index.tsx
// users/index.tsx
import React from 'react';
import styles from './IndexPage.css';
import { connect } from 'dva';
const index = (props: any) => {
const { dispatch, count } = props;
console.log(props, count, count.current)
const changeAdd = () => {
// dispatch({ type: 'count/add1', payload: { record: 10, current: 21, name: 'add' } })
dispatch({ type: 'count/getRemote' })
}
const changeMinus = (val: any) => {
console.log('changeMinus', val)
dispatch({ type: 'count/minus', payload: { record: 10, current: 21, name: 'add' } })
}
return (
<div className={styles.normal}>
<div className={styles.record}>
Highest Record: {count.record}
</div>
<div className={styles.current}>
{count.current}
</div>
<div className={styles.button}>
<button onClick={changeAdd} >
+
</button>
</div>
<div className={styles.button}>
<button onClick={() => changeMinus('sss')} >
-
</button>
</div>
</div >
);
}
function mapStateToProps({ count }: any) {
console.log('count', count)
return { count }
//return { count: count };
} // 获取state
//export default connect(({ count }) => ({ count }))(index)
export default connect(mapStateToProps)(index);
model.ts
import { delay } from '../../utils/utils';
import { getRemoteList } from './service';
import key from 'keymaster';
export default {
namespace: 'count',
state: {
record: 0,
current: 0,
},
reducers: {
add1(state: any, { payload }: any) {
console.log('ad', state, payload)
const newCurrent = state.current + 1;
return {
...state,
record: newCurrent > state.record ? newCurrent : state.record,
current: newCurrent,
};
},
minus(state: any) {
console.log('我进来了')
return { ...state, current: state.current - 1 };
},
},
effects: {
*getRemote(action, { put, call }) {
console.log('getRemoteList', getRemoteList)
yield call(delay, 1000)
const data = yield call(getRemoteList);
console.log('data', data)
yield put({
type: 'add1',
payload: data
})
}
},
subscriptions: {
keyboardWatcher({ dispatch }: any) {
console.log('我是建螃蟹')
key('⌘+r, ctrl+r', () => { dispatch({ type: 'minus' }) });
},
},
};
在typings.d.ts中
declare module 'keymaster';
在utils/utils.js
export function delay(timeout) { return new Promise((resolve) => { setTimeout(resolve, timeout); }); }
service.ts
// service.ts文件 export const getRemoteList = async (params: any) => { const data = [ { key: '1', name: 'John Brown', age: 32, address: 'New York No. 1 Lake Park', tags: ['nice', 'developer'], }, { key: '2', name: 'Jim Green', age: 42, address: 'London No. 1 Lake Park', tags: ['loser'], }, { key: '3', name: 'Joe Black', age: 32, address: 'Sidney No. 1 Lake Park', tags: ['cool', 'teacher'], }, ]; return data; }
总结:
reducers是同步方法,用来改变state的值,
dispatch({ type: 'count/add1', payload: { record: 10, current: 21, name: 'add' } }
effects既可以异步也可以同步(一般用来异步)
比如同步dispatch({ type: 'count/getRemote' })
异步
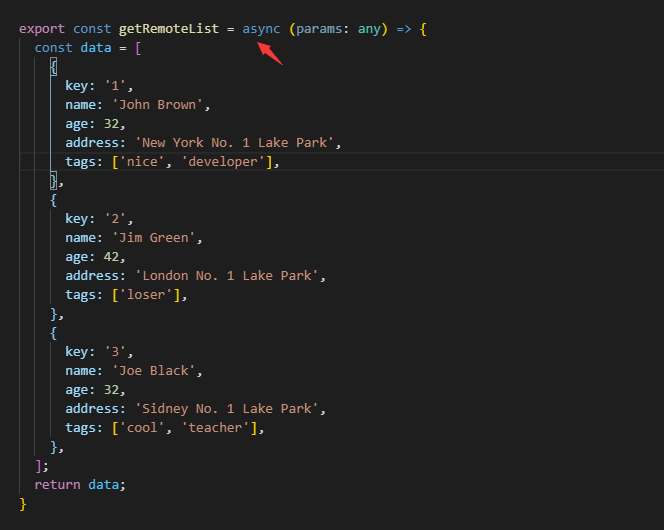
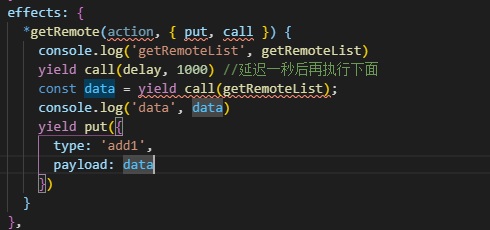
点击+按钮
f12控制台
subscriptions
以 key/value 格式定义 subscription,subscription 是订阅,用于订阅一个数据源,然后根据需要 dispatch 相应的 action
在 app.start() 时被执行,数据源可以是当前的时间、服务器的 websocket 连接、keyboard 输入、geolocation 变化、history 路由变化等等
格式为 ({ dispatch, history }, done) => unlistenFunction
注意:如果要使用 app.unmodel(),subscription 必须返回 unlisten 方法,用于取消数据订阅
pathname值为url后面的路由
// subscriptions 写法 subscriptions: { setup({ dispatch, history }) { // 监听路由的变化,请求页面数据 return history.listen(({ pathname, search }) => { const query = queryString.parse(search) let list = [] if (pathname === 'todoList') { dispatch({ type: 'save', payload: {list} }) } }) } }
参考https://www.jianshu.com/p/e184cd6d253c?utm_campaign=maleskine&utm_content=note&utm_medium=seo_notes&utm_source=recommendation
https://juejin.cn/post/6983855069850501127#heading-6
https://juejin.cn/post/6844903669301788685#heading-4