A. Squats
time limit per test:1 second
memory limit per test:256 megabytes
input:standard input
output:standard output
Pasha has many hamsters and he makes them work out. Today, n hamsters (n is even) came to work out. The hamsters lined up and each hamster either sat down or stood up.
For another exercise, Pasha needs exactly hamsters to stand up and the other hamsters to sit down. In one minute, Pasha can make some hamster ether sit down or stand up. How many minutes will he need to get what he wants if he acts optimally well?
The first line contains integer n (2 ≤ n ≤ 200; n is even). The next line contains n characters without spaces. These characters describe the hamsters' position: the i-th character equals 'X', if the i-th hamster in the row is standing, and 'x', if he is sitting.
In the first line, print a single integer — the minimum required number of minutes. In the second line, print a string that describes the hamsters' position after Pasha makes the required changes. If there are multiple optimal positions, print any of them.
4
xxXx
1
XxXx
2
XX
1
xX
6
xXXxXx
xXXxXx
题意 : X代表站着,x代表蹲着,要求n只中正好有一半是蹲着一半是站着,让一只仓鼠由蹲变为站或由站变为蹲需要1分钟,问最少需要多少分钟能够达到要求。

1 #include <stdio.h> 2 #include <string.h> 3 #include <iostream> 4 5 using namespace std ; 6 char sh[210] ; 7 int main() 8 { 9 int n ; 10 while(~scanf("%d",&n)) 11 { 12 scanf("%s",sh) ; 13 int cnt = 0 ; 14 for(int i = 0 ; i < n ; i++) 15 if(sh[i] == 'X') 16 cnt++ ; 17 if(cnt == n/2) 18 { 19 printf("0 %s ",sh) ; 20 } 21 else if(cnt > n/2) 22 { 23 printf("%d ",cnt-n/2) ; 24 for(int i = 0 ,k = 0; i < n && k < cnt-n/2 ; i++) 25 if(sh[i] == 'X') 26 { 27 sh[i] = 'x'; 28 k++ ; 29 } 30 printf("%s ",sh) ; 31 } 32 else if(cnt < n/2) 33 { 34 printf("%d ",n/2-cnt) ; 35 for(int i = 0 ,k = 0; i < n && k < n/2-cnt ; i++) 36 if(sh[i] == 'x') 37 { 38 sh[i] = 'X'; 39 k++ ; 40 } 41 printf("%s ",sh) ; 42 } 43 } 44 return 0 ; 45 }
B. Megacity
time limit per test:1 second
memory limit per test:256 megabytes
input:standard input
output:standard output
The administration of the Tomsk Region firmly believes that it's time to become a megacity (that is, get population of one million). Instead of improving the demographic situation, they decided to achieve its goal by expanding the boundaries of the city.
The city of Tomsk can be represented as point on the plane with coordinates (0; 0). The city is surrounded with n other locations, the i-th one has coordinates (xi, yi) with the population of ki people. You can widen the city boundaries to a circle of radius r. In such case all locations inside the circle and on its border are included into the city.
Your goal is to write a program that will determine the minimum radius r, to which is necessary to expand the boundaries of Tomsk, so that it becomes a megacity.
The first line of the input contains two integers n and s (1 ≤ n ≤ 103; 1 ≤ s < 106) — the number of locatons around Tomsk city and the population of the city. Then n lines follow. The i-th line contains three integers — the xi and yi coordinate values of the i-th location and the number ki of people in it (1 ≤ ki < 106). Each coordinate is an integer and doesn't exceed 104 in its absolute value.
It is guaranteed that no two locations are at the same point and no location is at point (0; 0).
In the output, print "-1" (without the quotes), if Tomsk won't be able to become a megacity. Otherwise, in the first line print a single real number — the minimum radius of the circle that the city needs to expand to in order to become a megacity.
The answer is considered correct if the absolute or relative error don't exceed 10 - 6.
4 999998
1 1 1
2 2 1
3 3 1
2 -2 1
2.8284271
4 999998
1 1 2
2 2 1
3 3 1
2 -2 1
1.4142136
2 1
1 1 999997
2 2 1
题意 : 周围有n个城市,这个城市目前有k个人,这个城市要往外扩充,来让人数达到1000000,以0,0为圆心,问你找一个半径为r的圆,要使r尽量小并且这个城市扩充之后的人数至少为1000000 。

1 #include <stdio.h> 2 #include <string.h> 3 #include <math.h> 4 #include <algorithm> 5 #include <iostream> 6 7 using namespace std ; 8 9 struct node 10 { 11 double x,y ; 12 int num ; 13 } p[1100]; 14 15 int cmp(const node &a,const node &b) 16 { 17 if(sqrt(a.x*a.x+a.y*a.y) == sqrt(b.x*b.x+b.y*b.y)) 18 return a.num > b.num ; 19 return sqrt(a.x*a.x+a.y*a.y) < sqrt(b.x*b.x+b.y*b.y) ; 20 } 21 int main() 22 { 23 int n , k; 24 while(~scanf("%d %d",&n,&k)) 25 { 26 int sum = 0 ; 27 for(int i = 0 ; i < n ; i++) 28 { 29 scanf("%lf %lf %d",&p[i].x,&p[i].y,&p[i].num) ; 30 sum += p[i].num ; 31 } 32 if(sum + k < 1000000) 33 { 34 printf("-1 ") ; 35 continue ; 36 } 37 sort(p,p+n,cmp) ; 38 int cnt = 1000000-k,j,i ; 39 for(i = 0 ,j = cnt; i < n && j >= 0 ; i++) 40 { 41 if(p[i].num >= j) 42 break ; 43 else { 44 j -= p[i].num ; 45 } 46 47 } 48 printf("%.7lf ",sqrt(p[i].x*p[i].x+p[i].y*p[i].y)) ; 49 } 50 return 0 ; 51 }
C. Magic Formulas
time limit per test:1 second
memory limit per test:256 megabytes
input:standard input
output:standard output
People in the Tomskaya region like magic formulas very much. You can see some of them below.
Imagine you are given a sequence of positive integer numbers p1, p2, ..., pn. Lets write down some magic formulas:


Here, "mod" means the operation of taking the residue after dividing.
The expression means applying the bitwise xor (excluding "OR") operation to integers x and y. The given operation exists in all modern programming languages. For example, in languages C++ and Java it is represented by "^", in Pascal — by "xor".
People in the Tomskaya region like magic formulas very much, but they don't like to calculate them! Therefore you are given the sequence p, calculate the value of Q.
The first line of the input contains the only integer n (1 ≤ n ≤ 106). The next line contains n integers: p1, p2, ..., pn (0 ≤ pi ≤ 2·109).
The only line of output should contain a single integer — the value of Q.
3
1 2 3
题意 : 给你p1,p2.....pn,根据给定的两个式子,让你求出Q 。
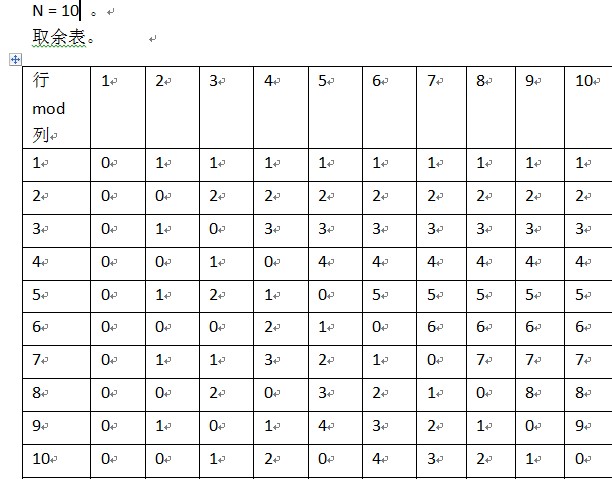

1 #include <stdio.h> 2 #include <iostream> 3 #include <string.h> 4 5 using namespace std ; 6 7 long long a[1000010] ; 8 long long m ; 9 10 void table() 11 { 12 a[0] = 0 ; 13 a[1] = 1 ; 14 for(int i = 2 ; i < 1000010 ; i++) 15 a[i] = a[i-1] ^ i ; 16 } 17 18 int main() 19 { 20 int n ; 21 table() ; 22 while(~scanf("%d",&n)) 23 { 24 long long sum = 0 ; 25 for(int i = 1 ; i <= n ; i++) 26 { 27 scanf("%I64d",&m) ; 28 sum = sum ^ m ; 29 } 30 for(int i = 2 ; i <= n ; i++) 31 { 32 int rest = n % i ; 33 int t = n / i ; 34 if(t % 2) 35 { 36 sum ^= a[i-1] ; 37 if(rest != 0) 38 sum ^= a[rest] ; 39 } 40 else 41 { 42 if(rest != 0) 43 sum ^= a[rest] ; 44 } 45 } 46 printf("%I64d ",sum) ; 47 } 48 return 0 ; 49 }
这个代码其实有更为简单的写法。。。。。

1 #include<stdio.h> 2 3 long long sum,a[1234567],n,m; 4 5 int main() 6 { 7 while(~scanf("%I64d",&n)) 8 { 9 for(int i = 1 ; i <= n ; i++) 10 { 11 a[i] = a[i-1] ^ i ; 12 scanf("%I64d",&m) ; 13 sum ^= m ^ a[n % i] ^ ( n / i % 2 ? a[i-1] : 0 ); 14 } 15 printf("%I64d ",sum) ; 16 } 17 return 0; 18 }
很神奇有没有。。。。