网页和Ajax和跨域的关系
一、JSON 介绍
1. JSON: JavaScript Object Notation
Because JSON syntax is derived from JavaScript object notation, very little extra software is needed to work with JSON within JavaScript.
A number (integer or floating point)
A string (in double quotes)
A Boolean (true or false)
An array (in square brackets)
An object (in curly braces)
null
2. Javascript中的JSON对象 MDN
JSON
object contains methods for parsing JavaScript Object Notation (JSON) and converting values to JSON. It can't be called or constructed, and aside from its two method properties it has no interesting functionality of its own.JSON.parse() 把JSON object 转化成 javascript中的 数值类型
JSON.stringify() 恰好相反
3. Josn解析
二、AJAX
Ajax (short for asynchronous JavaScript and XML(XML只是之前名字的来历,现在更多的是json格式的数据交换,当然也有其它数据格式)) is a set of(多种技术的合集) web development techniques using many web technologies on the client-side to create asynchronous Web applications. With Ajax, web applications can send data to and retrieve from a server asynchronously (in the background) without interfering with the display and behavior of the existing page. By decoupling the data interchange layer from the presentation layer, Ajax allows for web pages, and by extension web applications, to change content dynamically without the need to reload the entire page(允许网页或是web应用来动态地、异步地的交换数据). In practice, modern implementations commonly substitute JSON for XML(现在更多使用json代替xml) due to the advantages of being native to JavaScript.
Ajax is not a technology, but a group of technologies. HTML and CSS can be used in combination to mark up and style information. The DOM is accessed with JavaScript to dynamically display – and allow the user to interact with – the information presented. JavaScript and the XMLHttpRequest object provide a method for exchanging data asynchronously between browser and server to avoid full page reloads.
AJAX is a misleading name. You don't have to understand XML to use AJAX.
1 The XMLHttpRequest Object (MDN)
eg:
var xhttp;
if (window.XMLHttpRequest) {
xhttp = new XMLHttpRequest();
} else {
// code for IE6, IE5
xhttp = new ActiveXObject("Microsoft.XMLHTTP");
}
2 http请求
GET
is used to retrieve remote data, and POST
is used to insert/update remote data.1. status
- 1xx:指示信息--表示请求已接收,继续处理。
- 2xx:成功--表示请求已被成功接收、理解、接受。
- 3xx:重定向--要完成请求必须进行更进一步的操作。
- 4xx:客户端错误--请求有语法错误或请求无法实现。
- 5xx:服务器端错误--服务器未能实现合法的请求。
100——客户必须继续发出请求
101——客户要求服务器根据请求转换HTTP协议版本
200——成功
201——提示知道新文件的URL
300——请求的资源可在多处得到
301——删除请求数据
404——没有发现文件、查询或URl
500——服务器产生内部错误
2. readyState
0: 请求未初始化,open()方法还没有调用
1: 服务器连接已建立
2: 请求已接收,接收到头信息了
3: 请求处理中,接收到响应主体了
4: 请求已完成,且响应已就绪,也就是相应已经完成了
3. onreadystatechange
An EventHandler
that is called whenever the readyState
attribute changes. The callback is called from the user interface thread.
request.open(method,url,asy)
requset.send(string)
如果是get请求,则参数直接拼接在url里面了
如果是send请求,则参数需要写在send()方法里面
post请求
function post(){
var req = createXMLHTTPRequest();
if(req){
req.open("POST", "http://test.com/", true);
req.setRequestHeader("Content-Type","application/x-www-form-urlencoded; charset=gbk;");
req.send("keywords=手机");
req.onreadystatechange = function(){
if(req.readyState == 4){
if(req.status == 200){
alert("success");
}else{
alert("error");
}
}
}
}
}
get请求
function get(){
var req = createXMLHTTPRequest();
if(req){
req.open("GET", "http://test.com/?keywords=手机", true);
req.onreadystatechange = function(){
if(req.readyState == 4){
if(req.status == 200){
alert("success");
}else{
alert("error");
}
}
}
req.send(null);
}
}
三、同源策略
同源策略
同源策略限制了一个源(origin)中加载文本或脚本与来自其它源(origin)中资源的交互方式。
同源政策的目的,是为了保证用户信息的安全,防止恶意的网站窃取数据。
如果非同源,共有三种行为受到限制。
- (1) Cookie、LocalStorage 和 IndexDB 无法读取。
- (2) DOM 无法获得。
- (3) AJAX 请求不能发送。
Cookie
Cookie总是保存在客户端中,按在客户端中的存储位置,可分为内存Cookie和硬盘Cookie。
四、跨域
跨域的含义
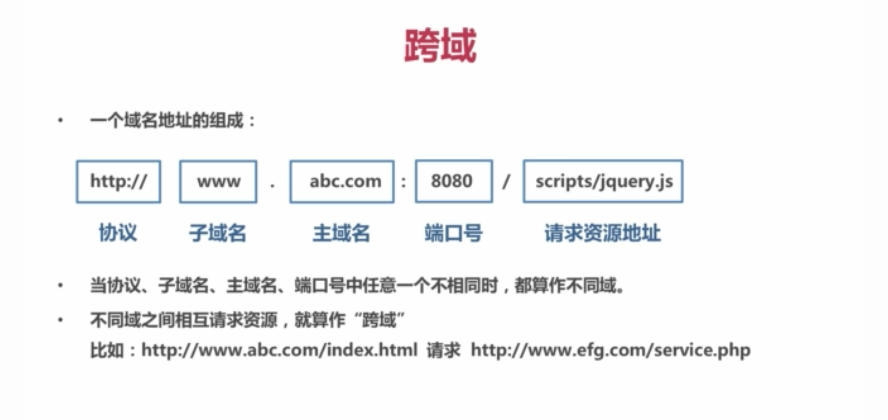
同源政策规定,AJAX请求只能发给同源的网址,否则就报错。
除了架设服务器代理(浏览器请求同源服务器,再由后者请求外部服务),有三种方法规避这个限制。
- JSONP
- WebSocket
- CORS
解决跨域问题的几种方法:
1 JSONP
说说JSON和JSONP,也许你会豁然开朗,含jQuery用例
<script>
元素,向服务器请求JSON数据,这种做法不受同源政策限制;服务器收到请求后,将数据放在一个指定名字的回调函数里传回来。2 WebSocket
3 CORS
The HTTP headers that relate to CORS are:
Request headers
- Origin
- Access-Control-Request-Method
- Access-Control-Request-Headers
Response headers
- Access-Control-Allow-Origin
- Access-Control-Allow-Credentials
- Access-Control-Expose-Headers
- Access-Control-Max-Age
- Access-Control-Allow-Methods
- Access-Control-Allow-Headers
CORS vs JSONP
CORS can be used as a modern alternative(现代浏览器替换jsonp的模式) to the JSONP pattern.
While JSONP supports only the GET request method, CORS also supports other types(支持其它请求) of HTTP requests.
Using CORS enables a web programmer to use regular XMLHttpRequest, which supports better error handling than JSONP.
On the other hand, JSONP works on legacy browsers(老式浏览器) which predate CORS support. CORS is supported by most modern web browsers. Also, while JSONP can cause cross-site scripting (XSS) issues where the external site is compromised, CORS allows websites to manually parse responses to ensure security.
CORS
CORS需要浏览器和服务器同时支持。目前,所有浏览器都支持该功能,IE浏览器不能低于IE10。
整个CORS通信过程,都是浏览器自动完成,不需要用户参与。对于开发者来说,CORS通信与同源的AJAX通信没有差别,代码完全一样。浏览器一旦发现AJAX请求跨源,就会自动添加一些附加的头信息,有时还会多出一次附加的请求,但用户不会有感觉。
因此,实现CORS通信的关键是服务器。只要服务器实现了CORS接口,就可以跨源通信。