掌握
• 1.项目中常见文件(Info.plist和pch文件的作用)
-
Info.plist
1>Info.plist常见的设置
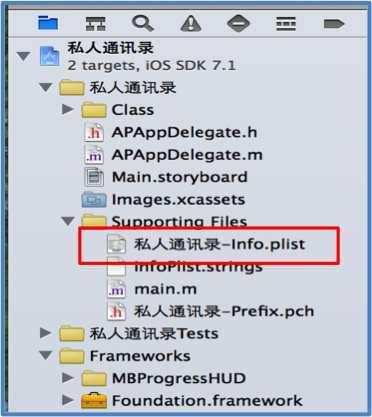
2>常见属性(红色部分是用文本编辑器打开时看到的key)
-
pch文件
#ifdef DEBUG #define Log(...) NSLog(__VA_ARGS__) #else #define Log(...) /* */ #endif
• 2.UIApplication
-
什么是UIApplication
-
UIApplication的常用属性
@property(nonatomic) NSInteger applicationIconBadgeNumber; 如写入下列代码后,应用图标将显示:
[UIApplication sharedApplication].applicationIconBadgeNumber = 10;
@property(nonatomic,getter=isNetworkActivityIndicatorVisible) BOOL networkActivityIndicatorVisible; 如写入下列代码后,应用图标将显示:
[[UIApplication sharedApplication] setNetworkActivityIndicatorVisible:YES];
-
UIApplication之强大的openURL方法
UIApplication有个功能十分强大的openURL:方法 - (BOOL)openURL:(NSURL*)url; openURL:方法的部分功能有 // 打电话 UIApplication *app = [UIApplication sharedApplication]; [app openURL:[NSURL URLWithString:@"tel://10086"]]; // 发短信 [app openURL:[NSURL URLWithString:@"sms://10086"]]; // 发邮件 [app openURL:[NSURL URLWithString:@"mailto://841657484@qq.com"]]; // 打开一个网页资源 [app openURL:[NSURL URLWithString:@"http://home.cnblogs.com/u/lszwhb/"]]; 通过上面的方法可以打开对应的其他app程序 不用你考虑要打开什么应用程序,系统会自动识别帮你打开
-
iOS7中的状态栏
ps: ios7之前,状态栏由UIApplication管理,状态栏和UIViewController是分离独立的。
- (UIStatusBarStyle)preferredStatusBarStyle;
• 状态栏的可见性
- (BOOL)prefersStatusBarHidden; 例如在UIViewController中写入下列方法,状态栏就隐藏了
- (BOOL)prefersStatusBarHidden { return YES; }

• 3.UIApplicationDelegate(AppDelegate的代理方法)
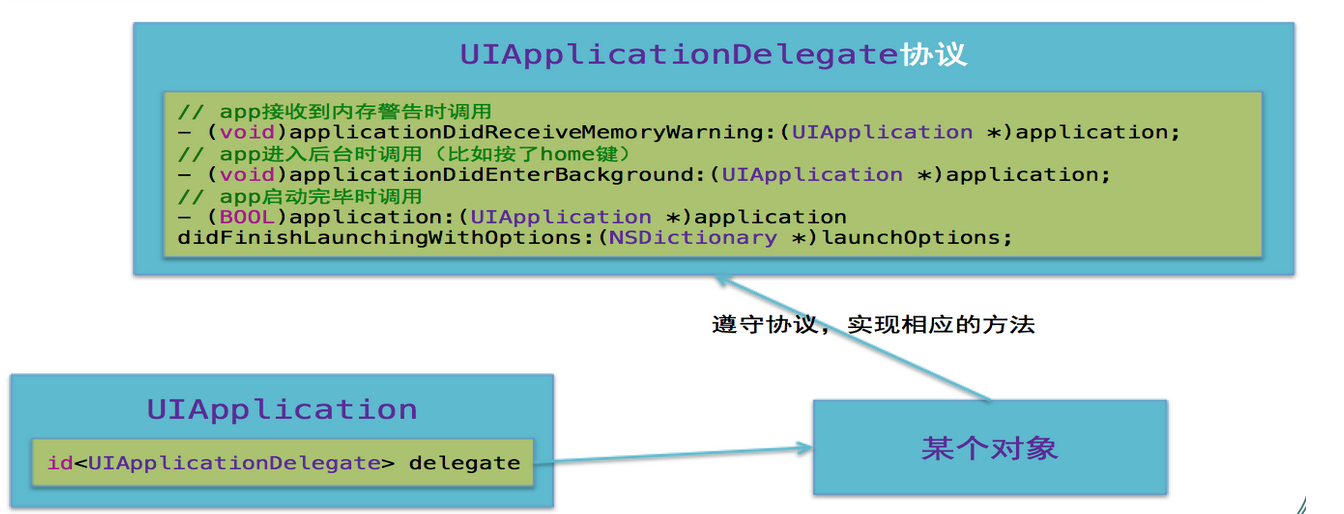
ØAPAppDelegate默认已经遵守了UIApplicationDelegate协议,已经是UIApplication的代理
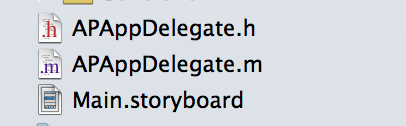
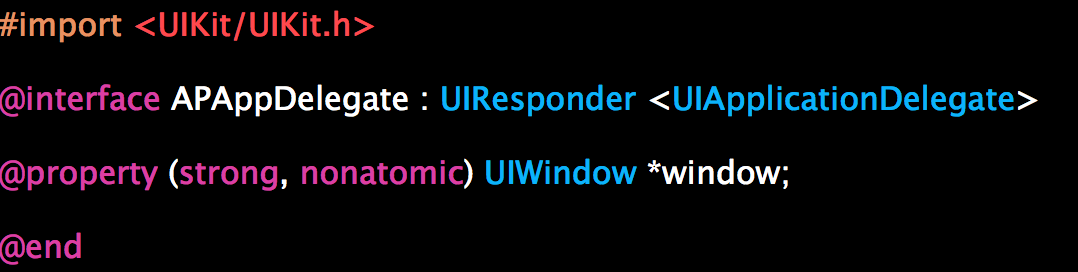

/** * app启动完毕后就会调用 */ - (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions { // Override point for customization after application launch. return YES; } /** * app程序失去焦点就会调用 */ - (void)applicationWillResignActive:(UIApplication *)application { // Sent when the application is about to move from active to inactive state. This can occur for certain types of temporary interruptions (such as an incoming phone call or SMS message) or when the user quits the application and it begins the transition to the background state. // Use this method to pause ongoing tasks, disable timers, and throttle down OpenGL ES frame rates. Games should use this method to pause the game. } /** * app进入后台的时候调用 * * 一般在这里保存应用的数据(游戏数据,比如暂停游戏) */ - (void)applicationDidEnterBackground:(UIApplication *)application { // Use this method to release shared resources, save user data, invalidate timers, and store enough application state information to restore your application to its current state in case it is terminated later. // If your application supports background execution, this method is called instead of applicationWillTerminate: when the user quits. } /** * app程序程序从后台回到前台就会调用 */ - (void)applicationWillEnterForeground:(UIApplication *)application { // Called as part of the transition from the background to the inactive state; here you can undo many of the changes made on entering the background. } /** * app程序获取焦点就会调用 */ - (void)applicationDidBecomeActive:(UIApplication *)application { // Restart any tasks that were paused (or not yet started) while the application was inactive. If the application was previously in the background, optionally refresh the user interface. } /** * 内存警告,可能要终止程序,清除不需要再使用的内存 */ - (void)applicationDidReceiveMemoryWarning:(UIApplication *)application { } /** * 程序即将退出调用 */ - (void)applicationWillTerminate:(UIApplication *)application { // Called when the application is about to terminate. Save data if appropriate. See also applicationDidEnterBackground:. }
• 4.iOS程序的完整启动过程(UIApplication、AppDelegate、UIWindow、UIViewController的关系)
程序启动的完整过程
1.main函数
2.UIApplicationMain
* 创建UIApplication对象
* 创建UIApplication的delegate对象
3.delegate对象开始处理(监听)系统事件(没有storyboard)
* 程序启动完毕的时候, 就会调用代理的application:didFinishLaunchingWithOptions:方法
* 在application:didFinishLaunchingWithOptions:中创建UIWindow
* 创建和设置UIWindow的rootViewController
* 显示窗口
3.根据Info.plist获得最主要storyboard的文件名,加载最主要的storyboard(有storyboard)
* 创建UIWindow
* 创建和设置UIWindow的rootViewController
* 显示窗口