队列课下作业
作业要求:
- 补充课上没有完成的作业
- 参考15.3节,用自己完成的队列(链队,循环数组队列)实现模拟票务柜台排队功能
- 用JDB或IDEA单步跟踪排队情况,画出队列变化图,包含自己的学号信息
- 把代码推送到代码托管平台
- 把完成过程写一篇博客:重点是单步跟踪过程和遇到的问题及解决过程
- 提交博客链接
代码实现:
链队实现:
import exception.*;
public class MyLinkedQueue<T> implements QueueADT<T>
{
private int total;
private LinearNode<T> front, rear;
public MyLinkedQueue()
{
total = 0;
front = null;
rear = null;
}
public void enqueue (T element)
{
LinearNode<T> e = new LinearNode<>(element);
if (!isEmpty()){
rear.setNext(e);
}else {
front = e;
}
total += 1;
rear = e;
}
public T dequeue() throws EmptyCollectionException
{
if (isEmpty())throw new EmptyCollectionException("LinearQueue");
LinearNode<T> e = front;
front = front.getNext();
total -= 1;
if (isEmpty())rear = null;
return e.getElement();
}
public T first() throws EmptyCollectionException
{
if (isEmpty())throw new EmptyCollectionException("LinearQueue");
return front.getElement();
}
public boolean isEmpty()
{
return total==0?true:false;
}
public int size()
{
return total;
}
public String toString()
{
String rlt = "REAR OF QUEUE
";
LinearNode<T> currentNode = rear;
while (currentNode !=null){
rlt += currentNode.getElement().toString();
rlt += "
";
currentNode = rear.getNext();
}
return rlt+"FRONT OF QUEUE";
}
}
数组队列实现:
import exception.*;
public class MyCircularArrayQueue<T> implements QueueADT<T> {
private final int DEFAULT_CAPACITY = 10;
private int front, rear, count;
private T[] queue;
public MyCircularArrayQueue() {
front = rear = count = 0;
queue = (T[]) (new Object[DEFAULT_CAPACITY]);
}
public void enqueue(T element) {
if (count == queue.length)
expandCapacity();
queue[rear] = element;
rear = (rear + 1) % queue.length;
count += 1;
}
@Override
public T dequeue() throws EmptyCollectionException {
if (isEmpty()) throw new EmptyCollectionException("CircularQueue");
T rlt = queue[front];
front = (front + 1) % queue.length;
count -= 1;
return rlt;
}
@Override
public int size() {
return count;
}
@Override
public boolean isEmpty() {
return count==0?true:false;
}
@Override
public T first() throws EmptyCollectionException {
if (isEmpty()) throw new EmptyCollectionException("CircularQueue");
return queue[front];
}
public void expandCapacity() {
T[] larger = (T[]) (new Object[queue.length * 2]);
for (int index = 0; index < count; index++)
larger[index] = queue[(front + index) % queue.length];
front = 0;
rear = count;
queue = larger;
}
}
模拟柜台实现:
import java.util.ArrayList;
public class MockBooking {
final static int PROCESS = 120;
final static int MAX_CASHIERS = 10;
final static int NUM_CUSTOMERS = 100;
public static void main(String[] args) {
Customer customer;
MyCircularArrayQueue<Customer> customerQueue = new MyCircularArrayQueue<>();
int[] cashierTime = new int[MAX_CASHIERS];
int totalTime, averageTime, departs;
for (int cashiers=0; cashiers < MAX_CASHIERS; cashiers++) {
for (int count=0; count < cashiers; count++)
cashierTime[count] = 0;
for (int count=1; count <= NUM_CUSTOMERS; count++)
customerQueue.enqueue(new Customer(count*15));
totalTime = 0;
while (!(customerQueue.isEmpty()))
{
for (int count=0; count <= cashiers; count++)
{
if (!(customerQueue.isEmpty()))
{customer = customerQueue.dequeue();
if (customer.getArrivalTime() > cashierTime[count])
departs = customer.getArrivalTime() + PROCESS;
else
departs = cashierTime[count] + PROCESS;
customer.setDepartureTime (departs);
cashierTime[count] = departs;
totalTime += customer.totalTime();
}
}
}
averageTime = totalTime / NUM_CUSTOMERS;
System.out.println ("Number of cashiers: " + (cashiers+1));
System.out.println ("Average time: " + averageTime + "
");
}
}
}
单步跟踪
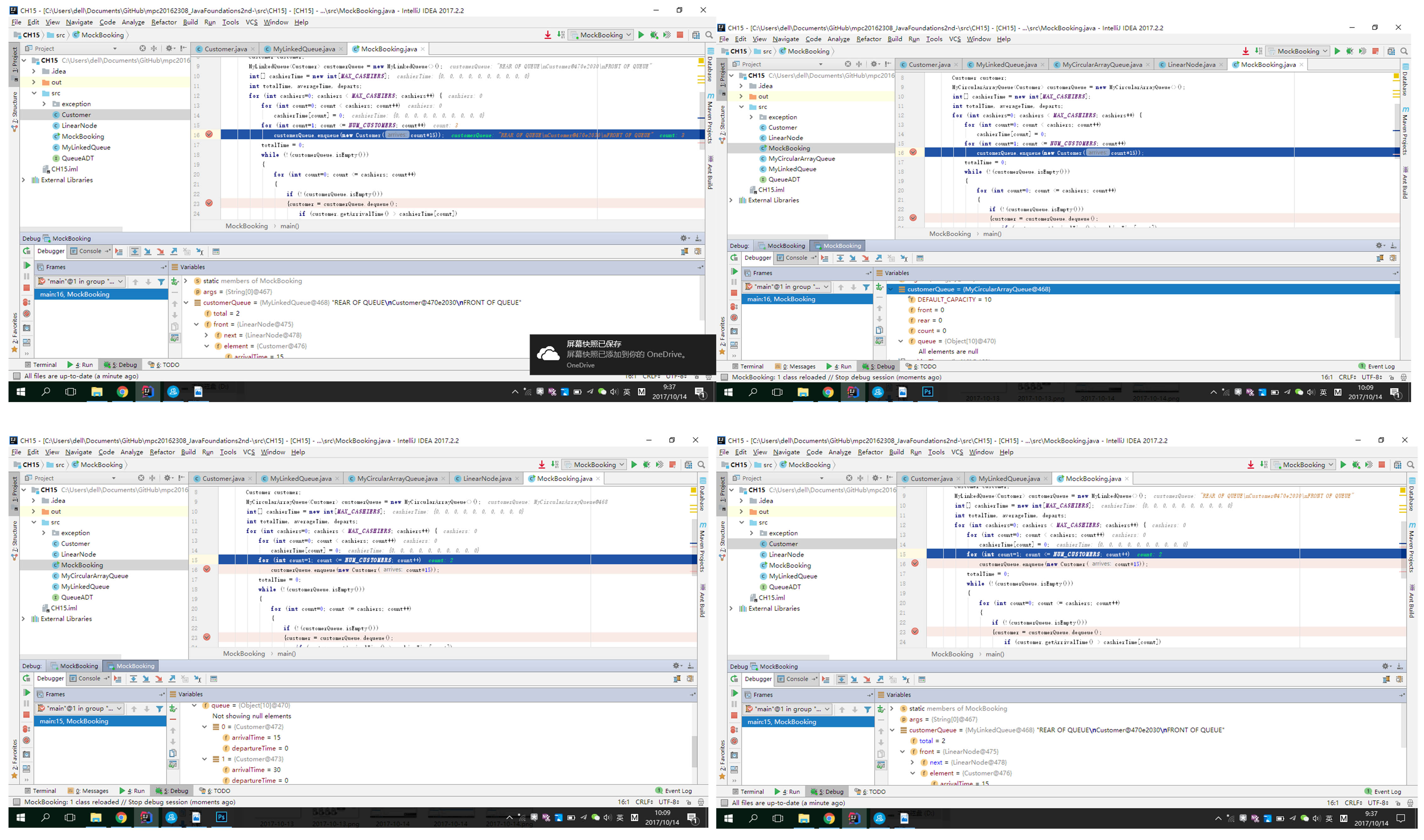
队列变化图:
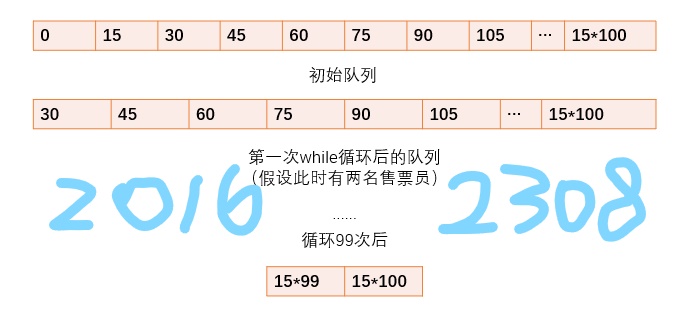