Masha has recently bought a cleaner robot, it can clean a floor without anybody's assistance.
Schematically Masha's room is a rectangle, consisting of w × h square cells of size 1 × 1. Each cell of the room is either empty (represented by character '.'), or occupied by furniture (represented by character '*').
A cleaner robot fully occupies one free cell. Also the robot has a current direction (one of four options), we will say that it looks in this direction.
The algorithm for the robot to move and clean the floor in the room is as follows:
- clean the current cell which a cleaner robot is in;
- if the side-adjacent cell in the direction where the robot is looking exists and is empty, move to it and go to step 1;
- otherwise turn 90 degrees clockwise (to the right relative to its current direction) and move to step 2.
The cleaner robot will follow this algorithm until Masha switches it off.
You know the position of furniture in Masha's room, the initial position and the direction of the cleaner robot. Can you calculate the total area of the room that the robot will clean if it works infinitely?
The first line of the input contains two integers, w and h (1 ≤ w, h ≤ 10) — the sizes of Masha's room.
Next w lines contain h characters each — the description of the room. If a cell of a room is empty, then the corresponding character equals '.'. If a cell of a room is occupied by furniture, then the corresponding character equals '*'. If a cell has the robot, then it is empty, and the corresponding character in the input equals 'U', 'R', 'D' or 'L', where the letter represents the direction of the cleaner robot. Letter 'U' shows that the robot is looking up according to the scheme of the room, letter 'R' means it is looking to the right, letter 'D' means it is looking down and letter 'L' means it is looking to the left.
It is guaranteed that in the given w lines letter 'U', 'R', 'D' or 'L' occurs exactly once. The cell where the robot initially stands is empty (doesn't have any furniture).
In the first line of the output print a single integer — the total area of the room that the robot will clean if it works infinitely.
2 3
U..
.*.
4
4 4
R...
.**.
.**.
....
12
3 4
***D
..*.
*...
6
In the first sample the robot first tries to move upwards, it can't do it, so it turns right. Then it makes two steps to the right, meets a wall and turns downwards. It moves down, unfortunately tries moving left and locks itself moving from cell (1, 3) to cell (2, 3) and back. The cells visited by the robot are marked gray on the picture.
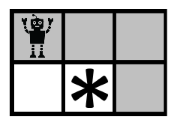
题意:
给你一个地图, ‘ . ’空地板,‘*’表示家具,字母表示机器人的位置,‘R''L'‘D’‘U’表示方向,
当机器人不能前进时,就顺时针旋转九十度,继续前进,注意相同的路,可以被重复访问
思路:
模拟题,dfs模拟每个状态,当经过某地的次数很大时退出
AC代码:

1 # include <iostream> 2 # include <cstring> 3 # include <cstdio> 4 using namespace std; 5 6 const int MAX = 12; 7 8 char map[MAX][MAX]; 9 int vis[MAX][MAX]; 10 int n, m; 11 12 bool ok(int x, int y) 13 { 14 if(x >= 1 && x <= n && y >= 1 && y <= m) 15 return true; 16 return false; 17 } 18 19 void dfs(int x, int y, char ch) 20 { 21 if(vis[x][y] > 10) 22 return; 23 vis[x][y]++; 24 25 if(ch == 'U') 26 { 27 int newx = x - 1; 28 int newy = y; 29 if(!ok(newx, newy) || map[newx][newy] == '*') 30 dfs(x, y, 'R'); 31 else 32 dfs(newx, newy, 'U'); 33 } 34 else if(ch == 'R') 35 { 36 int newx = x; 37 int newy = y + 1; 38 if(!ok(newx, newy) || map[newx][newy] == '*') 39 dfs(x, y, 'D'); 40 else 41 dfs(newx, newy, 'R'); 42 } 43 else if(ch == 'D') 44 { 45 int newx = x + 1; 46 int newy = y; 47 if(!ok(newx, newy) || map[newx][newy] == '*') 48 dfs(x, y, 'L'); 49 else 50 dfs(newx, newy, 'D'); 51 } 52 else if(ch == 'L') 53 { 54 int newx = x; 55 int newy = y - 1; 56 if(!ok(newx, newy) || map[newx][newy] == '*') 57 dfs(x, y, 'U'); 58 else 59 dfs(newx, newy, 'L'); 60 } 61 } 62 63 64 65 int main() 66 { 67 68 while(cin >> n >> m) 69 { 70 int h, l; 71 char ch; 72 73 memset(vis, 0, sizeof(vis)); 74 for(int i = 0; i <= MAX; i++) 75 for(int j = 0; j <= MAX; j++) 76 map[i][j] = '*'; 77 78 for(int i = 1; i <= n; i++) 79 for(int j = 1; j <= m; j++) 80 { 81 cin >> map[i][j]; 82 if(map[i][j] == 'U' || map[i][j] == 'R' || map[i][j] == 'D' || map[i][j] == 'L') 83 { 84 h = i; 85 l = j; 86 ch = map[i][j]; 87 } 88 } 89 90 dfs(h, l, ch); 91 92 int jishu = 0; 93 for(int i = 1; i <= n; i++) 94 for(int j = 1; j <= m; j++) 95 { 96 if(vis[i][j]) 97 jishu++; 98 } 99 cout << jishu << endl; 100 101 } 102 return 0; 103 }