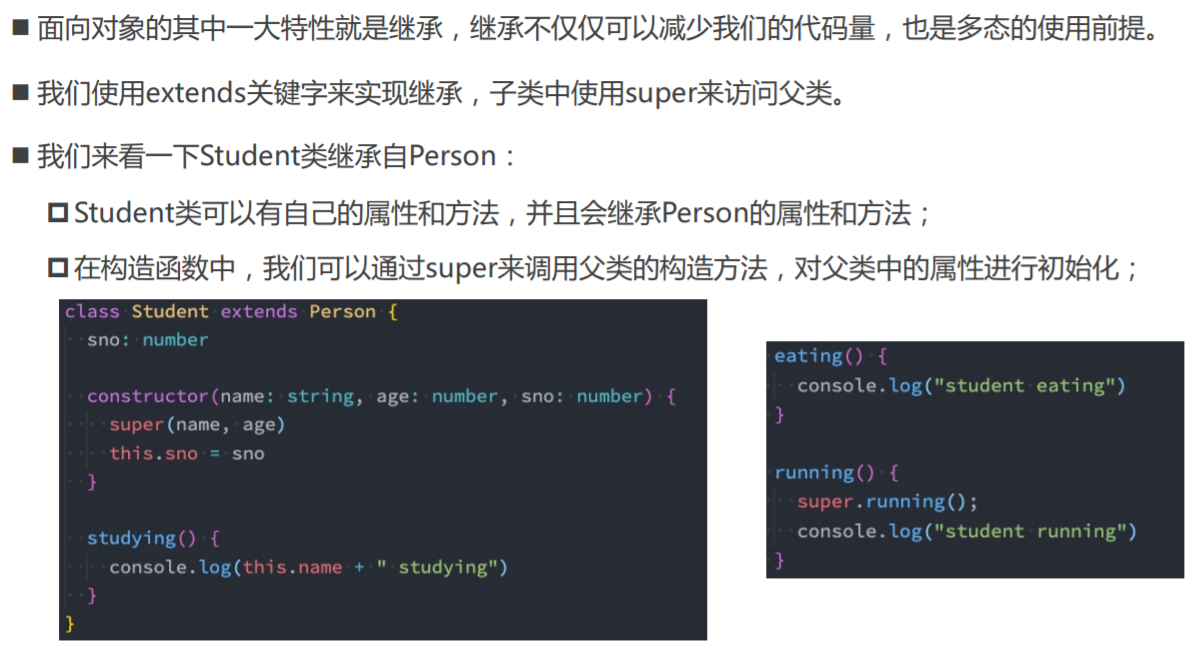
类的成员修饰符
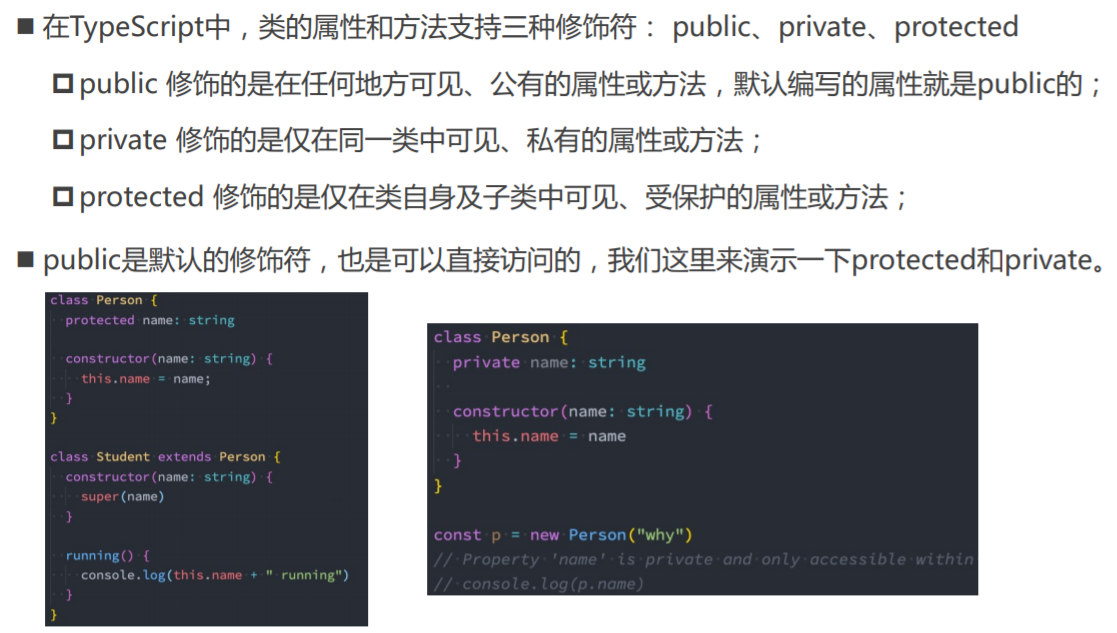
只读属性readonly
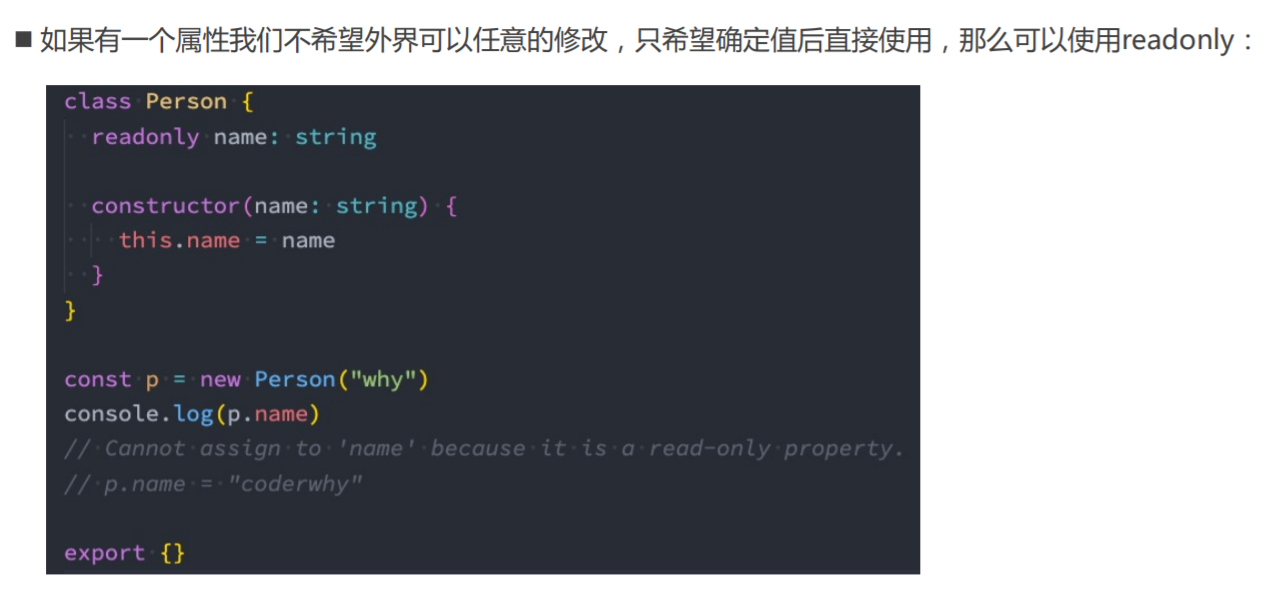
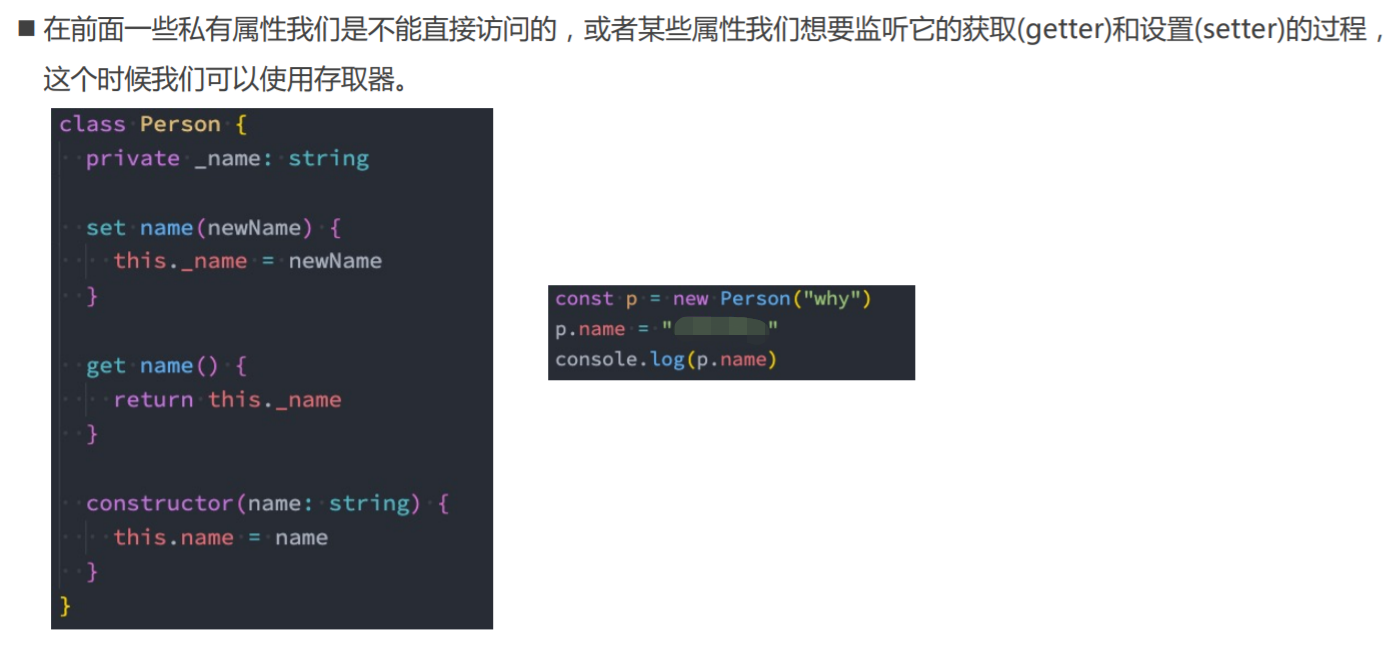
静态成员
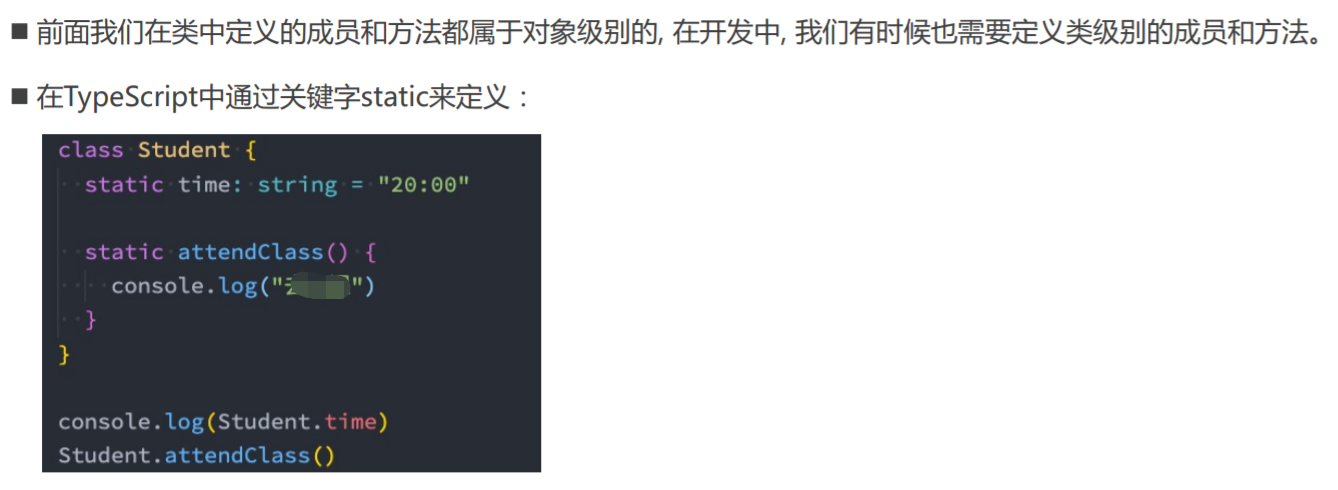
抽象类abstract
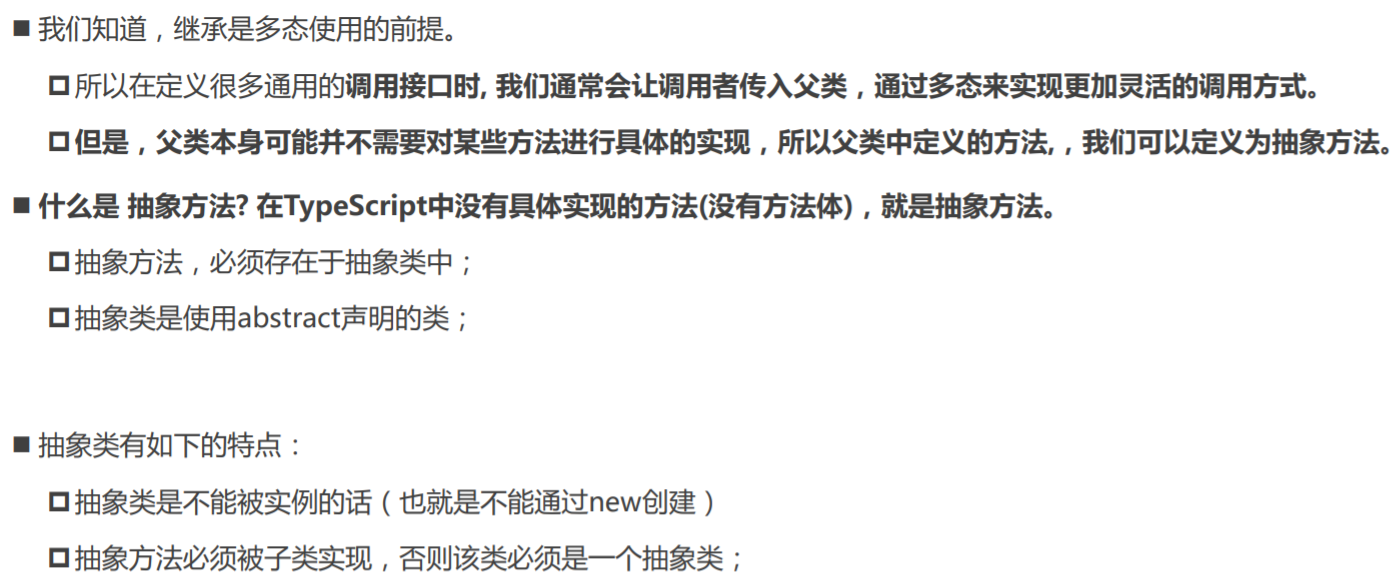
抽象类演练
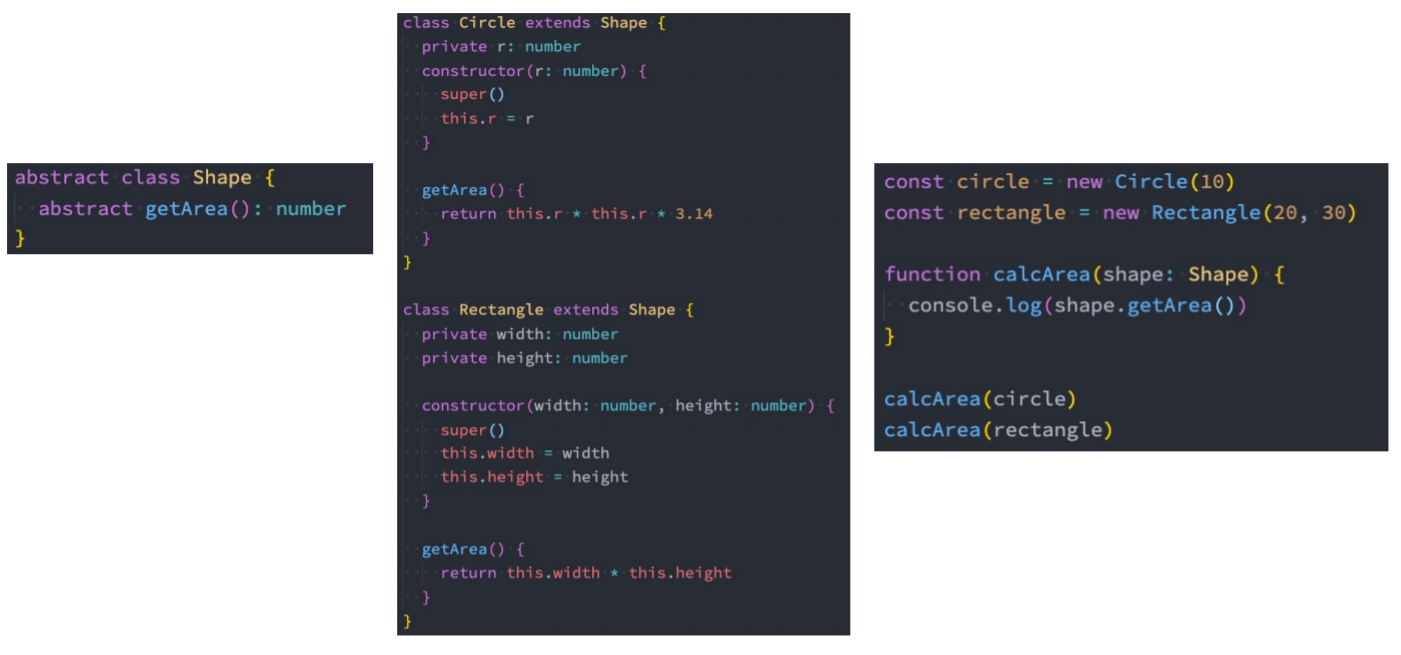
类的类型
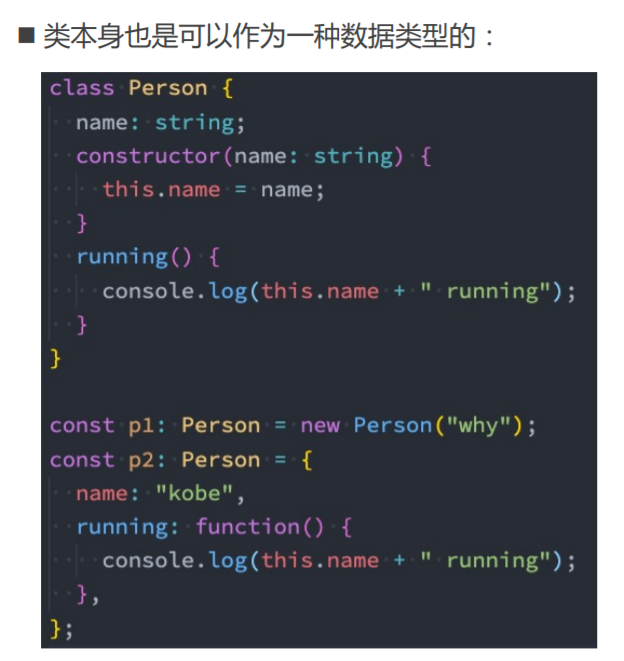
01_类的定义.ts
class Person {
name: string
age: number
constructor(name: string, age: number) {
this.name = name
this.age = age
}
eating() {
console.log(this.name + ' eating')
}
}
const p = new Person('why', 18)
console.log(p.name)
console.log(p.age)
p.eating()
export {}
02_类的继承.ts
class Person {
// 【记得初始化赋值。】
name: string = ''
age: number = 0
eating() {
console.log('eating')
}
}
class Student extends Person {
sno: number = 0
studying() {
console.log('studying')
}
}
class Teacher extends Person {
title: string = ''
teaching() {
console.log('teaching')
}
}
const stu = new Student()
stu.name = 'hahaha'
stu.age = 10
console.log(stu.name)
console.log(stu.age)
stu.eating()
03_类的继承2.ts
class Person {
name: string
age: number
constructor(name: string, age: number) {
this.name = name
this.age = age
}
eating() {
console.log('eating 100行')
}
}
class Student extends Person {
sno: number
constructor(name: string, age: number, sno: number) {
// super调用父类的构造器constructor
super(name, age)
this.sno = sno
}
// 【重写父类的方法。】
eating() {
console.log('student eating')
super.eating() // 【重写的同时,还可以调用父类的eating方法。】
}
studying() {
console.log('studying')
}
}
const stu = new Student('why', 18, 111)
console.log(stu.name)
console.log(stu.age)
console.log(stu.sno)
stu.eating()
export {}
04_类的多态.ts
class Animal {
action() {
console.log('animal action')
}
}
class Dog extends Animal {
action() {
console.log('dog running!!!')
}
}
class Fish extends Animal {
action() {
console.log('fish swimming')
}
}
class Person extends Animal {}
// animal: dog/fish
// 多态的目的是为了写出更加具备通用性的代码
function makeActions(animals: Animal[]) {
animals.forEach(animal => {
animal.action()
})
}
makeActions([new Dog(), new Fish(), new Person()])
export {}
05_成员的修饰符-private.ts
class Person {
private name: string = ''
// 封装了两个方法, 通过方法来访问name
getName() {
return this.name
}
setName(newName: string) {
this.name = newName
}
}
const p = new Person()
console.log(p.getName())
p.setName('why')
export {}
06_成员的修饰符-protected.ts
// protected: 在类内部和子类中可以访问
class Person {
protected name: string = '123'
}
class Student extends Person {
getName() {
return this.name
}
}
const stu = new Student()
console.log(stu.getName())
export {}
07_属性的只读-readonly.ts
class Person {
// 1.只读属性是可以在构造器中赋值, 赋值之后就不可以修改
// 2.属性本身不能进行修改, 但是如果它是对象类型, 对象中的属性是可以修改
readonly name: string
age?: number
readonly friend?: Person
constructor(name: string, friend?: Person) {
this.name = name
this.friend = friend
}
}
const p = new Person('why', new Person('kobe'))
console.log(p.name)
console.log(p.friend)
// 不可以直接修改friend
// p.friend = new Person("james")
if (p.friend) {
p.friend.age = 30
}
// p.name = "123"
08_getters-setter.ts
class Person {
private _name: string // 规范:一般私有属性前会加_
constructor(name: string) {
this._name = name
}
// 访问器setter/getter 【其实不是函数。】
// setter
set name(newName) {
this._name = newName
}
// getter
get name() {
return this._name
}
}
const p = new Person('why')
p.name = 'hahaha'
console.log(p.name)
export {}
09_类的静态成员.ts
// class Person {
// name: string = ""
// age: number = 12
// }
// const p = new Person()
// p.name = "123"
class Student {
static time: string = '20:00'
static attendClass() {
console.log('去学习~')
}
}
console.log(Student.time)
Student.attendClass()
10_抽象类abstract.ts
function makeArea(shape: Shape) {
return shape.getArea()
}
abstract class Shape {
// 【1、函数不应该有实现的时候,应该把函数定义为抽象函数。 2、抽象函数可以没有函数体。3、抽象函数要定义在抽象类里。 4、抽象类不能被实例化。 5、抽象类里的方法必须被子类实现,否则子类也要写成抽象类。】
abstract getArea() // 【编译失败,应该在getArea()后加 : number。】
}
class Rectangle extends Shape {
private number
private height: number
constructor( number, height: number) {
super() // 【即使父类没有属性,也要调用super。】
this.width = width
this.height = height
}
getArea() {
return this.width * this.height
}
}
class Circle extends Shape {
private r: number
constructor(r: number) {
super()
this.r = r
}
getArea() {
return this.r * this.r * 3.14
}
}
const rectangle = new Rectangle(20, 30)
const circle = new Circle(10)
console.log(makeArea(rectangle))
console.log(makeArea(circle))
// makeArea(new Shape()) // 【抽象类不能被实例化。】
// makeArea(123)
// makeArea("123")
11_类的类型.ts
class Person {
name: string = '123'
eating() {}
}
const p = new Person()
const p1: Person = {
name: 'why',
eating() {},
}
function printPerson(p: Person) {
console.log(p.name)
}
printPerson(new Person())
printPerson({
name: 'kobe',
eating: function () {},
})
export {}