With the release of Spring 4.2 version, Three new classes have been introduced to handle Requests Asynchronously of the Servlet Thread. Which are;
ResponseBodyEmitter enables to send DeferredResult with a compatible HttpMessageConverter. Results can be emitted from threads which are not necessarily the Servlet Request Thread of the Servlet Container.
SseEmitter is used to send Server Sent Events to the Client. Server Sent Events has a fixed format and the response type for the result will be text/event-stream.
StreamingResponseBody is used to send raw unformatted data such as bytes to the client asynchronously of the Servlet Thread.
ResponseBodyEmitter and SseEmitter has a method named complete to mark its completion and StreamingResponseBody will complete when there is no more data to send.
All three options will be keeping alive a connection to the endpoint until the end of the request.
StreamingResponseBody is particularly useful for streaming large files such as Media Files as writing of the bytes to the Response's OutputStream will be done asynchronously. StreamingResponseBody has a writeTo(OutputStream os) call back method which needs to be overridden inorder to support streaming.
I wrote a small Spring Boot Application to showcase the StreamingResponseBody capabilities in terms of Streaming large files. The application source code can be found at www.github.com/shazin/itube. Below is a screen shot of the application.
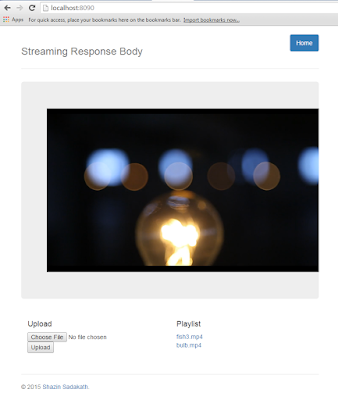
In order to send the Video files streaming to the Projekktor player in the web page following code snippet is used.
@RequestMapping(method = RequestMethod.GET, value = "/{video:.+}") public StreamingResponseBody stream(@PathVariable String video) throws FileNotFoundException { File videoFile = videos.get(video); final InputStream videoFileStream = new FileInputStream(videoFile); return (os) -> { readAndWrite(videoFileStream, os); }; }
And a Custom Web Configuration to over ride default timeout behavior to no timeout and finally configuring an AsyncTaskExecutor
@Configuration public static class WebConfig extends WebMvcConfigurerAdapter { @Override public void configureAsyncSupport(AsyncSupportConfigurer configurer) { configurer.setDefaultTimeout(-1); configurer.setTaskExecutor(asyncTaskExecutor()); } @Bean public AsyncTaskExecutor asyncTaskExecutor() { return new SimpleAsyncTaskExecutor("async"); } }
转载自:http://shazsterblog.blogspot.com/2016/02/asynchronous-streaming-request.html
笔者代码:
@RequestMapping(value = "{uuid}.m3u8") public ResponseEntity<StreamingResponseBody> m3u8Generator(@PathVariable("uuid") String uuid){ String key = "media.".concat(uuid); Map<String, Object> cached = cacheService.getCacheMap(key); if(CollectionUtils.isEmpty(cached)) { return new ResponseEntity(null, HttpStatus.OK); } String playlist = (String) cached.get("playlist"); String[] lines = playlist.split(" "); //人为在每个MPEG-2 transport stream文件前面加上一个地址前缀 StringBuffer buffer = new StringBuffer(); StreamingResponseBody responseBody = new StreamingResponseBody() { @Override public void writeTo (OutputStream out) throws IOException { for(int i = 0; i < lines.length; i++) { String line = lines[i]; if(line.endsWith(".ts")) { buffer.append("/streaming/"); buffer.append(uuid); buffer.append("/"); buffer.append(line); }else { buffer.append(line); } buffer.append(" "); } out.write(buffer.toString().getBytes()); out.flush(); } }; return new ResponseEntity(responseBody, HttpStatus.OK); }
其他类似功能代码:
//https://www.baeldung.com/spring-mvc-sse-streams //https://www.logicbig.com/tutorials/spring-framework/spring-web-mvc/streaming-response-body.html //@GetMapping("/{fileName:[0-9A-z]+}") @GetMapping("/streaming2/{uuid}/{fileName}") //https://stackoverflow.com/questions/47868352/how-to-stream-large-http-response-in-spring @ResponseBody public ResponseEntity<InputStreamResource> get_File2( @PathVariable String uuid, @PathVariable String fileName) throws IOException { String dbFile = fileRepository.findByUUID(uuid,fileName); dbFile=String.format("E:/hls/test/%s",fileName); if (dbFile.equals(null)) { return new ResponseEntity<>(HttpStatus.NOT_FOUND); } Resource file = storageService.loadAsResource(dbFile); long len = 0; try { len = file.contentLength(); } catch (IOException e) { e.printStackTrace(); } MediaType mediaType = MediaType.valueOf(FileTypeMap.getDefaultFileTypeMap().getContentType(file.getFile())); // if (filename.toLowerCase().endsWith("mp4") || filename.toLowerCase().endsWith("mp3") || // filename.toLowerCase().endsWith("3gp") || filename.toLowerCase().endsWith("mpeg") || // filename.toLowerCase().endsWith("mpeg4")) if(fileName.toLowerCase().endsWith("ts")) { mediaType = MediaType.APPLICATION_OCTET_STREAM; } InputStreamResource resource = new InputStreamResource(new FileInputStream(file.getFile())); return ResponseEntity.ok() .contentType(mediaType) .contentLength(len) .header(HttpHeaders.ACCEPT_RANGES, "bytes") .body(resource); }
@RequestMapping("/111111") public StreamingResponseBody handleRequest (HttpServletRequest request) { return outputStream -> { Map<String, BigInteger> map = new HashMap<>(); map.put("one", BigInteger.ONE); map.put("ten", BigInteger.TEN); try(ObjectOutputStream oos = new ObjectOutputStream(outputStream)){ oos.writeObject(map); } }; }
@GetMapping("/media/{uuid}/{fileName}") @ResponseBody public ResponseEntity<ByteArrayResource> getStream(@PathVariable String uuid, @PathVariable String fileName) throws IOException { String key = "media.".concat(uuid); String encoded = cacheService.getCachedHashValue(key, fileName, String.class); byte[] bytes = Base64.getDecoder().decode(encoded); long len = bytes.length; //InputStreamResource resource = new InputStreamResource(new FileInputStream(file.getFile())); ByteArrayResource resource = new ByteArrayResource(bytes); return ResponseEntity.ok().contentType(MediaType.APPLICATION_OCTET_STREAM).contentLength(len).header(HttpHeaders.ACCEPT_RANGES, "bytes").body(resource); // return ResponseEntity.ok() // .contentType(MediaType.APPLICATION_OCTET_STREAM) // .contentLength(len) // .header(HttpHeaders.ACCEPT_RANGES, "bytes") // .body(resource); }