前言
异常提供了一种从错误状况进行可靠恢复的途径
异常的分类
Java中的异常大体可以分为两类:checkedException,runtimeException
- checkedException 编译阶段异常,其为可检测到的异常,程序要求强制处理,应用场景:如文件读取
- runtimeException 运行时异常,不要求强制处理,应用场景:数据库读取数据
异常处理的分类
异常的处理大致可分为两类:
- 继续抛出:继续向上层抛出(throws)
- 主动处理:如try catch 进行日志记录
throw和throws的区别
- throw 用在函数内,后面跟的是异常对象。当程序执行到throw功能就已经结束了,具体的问题对象会抛给调用者。
- throws 用在函数上,后面跟的是异常类(可以跟多个)用来声明异常,让调用者知道该函数可能会出现的问题。
SpringBoot全局异常处理
全局异常统一封装
异常类定义
- HttpException.java
/**
* @Description Http异常类
* @author coisini
* @date Aug 10, 2021
* @Version 1.0
*/
public class HttpException extends RuntimeException{
protected Integer code;
protected Integer httpStatusCode = 500;
public Integer getCode() {
return code;
}
public Integer getHttpStatusCode() {
return httpStatusCode;
}
}
- NotFoundException.java
/**
* @Description http 404 异常
* @author coisini
* @date Aug 9, 2021
* @Version 1.0
*/
public class NotFoundException extends HttpException{
public NotFoundException(int code) {
this.httpStatusCode = 404;
this.code = code;
}
}
统一返回定义
- UnifyMessage.java
/**
* @Description 统一消息返回
* @author coisini
* @date Aug 9, 2021
* @Version 1.0
*/
public class UnifyMessage {
private int code;
private String message;
private String requestUrl;
public int getCode() {
return code;
}
public String getMessage() {
return message;
}
public String getRequestUrl() {
return requestUrl;
}
public UnifyMessage(int code, String message, String requestUrl) {
this.code = code;
this.message = message;
this.requestUrl = requestUrl;
}
}
异常信息配置
- exception-code.properties(目录:srcmain esourcesconfigexception-code.properties)
coisini.codes[404] = 资源不存在
异常Code码配置
- ExceptionCodeConfiguration.class
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.context.annotation.PropertySource;
import org.springframework.stereotype.Component;
import java.util.HashMap;
import java.util.Map;
/**
* @Description 异常Code码配置类
* @author coisini
* @date Aug 10, 2021
* @Version 1.0
*/
@ConfigurationProperties(prefix = "coisini")
@PropertySource(value = "classpath:config/exception-code.properties")
@Component
public class ExceptionCodeConfiguration {
private Map<Integer,String> codes = new HashMap<>();
public Map<Integer, String> getCodes() {
return codes;
}
public void setCodes(Map<Integer, String> codes) {
this.codes = codes;
}
public String getMessage(int code) {
String message = codes.get(code);
return message;
}
}
全局异常统一处理
import com.coisini.springbootlearn.config.ExceptionCodeConfiguration;
import com.coisini.springbootlearn.exception.http.HttpException;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpStatus;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.bind.annotation.ResponseStatus;
import javax.servlet.http.HttpServletRequest;
/**
* @Description 全局异常统一处理
* @author coisini
* @date Aug 9, 2021
* @Version 1.0
*/
@ControllerAdvice
public class GlobalExceptionAdvice {
@Autowired
private ExceptionCodeConfiguration exceptionCodeConfiguration;
/**
* 通用异常、未知异常处理器
*/
@ExceptionHandler(value = Exception.class)
@ResponseBody
@ResponseStatus(code = HttpStatus.INTERNAL_SERVER_ERROR)
public UnifyMessage handleException(HttpServletRequest request, Exception e) {
String method = request.getMethod();
String requestUrl = request.getRequestURI();
System.out.println(e);
UnifyMessage message = new UnifyMessage(9999, "服务异常", method + " " + requestUrl);
return message;
}
/**
* http异常处理器
* @param request
* @param e
*/
@ExceptionHandler(HttpException.class)
public ResponseEntity handleHttpException (HttpServletRequest request, HttpException e) {
String method = request.getMethod();
String requestUrl = request.getRequestURI();
System.out.println(e);
UnifyMessage message = new UnifyMessage(e.getCode(), exceptionCodeConfiguration.getMessage(e.getCode()), method + " " + requestUrl);
// 设置返回类型
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_JSON);
HttpStatus status = HttpStatus.resolve(e.getHttpStatusCode());
ResponseEntity<UnifyMessage> responseEntity = new ResponseEntity<>(message, headers, status);
return responseEntity;
}
}
异常测试
- 通用异常测试
@RestController
@RequestMapping("/exception")
public class ExceptionController {
@GetMapping(value = "/test")
public String test(){
throw new RuntimeException("通用异常");
}
}
- 测试结果
- 自定义http异常测试
@GetMapping(value = "/test1")
public String test1(){
throw new NotFoundException(404);
}
- 测试结果
中文乱码处理
由于异常Code码配置在properties文件中,properties默认的编码格式是ISO-8859-1,响应信息中文会出现乱码,需要手动设置编码为UTF-8,如下IDEA配置,配置后不能正常显示需重启编译器。
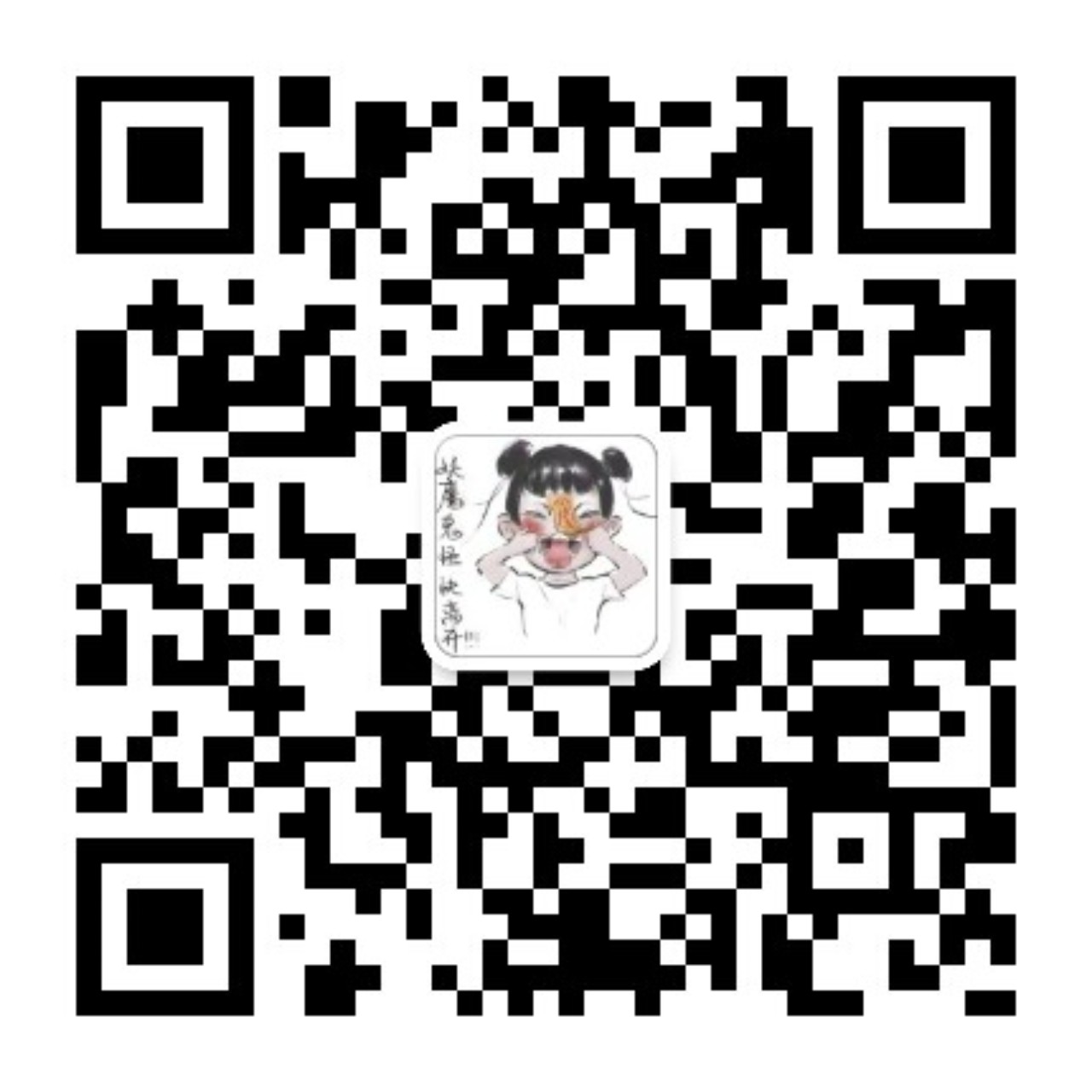