学习总结
1.阅读下面程序,分析是否能编译通过?如果不能,说明原因。应该如何修改?程序的运行结果是什么?为什么子类的构造方法在运行之前,必须调用父 类的构造方法?能不能反过来?
class Grandparent { public Grandparent() { System.out.println("GrandParent Created."); } public Grandparent(String string) { System.out.println("GrandParent Created.String:" + string); } } class Parent extends Grandparent { public Parent() { System.out.println("Parent Created"); super("Hello.Grandparent."); } } class Child extends Parent { public Child() { System.out.println("Child Created"); } } public class Test{ public static void main(String args[]) { Child c = new Child(); } }
不能通过编译,super语句应该放在第一句。对父类含参数的构造方法,子类可以通过在定义自己的构造方法中使用super关键字来调用它,但这个调用语句必须是子类构造方法的第一个可执行语句。
修改:
class Grandparent { public Grandparent() { System.out.println("GrandParent Created."); } public Grandparent(String string) { System.out.println("GrandParent Created.String:" + string); } } class Parent extends Grandparent { public Parent() { super("Hello.Grandparent."); System.out.println("Parent Created"); } } class Child extends Parent { public Child() { System.out.println("Child Created"); } } public class Test{ public static void main(String args[]) { Child c = new Child(); } }
运行结果:
2.阅读下面程序,分析程序中存在哪些错误,说明原因,应如何改正?正确程序的运行结果是什么?
class Animal{ void shout(){ System.out.println("动物叫!"); } } class Dog extends Animal{ public void shout(){ System.out.println("汪汪......!"); } public void sleep() { System.out.println("狗狗睡觉......"); } } public class Test{ public static void main(String args[]) { Animal animal = new Dog(); animal.shout(); animal.sleep(); Dog dog = animal; dog.sleep(); Animal animal2 = new Animal(); dog = (Dog)animal2; dog.shout(); } }
1.animal.sleep();错误.sleep是子类中的方法,声明父类的对象,父类的对象不能直接调用子类中的slepp方法。
2.Dog dog = animal;错误,下转型时要注意,父类是可以有多个子类的,而子类只能有一个父类。
3.dog = (Dog)animal2;错误,强制转换,声明的对象需要父类指向子类。
改正:
class Animal{ void shout(){ System.out.println("动物叫!"); } } class Dog extends Animal{ public void shout(){ System.out.println("汪汪......!"); } public void sleep() { System.out.println("狗狗睡觉......"); } } public class Test{ public static void main(String args[]) { Animal animal = new Dog(); animal.shout(); //animal.sleep(); Dog dog = (Dog) animal; dog.sleep(); Animal animal2 = new Animal(); if(animal2 instanceof Dog) { dog = (Dog)animal2; dog.shout(); } } }
运行结果:
3.运行下列程序
class Person { private String name ; private int age ; public Person(String name,int age){ this.name = name ; this.age = age ; } } public class Test{ public static void main(String args[]){ Person per = new Person("张三",20) ; System.out.println(per); System.out.println(per.toString()) ; } }
(1)程序的运行结果如下,说明什么问题?
Person@166afb3 Person@166afb3
说明toString方法不写也可以调用
(2)那么,程序的运行结果到底是什么呢?利用eclipse打开println(per)方法的源码,查看该方法中又调用了哪些方法,能否解释本例的运行结果?
public String toString() { return getClass().getName() + "@" + Integer.toHexString(hashCode()); }
返回了该类的类名,
(3)在Person类中增加如下方法
public String toString(){ return "姓名:" + this.name + ",年龄:" + this.age ; }
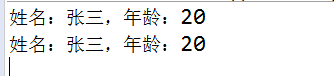
所有的类均为Object类的子类,当该类写了了toString方法后,再调用时就会调用类中的方法,不写的话,调用的是Object类默认的toString方法。
4.汽车租赁公司,出租汽车种类有客车、货车和皮卡三种,每辆汽车除了具有编号、名称、租金三个基本属性之外,客车有载客量,货车有载货量,皮卡则同时具有载客量和载货量。用面向对象编程思想分析上述问题,将其表示成合适的类、抽象类或接口,说明设计思路。现在要创建一个可租车列表,应当如何创建?
定义两个接口,分别定义载客量和载货量属性。
定义一个抽象类,具有编号、名称、租金属性。
定义客车类继承抽象类和实现载客量接口。
火车类继承抽象类和载货量接口。
皮卡类继承抽象类和载客量和载货量接口。
5.阅读下面程序,分析代码是否能编译通过,如果不能,说明原因,并进行改正。如果能,列出运行结果
interface Animal{ void breathe(); void run(); void eat(); } class Dog implements Animal{ public void breathe(){ System.out.println("I'm breathing"); } void eat(){ System.out.println("I'm eating"); } } public class Test{ public static void main(String[] args){ Dog dog = new Dog(); dog.breathe(); dog.eat(); } }
不能通过编译。
1.子类Dog要用接口的话,需要重新写接口中的方法。
2.eat()方法的权限没有指明,缺public
interface Animal{ void breathe(); void run(); void eat(); } class Dog implements Animal{ public void breathe(){ System.out.println("I'm breathing"); } public void eat(){ System.out.println("I'm eating"); } public void run() { } } public class lianxi{ public static void main(String[] args){ Dog dog = new Dog(); dog.breathe(); dog.eat(); } }
(二)实验总结
1.银行
程序设计思路:
①定义一个银行类和一个交易类
②定义两个静态方法welcome()和welcomenext()
③构造方法需要接受的用户信息有用户名、密码、交易额,构造方法中用交易额减去10开卡费为当前余额
④存款需要修改余额,让余额直接加上本次交易额
⑤<5>取款需要验证密码和余额,首先用equalsIIgnoreCase()方法判断密码是否正确,不正确返回0,正确则继续比较余额和本次交易额,若小于则返回-1,大于则返回1,通过trade类判断
⑥Trade类编写一个菜单方法,调用菜单和switch case结构,选择开户、存款、取款和退出,分别调用Bank类的方法
2.员工
①定义员工类,具有姓名、年龄、性别属性,并具有构造方法和显示数据方法。 设计思路:
②定义管理层类,继承员工类,有自己的属性职务和年薪。
③定义职员类,继承员工类,并有自己的属性所属部门和月薪。
④定义一个测试类,进行测试。
3.平面图形
①定义平面类和立体类
②义圆,矩形,三角形三个类,继承平面类,分别重写抽象方法
③定义圆柱,圆锥,球三个类继承立体类,重写抽象方法,测试类中,产生随机数,声明对象,随机产生不同对象的面积周长(表面积体积),判断输入是否相等。
4.饲养员
①定义了一个Animal的抽象类,包含food属性和name属性以及两个抽象方法(传数组的和传单个对象的)
②利用上转型,将eat方法里面的形参定义为Animal对象,实参为子类实例
③定义饲养员类,调用Animal的eat方法,当定义一个food属性时,可以把代码简化
1.宠物商店
①定义接口,包含编号、种类、品种、单价、数量等属性,
②声明Cat和Dog,实现接口,
③定义petshop类,
④构造方法开辟空间,购买时不能超过宠物剩余量。