效果:把蓝天部分给提取出来
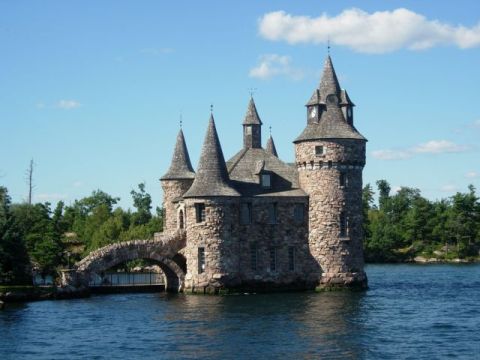
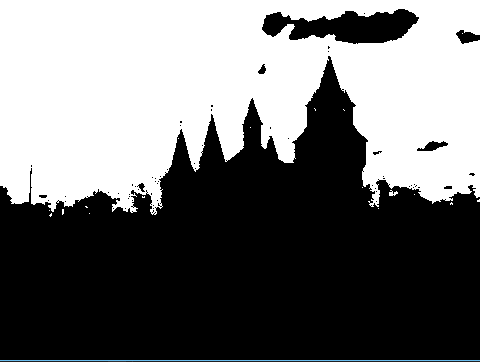
#include <opencv2/core/core.hpp> #include<opencv2/imgproc/imgproc.hpp> #include <opencv2/highgui/highgui.hpp> using namespace cv; using namespace std; class ColorDetector{ private: int minDist; Vec3b target; Mat result; public: ColorDetector():minDist(100){ target[0] = target[1] = target[2] = 0; } //设置色彩距离阈值 void setColorDistanceThreshold(int distance){ if (distance < 0) distance = 0; minDist = distance; } //get set方法设置Private里面的变量 int getColorDistanceThreshold()const{ return minDist; } //设置需检测的颜色 void setTargetColor(unsigned char red, unsigned char green, unsigned char blue){ target[2] = red; target[1] = green; target[0] = blue; } void setTargetColor(Vec3b color){ target = color; } Vec3b getTargetColor()const{ return target; } //计算与目标颜色的距离,采用的是街区距离 int getDistance(const Vec3b& color) const{ return abs(color[0] - target[0]) + abs(color[1] - target[1]) + abs(color[2] - target[2]); } Mat ColorDetector::process(const Mat &image){ result.create(image.rows,image.cols, CV_8U); Mat_<Vec3b>::const_iterator it = image.begin<Vec3b>(); Mat_<Vec3b>::const_iterator itend = image.end<Vec3b>(); Mat_<uchar>::iterator itout = result.begin<uchar>(); for (; it != itend; ++it, ++itout){ if (getDistance(*it) < minDist){ *itout = 255; } else{ *itout = 0; } } return result; } }; int main(){ //创建对象 ColorDetector cdector; Mat image = imread("boldt.jpg"); if (!image.data){ return 0; } cdector.setTargetColor(130, 190, 230); namedWindow("result"); imshow("result", cdector.process(image)); waitKey(0); return 0; }