前言:
1.前面讲的是在用例前加前置条件,相当于setup,既然有setup那就有teardown,fixture里面的teardown用yield来唤醒teardown的执行
看以下的代码:
#!/usr/bin/env/python # -*-coding:utf-8-*- # authour:xiapmin_pei import pytest @pytest.fixture(scope="module") def open(): print("打开浏览器,并且打开百度首页") yield print("执行teardown!") print("最后关闭浏览器") def test_s1(open): print("用例1:搜索python-1") def test_s2(open): print("用例2:搜索python-2") def test_s3(open): print("用例3:搜索python-3")
执行结果:yield之后的代码在最后才运行。
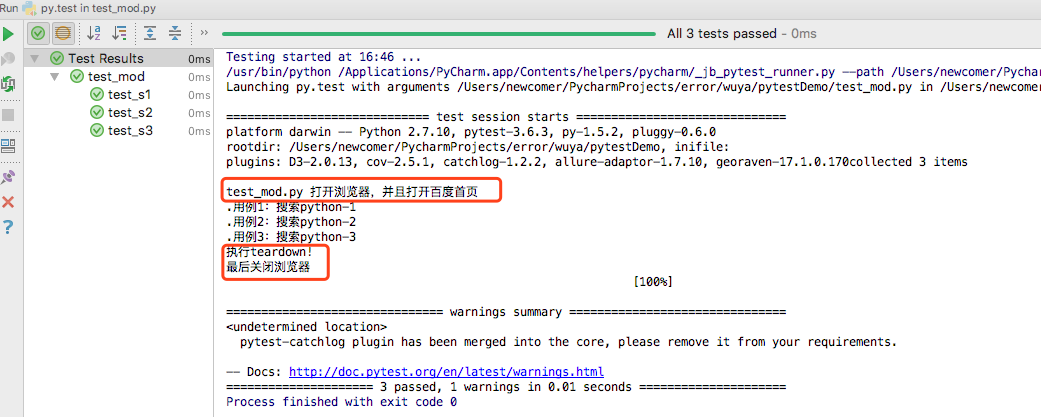
yield遇到异常:
1.如果其中一个用例出现异常,不影响yield后面的teardown执行,运行结果互不影响,并且在用例全部执行完之后,会呼唤teardown的内容
#!/usr/bin/env/python # -*-coding:utf-8-*- # authour:xiapmin_pei import pytest @pytest.fixture(scope="module") def open(): print("打开浏览器,并且打开百度首页") yield print("执行teardown!") print("最后关闭浏览器") def test_s1(open): print("用例1:搜索python-1") #如果第一个用例异常了,不影响其他的用例执行 raise NameError # 模拟异常 def test_s2(open): print("用例2:搜索python-2") def test_s3(open): print("用例3:搜索python-3")
运行结果:
============================= test session starts ==============================
platform darwin -- Python 2.7.10, pytest-3.6.3, py-1.5.2, pluggy-0.6.0
rootdir: /Users/newcomer/PycharmProjects/error/wuya/pytestDemo, inifile:
plugins: D3-2.0.13, cov-2.5.1, catchlog-1.2.2, allure-adaptor-1.7.10, georaven-17.1.0.170collected 3 items
test_mod.py 打开浏览器,并且打开百度首页
F用例1:搜索python-1
test_mod.py:14 (test_s1)
open = None
def test_s1(open):
print("用例1:搜索python-1")
#如果第一个用例异常了,不影响其他的用例执行
> raise NameError # 模拟异常
E NameError
test_mod.py:18: NameError
.用例2:搜索python-2
.用例3:搜索python-3
执行teardown!
最后关闭浏览器
[100%]
=================================== FAILURES ===================================
___________________________________ test_s1 ____________________________________
open = None
def test_s1(open):
print("用例1:搜索python-1")
#如果第一个用例异常了,不影响其他的用例执行
> raise NameError # 模拟异常
E NameError
test_mod.py:18: NameError
---------------------------- Captured stdout setup -----------------------------
打开浏览器,并且打开百度首页
----------------------------- Captured stdout call -----------------------------
用例1:搜索python-1
=============================== warnings summary ===============================
<undetermined location>
pytest-catchlog plugin has been merged into the core, please remove it from your requirements.
-- Docs: http://doc.pytest.org/en/latest/warnings.html
================ 1 failed, 2 passed, 1 warnings in 0.07 seconds ================
Process finished with exit code 0
2.如果在setup就异常了,那么是不会去执行yield后面的teardown内容了
#!/usr/bin/env/python # -*-coding:utf-8-*- # authour:xiapmin_pei import pytest @pytest.fixture(scope="module") def open(): print("打开浏览器,并且打开百度首页") raise NameError # 模拟异常 yield print("执行teardown!") print("最后关闭浏览器") def test_s1(open): print("用例1:搜索python-1") #如果第一个用例异常了,不影响其他的用例执行 raise NameError # 模拟异常 def test_s2(open): print("用例2:搜索python-2") def test_s3(open): print("用例3:搜索python-3")
运行结果:
============================= test session starts ==============================
platform darwin -- Python 2.7.10, pytest-3.6.3, py-1.5.2, pluggy-0.6.0
rootdir: /Users/newcomer/PycharmProjects/error/wuya/pytestDemo, inifile:
plugins: D3-2.0.13, cov-2.5.1, catchlog-1.2.2, allure-adaptor-1.7.10, georaven-17.1.0.170collected 3 items
test_mod.py E打开浏览器,并且打开百度首页
test setup failed
@pytest.fixture(scope="module")
def open():
print("打开浏览器,并且打开百度首页")
> raise NameError # 模拟异常
E NameError
test_mod.py:10: NameError
E
test setup failed
@pytest.fixture(scope="module")
def open():
print("打开浏览器,并且打开百度首页")
> raise NameError # 模拟异常
E NameError
test_mod.py:10: NameError
E
test setup failed
@pytest.fixture(scope="module")
def open():
print("打开浏览器,并且打开百度首页")
> raise NameError # 模拟异常
E NameError
test_mod.py:10: NameError
[100%]
==================================== ERRORS ====================================
__________________________ ERROR at setup of test_s1 ___________________________
@pytest.fixture(scope="module")
def open():
print("打开浏览器,并且打开百度首页")
> raise NameError # 模拟异常
E NameError
test_mod.py:10: NameError
---------------------------- Captured stdout setup -----------------------------
打开浏览器,并且打开百度首页
__________________________ ERROR at setup of test_s2 ___________________________
@pytest.fixture(scope="module")
def open():
print("打开浏览器,并且打开百度首页")
> raise NameError # 模拟异常
E NameError
test_mod.py:10: NameError
__________________________ ERROR at setup of test_s3 ___________________________
@pytest.fixture(scope="module")
def open():
print("打开浏览器,并且打开百度首页")
> raise NameError # 模拟异常
E NameError
test_mod.py:10: NameError
=============================== warnings summary ===============================
<undetermined location>
pytest-catchlog plugin has been merged into the core, please remove it from your requirements.
-- Docs: http://doc.pytest.org/en/latest/warnings.html
===================== 1 warnings, 3 error in 0.08 seconds ======================
Process finished with exit code 0