
1 public class One{ 2 public static void main(String args[]){ 3 String s1,s2; 4 s1=new String("天道酬勤"); 5 s2=new String("天道酬勤"); 6 System.out.println(s1.equals(s2));//true 7 System.out.println(s1==s2);//false 8 String s3,s4; 9 s3="勇者无敌"; 10 s4="勇者无敌"; 11 System.out.println(s1.equals(s2));//true 12 System.out.println(s1==s2);//true 13 } 14 }

1 import java.util.*; 2 public class Two{ 3 public static void mian(String args[]){ 4 String a[]={"melon","apple","pear","banana"}; 5 String b[]=new String(a,a.length); 6 System.out.println("使用用户定义SortString类,按照字典序列排列数组a:"); 7 SortString.sort(a);//自定义排列 8 for(String s:a){ 9 System.out.print(" "+s); 10 System.out.println(""); 11 System.out.println("使用类库中的Arrays类,按照字典序列排列数组b:"); 12 Arrays.sort(b); 13 for(String s:b//系统库类排列 14 System.out.print(" "+s); 15 } 16 } 17 } 18 } 19 class SortString{ 20 public static void sort(String a[]){ 21 int count=0; 22 for(int i=0;i<a.lengt-1;i++){ 23 for(int j=i;j<a.length;j++){ 24 if(a[i].compareTo(a[j])>0){//按照字典序比较(升序排序),若a[i]>a[j],交换 25 System.out.printf("交换%s和%s",a[i],a[j]); 26 count++; 27 String temp=a[i]; 28 a[i]=a[j]; 29 a[j]=temp; 30 System.out.println(“第”+count+"次的排序结果:"); 31 System.out.println(Arrays.toString(a)); 32 } 33 } 34 } 35 } 36 }

1 //字符串与字符数组 2 //getChars方法和toArrayString方法 3 public class String3{ 4 public static void main(String args[]){ 5 char[] a,b,c; 6 String s="2009年10月1日是国庆60周年"; 7 a=new char[2]; 8 s.getChars(11,13,a,0);//用getChars,数组a的值为国庆 9 System.out.println(a);//只有char类型数组才能直接用数组名输出数组值,若要输出字符数组的首地址,则System.out.println(""+a) 10 c="十一长假期间,学校都放假了".toCharArray();//字符串转变成字符数组 11 System.out.println(c); 12 } 13 }

1 //public byte[] getBytes()....默认的字符编码 2 //public byte[] getBytes(String charsetName).....使用参数制定的字符编码 3 //如果平台默认的字符编码是GB_2312(国际,中文简体),那么调用getBytes()方法等同于调用getBytes("GB2312"),但需要注意的是,带参数的getBytes(String charsetName)抛出UnsupportedEncodingException异常,因此,必须在try~catch语句中调用getBytes(String charsetName)。 4 public class String4{ 5 public static void main(String args[]){ 6 byte[] d="Java你好".getBytes(); 7 System.out.println("数组d的长度是:"+d.length); 8 String s=new String(d.6,2); 9 System.out.println(S);//输出:好 (一个汉字站两个字节) 10 s=new String(d,0,6); 11 System.out.println(s);//输出:你 12 } 13 }

1 //正则表达式 2 import java.util.Scanner; 3 public class String5{ 4 public static void main(String args[]){ 5 String regex="[a-zA-Z]+";//一个或者多个字母组成 6 Scanner s=new Scanner(System.in); 7 String str=s.nextLine();//输入字符串 8 if(str.matches(regex)){ 9 System.out.println(str+"中的字符都是英文字符"); 10 } 11 } 12 }

1 //public String replaceAll(String regex,String replacement); 2 public class String6{ 3 public static void mian(String args[]){ 4 String str="欢迎大家访问http://www.xiaojiang.cn了解、参观公司"; 5 String regex="(http://|www)\56?\\w+\56{1}\\w+\56{1}\\p{Alpha}+"; 6 System.out.printf("剔除\n\"%s\"\n中的网站链接信息后得到的字符串:\n",str); 7 String s=str.replaceAll(regex,"");//把字符串str与regex匹配的内容替换成空内容 8 System.out.println(s);//输出:欢迎大家访问了解、参观公司 9 } 10 }

1 //public String[] split(String regex) 2 import java.util.Scanner; 3 public class Stringl{ 4 public static void main(String args[]){ 5 System.out.println("一行文本:"); 6 Scanner scanner=new Scanner(System.in); 7 String str=scanner.nextLine(); 8 String regex="[\\s\\d\\p{Punct}]+";//空格、数字和符号 9 int count=0; 10 String a[]=str.split(regex); 11 for(String s:a){ 12 count++; 13 System.out.printf("第%d个单词:%s\n",count,s); 14 } 15 } 16 }
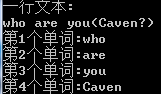

1 //StringBuffer类 2 //StringBuffer类的常用方法: 3 //1、append方法:StringBuffer append(String s)、StringBuffer append(int n)、StringBuffer append(Object o)……) 4 //2、public char charAt(int n)和public void setCharAt(int n,char ch): charAt(int n)返回参数n指定位置上的单个字符(从0开始),n必须是非负数;setCharAt(int n,char ch)将当前对象实体中的字符串位置n处的字符用参数ch指定的字符替换 5 //3、StringBuffer insert(int index,String str)将参数str指定的字符串插入到index指定的位置,并返回当前对象的引用 6 //4、public StringBuffer reverse()将该实体中的字符翻转,并返回当前的引用 7 //5、StringBuffer delete(int startindex,int endindex)将对象实体中的字符串中删除一个子字符串(从startindex到endindex-1) 8 //6、StringBuffer replace(int startindex,int endindex,String str) 9 10 public class Stringl{ 11 public static void main(String args[]){ 12 StringBuffer str=new StringBuffer(); 13 str.append("大家好"); 14 System.out.println("str:"+str); 15 System.out.println("length:"+str.length());//输出:length:3 16 System.out.println("capacity:"+str.capacity());//输出:capacity:16 17 str.setCharAt(0,'w');//用"w"把"0"位置上的字符(即"大")替换 18 str.setCharAt(1,'e');//用"e"把"1"位置上的字符(即"家")替换 19 System.out.println("str:"+str);//输出:we好 20 str.insert(2,"are all");//在下标为2的地方插入"are all" 21 System.out.println("str:"+str);//输出:we are all好 22 int index=str.indexOf("好");//返回字符串“好”在对象字符串中首次出现的索引 23 str.replace(index,str.length()," right");//将index到str.length()-1的子字符串用“right”替换 24 System.out.println("str:"+str);//输出:we are all right 25 } 26 }

1 //StringTokenizer类分解字符串(不用正则表达时) 2 //两个构造方法(一个StringTokenizer对象为一个字符分析器,使用nextToken()方法逐个获取字符串中的语言符号(单词),每当调用nextToken()时,分析器中负责计数的变量就会自动减1,计数变量的初始值等于字符串的单词数目。通常用while循环逐个获取单词,用hasMoreTokens()方法,只要字符串中还有语言符号即计数变量大于0,该方法返回true,否则返回false。另外还可以随时让分析器调用countTokens()方法得到分析器中计数变量的值):1、StringTokenizer(String s)使用默认的分隔标记符(空格、换行符、回车符…); 2、StringTokenizer(String s,String delim)参数delim中的字符被作为分隔标记 3 import java.util.*; 4 public class Stringl{ 5 public static void main(String[] args){ 6 String s="you are welcome(thank you),nice to meet you"; 7 StringTokenizer fenxi=new StringTokenizer(s,"() ,"); 8 System.out.println("读取前构造器中共有单词:"+fenxi.countTokens()); 9 while(fenxi.hasMoreTokens()){ 10 String str=fenxi.nextToken(); 11 System.out.print(str+" "); 12 } 13 System.out.println(""); 14 System.out.println("读取后构造器中还有单词:"+fenxi.countTokens()); 15 } 16 }

1 //Scanner类 2 //用Scanner类从字符串中解析程序所需要的数据 3 //Scanner对象可以调用nextInt()和nextDouble()方法将数字型单词传化为int型或 4 者double型数据返回,但需要注意的是,如果单词不是数字型单词,调用nextInt()或 5 nextDouble()将发生InputMismatchException异常,在处理异常时可以调用next方法返回该非数字化的单词。 6 7 8 //1、使用默认分隔标记解析字符串 9 import java.util.*; 10 public class Stringl{ 11 public static void main(String args[]){ 12 String cost="Tv cost 876 dollar.Computer cost 2398 dollar.telephone cost 1278 dollar"; 13 Scanner scanner=new Scanner(cost); 14 double sum=0; 15 while(scanner.hasNext()){ 16 try{ 17 double price=scanner.nextDouble(); 18 sum+=price; 19 } 20 catch(InputMismatchException e){ 21 String t=scanner.next(); 22 } 23 } 24 System.out.println("总消费:"+sum+"元"); 25 } 26 } 27 28 //2、使用正则表达式作为分隔标记来解析字符串(Scanner对象调用useDelimiter(正则表达式)) 29 import java.util.*; 30 public class Stringl{ 31 public static void main(String args[]){ 32 String cost="话费清单:市话费76.89元,长途话费:167.38元,短信费:12.68元"; 33 Scanner scanner=new Scanner(cost); 34 scanner.useDelimiter("[^1234567890.]+"); 35 double sum=0; 36 while(scanner.hasNext()){ 37 try{ 38 double price=scanner.nextDouble(); 39 sum+=price; 40 System.out.println(price); 41 } 42 catch(InputMismatchException e){ 43 String t=scanner.next(); 44 } 45 } 46 System.out.println("总和为:"+sum); 47 } 48 }

1 //Math类 2 //java.lang包中Math类,以下是常用的Math类 3 /* 4 public static long abs(double a) 5 public static double max(double a,double b) 6 public static double min(double a,double b) 7 public static double max(double a,double b) 8 public static double random();产生一个0到1之间的随机数(不包括0和1) 9 public static double pow(double a,double b);a的b次幂 10 public static double sqrt(double a);a的平方根 11 public static double log(double a);a的对的 12 public static double sin(double a);返回正弦值 13 public static double asin(double a);返回反正弦值 14 */ 15 16 //BigInteger类 17 //java.math包中的BigInteger提供任意精度的整数运算,可用构造方法public BigIneger(String val)构造一个十进制 BigInteger对象。该构造方法可以发生NumberFormatException异常,也就是说,字符串参数val中如果含有非数字字符就会发生NumberFormatException异常。常用方法: 18 /* 19 1、public BigInteger add(BigInteger val);加 20 2、public BigInteger subtract(BigInteger val);差 21 3、public BigInteger multiply(BigInteger val);积 22 4、public BigInteger divide(BigInteger val);商 23 5、public BigInteger remainder(BigInteger val);余 24 6、public int compareTo(BigInteger val);比较 25 7、public BigInteger abs(); 26 8、public BigInteger pow(int a);大整数的a次幂 27 9、public BigInteger toString();返回当前大正整数对象十进制的字符串表示 28 10、public BigInteger toString(int p);//返回当前大正整数对象p进制的字符串表示 29 */ 30 31 //例题 32 import java.math.*; 33 public class Stringl{ 34 public static void main(String args[]){ 35 double a=5.0; 36 double st=Math.sqrt(a);//平方根 37 System.out.println(a+"的平方根"+st); 38 BigInteger result=new BigInteger("0"),//构造大整数对象() 39 one=new BigInteger("123456789"), 40 two=new BigInteger("987654321"); 41 result=one.add(two); 42 System.out.println("和:"+result); 43 result=one.multiply(two); 44 System.out.println("积:"+result); 45 } 46 }

1 import java.util.*; 2 public class Stringl{ 3 public static void main(String args[]){ 4 Scanner scanner=new Scanner(System.in); 5 int sum=0; 6 System.out.println("输入一个整数:"); 7 while(scanner.hasNext()){ 8 int number=scanner.nextInt(); 9 sum+=number; 10 System.out.println("目前和:"+sum); 11 if(sum>8000) 12 System.exit(0); 13 System.out.println("输入一个整数(输入非整数结束)"); 14 } 15 System.out.println("总和:"+sum); 16 } 17 }

1 //Pattern与Match类 2 /*模式对象Pattern(对正则表达式的封装),Pattern类调用compile(String regex)方法返回一个 3 4 模式对象,如Pattern p=Pattern.compile("hello\\d");如果regex指定的正则表达式有错, 5 6 compile方法将抛出异常:PatternSyntaxException。Pattern还可以调用方法compile(String 7 8 regex,int flags)返回一个Pattern对象,参数flags可以取有效值:Pattern.CASE_INSENSITIVE、 9 10 Pattern.MULTLINE、Pattern.DOTALL、Pattern.UNICODE_CASE、Pattern.CANON_EQ,例如,当flags 11 12 取值Pattern.CASE_INSENSITIVE, 13 模式匹配将忽略大小写 14 */ 15 //匹配对象Match 16 import java.util.regex.*; 17 public class Stringl{ 18 public static void main(String args[]){ 19 Pattern p;//模式对象 20 Matcher m;//匹配对象 21 String regex="(http://|www)\56?\\w+\56{1}\\w+\56{1}\\p{Alpha}+"; 22 p=Pattern.compile(regex); 23 String s="清华大学网址:www.tsinghua.edu.cn,邮电出版社的网址:http://www.ptpress.com"; 24 m=p.matcher(s); 25 while(m.find()){ 26 String str=m.group(); 27 System.out.println(str); 28 } 29 System.out.println("剔除字符串中网址后得到的新字符串:"); 30 String str1=m.replaceAll(""); 31 System.out.println(str1); 32 } 33 }