Word Puzzles
Time Limit: 5000MS | Memory Limit: 65536K | |||
Total Submissions: 10244 | Accepted: 3864 | Special Judge |
Description
Word puzzles are usually simple and very entertaining for all ages. They are so entertaining that Pizza-Hut company started using table covers with word puzzles printed on them, possibly with the intent to minimise their client's
perception of any possible delay in bringing them their order.
Even though word puzzles may be entertaining to solve by hand, they may become boring when they get very large. Computers do not yet get bored in solving tasks, therefore we thought you could devise a program to speedup (hopefully!) solution finding in such puzzles.
The following figure illustrates the PizzaHut puzzle. The names of the pizzas to be found in the puzzle are: MARGARITA, ALEMA, BARBECUE, TROPICAL, SUPREMA, LOUISIANA, CHEESEHAM, EUROPA, HAVAIANA, CAMPONESA.
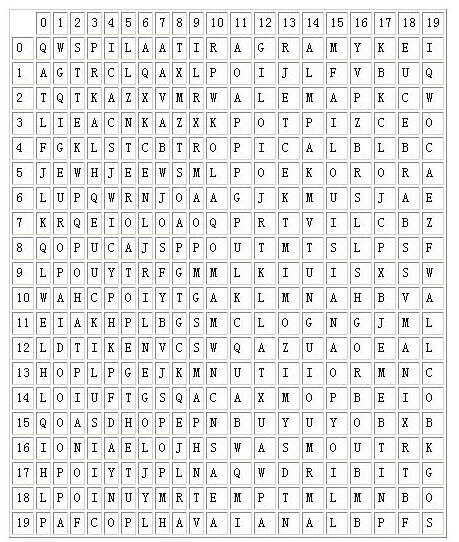
Your task is to produce a program that given the word puzzle and words to be found in the puzzle, determines, for each word, the position of the first letter and its orientation in the puzzle.
You can assume that the left upper corner of the puzzle is the origin, (0,0). Furthemore, the orientation of the word is marked clockwise starting with letter A for north (note: there are 8 possible directions in total).
Even though word puzzles may be entertaining to solve by hand, they may become boring when they get very large. Computers do not yet get bored in solving tasks, therefore we thought you could devise a program to speedup (hopefully!) solution finding in such puzzles.
The following figure illustrates the PizzaHut puzzle. The names of the pizzas to be found in the puzzle are: MARGARITA, ALEMA, BARBECUE, TROPICAL, SUPREMA, LOUISIANA, CHEESEHAM, EUROPA, HAVAIANA, CAMPONESA.
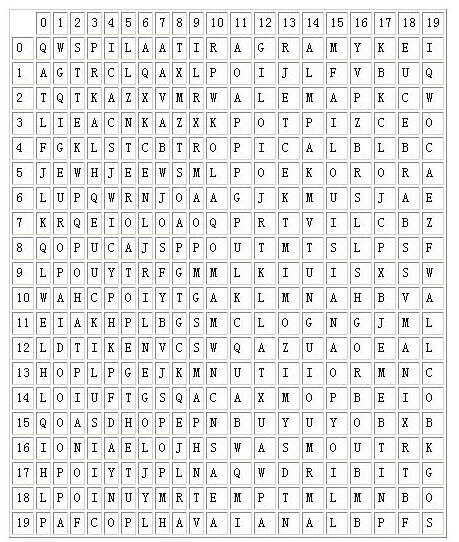
Your task is to produce a program that given the word puzzle and words to be found in the puzzle, determines, for each word, the position of the first letter and its orientation in the puzzle.
You can assume that the left upper corner of the puzzle is the origin, (0,0). Furthemore, the orientation of the word is marked clockwise starting with letter A for north (note: there are 8 possible directions in total).
Input
The first line of input consists of three positive numbers, the number of lines, 0 < L <= 1000, the number of columns, 0 < C <= 1000, and the number of words to be found, 0 < W <= 1000. The following L input lines, each one of
size C characters, contain the word puzzle. Then at last the W words are input one per line.
Output
Your program should output, for each word (using the same order as the words were input) a triplet defining the coordinates, line and column, where the first letter of the word appears, followed by a letter indicating the orientation
of the word according to the rules define above. Each value in the triplet must be separated by one space only.
Sample Input
20 20 10 QWSPILAATIRAGRAMYKEI AGTRCLQAXLPOIJLFVBUQ TQTKAZXVMRWALEMAPKCW LIEACNKAZXKPOTPIZCEO FGKLSTCBTROPICALBLBC JEWHJEEWSMLPOEKORORA LUPQWRNJOAAGJKMUSJAE KRQEIOLOAOQPRTVILCBZ QOPUCAJSPPOUTMTSLPSF LPOUYTRFGMMLKIUISXSW WAHCPOIYTGAKLMNAHBVA EIAKHPLBGSMCLOGNGJML LDTIKENVCSWQAZUAOEAL HOPLPGEJKMNUTIIORMNC LOIUFTGSQACAXMOPBEIO QOASDHOPEPNBUYUYOBXB IONIAELOJHSWASMOUTRK HPOIYTJPLNAQWDRIBITG LPOINUYMRTEMPTMLMNBO PAFCOPLHAVAIANALBPFS MARGARITA ALEMA BARBECUE TROPICAL SUPREMA LOUISIANA CHEESEHAM EUROPA HAVAIANA CAMPONESA
Sample Output
0 15 G 2 11 C 7 18 A 4 8 C 16 13 B 4 15 E 10 3 D 5 1 E 19 7 C 11 11 H
Source
题目大意:给你一个矩阵,然后问每一个串在矩阵中的起点和方向,方向从上(A)顺时针8个方向
就是也8个方向的查询就好。。
ac代码
#include<stdio.h> #include<string.h> char map[1010][1010],key[1010]; int dx[8]={-1,-1,0,1,1,1,0,-1}; int dy[8]={0,1,1,1,0,-1,-1,-1}; int ansx[1010],ansy[1010],ansd[1010],len[1010]; int head,tail; int n,m,w; struct node { node *fail; node *next[26]; int id; node() { fail=NULL; id=0; for(int i=0;i<26;i++) next[i]=NULL; } }*q[50005000]; node *root; void insert(char *s,int id) { int temp,len,i; node *p=root; len=strlen(s); for(i=0;i<len;i++) { temp=s[i]-'A'; if(p->next[temp]==NULL) p->next[temp]=new node(); p=p->next[temp]; } p->id=id; } void build_ac() { head=tail=0; q[tail++]=root; while(head!=tail) { node *p=q[head++]; node *temp=NULL; for(int i=0;i<26;i++) { if(p->next[i]!=NULL) { if(p==root) p->next[i]->fail=root; else { temp=p->fail; while(temp!=NULL) { if(temp->next[i]!=NULL) { p->next[i]->fail=temp->next[i]; break; } temp=temp->fail; } if(temp==NULL) { p->next[i]->fail=root; } } q[tail++]=p->next[i]; } } } } void query(int x,int y,int dir) { node *p=root,*temp; int i,j; for(i=x,j=y;i>=0&&i<n&&j>=0&&j<m;i+=dx[dir],j+=dy[dir]) { int x=map[i][j]-'A'; while(p->next[x]==NULL&&p!=root) p=p->fail; p=p->next[x]; if(p==NULL) { p=root; } temp=p; while(temp!=root&&temp->id!=-1) { int id=temp->id; ansx[id]=i-(len[id]-1)*dx[dir]; ansy[id]=j-(len[id]-1)*dy[dir]; ansd[id]=dir; temp->id=-1; temp=temp->fail; } } } int main() { // int n,m,w; while(scanf("%d%d%d",&n,&m,&w)!=EOF) { int i,j; root=new node(); for(i=0;i<n;i++) { scanf("%s",map[i]); } for(i=1;i<=w;i++) { scanf("%s",key); len[i]=strlen(key); insert(key,i); } build_ac(); for(i=0;i<m;i++) { for(j=0;j<8;j++) { query(0,i,j); query(n-1,i,j); } } for(i=0;i<n;i++) { for(j=0;j<8;j++) { query(i,0,j); query(i,m-1,j); } } for(i=1;i<=w;i++) { printf("%d %d %c ",ansx[i],ansy[i],ansd[i]+'A'); } } }