#include <stdio.h>
#include <stdlib.h>
/* run this program using the console pauser or add your own getch, system("pause") or input loop */
//Q:打印一串数字1,2,3 用两种方法
//知识点:指针、for循环、数组
int main(int argc, char *argv[])
{
int array[] = {1,2,3}; //定义数组
int i,j; //定义变量
int *pt; //定义指针
//1、传统方法
printf("---------传统方法for循环----------");
for(i=0;i<3;i++)
{
printf("
%d",array[i]);
}
//2、使用指针
printf("
---------使用指针----------
");
printf("
---------只打印一个数字----------");
//只打印一个数字
pt = array+1;//指针先要初始化才能使用 array+x 加几个就是第几个的数字
printf("
数组里的数字 %d",*pt);//有星号的就打印指针所对应的值
printf("
数组里数字所储存的地址 %d",pt); //没有星号的就打印指针的地址
printf("
---------------循环打印全部-------------------");
for(j=0;j<3;j++) //循环依次打印数组里的数字
{
pt = array+j;
printf("
数组里的数字 %d
",*pt);
printf("数组里数字所储存的地址 %d
",pt);
}
return 0;
}
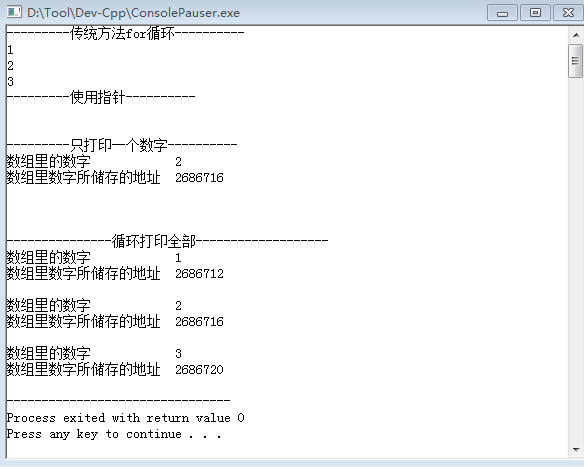
1 /*
2 ============================================================================
3 Name : Hello.c
4 Author :
5 Version :
6 Copyright : Your copyright notice
7 Description : Hello World in C, Ansi-style
8 ============================================================================
9 */
10
11 #include <stdio.h>
12 #include <stdlib.h>
13
14 int main(void) {
15
16 printf("%d
",sizeof(char));
17 printf("%d
",sizeof(int));
18 int a = 10;
19 int *p1 = &a;
20 char *p2 = p1;
21
22 printf("%d
",p1);
23 printf("%d
",p2);
24
25 printf("%d
",*p1);//10
26 printf("%d
",*p2);
27 }