一、Spring发展史
1、Spring1.x
版本一时代主要是通过XML文件配置bean,在java和xml中不断切换,在学习java web 初期的时候经常使用
2、Spring2.x
版本二可以支持注解模式,这样大大简化开发,减少XML文件配置
注意:
IOC推荐用注解方式实现,AOP推荐用配置方式实现
2.0版本时候的问题,到底采用注解还是文本配置
最后结论最佳实践:
(1)应用的基本配置用xml,比如:数据源、资源文件等;
(2)业务开发用注解,比如:Service中注入bean等;
(2)业务开发用注解,比如:Service中注入bean等;
3、Spring3.X到Spring4.X
从版本三开始启用通过java代码配置取代XML,可以更好的理解Bean
二、Spring的java配置
版本三提出,版本四极力推荐使用。完全替代XML配置
1、最基本的两个java配置
(1)@Configuration 作用于类上,相当于一个xml配置文件;
(2)@Bean 作用于方法上,相当于xml配置中的<bean>;
2、实战演练
(1)创建Spring项目,详见http://www.cnblogs.com/liuyangfirst/p/8298588.html
(2) 创建目录,如图所示:
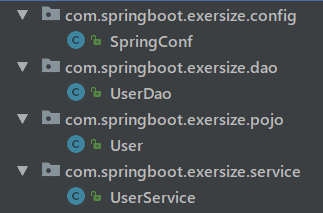
(3)POJO源码

1 package com.springboot.exersize.pojo; 2 3 /** 4 * Created by liuya 5 * User: liuya 6 * Date: 2018/3/24 7 * Time: 21:35 8 * projectName:20180324versionone 9 */ 10 public class User { 11 12 13 private String userName; 14 15 private String passWord; 16 17 private Integer age; 18 19 public String getUserName() { 20 return userName; 21 } 22 23 public void setUserName(String userName) { 24 this.userName = userName; 25 } 26 27 public String getPassWord() { 28 return passWord; 29 } 30 31 public void setPassWord(String passWord) { 32 this.passWord = passWord; 33 } 34 35 public Integer getAge() { 36 return age; 37 } 38 39 public void setAge(Integer age) { 40 this.age = age; 41 } 42 }
) Dao源码

1 package com.springboot.exersize.dao; 2 3 import com.springboot.exersize.pojo.User; 4 5 import java.util.ArrayList; 6 import java.util.List; 7 8 /** 9 * Created by liuya 10 * User: liuya 11 * Date: 2018/3/24 12 * Time: 22:00 13 * projectName:20180324versionone 14 */ 15 public class UserDao { 16 17 18 public List<User> userList() { 19 20 List<User> userList = new ArrayList<>(); 21 22 for (int i = 0; i < 10; i++) { 23 User user = new User(); 24 user.setUserName("username_" + i); 25 user.setPassWord("password_" + i); 26 user.setAge(i + 1); 27 userList.add(user); 28 } 29 return userList; 30 } 31 }
(5)Service源码

1 package com.springboot.exersize.service; 2 3 import com.springboot.exersize.dao.UserDao; 4 import com.springboot.exersize.pojo.User; 5 import org.springframework.beans.factory.annotation.Autowired; 6 import org.springframework.stereotype.Service; 7 8 import java.util.List; 9 10 /** 11 * Created by liuya 12 * User: liuya 13 * Date: 2018/3/24 14 * Time: 22:04 15 * projectName:20180324versionone 16 */ 17 @Service 18 public class UserService { 19 20 @Autowired 21 private UserDao userDao; 22 23 24 public List<User> userList() { 25 return this.userDao.userList(); 26 } 27 28 29 }
(6) Java配置文档

1 package com.springboot.exersize.config; 2 3 import com.springboot.exersize.dao.UserDao; 4 import org.springframework.context.annotation.Bean; 5 import org.springframework.context.annotation.ComponentScan; 6 import org.springframework.context.annotation.Configuration; 7 8 /** 9 * Created by liuya 10 * User: liuya 11 * Date: 2018/3/24 12 * Time: 22:06 13 * projectName:20180324versionone 14 */ 15 16 @Configuration 17 @ComponentScan(basePackages = "com.springboot.exersize.service") 18 public class SpringConf { 19 20 21 @Bean 22 public UserDao getUserDao() { 23 return new UserDao(); 24 } 25 26 27 }
(7)测试代码

1 package com.liuyangfirst.test; 2 3 4 import com.liuyangfirst.conf.SpringConf; 5 import com.liuyangfirst.pojo.User; 6 import com.liuyangfirst.service.UserService; 7 import org.springframework.context.annotation.AnnotationConfigApplicationContext; 8 9 import java.util.List; 10 11 /** 12 * Created by liuya 13 * User: liuya 14 * Date: 2018/3/24 15 * Time: 22:10 16 * projectName:20180324versionone 17 */ 18 public class Test { 19 20 public static void main(String[] args) { 21 // 通过Java配置来实例化Spring容器 22 AnnotationConfigApplicationContext context = new AnnotationConfigApplicationContext(SpringConf.class); 23 24 // 在Spring容器中获取Bean对象 25 UserService userService = context.getBean(UserService.class); 26 27 // 调用对象中的方法 28 List<User> list = userService.userList(); 29 30 for (User user : list) { 31 System.out.println(user.getUserName() + ", " + user.getPassWord() + ", " + user.getPassWord()); 32 } 33 34 // 销毁该容器 35 context.destroy(); 36 } 37 38 39 }
(8)成功运行
(9)原理图解