Reversing Linked List
Given a constant K and a singly linked list L, you are supposed to reverse the links of every K elements on L. For example, given L being 1→2→3→4→5→6, if K=3, then you must output 3→2→1→6→5→4; if K=4, you must output 4→3→2→1→5→6.
Input Specification:
Each input file contains one test case. For each case, the first line contains the address of the first node, a positive N (≤) which is the total number of nodes, and a positive K (≤) which is the length of the sublist to be reversed. The address of a node is a 5-digit nonnegative integer, and NULL is represented by -1.
Then N lines follow, each describes a node in the format:
Address Data Next
where Address
is the position of the node, Data
is an integer, and Next
is the position of the next node.
Output Specification:
For each case, output the resulting ordered linked list. Each node occupies a line, and is printed in the same format as in the input.
Sample Input:
00100 6 4
00000 4 99999
00100 1 12309
68237 6 -1
33218 3 00000
99999 5 68237
12309 2 33218
Sample Output:
00000 4 33218
33218 3 12309
12309 2 00100
00100 1 99999
99999 5 68237
68237 6 -1
AC Code
1 #include<stdio.h>
2 typedef struct _Node{ //创建节点
3 int data;
4 int next;
5 }node;
6 void Input_array(node a[],int N);
7 void reverse_once(node a[],int *sub_start,int k);
8 void Output_array(node a[],int start);
9 int main(){
10 node a[100002]; //节点数组
11 int N;
12 int k;
13 int times_of_reverse; //需要反转的次数
14 int sub_start; //子头节点
15 int n;
16 int p;
17 //读入
18 scanf("%d %d %d",&a[100001].next,&N,&k);
19 Input_array(a,N);
20 //处理
21 p = a[100001].next;
22 n = 0;
23 while(p!=-1){ //找到有效的个数
24 n++;
25 p = a[p].next;
26 }
27 times_of_reverse = n/k;
28 sub_start = 100001; //头节点
29 while(times_of_reverse--){
30 reverse_once(a,&sub_start,k);
31 }
32
33 //输出
34 Output_array(a,a[100001].next);
35 return 0;
36 }
37
38 void Input_array(node a[],int N){ //读入
39 int addr_tem,data_tem,next_tem;
40 while(N--){
41 scanf("%d %d %d",&addr_tem,&data_tem,&next_tem);
42 a[addr_tem].data = data_tem;
43 a[addr_tem].next = next_tem;
44 }
45 }
46 void reverse_once(node a[],int *sub_start,int k){ //反转一次
47 int thisP,preP,lastP,temP; //比经典单链表的反转多记录一个需返回的下一个头节点,temP
48 thisP = a[*sub_start].next;
49 temP = thisP;
50 lastP = -1;
51 for(int i = 0; i < k ; i++){
52 preP = a[thisP].next;
53 a[thisP].next = lastP;
54 lastP = thisP;
55 thisP = preP;
56 }
57 a[temP].next = thisP; //普通反转没有这一步
58 a[*sub_start].next = lastP;
59 *sub_start = temP;
60 }
61 void Output_array(node a[],int start){
62 int p = start;
63 while(a[p].next!= -1){
64 printf("%05d %d %05d
",p,a[p].data,a[p].next);
65 p = a[p].next;
66 }
67 printf("%05d %d %d
",p,a[p].data,a[p].next);
68
69 }
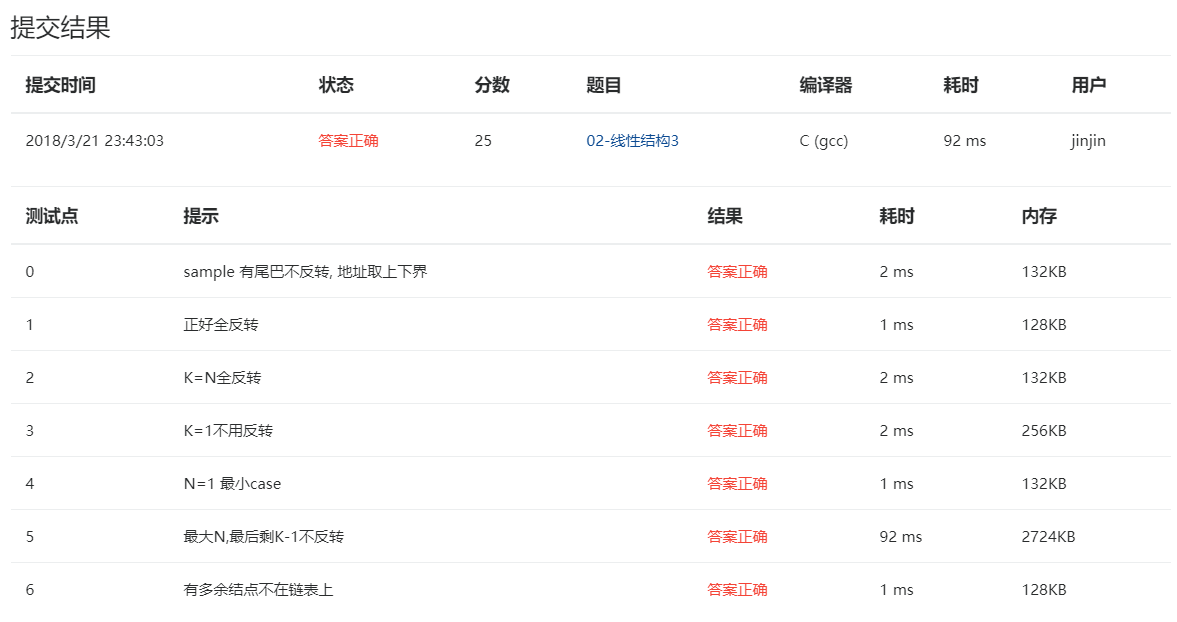