摘要
网上有很多个人站来分享电影资源,其实有时候我们自己也想做这个一个电影站来分享资源。但是这个时候就有一个问题,电影的资源应该从哪里来呢?难道要自己一条条手动去从网络上获取,这样无疑是缓慢而又效率低下的。这个时候我们可以用自己掌握的知识去写一个小小爬虫程序,在网络上爬去电影资源。
爬去对象---电影天堂
首先打开电影天堂的链接,从下面的图片可以看出电影天堂的电影资源都是已列表页--详情页的方式展示得,这样是非常易于爬去的。那么我这次就以电影天堂导航栏中的最新资源为例。点开最新资源,是列表也展示的,每页25条资源。列表页的访问链接是:http://www.ygdy8.net/html/gndy/dyzz/list_23_PAGE.html (其中PAGE是分页参数)
列表页:
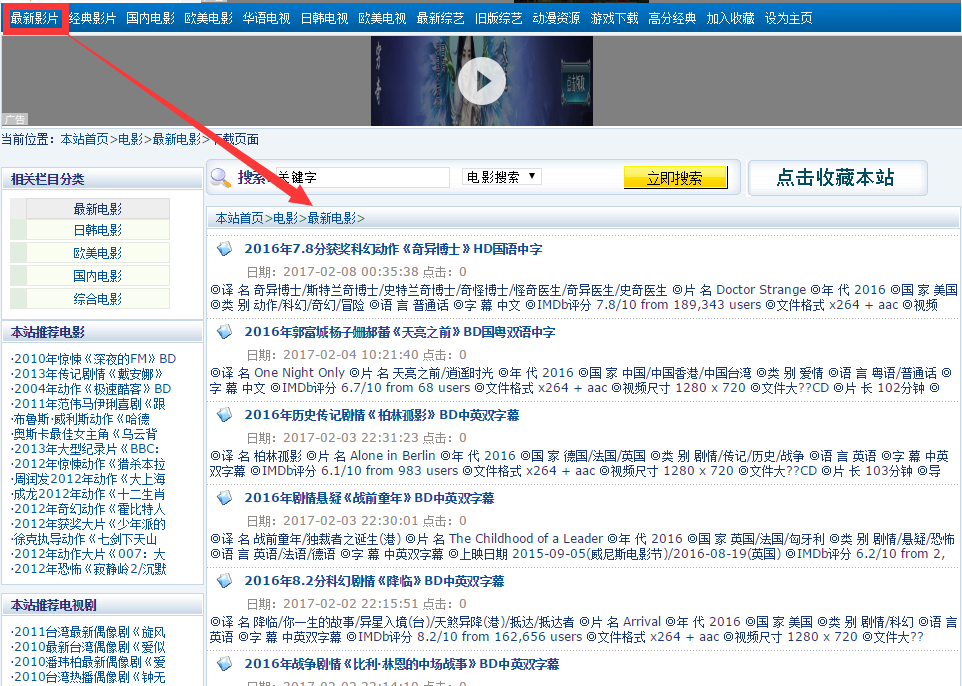

其中PAGE是分页参数,通过更改分页参数可以达到获取每一页中对应电影的详情页访问链接。再获取完详情页的链接后,再次通过访问详情页的方法,获取对应电影的下载链接和电影名字。
那么梳理一下整个的爬去过程如下:
1.找到电影天堂最新资源的列表页访问链接
2.循环更改电影列表访问链接的分页参数.
3.分析列表页页面排版规则,获取电影详情页的URL
5.访问获取的每一个详情页的的URL.分析页面.获取电影名称和电影下载链接
6.将获取的信息存入到存储介质中.
通过以上6步即可完成电影的爬去!!!
废话不多说,直接上代码!!!
爬取代码
添加需要依赖的jar:
<dependency> <groupId>org.jsoup</groupId> <artifactId>jsoup</artifactId> <version>1.7.2</version> </dependency>
CommonMethod.java 该方法类是用于获取页面的元素以及将信息写入文件中

package com.penglei.util; import com.penglei.vo.MovieVo; import java.io.*; import java.net.URL; import java.net.URLConnection; import java.util.Iterator; import java.util.List; /** * 公用的方法 * Created by penglei on 2017/2/13. */ public class CommonMethod { /** * 获取页面元素 * @param url * @return * @throws IOException */ public static String getHtmlPage(String url) throws IOException { String line; URL oul = new URL(url); //建立连接 URLConnection conn = oul.openConnection(); //获得数据 BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(conn.getInputStream(), "gbk")); StringBuilder htmlpage = new StringBuilder(); while ((line = bufferedReader.readLine()) != null) { htmlpage.append(line); } //返回页面(String类型的页面数据) return htmlpage.toString(); } /** * 将List中的信息写入txt * @param file * @param urlList */ public static void insertUrlToFile(String file, List<MovieVo> urlList) throws IOException { //下面是写文件 boolean flag = false; FileWriter fw = null; BufferedWriter bw = null; try { fw = new FileWriter(file, true); bw = new BufferedWriter(fw, 100); Iterator<MovieVo> iter=urlList.iterator(); while(iter.hasNext()) { bw.write(iter.next().getTitle()+"----["+iter.next().getDownloadUrl()+"]" + " "); } flag = true; } catch (IOException e) { System.out.println("write error"); flag = false; } finally { if (bw != null) { bw.flush(); bw.close(); } if (fw != null) fw.close(); } } }
SpiderCommon.java 该方法将被重新

package com.penglei.service; import java.io.IOException; /** * Created by penglei on 2017/2/13. */ public class SpiderCommon { public void search(String url) throws IOException { System.out.println("The method will be override!"); } }

package com.penglei.service; import com.penglei.util.CommonMethod; import org.jsoup.Jsoup; import org.jsoup.nodes.Document; import org.jsoup.nodes.Element; import org.jsoup.select.Elements; import java.io.IOException; import java.util.ArrayList; import java.util.List; /** *抓取电影天堂列表页的数据 * Created by penglei on 2017/2/13. */ public class SpiderMovieList extends SpiderCommon { private static List<String> uList =new ArrayList<String>(); @Override public void search(String url) throws IOException { //根据Url地址获取网页内容 String htmlPage = CommonMethod.getHtmlPage(url); if (htmlPage != null){ //对网页内容进行分析和提取 Document docx = Jsoup.parse(htmlPage); //获取列表页对应的table元素 Elements htmltables = docx.select("table.tbspan"); //获取a标签链接元素 Elements links = htmltables.select("a[href]"); for (Element link : links) { String linkHref = link.attr("href"); uList.add("http://www.ygdy8.net"+linkHref); } } } public List<String> getUrlList() { return uList; } /** * 得到列表页的url * @param n 一共有多少页 * @param indexurl 页面的url * @throws IOException */ public static List getAllMovieUrl(int n,String indexurl) throws IOException { Integer i=1; SpiderMovieList spiderMovieList = new SpiderMovieList(); System.out.println("***********开始爬取列表页***********"); for (i = 1; i <= n; i++) { String url = indexurl.replace("PAGE",i.toString()); spiderMovieList.search(url); System.out.println("info"+"爬取第"+i+"页"); } System.out.println("***********列表页爬取完成***********"); List<String> resultList=spiderMovieList.getUrlList(); return resultList; } }
SpiderMovieDetail.java 获取电影详情页资源

package com.penglei.service; /** * Created by penglei on 2017/2/13. */ import com.penglei.util.CommonMethod; import com.penglei.vo.MovieVo; import org.jsoup.Jsoup; import org.jsoup.nodes.Document; import org.jsoup.nodes.Element; import org.jsoup.select.Elements; import java.io.IOException; /** * 抓取每个电影对应的列表页的数据,获取对应的电影名称和电影下载链接 */ public class SpiderMovieDetail extends SpiderCommon { private MovieVo movieVo; @Override public void search(String url) throws IOException { movieVo = new MovieVo(); String htmlpage = CommonMethod.getHtmlPage(url); if (htmlpage != null){ //对网页内容进行分析和提取 Document doc = Jsoup.parse(htmlpage); //获取标题 Element link = doc.select("div.title_all").last(); if(link!=null){ String title = link.text(); //获取链接 Elements elements = doc.select("div#Zoom"); Element element = elements.select("a[href]").first(); if(element!=null){ String href = element.attr("href"); movieVo.setDownloadUrl(href); } //将标题加入到MovieVo对象中 movieVo.setTitle(title); } } } public MovieVo getMovie() { return movieVo; } }

package com.penglei; import com.penglei.service.SpiderMovieDetail; import com.penglei.service.SpiderMovieList; import com.penglei.util.CommonMethod; import com.penglei.vo.MovieVo; import java.io.IOException; import java.util.ArrayList; import java.util.List; /** * Created by penglei on 2017/2/13. */ public class DemoRun { /** * PAGE是电影天堂的分页参数,所以通过循环更改PAGE来抓取某一页 */ //最新 private static String INDEX_DYZZ="http://www.ygdy8.net/html/gndy/dyzz/list_23_PAGE.html"; public static void main(String[] args) throws IOException, InterruptedException { List<MovieVo> movieVos=new ArrayList<MovieVo>(); SpiderMovieDetail detail=new SpiderMovieDetail(); //获取列表页中所有电影的详情页访问链接 List<String> list = SpiderMovieList.getAllMovieUrl(157, INDEX_DYZZ); System.out.println(list.size()); for (int i = 0; i < list.size(); i++) { //获取详情页数据 detail.search(list.get(i)); movieVos.add(detail.getMovie()); System.out.println("**********爬取详情页**********"+i+"完成"); } System.out.println("**********开始执行插入**********"); //将获取资源写入txt CommonMethod.insertUrlToFile("E:\AMark\StudyCode\seed.txt",movieVos); System.out.println("**********插入完成**********"); } }
代码结构如下:
访问最新资源共获取3923条电影资源。
对应代码资源地址是:https://github.com/whitescholars/spider.git
结语
如果你还需要了解更多技术文章信息,请继续关注白衣秀才的博客
个人网站:http://penglei.top/
Github:https://github.com/whitescholars
微博:http://weibo.com/u/3034107691?refer_flag=1001030102_&is_all=1