主机环境:win10系统,pcl库1.11.1,
vs2019 pcl库安装以及环境配置如下连接:
https://www.jb51.net/article/190710.htm
代码很简单,主要是做个坐标转换,如下:
#include <io.h>
#include <fstream>
#include <string>
#include <vector>
#include <iostream>
#include <iostream> //标准输入输出流
#include <pcl/io/pcd_io.h> //PCL的PCD格式文件的输入输出头文件
#include <pcl/point_types.h> //PCL对各种格式的点的支持头文件
#include <pcl/visualization/pcl_visualizer.h>
#include <pcl/common/transforms.h>
#include <pcl/visualization/cloud_viewer.h>
#include <fstream>
#include <string>
#include <vector>
#include <iostream>
#include <iostream> //标准输入输出流
#include <pcl/io/pcd_io.h> //PCL的PCD格式文件的输入输出头文件
#include <pcl/point_types.h> //PCL对各种格式的点的支持头文件
#include <pcl/visualization/pcl_visualizer.h>
#include <pcl/common/transforms.h>
#include <pcl/visualization/cloud_viewer.h>
using namespace std;
//获取所有的文件名
void GetAllFiles(string path, vector<string>& files)
{
long hFile = 0;
//文件信息
struct _finddata_t fileinfo;
string p;
if ((hFile = _findfirst(p.assign(path).append("\*").c_str(), &fileinfo)) != -1)
{
do
{
if ((fileinfo.attrib & _A_SUBDIR))
{
if (strcmp(fileinfo.name, ".") != 0 && strcmp(fileinfo.name, "..") != 0)
{
files.push_back(p.assign(path).append("\").append(fileinfo.name));
void GetAllFiles(string path, vector<string>& files)
{
long hFile = 0;
//文件信息
struct _finddata_t fileinfo;
string p;
if ((hFile = _findfirst(p.assign(path).append("\*").c_str(), &fileinfo)) != -1)
{
do
{
if ((fileinfo.attrib & _A_SUBDIR))
{
if (strcmp(fileinfo.name, ".") != 0 && strcmp(fileinfo.name, "..") != 0)
{
files.push_back(p.assign(path).append("\").append(fileinfo.name));
GetAllFiles(p.assign(path).append("\").append(fileinfo.name), files);
}
}
else
{
files.push_back(p.assign(path).append("\").append(fileinfo.name));
}
} while (_findnext(hFile, &fileinfo) == 0);
_findclose(hFile);
}
}
}
}
else
{
files.push_back(p.assign(path).append("\").append(fileinfo.name));
}
} while (_findnext(hFile, &fileinfo) == 0);
_findclose(hFile);
}
}
//获取特定格式的文件名
void GetAllFormatFiles(string path, vector<string>& files, string format)
{
//文件句柄
long long hFile = 0;
//文件信息
struct _finddata_t fileinfo;
string p;
if ((hFile = _findfirst(p.assign(path).append("\*" + format).c_str(), &fileinfo)) != -1)
{
do
{
if ((fileinfo.attrib & _A_SUBDIR))
{
if (strcmp(fileinfo.name, ".") != 0 && strcmp(fileinfo.name, "..") != 0)
{
//files.push_back(p.assign(path).append("\").append(fileinfo.name) );
GetAllFormatFiles(p.assign(path).append("\").append(fileinfo.name), files, format);
}
}
else
{
files.push_back(p.assign(path).append("\").append(fileinfo.name));
}
} while (_findnext(hFile, &fileinfo) == 0);
_findclose(hFile);
}
}
void GetAllFormatFiles(string path, vector<string>& files, string format)
{
//文件句柄
long long hFile = 0;
//文件信息
struct _finddata_t fileinfo;
string p;
if ((hFile = _findfirst(p.assign(path).append("\*" + format).c_str(), &fileinfo)) != -1)
{
do
{
if ((fileinfo.attrib & _A_SUBDIR))
{
if (strcmp(fileinfo.name, ".") != 0 && strcmp(fileinfo.name, "..") != 0)
{
//files.push_back(p.assign(path).append("\").append(fileinfo.name) );
GetAllFormatFiles(p.assign(path).append("\").append(fileinfo.name), files, format);
}
}
else
{
files.push_back(p.assign(path).append("\").append(fileinfo.name));
}
} while (_findnext(hFile, &fileinfo) == 0);
_findclose(hFile);
}
}
int main()
{
string filePath = "C:\Users\CTOS\Desktop\newpcd";
vector<string> files;
const char* distAll = "*.pcd";
string format = ".pcd";
GetAllFormatFiles(filePath, files, format);
ofstream ofn(distAll);
int size = files.size();
cout << size << endl;
ofn << size << endl;
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud(new pcl::PointCloud<pcl::PointXYZ>); // 创建点云(指针)
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud_prt(new pcl::PointCloud<pcl::PointXYZ>); // 创建点云(指针)
pcl::PointCloud<pcl::PointXYZ>::Ptr transformed_cloud(new pcl::PointCloud<pcl::PointXYZ>); // 创建点云(指针)
for (int i = 0; i < size; i++)
{
ofn << files[i] << endl;
cout << files[i] << endl;
if (pcl::io::loadPCDFile<pcl::PointXYZ>(files[i], *cloud_prt) == -1) //* 读入PCD格式的文件,如果文件不存在,返回-1
{
cout << "Couldn't read file pcd "<< endl; //文件不存在时,返回错误,终止程序。
return (-1);
}
Eigen::Vector4f p = cloud_prt->sensor_origin_.matrix();
Eigen::Quaternionf q;
q = cloud_prt->sensor_orientation_.matrix();
Eigen::Vector3f eulerAngle = q.matrix().eulerAngles(2, 1, 0);
Eigen::Affine3f transform = Eigen::Affine3f::Identity();
transform.translation() << p[0], p[1], p[2];
transform.rotate(q);
std::cout << transform.matrix() << std::endl;
pcl::transformPointCloud(*cloud_prt, *transformed_cloud, transform);
*cloud += *transformed_cloud;
cloud_prt->clear();
transformed_cloud->clear();
Sleep(1);
}
pcl::visualization::CloudViewer viewer("pcd viewer");
viewer.showCloud(cloud);
while (!viewer.wasStopped())
{
}
ofn.close();
return 0;
}
{
string filePath = "C:\Users\CTOS\Desktop\newpcd";
vector<string> files;
const char* distAll = "*.pcd";
string format = ".pcd";
GetAllFormatFiles(filePath, files, format);
ofstream ofn(distAll);
int size = files.size();
cout << size << endl;
ofn << size << endl;
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud(new pcl::PointCloud<pcl::PointXYZ>); // 创建点云(指针)
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud_prt(new pcl::PointCloud<pcl::PointXYZ>); // 创建点云(指针)
pcl::PointCloud<pcl::PointXYZ>::Ptr transformed_cloud(new pcl::PointCloud<pcl::PointXYZ>); // 创建点云(指针)
for (int i = 0; i < size; i++)
{
ofn << files[i] << endl;
cout << files[i] << endl;
if (pcl::io::loadPCDFile<pcl::PointXYZ>(files[i], *cloud_prt) == -1) //* 读入PCD格式的文件,如果文件不存在,返回-1
{
cout << "Couldn't read file pcd "<< endl; //文件不存在时,返回错误,终止程序。
return (-1);
}
Eigen::Vector4f p = cloud_prt->sensor_origin_.matrix();
Eigen::Quaternionf q;
q = cloud_prt->sensor_orientation_.matrix();
Eigen::Vector3f eulerAngle = q.matrix().eulerAngles(2, 1, 0);
Eigen::Affine3f transform = Eigen::Affine3f::Identity();
transform.translation() << p[0], p[1], p[2];
transform.rotate(q);
std::cout << transform.matrix() << std::endl;
pcl::transformPointCloud(*cloud_prt, *transformed_cloud, transform);
*cloud += *transformed_cloud;
cloud_prt->clear();
transformed_cloud->clear();
Sleep(1);
}
pcl::visualization::CloudViewer viewer("pcd viewer");
viewer.showCloud(cloud);
while (!viewer.wasStopped())
{
}
ofn.close();
return 0;
}
最终效果:
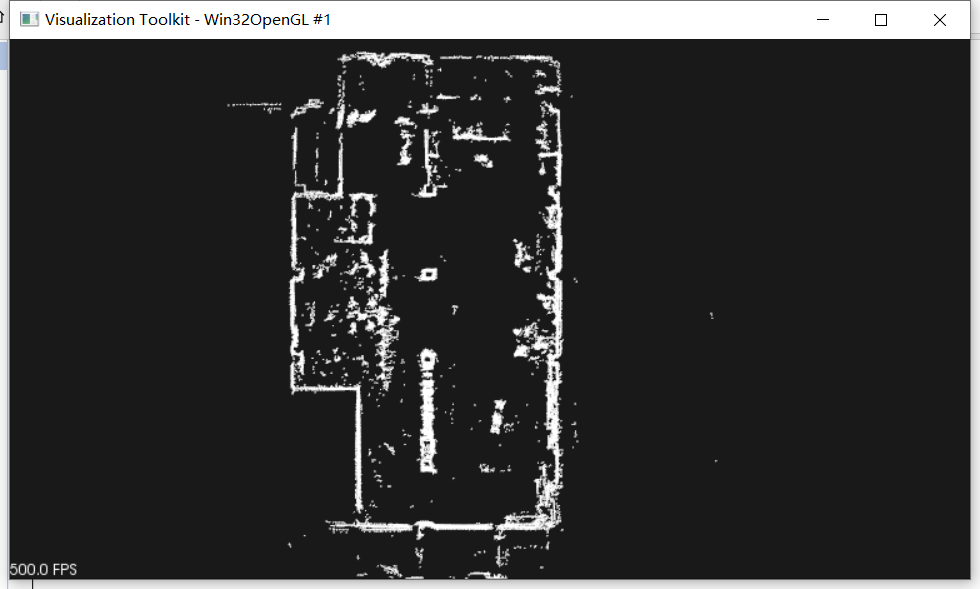