发送纯文本邮件
一、在pom.xml中添加邮件服务的依赖启动器
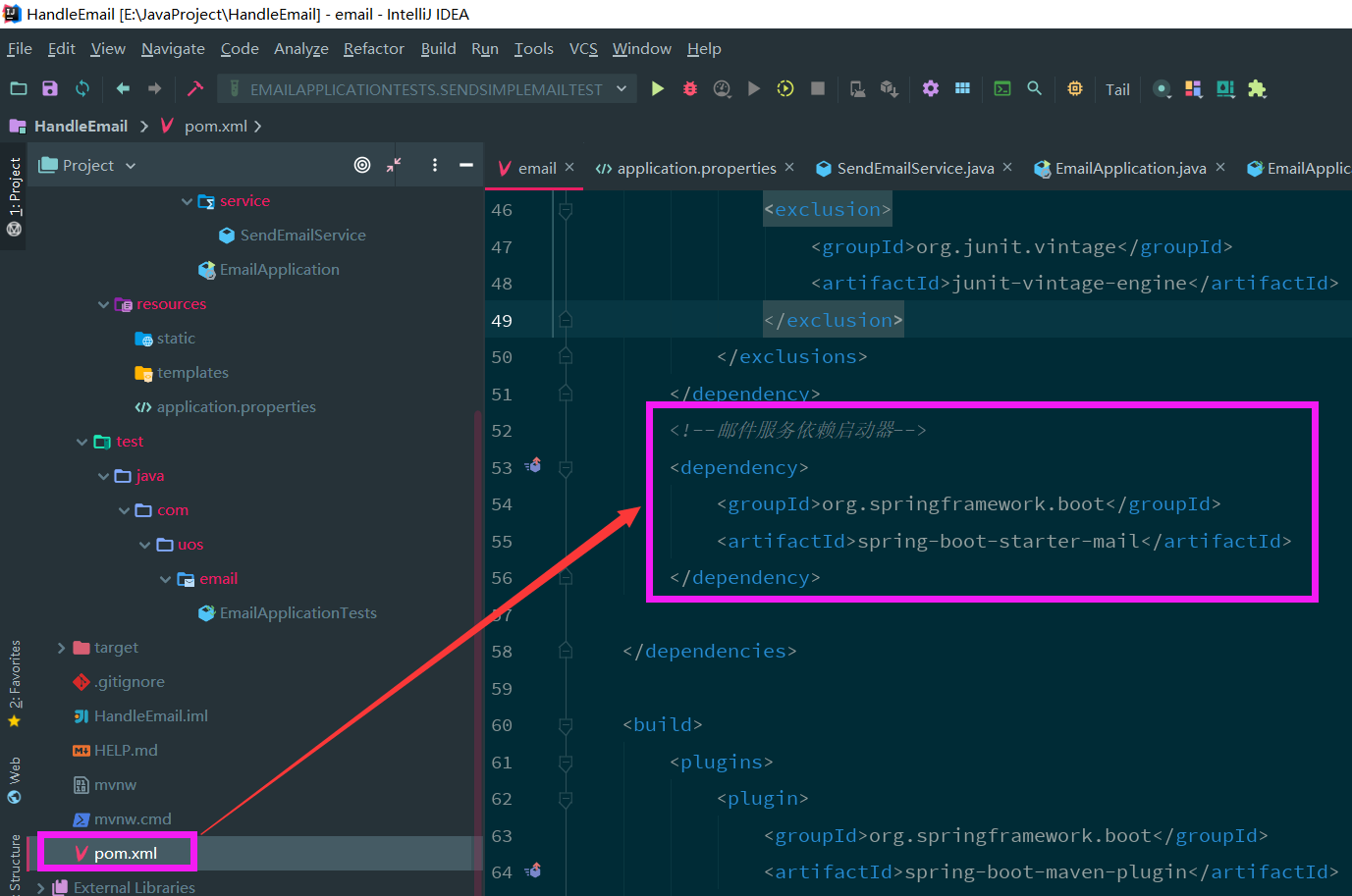
<!--邮件服务依赖启动器-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-mail</artifactId>
</dependency>
二、在全局配置文件中添加邮件服务配置
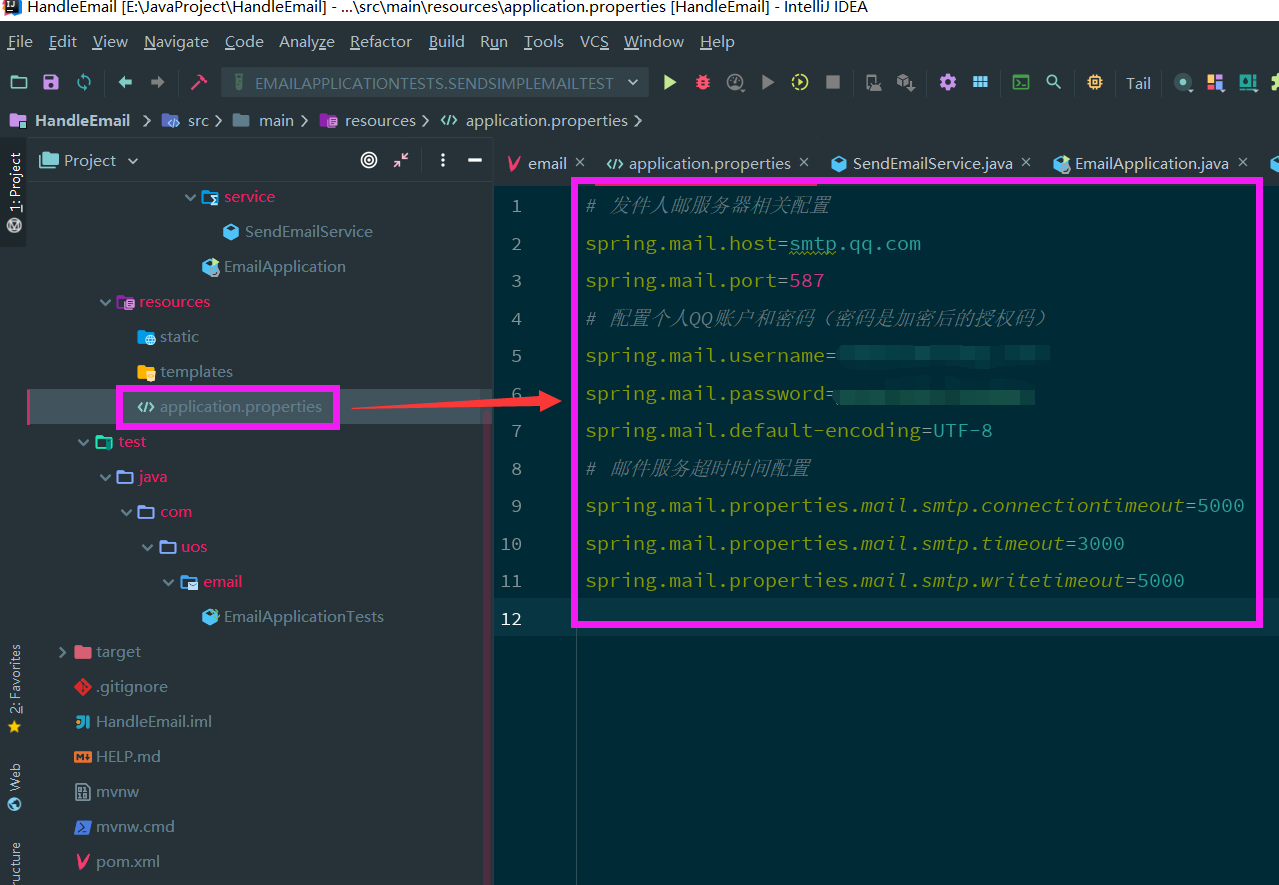
三、定制邮件发送任务
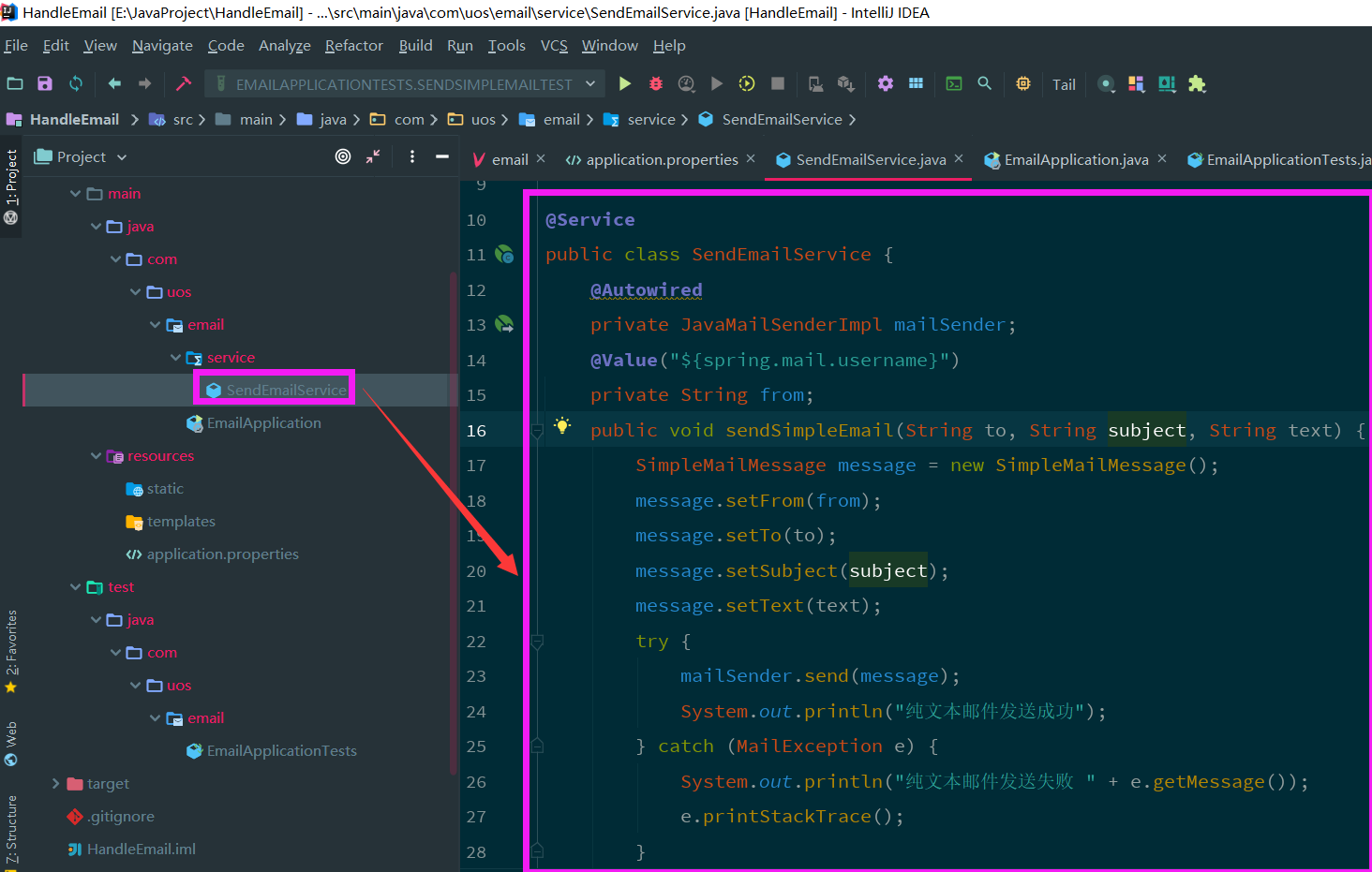
package com.uos.email.service;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.mail.MailException;
import org.springframework.mail.SimpleMailMessage;
import org.springframework.mail.javamail.JavaMailSenderImpl;
import org.springframework.stereotype.Service;
@Service
public class SendEmailService {
@Autowired
private JavaMailSenderImpl mailSender;
@Value("${spring.mail.username}")
private String from;
public void sendSimpleEmail(String to, String subject, String text) {
SimpleMailMessage message = new SimpleMailMessage();
message.setFrom(from);
message.setTo(to);
message.setSubject(subject);
message.setText(text);
try {
mailSender.send(message);
System.out.println("纯文本邮件发送成功");
} catch (MailException e) {
System.out.println("纯文本邮件发送失败 " + e.getMessage());
e.printStackTrace();
}
}
}
四、测试类
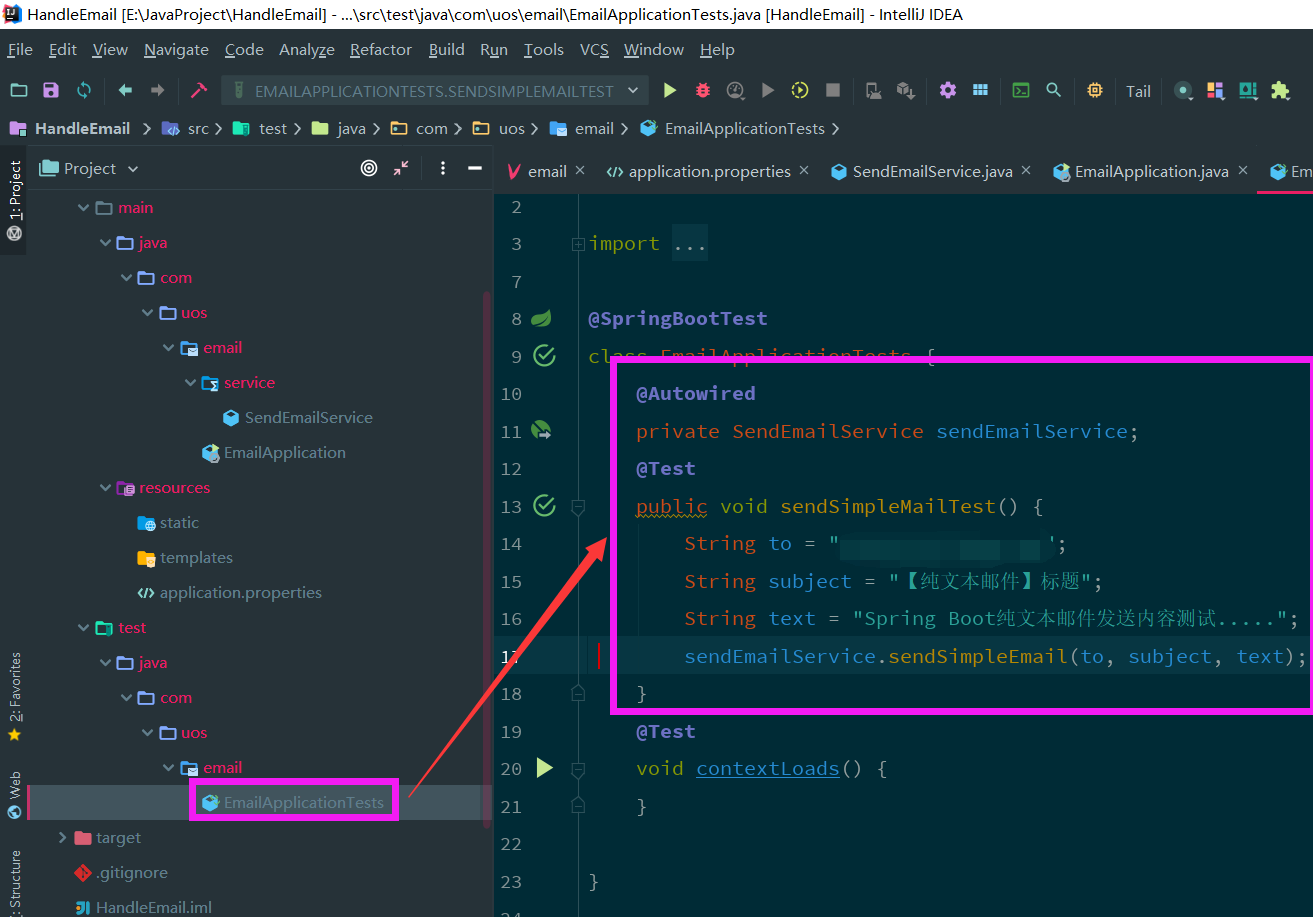
五、运行结果
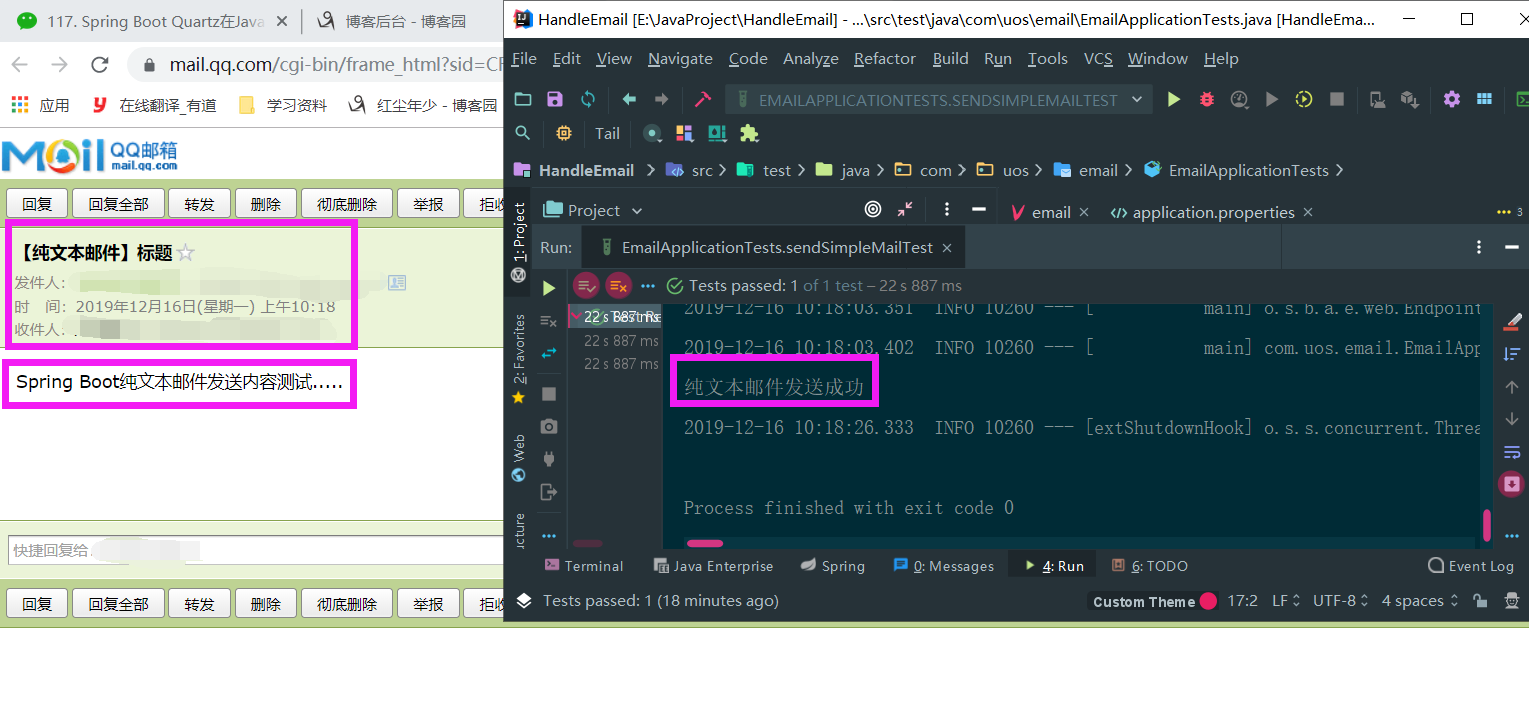
发送带附件和图片的邮件
一、在SendEmailService中添加
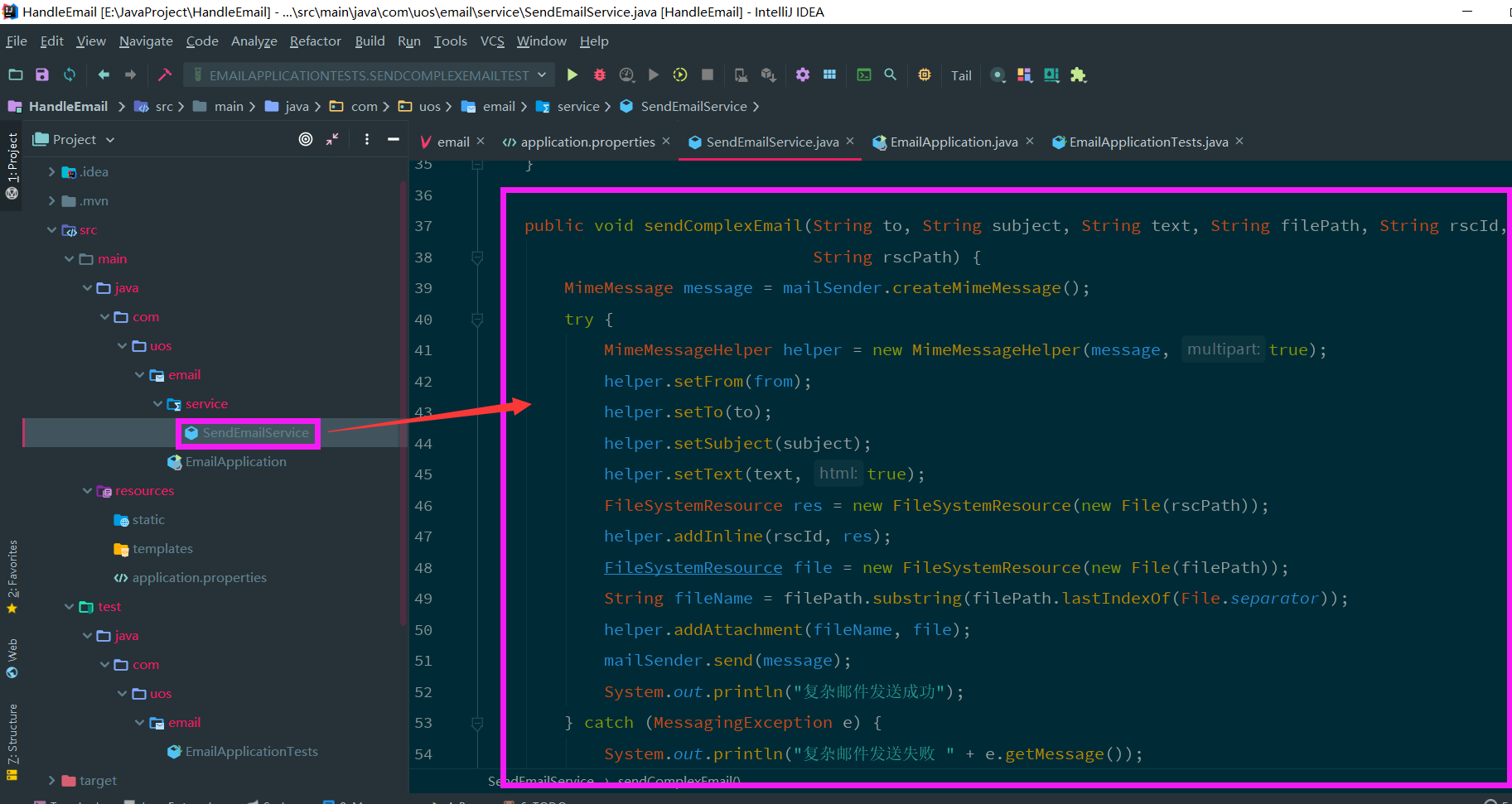
public void sendComplexEmail(String to, String subject, String text, String filePath, String rscId,
String rscPath) {
MimeMessage message = mailSender.createMimeMessage();
try {
MimeMessageHelper helper = new MimeMessageHelper(message, true);
helper.setFrom(from);
helper.setTo(to);
helper.setSubject(subject);
helper.setText(text, true);
FileSystemResource res = new FileSystemResource(new File(rscPath));
helper.addInline(rscId, res);
FileSystemResource file = new FileSystemResource(new File(filePath));
String fileName = filePath.substring(filePath.lastIndexOf(File.separator));
helper.addAttachment(fileName, file);
mailSender.send(message);
System.out.println("复杂邮件发送成功");
} catch (MessagingException e) {
System.out.println("复杂邮件发送失败 " + e.getMessage());
e.printStackTrace();
}
}
二、在测试类中添加
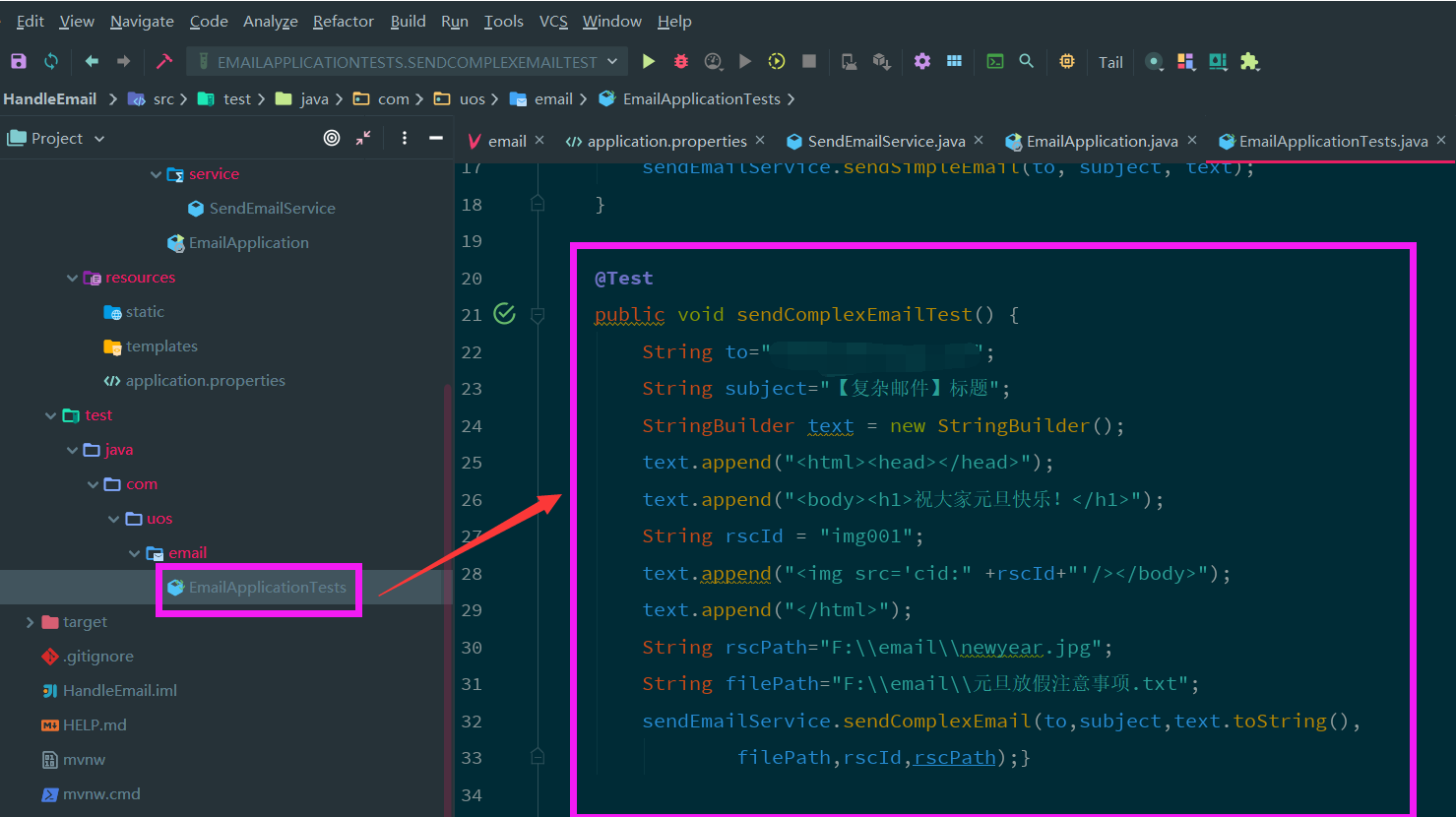
三、测试结果
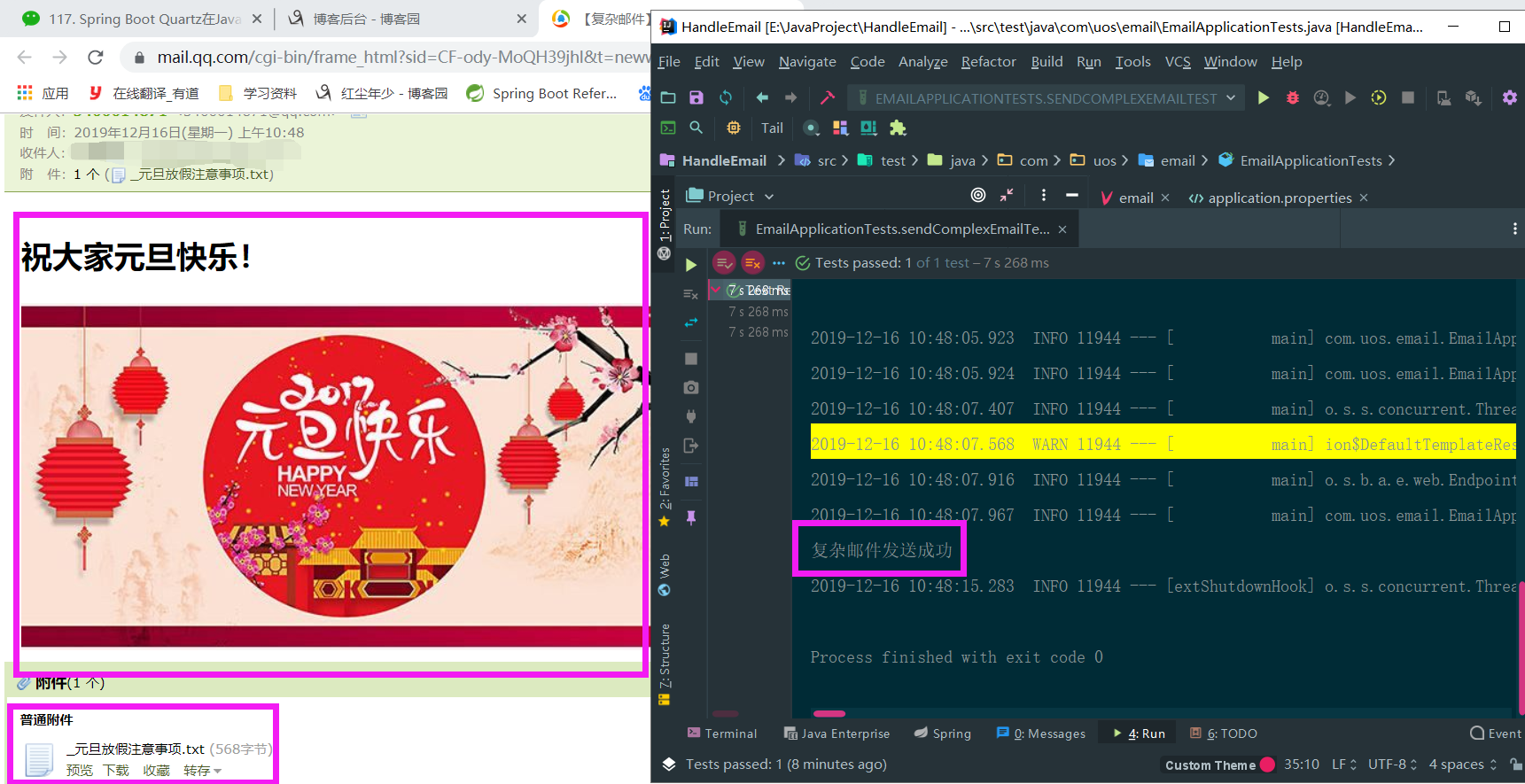
发送模板邮件
一、在pom.xml中添加Thymeleaf模板引擎依赖启动器
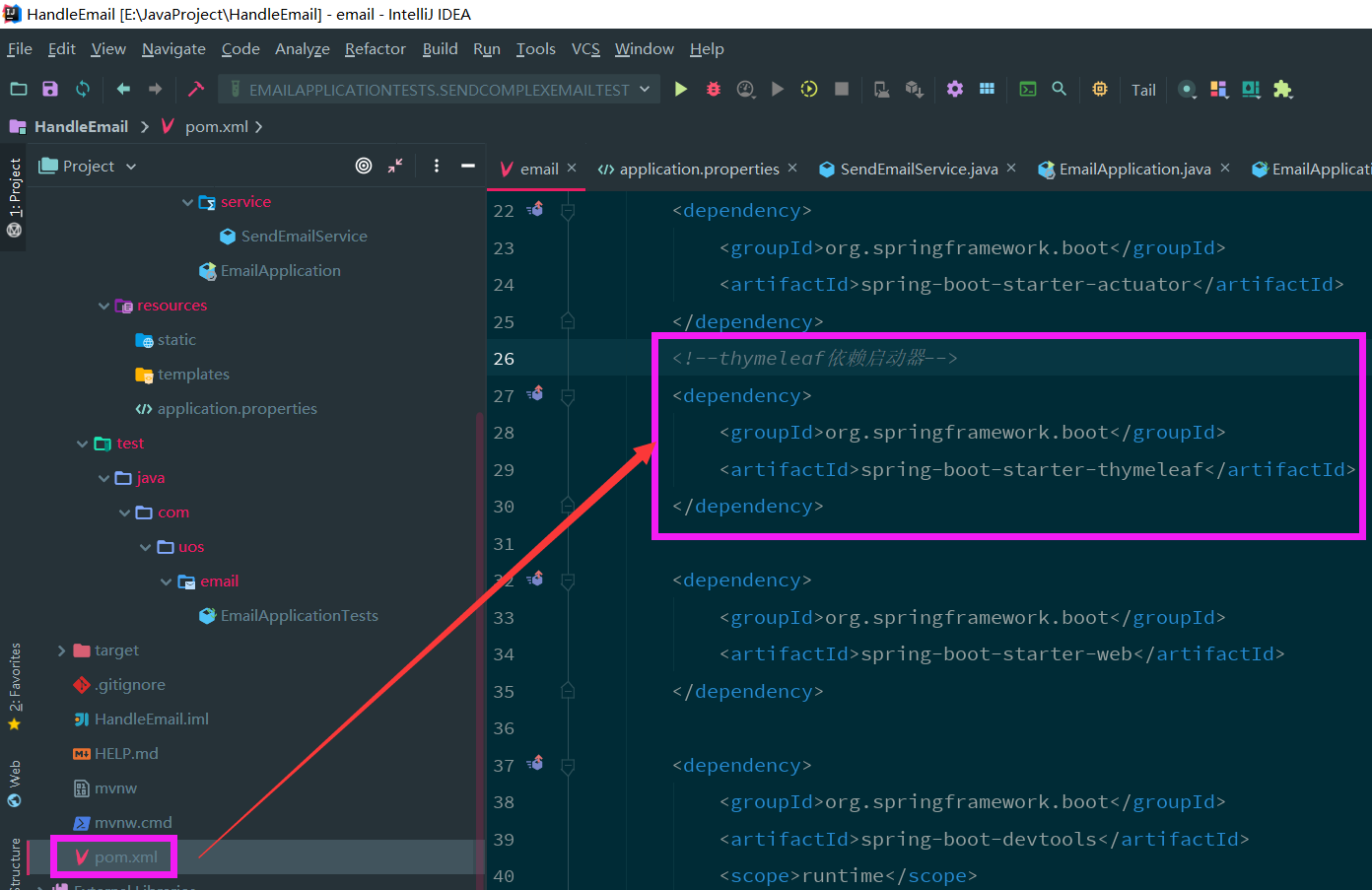
二、定制模板邮件
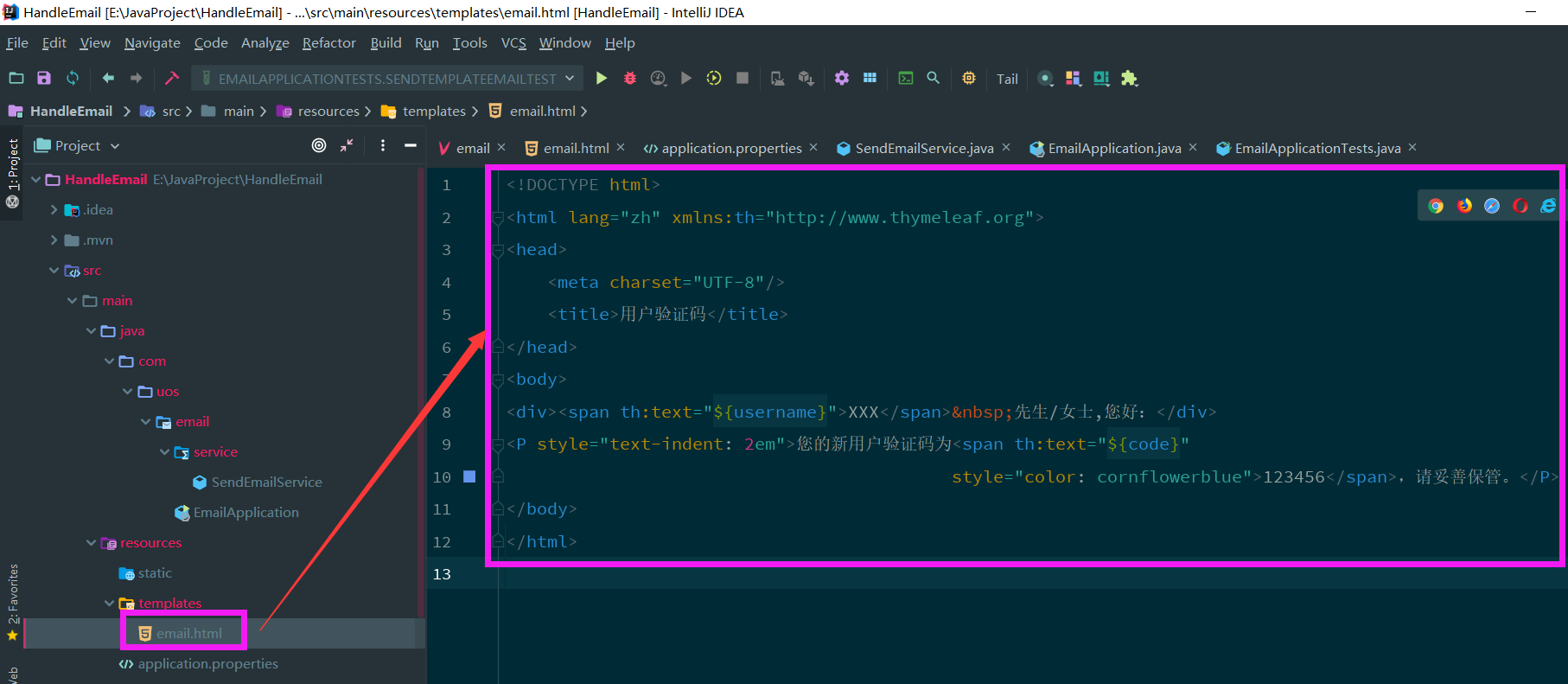
<!DOCTYPE html>
<html lang="zh" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8"/>
<title>用户验证码</title>
</head>
<body>
<div><span th:text="${username}">XXX</span> 先生/女士,您好:</div>
<P style="text-indent: 2em">您的新用户验证码为<span th:text="${code}"
style="color: cornflowerblue">123456</span>,请妥善保管。</P>
</body>
</html>
三、在SendEmailService中添加
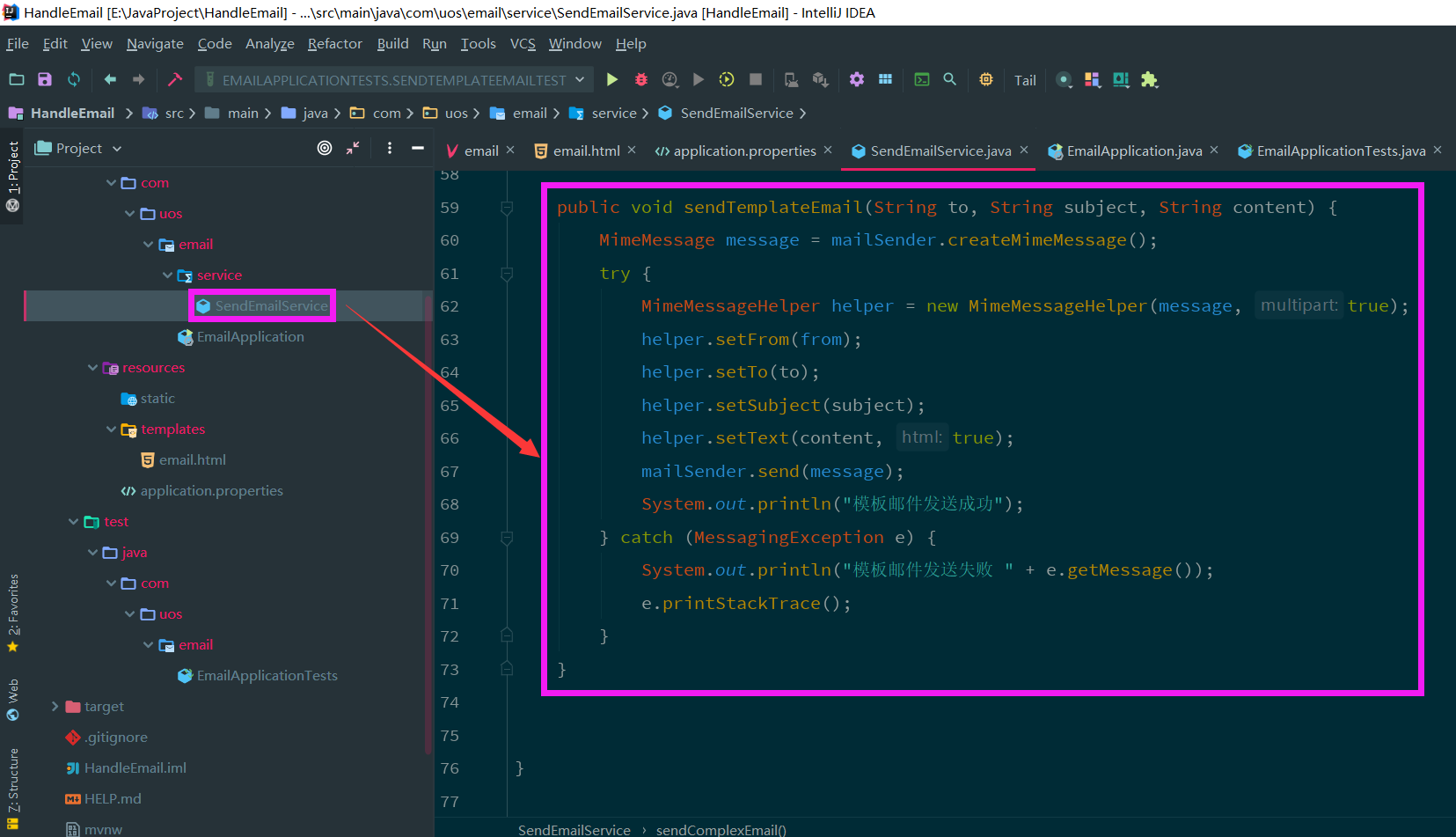
四、在测试类中添加
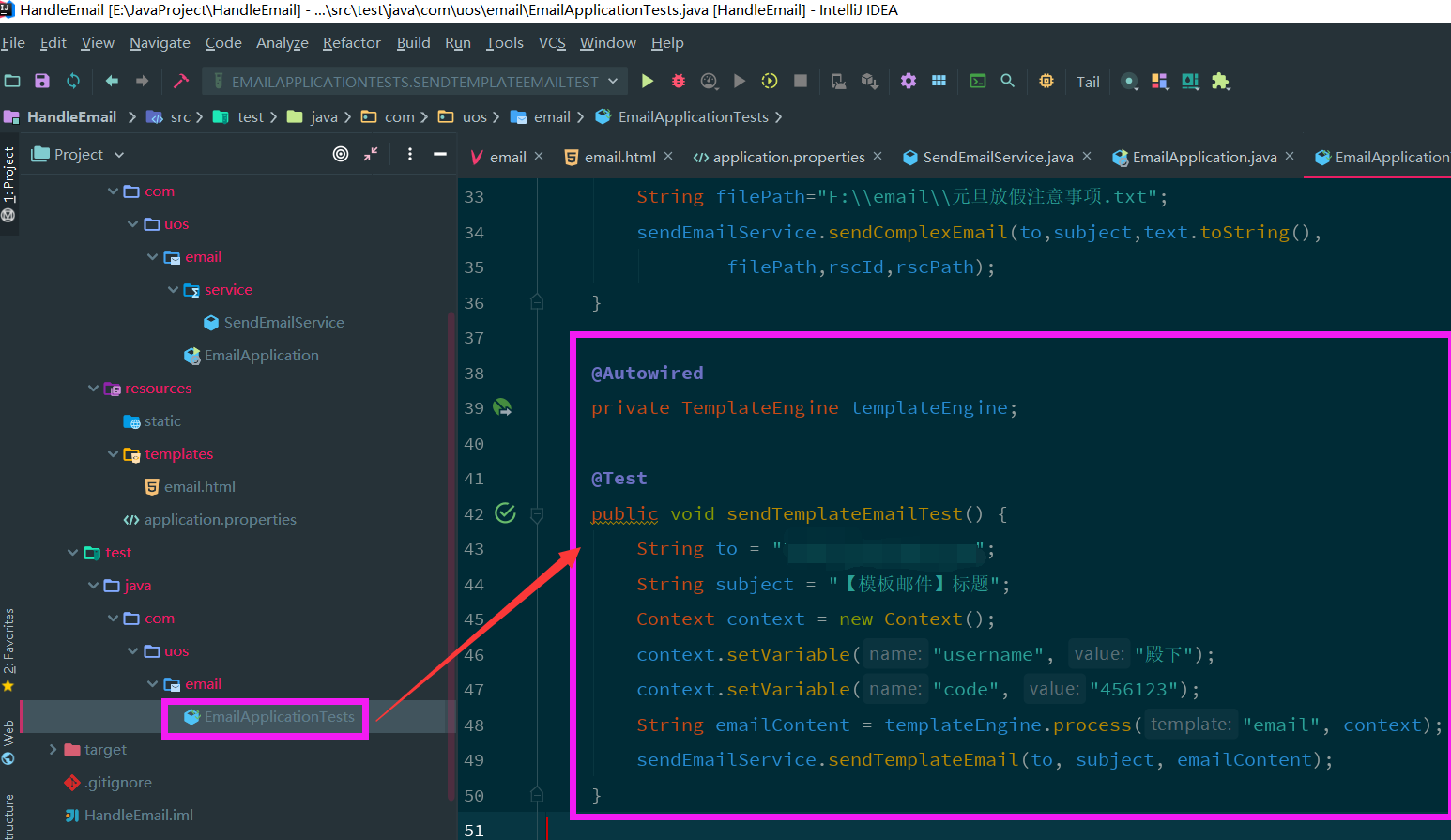
五、测试结果
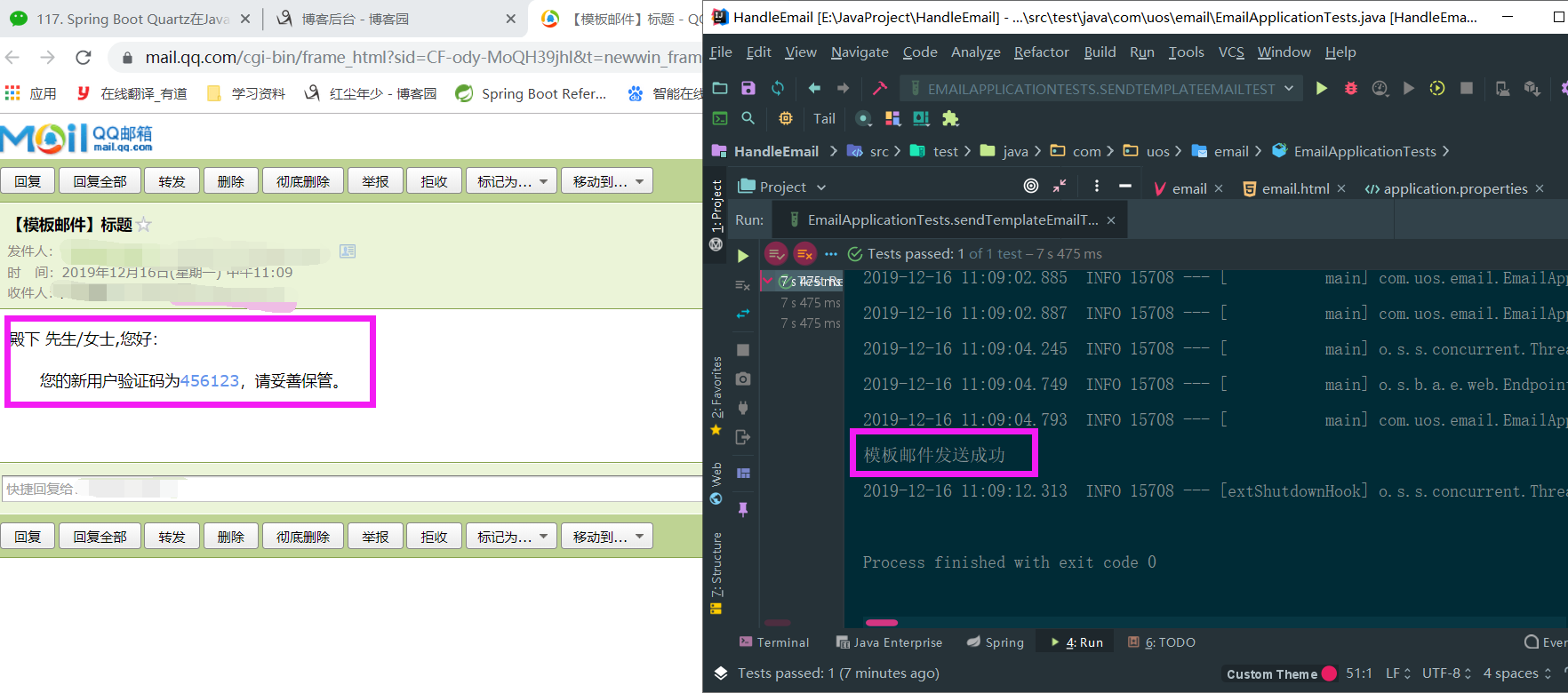