为现有图像处理程序添加读取exif的功能
exif是图片的重要参数,在使用过程中很关键的一点是exif的数据能够和图片一起存在。exif的相关功能在操作系统中就集成了,在csharp中也似乎有了实现。但是使用mfc来实现这个功能,的确费了我的一些时间。下面是我的实现方式。
读取exif,参考了网络资料
添加cexif.h和cexif.cpp,添加入现有程序
在需要读取的地方添加相关代码
FILE* hFile=fopen(FilePathName.c_str(),"rb"); if (hFile){ memset(&m_exifinfo,0,sizeof(EXIFINFO)); Cexif exif(&m_exifinfo); exif.DecodeExif(hFile); fclose(hFile); if (m_exifinfo.IsExif) { string cameramaker = m_exifinfo.CopyRight;//获得CopyRight vector<string> vecParams; if ("@@" == cameramaker.substr(0,2)) //确认为带参数图片 { cameramaker = cameramaker.substr(2,cameramaker.length()-2); SplitString(cameramaker,vecParams,"|");//保存各参数到vecParams中去 //updateGuides给的是图像真实像素值 if (vecParams.size()<13) return; m_freal2screen = Str2RealPixF(vecParams[12]);//获得之前保存的比值 if (m_freal2screen<0) return; UpdateGuides(Str2RealPix(vecParams[0]),Str2RealPix(vecParams[1]),Str2RealPix(vecParams[2]),Str2RealPix(vecParams[3]),Str2RealPix(vecParams[4]),Str2RealPix(vecParams[5]),Str2RealPix(vecParams[6]),Str2RealPix(vecParams[7]),Str2RealPix(vecParams[8]),Str2RealPix(vecParams[9]),Str2RealPix(vecParams[10]),Str2RealPix(vecParams[11])); } } else MessageBox("没有找到矢量数据信息!","EXIF",MB_OK); } fclose(hFile);
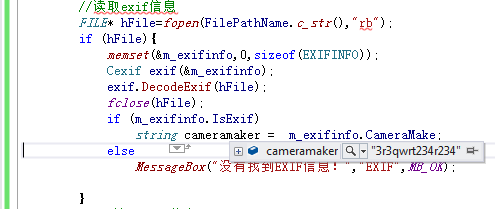
但是这里的exif读取的信息不实很全
同时找到另一个代码,但是版本比较老,只能在vc6下面运行
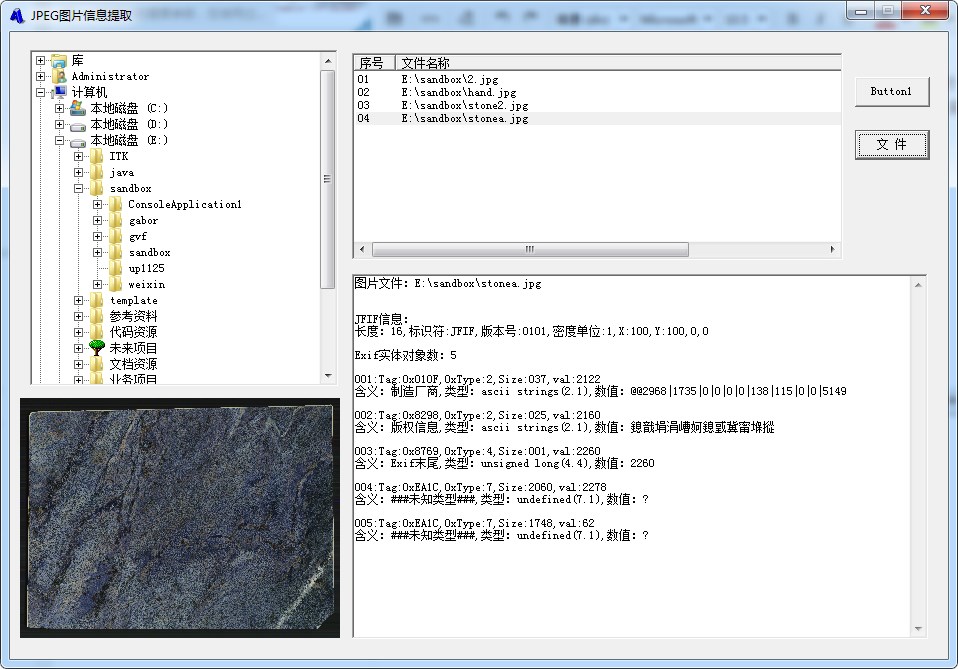
这个时候,我需要考虑的就是写入的数据是否够长的问题。我进行了边界测试
经过测试,在这种模式下是没有问题的

对原有代码进行初步修改就可以。考虑到还是现有集成的比较好用,还是用集成好的。但是集成好的版本没有 版权这个段?这个如何办了?
反思一想,问题不就出现在位置信息吗?我将正确的版权信息覆盖掉make信息,问题解决。
最后提供我修改完成的带版权信息的exif.c。
解决问题的思路很重要。
附全部代码
/* * File: exif.cpp * Purpose: cpp EXIF reader * 16/Mar/2003 <ing.davide.pizzolato@libero.it> * based on jhead-1.8 by Matthias Wandel <mwandel(at)rim(dot)net> */ #include "stdafx.h" #include "Exif.h" //////////////////////////////////////////////////////////////////////////////// Cexif::Cexif(EXIFINFO* info) { if (info) { m_exifinfo = info; freeinfo = false; } else { m_exifinfo = new EXIFINFO; memset(m_exifinfo,0,sizeof(EXIFINFO)); freeinfo = true; } m_szLastError[0]='