1.求矩形、正方形和圆形的面积与周长
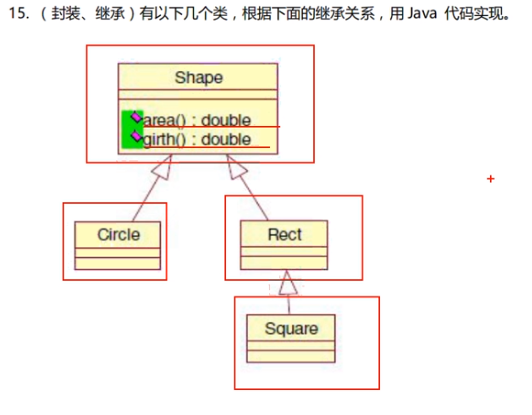
package randi;
import static java.lang.Math.PI;
public class ShapeDemo {
public static void main(String[] args) {
Shape[] shas = new Shape[] { new Circle(10), new Rect(5, 6), new Squr(10) };
Shape sha = new Shape();
for (int i = 0; i < shas.length; i++) {
sha.print(shas[i]);
}
// Circle s1 = new Circle(10);
// Rect s2 = new Rect(5, 6);
// Squr s3 = new Squr(10);
//
// sha.print(s1);
// sha.print(s2);
// sha.print(s3);
}
}
class Shape {
public void print(Shape sha) {
System.out.println(sha.getName() + "面积: " + sha.getArea());
System.out.println(sha.getName() + "周长: " + sha.getGirth());
System.out.println("---------------");
}
public double getArea() {
return -1;
}
public double getGirth() {
return -1;
}
public String getName() {
return null;
}
}
class Circle extends Shape {
private double radius;
public Circle() {}
public Circle(double radius) {
this.radius = radius;
}
public double getArea() {
return PI * radius * radius;
}
public double getGirth() {
return PI * radius * 2;
}
public String getName() {
return "Cir";
}
}
class Rect extends Shape {
private double length;
private double width;
public Rect() {}
public Rect(double length, double width) {
this.length = length;
this.width = width;
}
public double getArea() {
return width * length;
}
public double getGirth() {
return (width + length) * 2;
}
public String getName() {
return "Rect";
}
}
class Squr extends Rect {
public Squr() {}
public Squr(double length) {
super(length, length);
}
public String getName() {
return "Squr";
}
}