1、首先第一步需要导入对应的jar包
我们来看下面的一个例子
OK,在前面的一系列博客里面,我整理过了Assert类下面常用的断言方法,比如assertEquals等等,但是org.junit.Assert类下还有一个方法也用来断言,而且更加强大。这就是我们这里要这里的:
Assert的AssertThat()方法和Hamcrest匹配器
1,断言抛出的异常
明显的,有的时候我们想测试我们的代码在某种情况下抛出异常。比如说对于无效输入,我们希望代码抛出IllegalArgumentException。前面我也已经说过了,可以使用Test注解的一个expected属性来遇见我们抛出的异常。代码如下:
package test.junit4test;
import org.junit.Test;
public class LinkinTest
{
@Test(expected=NullPointerException.class)
public void test()
{
String str = null;
System.out.println(str.toString());
}
}
package test.junit4test; import org.hamcrest.Matchers; import org.junit.Assert; import org.junit.Test; public class LinkinTest { @Test public void test() { try { throw new Exception("吆西,这里应该抛出异常的呢。。。"); } catch (Exception e) { Assert.assertThat(e.getMessage(), Matchers.containsString("吆西")); } } }
代码写成下面的形式
package com.weiyuan.test; import java.security.AlgorithmParameterGenerator; import java.security.AlgorithmParameters; import org.hamcrest.Matchers; import org.junit.Assert; import org.junit.Before; import org.junit.Test; import static org.junit.Assert.*; public class TestCalcuate { private Calcuate calcuate; /** * * 执行任何测试方法之前都会调用该方法 * */ @Before public void setUp(){ calcuate = new Calcuate(); } /** * 为了兼容junit3 * 按照testXX方法命名 * public int (int a,int b){ * */ @Test public void testAdd(){ int result = calcuate.add(12, 20); assertEquals("加法有问题", result, 32); } @Test(expected=ArithmeticException.class) public void testDivide(){ int result = calcuate.divide(12, 0); assertEquals("触发有问题", result, 4); } /** * 如果测试方法执行超过200毫秒 * 就会报错 * */ @Test(timeout=200) public void testTimeout(){ try { Thread.sleep(100); } catch (InterruptedException e) { // TODO Auto-generated catch block e.printStackTrace(); } } @Test public void testException(){ try { throw new Exception("吆西,这里应该抛出异常的呢。。。"); } catch (Exception e) { Assert.assertThat(e.getMessage(), Matchers.containsString("555")); } } }
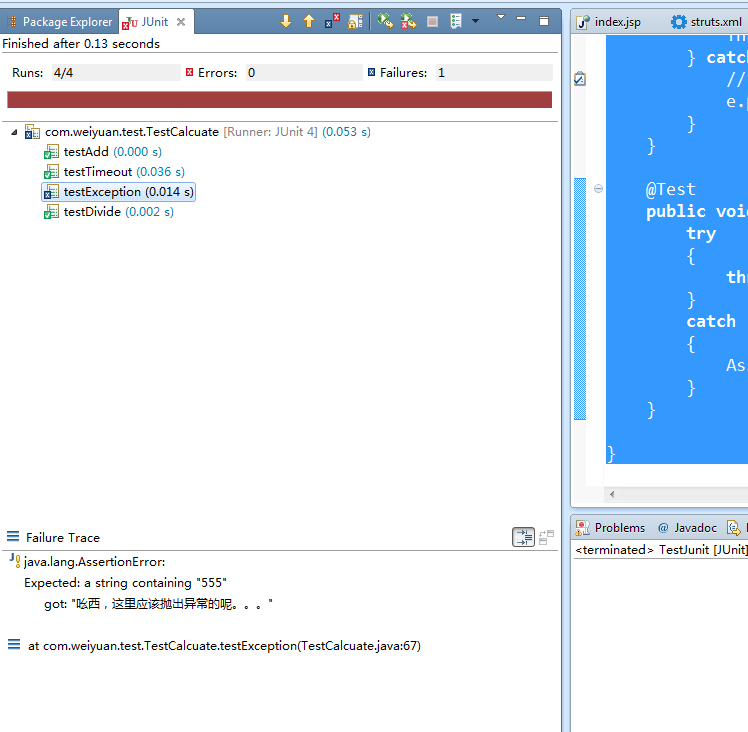
因为上面抛出的异常的message不包含555字符串所以运行失败,我们可以通过hamcrest包提供的Matches来增加junit的测试功能
需要注意点:hamcrest需要静态导入对应的包
import static org.hamcrest.Matchers.*;
案例2:使用hamcrest判断一个数的大小
package com.weiyuan.test; import java.security.AlgorithmParameterGenerator; import java.security.AlgorithmParameters; import org.hamcrest.Matchers; import org.junit.Before; import org.junit.Test; import static org.junit.Assert.*; import static org.hamcrest.Matchers.*; public class TestCalcuate { private Calcuate calcuate; /** * * 执行任何测试方法之前都会调用该方法 * */ @Before public void setUp(){ calcuate = new Calcuate(); } /** * 为了兼容junit3 * 按照testXX方法命名 * public int (int a,int b){ * */ @Test public void testAdd(){ int result = calcuate.add(12, 20); assertEquals("加法有问题", result, 32); } @Test(expected=ArithmeticException.class) public void testDivide(){ int result = calcuate.divide(12, 0); assertEquals("触发有问题", result, 4); } /** * 如果测试方法执行超过200毫秒 * 就会报错 * */ @Test(timeout=200) public void testTimeout(){ try { Thread.sleep(100); } catch (InterruptedException e) { // TODO Auto-generated catch block e.printStackTrace(); } } @Test public void testHamcrest(){ /* * 判断10是否大于20*/ assertThat(10, greaterThan(20)); } }
使用了hamacet提供的greaterThan功能
程序运行的结果如下
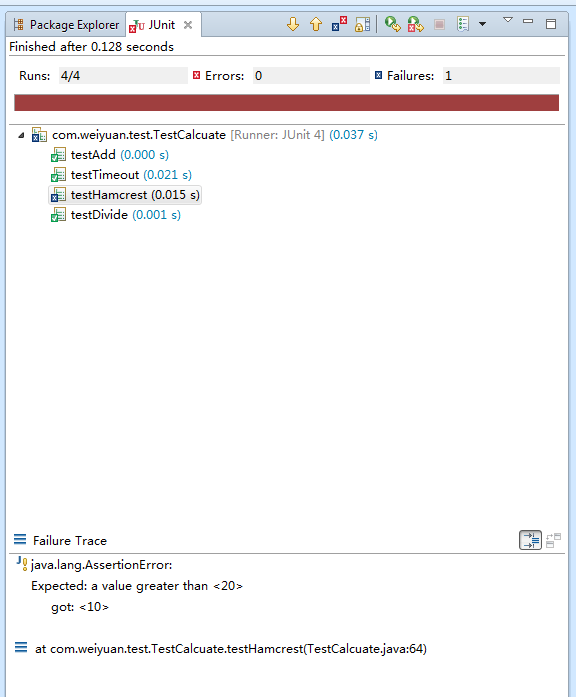
hamcrest还定义了下面的很多规则
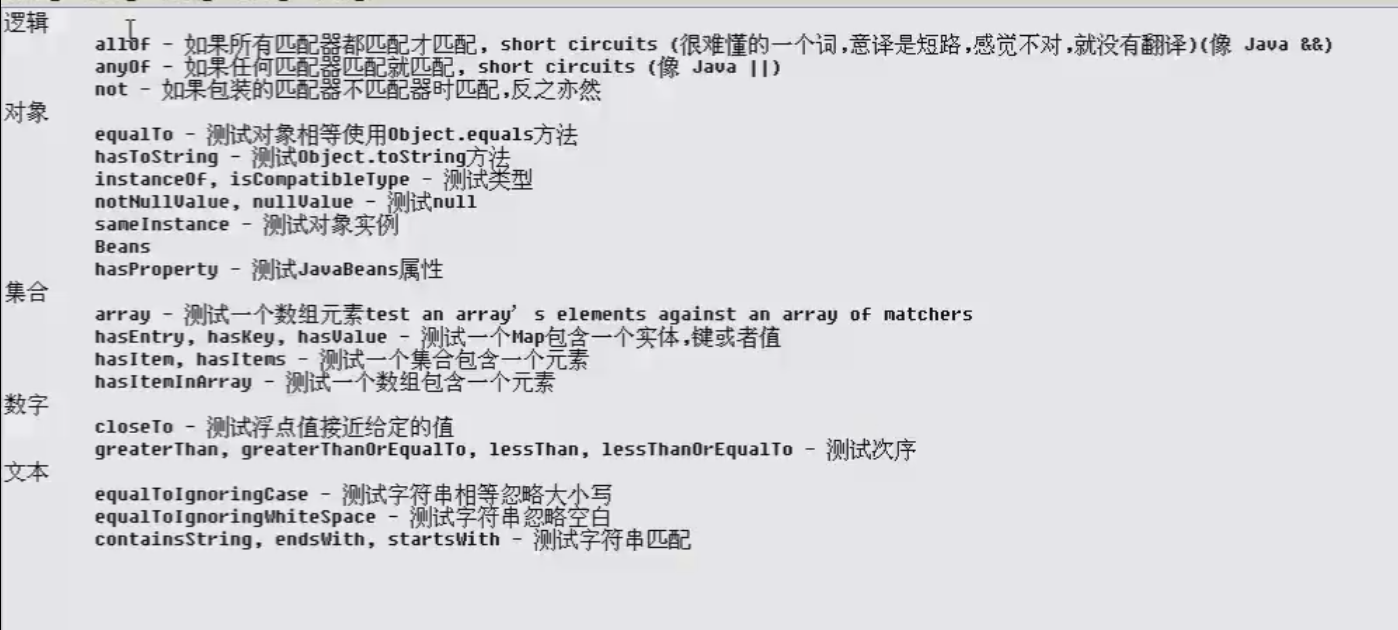
我们判断10是否大于5,小于12
@Test public void testHamcrest(){ assertThat(10, allOf(greaterThan(5),lessThan(12))); }
在运行的时候报错
这是由于junit4的java包和hamcijava冲突引起的,如何解决了
需要把ham的jar包移动到juint的上面
我们在运行的时候,就不会报错了
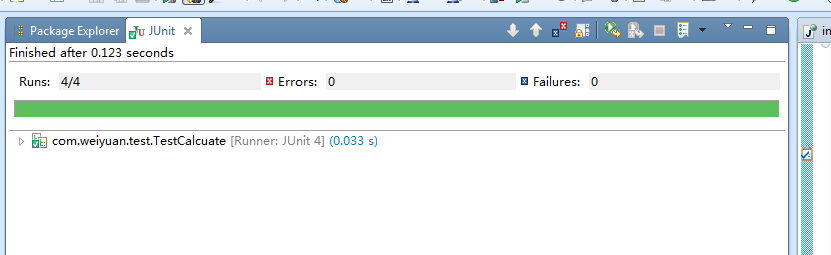
TestSuite:测试集合,即一组测试。一个test suite是把多个相关测试归入一组的快捷方式。如果自己没有定义,Junit会自动提供一个test suite ,包括TestCase中的所有测试。
依赖性指的是每个测试方法都是独立的,执行测试方法2之前,才能执行测试方法3,测试的时候必须保证测试类的独立性
我们以前执行每个测试类都是独立的,我们现在有很多测试类,我们能不能使用一个组件在管理执行测试类,当然可以
package com.weiyuan.test; import org.junit.runner.RunWith; import org.junit.runners.Suite; import org.junit.runners.Suite.SuiteClasses; //说明这个测试类,是一个组件测试类,用来管理所有的测试类 @RunWith(Suite.class) //指定管理那些测试类 @SuiteClasses({TestA.class,TestB.class,TestCalcuate.class}) public class TestSuite { }
package com.weiyuan.test; import org.junit.Test; public class TestA { @Test public void testA(){ System.out.println("testA is called"); } }
package com.weiyuan.test; import org.junit.Test; public class TestB { @Test public void testB(){ System.out.println("testB is called"); } }
整个项目的工程代码如下所示
我们在组件的java类上面运行测试
我们可以看到控制台的打印
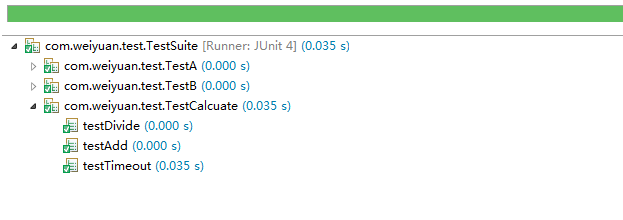
先执行TestA中的所有方法,然后是TestB中的,然后是TestCalcuate的