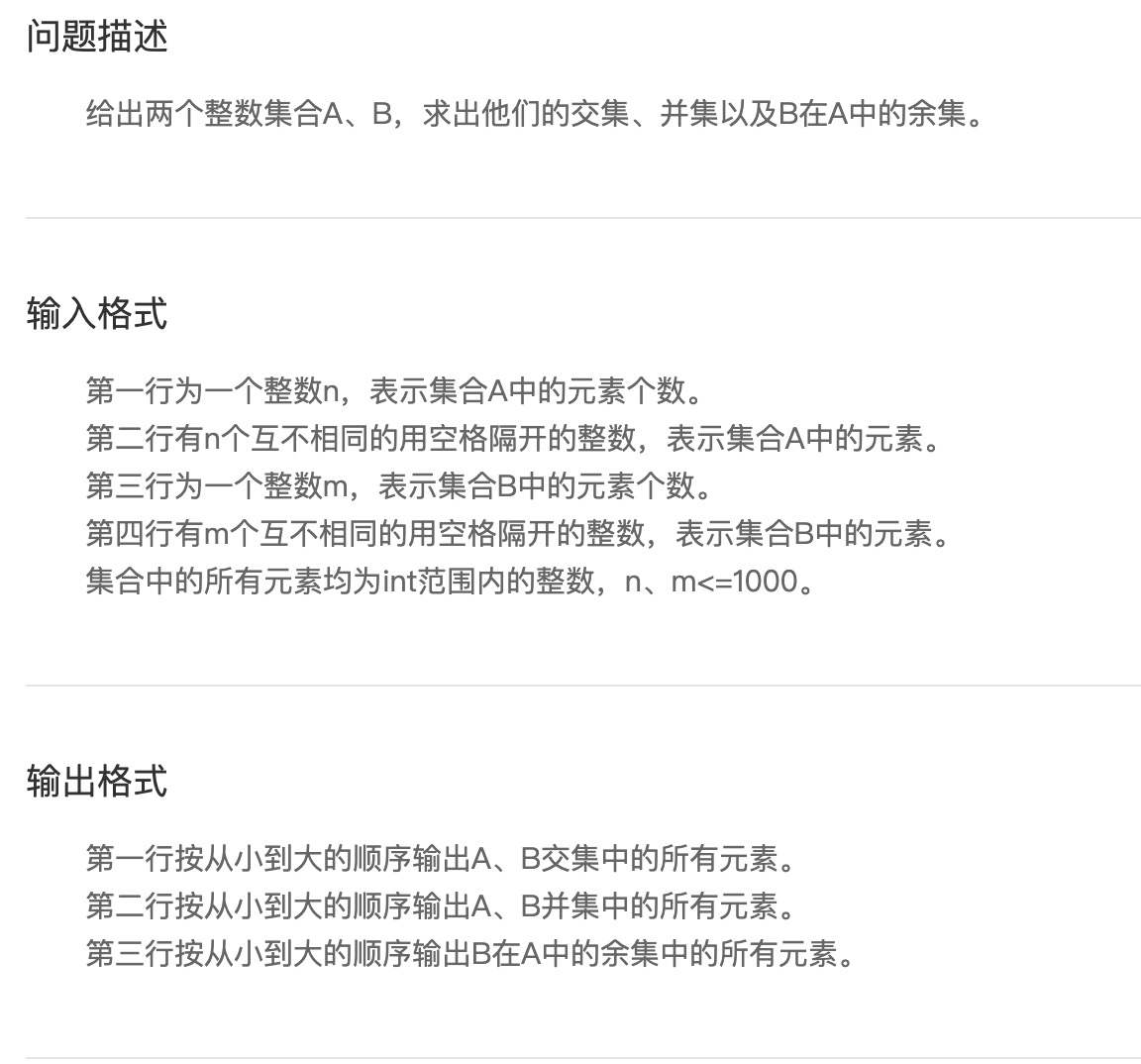
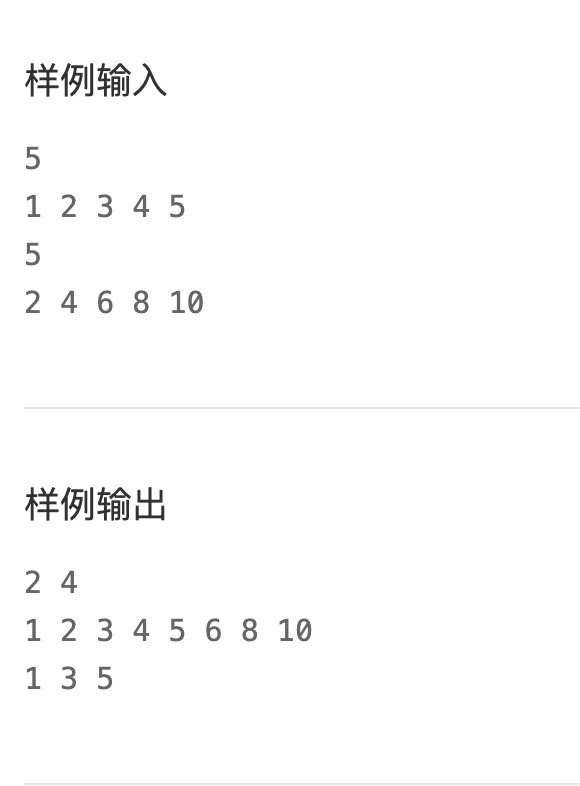
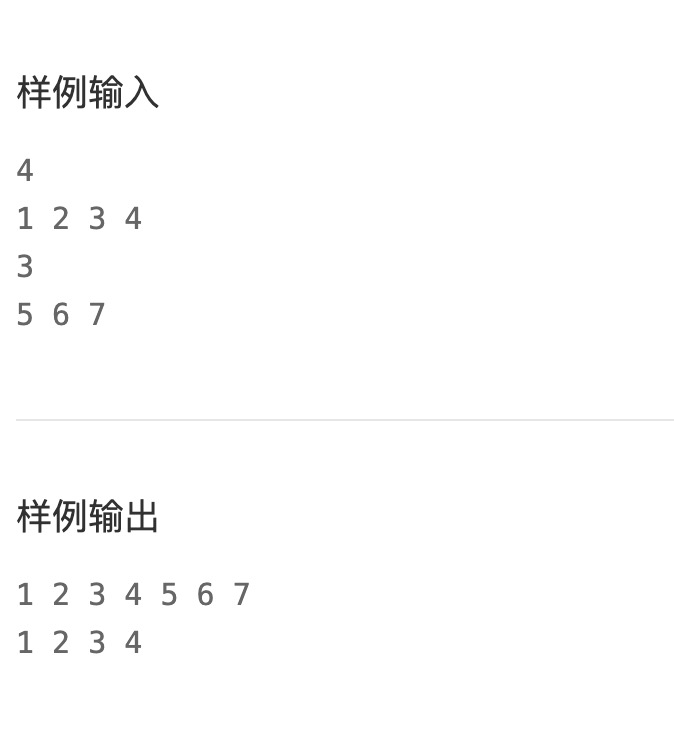
解析: 根据交集、并集和余集的特性,封装了三个方法分别进行处理。因为交集、并集和余集中的数字个数都是不确定的,所以用集合ArrayList接收数据比较适合,再将集合转变成数组,对数组进行升序处理就得到了最终的结果。
package _4_9_test;
import java.lang.reflect.Array;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Scanner;
import java.util.concurrent.locks.ReentrantLock;
/* 集合运算
* B在A中的余集,A中除去A和B相交元素的所有元素
* */
public class NightyThree {
public static void main(String[] args) {
// TODO Auto-generated method stub
Scanner scanner = new Scanner(System.in);
// 得到两个输入的数组
int n = scanner.nextInt();
int A[] = new int[n];
for (int i = 0; i < n; i++) {
A[i] = scanner.nextInt();
}
int m = scanner.nextInt();
int B[] = new int[m];
for (int i = 0; i < m; i++) {
B[i] = scanner.nextInt();
}
// 调用三个方法,返回三个集合的结果
Object jiaoji[] = jiao(A, B);
Object bingji[] = bing(A, B);
Object yuji[] = yu(A, B);
// 输出三个集合的结果
for (Object i : jiaoji) {
System.out.print(i + " ");
}
System.out.println();
for (Object i : bingji) {
System.out.print(i + " ");
}
System.out.println();
for (Object i : yuji) {
System.out.print(i + " ");
}
}
// 交集
public static Object[] jiao(int a[], int b[]) {
ArrayList<Integer> arrayList = new ArrayList<>();
// 如果两个数组中有相同的数,就添加到集合中
for (int i = 0; i < a.length; i++) {
for (int j = 0; j < b.length; j++) {
if (a[i] == b[j]) {
arrayList.add(a[i]);
}
}
}
// 将集合转成数组
Object[] result = new Object[arrayList.size()];
result = arrayList.toArray();
// 对数组进行升序排序
Arrays.sort(result);
return result;
}
// 并集
public static Object[] bing(int a[], int b[]) {
ArrayList<Integer> arrayList = new ArrayList<Integer>();
// 先将第一个数组中有,第二个数组中没有的数字添加进集合中。
// 再将第二个数组中所有的数字添加进集合中
for (int i = 0; i < a.length; i++) {
boolean flag = true;
for (int j = 0; j < b.length; j++) {
if (a[i] == b[j]) {
flag = false;
break;
}
}
if (flag) {
arrayList.add(a[i]);
}
}
for (int i = 0; i < b.length; i++) {
arrayList.add(b[i]);
}
Object[] result = new Object[arrayList.size()];
result = arrayList.toArray();
Arrays.sort(result);
return result;
}
// 余集
public static Object[] yu(int a[], int b[]) {
ArrayList<Integer> arrayList = new ArrayList<Integer>();
// 将第一个数组中有,第二个数组中没有的数字添加进集合中。
for (int i = 0; i < a.length; i++) {
boolean flag = true;
for (int j = 0; j < b.length; j++) {
if (a[i] == b[j]) {
flag = false;
}
}
if (flag) {
arrayList.add(a[i]);
}
}
Object[] result = new Object[arrayList.size()];
result = arrayList.toArray();
Arrays.sort(result);
return result;
}
}