ASP.NET Page Life Cycle Overview
When an ASP.NET page runs, the page goes through a life cycle in which it performs a series of processing steps. These include initialization, instantiating controls, restoring and maintaining state, running event handler code, and rendering. It is important for you to understand the page life cycle so that you can write code at the appropriate life-cycle stage for the effect you intend.
If you develop custom controls, you must be familiar with the page life cycle in order to correctly initialize controls, populate control properties with view-state data, and run control behavior code. The life cycle of a control is based on the page life cycle, and the page raises many of the events that you need to handle in a custom control.
This topic contains the following sections:
In general terms, the page goes through the stages outlined in the following table. In addition to the page life-cycle stages, there are application stages that occur before and after a request but are not specific to a page.
Some parts of the life cycle occur only when a page is processed as a postback. For postbacks, the page life cycle is the same during a partial-page postback (as when you use an UpdatePanel control) as it is during a full-page postback.Stage | Description |
---|---|
Page request | The page request occurs before the page life cycle begins. When the page is requested by a user, ASP.NET determines whether the page needs to be parsed and compiled (therefore beginning the life of a page), or whether a cached version of the page can be sent in response without running the page. |
Start | In the start stage, page properties such as Request and Response are set. At this stage, the page also determines whether the request is a postback or a new request and sets the IsPostBack property. The page also sets the UICulture property. |
Initialization | During page initialization, controls on the page are available and each control's UniqueID property is set. A master page and themes are also applied to the page if applicable. If the current request is a postback, the postback data has not yet been loaded and control property values have not been restored to the values from view state. |
Load | During load, if the current request is a postback, control properties are loaded with information recovered from view state and control state. |
Postback event handling | If the request is a postback, control event handlers are called. After that, the Validate method of all validator controls is called, which sets the IsValidproperty of individual validator controls and of the page. (There is an exception to this sequence: the handler for the event that caused validation is called after validation.) |
Rendering | Before rendering, view state is saved for the page and all controls. During the rendering stage, the page calls the Render method for each control, providing a text writer that writes its output to the OutputStream object of the page's Response property. |
Unload | The Unload event is raised after the page has been fully rendered, sent to the client, and is ready to be discarded. At this point, page properties such as Response and Request are unloaded and cleanup is performed. |
Within each stage of the life cycle of a page, the page raises events that you can handle to run your own code. For control events, you bind the event handler to the event, either declaratively using attributes such as onclick, or in code.
Pages also support automatic event wire-up, meaning that ASP.NET looks for methods with particular names and automatically runs those methods when certain events are raised. If the AutoEventWireup attribute of the @ Page directive is set to true, page events are automatically bound to methods that use the naming convention of Page_event, such as Page_Load and Page_Init. For more information on automatic event wire-up, see ASP.NET Web Server Control Event Model.
The following table lists the page life-cycle events that you will use most frequently. There are more events than those listed; however, they are not used for most page-processing scenarios. Instead, they are primarily used by server controls on the ASP.NET Web page to initialize and render themselves. If you want to write custom ASP.NET server controls, you need to understand more about these events. For information about creating custom controls, see Developing Custom ASP.NET Server Controls.
Page Event | Typical Use |
---|---|
Raised after the start stage is complete and before the initialization stage begins. Use this event for the following:
| |
Raised after all controls have been initialized and any skin settings have been applied. The Init event of individual controls occurs before theInit event of the page. Use this event to read or initialize control properties. | |
Raised at the end of the page's initialization stage. Only one operation takes place between the Init and InitComplete events: tracking of view state changes is turned on. View state tracking enables controls to persist any values that are programmatically added to the ViewStatecollection. Until view state tracking is turned on, any values added to view state are lost across postbacks. Controls typically turn on view state tracking immediately after they raise their Init event. Use this event to make changes to view state that you want to make sure are persisted after the next postback. | |
Raised after the page loads view state for itself and all controls, and after it processes postback data that is included with the Requestinstance. | |
The Page object calls the OnLoad method on the Page object, and then recursively does the same for each child control until the page and all controls are loaded. The Load event of individual controls occurs after the Load event of the page. Use the OnLoad event method to set properties in controls and to establish database connections. | |
Control events | Use these events to handle specific control events, such as a Button control's Click event or a TextBox control's TextChanged event. |
Raised at the end of the event-handling stage. Use this event for tasks that require that all other controls on the page be loaded. | |
Raised after the Page object has created all controls that are required in order to render the page, including child controls of composite controls. (To do this, the Page object calls EnsureChildControls for each control and for the page.) The Page object raises the PreRender event on the Page object, and then recursively does the same for each child control. The PreRenderevent of individual controls occurs after the PreRender event of the page. Use the event to make final changes to the contents of the page or its controls before the rendering stage begins. | |
Raised after each data bound control whose DataSourceID property is set calls its DataBind method. For more information, see Data Binding Events for Data-Bound Controls later in this topic. | |
Raised after view state and control state have been saved for the page and for all controls. Any changes to the page or controls at this point affect rendering, but the changes will not be retrieved on the next postback. | |
This is not an event; instead, at this stage of processing, the Page object calls this method on each control. All ASP.NET Web server controls have a Render method that writes out the control's markup to send to the browser. If you create a custom control, you typically override this method to output the control's markup. However, if your custom control incorporates only standard ASP.NET Web server controls and no custom markup, you do not need to override the Render method. For more information, see Developing Custom ASP.NET Server Controls. A user control (an .ascx file) automatically incorporates rendering, so you do not need to explicitly render the control in code. | |
Raised for each control and then for the page. In controls, use this event to do final cleanup for specific controls, such as closing control-specific database connections. For the page itself, use this event to do final cleanup work, such as closing open files and database connections, or finishing up logging or other request-specific tasks. ![]() During the unload stage, the page and its controls have been rendered, so you cannot make further changes to the response stream. If you attempt to call a method such as the Response.Write method, the page will throw an exception. |
Individual ASP.NET server controls have their own life cycle that is similar to the page life cycle. For example, a control's Init and Load events occur during the corresponding page events.
Although both Init and Load recursively occur on each control, they happen in reverse order. The Init event (and also the Unload event) for each child control occur before the corresponding event is raised for its container (bottom-up). However the Load event for a container occurs before the Load events for its child controls (top-down). Master pages behave like child controls on a page: the master page Init event occurs before the page Init and Load events, and the master page Loadevent occurs after the page Init and Load events.
When you create a class that inherits from the Page class, in addition to handling events raised by the page, you can override methods from the page's base class. For example, you can override the page's InitializeCulture method to dynamically set culture information. Note that when an event handler is created using thePage_event syntax, the base implementation is implicitly called and therefore you do not need to call it in your method. For example, the base page class's OnLoadmethod is always called, whether you create a Page_Load method or not. However, if you override the page OnLoad method with the override keyword (Overrides in Visual Basic), you must explicitly call the base method. For example, if you override the OnLoad method on the page, you must call base.Load(MyBase.Load in Visual Basic) in order for the base implementation to be run.
The following illustration shows some of the most important methods of the Page class that you can override in order to add code that executes at specific points in the page life cycle. (For a complete list of page methods and events, see the Page class.) The illustration also shows how these methods relate to page events and to control events. The sequence of methods and events in the illustration is from top to bottom, and within each row from left to right.
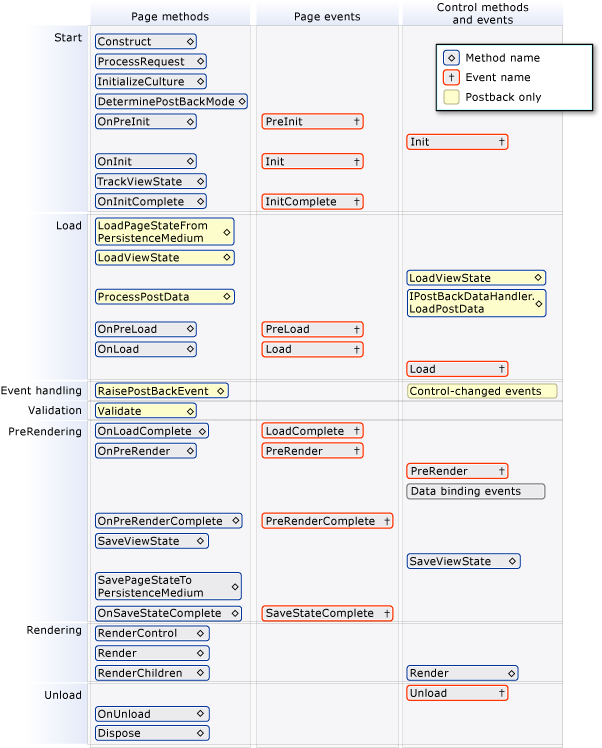
If controls are created dynamically at run time or declaratively within templates of data-bound controls, their events are initially not synchronized with those of other controls on the page. For example, for a control that is added at run time, the Init and Load events might occur much later in the page life cycle than the same events for controls created declaratively. Therefore, from the time that they are instantiated, dynamically added controls and controls in templates raise their events one after the other until they have caught up to the event during which it was added to the Controls collection.
To help you understand the relationship between the page life cycle and data binding events, the following table lists data-related events in data-bound controls such as the GridView, DetailsView, and FormView controls.
Control Event | Typical Use |
---|---|
Raised after the control's PreRender event, which occurs after the page's PreRender event. (This applies to controls whose DataSourceID property is set declaratively. Otherwise the event happens when you call the control's DataBind method.) This event marks the beginning of the process that binds the control to the data. Use this event to manually open database connections, if required, and to set parameter values dynamically before a query is run. | |
RowCreated (GridView only) or ItemCreated (DataList,DetailsView, SiteMapPath, DataGrid, FormView, Repeater, and ListView controls) | Raised after the control's DataBinding event. Use this event to manipulate content that is not dependent on data binding. For example, at run time, you might programmatically add formatting to a header or footer row in a GridView control. |
RowDataBound (GridView only) or ItemDataBound(DataList, SiteMapPath, DataGrid, Repeater, and ListViewcontrols) | Raised after the control's RowCreated or ItemCreated event. When this event occurs, data is available in the row or item, so you can format data or set theFilterExpression property on child data source controls in order to display related data within the row or item. |
Raised at the end of data-binding operations in a data-bound control. In a GridView control, data binding is complete for all rows and any child controls. Use this event to format data-bound content or to initiate data binding in other controls that depend on values from the current control's content. (For more information, see Catch-Up Events for Added Controls earlier in this topic.) |
Nested Data-Bound Controls
If a child control has been data bound, but its container control has not yet been data bound, the data in the child control and the data in its container control can be out of sync. This is true particularly if the data in the child control performs processing based on a data-bound value in the container control.
For example, suppose you have a GridView control that displays a company record in each row, and it displays a list of the company officers in a ListBox control. To fill the list of officers, you would bind the ListBox control to a data source control (such as SqlDataSource) that retrieves the company officer data using the company ID in a query.
If the ListBox control's data-binding properties, such as DataSourceID and DataMember, are set declaratively, the ListBox control will try to bind to its data source during the containing row's DataBinding event. However, the CompanyID field of the row does not contain a value until the GridView control's RowDataBound event occurs. In this case, the child control (the ListBox control) is bound before the containing control (the GridView control) is bound, so their data-binding stages are out of sync.
To avoid this condition, put the data source control for the ListBox control in the same template item as the ListBox control itself, and do not set the data binding properties of the ListBox declaratively. Instead, set them programmatically at run time during the RowDataBound event, so that the ListBox control does not bind to its data until the CompanyID information is available.
For more information, see Binding to Data Using a Data Source Control.
The Login control can use settings in the Web.config file to manage membership authentication automatically. However, if your application requires you to customize how the control works, or if you want to understand how Login control events relate to the page life cycle, you can use the events listed in the following table.
Control Event | Typical Use |
---|---|
Raised during a postback, after the page's LoadComplete event has occurred. This event marks the beginning of the login process. Use this event for tasks that must occur prior to beginning the authentication process. | |
Raised after the LoggingIn event. Use this event to override or enhance the default authentication behavior of a Login control. | |
Raised after the user name and password have been authenticated. Use this event to redirect to another page or to dynamically set the text in the control. This event does not occur if there is an error or if authentication fails. | |
Raised if authentication was not successful. Use this event to set text in the control that explains the problem or to direct the user to a different page. |
Server Event Handling in ASP.NET Web Pages
The following are the main ten steps in the ASP.NET page life cycle.
1. Object Initialization
A page's controls (and the page itself) are first initialized in their raw form. By declaring your objects within the constructor of your C# code-behind file, the page knows what types of objects and how many to create. Once you have declared your objects within your constructor, you may then access them from any sub class, method, event, or property. However, if any of your objects are controls specified within your ASPX file, at this point the controls have no attributes or properties. It is dangerous to access them through code, as there is no guarantee of what order the control instances will be created (if they are created at all). The initialization event can be overridden using the OnInit method.
2. Load Viewstate Data
After the Init event, controls can be referenced using their IDs only (no DOM is established yet for relative references). At LoadViewState event, the initialized controls receive their first properties: viewstate information that was persisted back to the server on the last submission. The page viewstate is managed by ASP.NET and is used to persist information over a page roundtrip to the server. Viewstate information is saved as a string of name/value pairs and contains information such as control text or value. The viewstate is held in the value property of a hidden <input> control that is passed from page request to page request. As you can see, this is a giant leap forward from the old ASP 3.0 techniques of maintaining state. This event can be overridden using the LoadViewState method and is commonly used to customize the data received by the control at the time it is populated.
3. LoadPostData Processes Postback Data
During this phase of the page creation, form data that was posted to the server (termed postback data in ASP.NET) is processed against each control that requires it. When a page submits a form, the framework will implement the IPostBackDataHandler interface on each control that submitted data. The page then fires the LoadPostData event and parses through the page to find each control that implements this interface and updates the control state with the correct postback data. ASP.NET updates the correct control by matching the control's unique ID with the name/value pair in the NameValueCollection. This is one reason that ASP.NET requires unique IDs for each control on any given page. Extra steps are taken by the framework to ensure each ID is unique in situations, such as several custom user controls existing on a single page. After the LoadPostData event triggers, the RaisePostDataChanged event is free to execute (see below).
Steps 4 Through 6
4. Object Load
Objects take true form during the Load event. All object are first arranged in the page DOM (called the Control Tree in ASP.NET) and can be referenced easily through code or relative position (crawling the DOM). Objects are then free to retrieve the client-side properties set in the HTML, such as width, value, or visibility. During Load, coded logic, such as arithmetic, setting control properties programmatically, and using the StringBuilder to assemble a string for output, is also executed. This stage is where the majority of work happens. The Load event can be overridden by calling OnLoad.
5. Raise PostBack Change Events
As stated earlier, this occurs after all controls that implement the IPostBackDataHandler interface have been updated with the correct postback data. During this operation, each control is flagged with a Boolean on whether its data was actually changed or remains the same since the previous submit. ASP.NET then sweeps through the page looking for flags indicating that any object's data has been updated and fires RaisePostDataChanged. The RaisePostDataChanged event does not fire until all controls are updated and after the Load event has occurred. This ensures data in another control is not manually altered during the RaisePostDataChanged event before it is updated with postback data.
6. Process Client-Side PostBack Event
After the server-side events fire on data that was changed due to postback updates, the object which caused the postback is handled at the RaisePostBackEvent event. The offending object is usually a control that posted the page back to the server due to a state change (with autopostback enabled) or a form submit button that was clicked. There is often code that will execute in this event, as this is an ideal location to handle event-driven logic. The RaisePostBackEvent event fires last in the series of postback events due to the accuracy of the data that is rendered to the browser. Controls that are changed during postback should not be updated after the executing function is called due to the consistency factor. That is, data that is changed by an anticipated event should always be reflected in the resulting page. The RaisePostBackEvent can be trapped by catching RaisePostBackEvent.
Steps 7 Through 10
7. Prerender the Objects
The point at which the objects are prerendered is the last time changes to the objects can be saved or persisted to viewstate. This makes the PreRender step a good place to make final modifications, such as changing properties of controls or changing Control Tree structure, without having to worry about ASP.NET making changes to objects based off of database calls or viewstate updates. After the PreRender phase those changes to objects are locked in and can no longer be saved to the page viewstate. The PreRender step can be overridden using OnPreRender.
8. ViewState Saved
The viewstate is saved after all changes to the page objects have occurred. Object state data is persisted in the hidden <input> object and this is also where object state data is prepared to be rendered to HTML. At the SaveViewState event, values can be saved to the ViewState object, but changes to page controls are not. You can override this step by usingSaveViewState.
9. Render To HTML
The Render event commences the building of the page by assembling the HTML for output to the browser. During the Render event, the page calls on the objects to render them into HTML. The page then collects the HTML for delivery. When the Render event is overridden, the developer can write custom HTML to the browser that nullifies all the HTML the page has created thus far. The Render method takes an HtmlTextWriter object as a parameter and uses that to output HTML to be streamed to the browser. Changes can still be made at this point, but they are reflected to the client only.
10. Disposal
After the page's HTML is rendered, the objects are disposed of. During the Dispose event, you should destroy any objects or references you have created in building the page. At this point, all processing has occurred and it is safe to dispose of any remaining objects, including the Page object. You can override Dispose, as well as Render by setting the appropriate selection in the object parameter.
Guidelines to Creating a Good ASP.NET Application
The following are the some of the guidelines to create a good ASP.NET application.
- Disable session when not using it. This can be done at the application level in the "machine.config" file or at a page level.
- The in-proc model of session management is the fastest of the three options. SQL Server option has the highest performance hit.
- Minimize the amount and complexity of data stored in a session state. The larger and more complex the data is, the cost of serializing/deserializing of the data is higher (for SQL Server and State server options).
- Use Server.Transfer for redirecting between pages in the same application. This will avoid unnecessary client-side redirection.
- Choose the best suited session-state provider - In-process is the fastest option.
- Avoid unnecessary round-trips to the server - Code like validating user input can be handled at the client side itself.
- Use Page.IsPostback to avoid unnecessary processing on a round trip.
- Use server controls in appropriate circumstances. Even though are they are very easy to implement, they are expensive because they are server resources. Sometimes, it is easier to use simple rendering or data-binding.
- Save server control view state only when necessary.
- Buffering is on by default. Turning it off will slow down the performance. Don't code for string buffering - Response.Write will automatically buffer any responses without the need for the user to do it. Use multiple Response.Writes rather than create strings via concatenation, especially if concatenating long strings.
- Don't rely on exceptions in the code. Exceptions reduce performance. Do not catch the exception itself before handling the condition.
// Consider changing this...
try { result = 100 / num;}
catch (Exception e) { result = 0;}// to this...
if (num != 0)
result = 100 / num;
else
result = 0;
- Use early binding in VB.NET and Jscript code. Enable Option Strict in the page directive to ensure that the type-safe programming is maintained.
- Port call-intensive COM components to managed code. While doing Interop try avoiding lot of calls. The cost of marshalling the data ranges from relatively cheap (i.e. int, bool) to more expensive (i.e. strings). Strings, a common type of data exchanged for web applications, can be expensive because all strings in the CLR are in Unicode, but COM or native methods may require other types of encoding (i.e. ASCII).
- Release the COM objects or native resources as soon as the usage is over. This will allow other requests to utilize them, as well as reducing performance issues, such as having the GC release them at a later point.
- Use SQL server stored procedures for data access.
- Use the SQLDataReader class for a fast forward-only data cursor.
- Datagrid is a quick way of displaying data, but it slows down the application. The other alternative, which is faster, is rendering the data for simple cases. But this difficult to maintain. A middle of the road solution could be a repeater control, which is light, efficient, customizable and programmable.
- Cache data and page output whenever possible.
- Disable debug mode before deploying the application.
- For applications that rely extensively one external resource, consider enabling web gardening on multiprocessor computers. The ASP.NET process model helps enable scalability by distributing work to several processes, one on each CPU. If the application is using a slow database server or calls COM objects that access external resources, web gardening could be a solution.
- Enumerating into collections sometimes is more expensive than index access in a loop. This is because the CLR can sometimes optimize array indexes and bounds checks away in loops, but can't detect them in for each type of code.
- JScript .NET allows methods within methods - to implement these in the CLR required a more expensive mechanism which can be much slower, so avoid them by moving inner methods to be just regular methods of the page.
- Do a "pre-batch" compilation. To achieve this, request a page from the site.
The Config File
- Avoid making changes to pages or assemblies that are there in the bin directory of the application. A changed page will only recompile the page. Any change to the bin directory will result in recompile of the entire application.
- The config file is configured to enable the widest set of features. For a performance boost it is better to tune it to the requirements. Some key points here are:
- Encoding - Request/Response - The default is UTF-8 encoding. If the site is completely ASCII, change the option to ASCII encoder.
- Session State - By default is ON. If session state is not maintained then the value should be changed to OFF.
- ViewState - Default is ON. Turn it off if not being used. If ViewState is being used there are different levels of security that need to considered which can impact the performance of the application.
- AutoEventWireup - Turning off AutoEventWireup means that the page will not try and match up method names to events and hook them up (i.e. Page_Load, etc). Instead, if the application writer wishes to receive them, they need to override the methods in the base class (i.e. override OnLoad for the page load event instead of using a Page_Load method). By doing so, the page will get a slight performance boost by not having to do the extra work itself, but leaving it to the page author.
- Encoding - Request/Response - The default is UTF-8 encoding. If the site is completely ASCII, change the option to ASCII encoder.
- For efficient debugging Use ASP.NET trace feature to debug instead of Response.Write.
Conclusion
Performance is the main objective for most of the ASP.NET applications. Each time we request an ASP.NET page, we run through the same process from initialization to disposal. By understanding the inner workings of the ASP.NET page process and following the above mentioned tips, writing and debugging our code will be much easier and effective (not to mention less frustrating).
Read more at http://www.aspfree.com/c/a/ASP.NET/ASP.NET-Life-Cycle-and-Best-Practices/#yQHMUZcAXVLVb5L8.99