public class AntivirusActivity extends Activity { TextView tv_init_virus; ProgressBar pb; Message msg; ImageView iv_scanning; LinearLayout ll_content; protected void onCreate(Bundle savedInstanceState) { // TODO Auto-generated method stub super.onCreate(savedInstanceState); initUI(); initData(); } static class ScanInfo{ boolean desc; String appname; String packagename; } Handler handler = new Handler(){ public void handleMessage(android.os.Message msg) { switch(msg.what){ case 1: tv_init_virus.setText("初始化八核杀毒引擎"); break; case 2: //病毒扫描中 TextView child = new TextView(AntivirusActivity.this); ScanInfo scaninfo = (ScanInfo) msg.obj; if(scaninfo.desc){//true表示有病毒 child.setTextColor(Color.RED); child.setText(scaninfo.appname+"有病毒"); }else{ child.setTextColor(Color.BLACK); child.setText(scaninfo.appname+"扫描安全"); } ll_content.addView(child); break; case 3: //当扫描结束的时候,停止动画。 iv_scanning.clearAnimation(); break; } }; }; public void initData(){ new Thread(){ public void run(){ // //获取手机里面所有的应用程序 // List<AppInfo> appinfos = AppInfos.getAppInfos(AntivirusActivity.this); // //迭代手机上边所有的软件 // for(AppInfo appinfo:appinfos){ // String name = appinfo.getApkname(); // } msg = Message.obtain(); msg.what = 1;//初始化病毒 PackageManager packageManager = getPackageManager(); List<PackageInfo> installPackages = packageManager.getInstalledPackages(0); int size = installPackages.size(); pb.setMax(size); int progress = 0 ; for(PackageInfo packageinfo:installPackages){ //获取当前手机上app的名字 String appname = packageinfo.applicationInfo.loadLabel(packageManager).toString(); //首先需要获取到每个应用程序的位置 String sourceDir = packageinfo.applicationInfo.sourceDir; ScanInfo scaninfo = new ScanInfo(); scaninfo.appname = appname; scaninfo.packagename = packageinfo.applicationInfo.packageName; //MD5是病毒特征码,得到应用程序的MD5值 String md5 = null; try { md5 = MD5Utils.getFileMd5(sourceDir); } catch (Exception e) { // TODO Auto-generated catch block e.printStackTrace(); } String desc = AntivirusDao.checkFileVirus(md5); if(desc == null){ scaninfo.desc = false; }else{ //这里有病毒 scaninfo.desc = true; } progress++; pb.setProgress(progress); msg = Message.obtain(); msg.what = 2;//正在浏览 msg.obj = scaninfo; handler.sendMessage(msg); //判断当前的文件是否在病毒数据库中? } msg = Message.obtain(); msg.what = 3;//结束 handler.sendMessage(msg); } }.start(); } public void initUI(){ setContentView(R.layout.activity_antivirus); iv_scanning = (ImageView) findViewById(R.id.iv_scanning); ll_content = (LinearLayout) findViewById(R.id.ll_content); tv_init_virus = (TextView) findViewById(R.id.tv_init_virus); pb = (ProgressBar) findViewById(R.id.progressBar1); /** * fromDegrees 開始的角度 * toDegrees 結束的角度 * pivotXType 參照自己 * pivotXValue * pivotYType * pivotYValue * 初始化旋转动画 */ RotateAnimation rotationmation = new RotateAnimation(0,360,Animation.RELATIVE_TO_SELF,0.5f, Animation.RELATIVE_TO_SELF,0.5f); rotationmation.setDuration(5000); //rotationmation.setRepeatMode(-1); rotationmation.setRepeatCount(-1); iv_scanning.startAnimation(rotationmation); } }
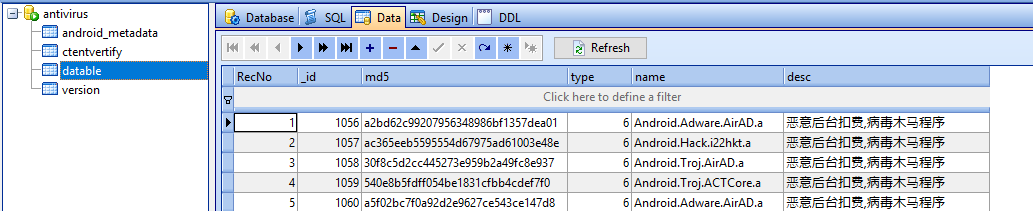
这个是病毒数据库,在闪屏页将assets目录下的数据库拷贝到data/data/ .../files 目录下
我们需要用的是antivirus.db里面的datable表
AntivirusDao.java
public class AntivirusDao { //检查当前MD5是否在数据库中 public static String checkFileVirus(String md5){ String desc = null; SQLiteDatabase db = SQLiteDatabase.openDatabase("data/data/com.mxn.mobilesafe/files/antivirus.db", null,
SQLiteDatabase.OPEN_READONLY); //查询 Cursor cursor = db.rawQuery("select desc from datable where md5 = ?", new String[]{md5}); if(cursor.moveToNext()){ cursor.getString(0); } return desc; } //添加病毒数据库 public static void adddVirus(String md5,String desc){ SQLiteDatabase db = SQLiteDatabase.openDatabase("data/data/com.mxn.mobilesafe/files/antivirus.db", null, SQLiteDatabase.OPEN_READONLY); ContentValues values = new ContentValues(); values.put("md5", md5); values.put("desc", desc); values.put("type", 6); values.put("name", "Android.Adware.AirAD.a"); db.insert("datable", null, values); db.close(); } }
activity_antivirus.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="vertical" xmlns:android="http://schemas.android.com/apk/res/android"> <TextView style="@style/TitleStyle" android:text="病毒查杀" /> <LinearLayout android:layout_width="match_parent" android:layout_height="match_parent" android:layout_margin="10dp" android:orientation="horizontal" > <FrameLayout android:layout_width="wrap_content" android:layout_height="wrap_content" > <ImageView android:layout_width="wrap_content" android:layout_height="wrap_content" android:background="@drawable/ic_scanner_malware" /> <ImageView android:id="@+id/iv_scanning" android:layout_width="wrap_content" android:layout_height="wrap_content" android:background="@drawable/act_scanning_03" /> </FrameLayout> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="vertical" android:layout_margin="5dp" > <TextView android:id="@+id/tv_init_virus" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="初始化8核杀毒引擎" /> <ProgressBar style="?android:attr/progressBarStyleHorizontal" android:layout_width="match_parent" android:layout_height="wrap_content" android:id="@+id/progressBar1" ></ProgressBar> </LinearLayout> </LinearLayout> <LinearLayout android:orientation="vertical" android:id="@+id/ll_content" android:layout_width="match_parent" android:layout_height="match_parent" ></LinearLayout> </LinearLayout>