<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>vue</title>
</head>
<body>
<div id="app">
<h2>{{ msg }}</h2>
<h2>{{ 1+1 }}</h2>
<h2>{{ {"name":"日天"} }}</h2>
<h3>{{ person.name }}</h3>
<h2>{{ 1>2? "真的":"假的" }}</h2>
<p>{{ msg2.split("").reverse().join("") }}</p>
<div>{{ text }}</div>
</div>
<script src="vue.js"></script>
<script>
new Vue({
el:"#app",
data:{
msg:"呵呵",
person:{
name:"dasabi"
},
msg2:"hello vue",
text:"<h2>ritian</h2>"
}
})
</script>
</body>
</html>
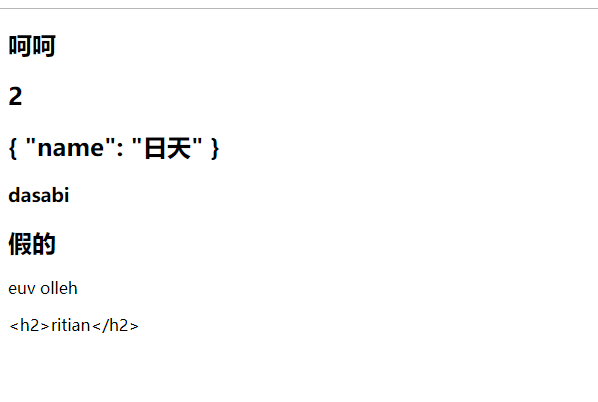
1. v-text和v-html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>v-text和v-html</title>
</head>
<body>
<div id="app">
{{ msg }}
<div v-text="msg"></div>
<hr>
<div v-html="msg"></div>
</div>
<script src="vue.js"></script>
<script>
new Vue({
el:"#app",
data(){
//data中是一个函数,函数中return一个对象,可以是空对象,但不能不return
return {msg:"<h2>ritian</h2>"}
}
})
</script>
</body>
</html>
2. v-if和v-show
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>v-if和v-show</title>
<style>
.box{
width: 200px;
height: 200px;
background-color: red;
}
.box2{
width: 200px;
height: 200px;
background-color: blue;
}
</style>
</head>
<body>
<div id="app">
{{ add(1,2) }}
<button v-on:click = "handlerClick">隐藏</button>
<div class="box" v-show = "isShow"></div>
<div class="box2" v-if = "isShow"></div>
<div v-if = "Math.random() > 0.5">有了</div>
<div v-else>没了</div>
</div>
<script src="vue.js"></script>
<script>
new Vue({
el:"#app",
data() {
return {
msg:"<h2>ritian</h2>",
num:1,
isShow:true
}
},
methods:{
add(x,y){
console.log(this.num);
return x + y;
},
handlerClick(){
console.log(this);
this.isShow = !this.isShow;
}
}
})
</script>
</body>
</html>
3. v-bind和v-on
<!DOCTYPE html>
<html lang="en" xmlns:v-bind="http://www.w3.org/1999/xhtml">
<head>
<meta charset="UTF-8">
<title>v-bind和v-on</title>
<style>
.box{
width: 200px;
height: 200px;
background-color: red;
}
.active{
background-color: green;
}
</style>
</head>
<body>
<div id="app">
<!-- v-bind 标签中的所有属性 img标签sr alt, a标签的href title id class -->
<button v-on:click = "handlerChange">切换颜色</button>
<img v-bind:src="imgSrc" v-bind:alt="msg">
<div class="box" v-bind:class = "{active:isActive}"></div>
</div>
<script src="./vue/dist/vue.js"></script>
<script>
// v-bind和v-on的简便写法: v-bind为:,v-on为@
new Vue({
el:"#app",
data(){
return {
imgSrc:"./img/1.jpg",
msg:"美女",
isActive:true
}
},
methods:{
handlerChange(){
this.Active = !this.isActive;
this.isActive = false;
},
handlerLeave(){
this.isActive = true;
}
}
})
</script>
</body>
</html>
4. v-for
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<style>
.box{
width: 200px;
height: 200px;
background-color: red;
}
.avtive{
background-color: green;
}
</style>
</head>
<body>
<div id="app">
<ul v-if = "data.status === 'ok'">
<li v-for = "(item,index) in data.users" :key = "item.id" >
<h3>{{ item.id }} -----------{{ item.name }} ----------{{ item.age }}</h3>
</li>
</ul>
<div v-for = "(value,key) in peron">{{ key }} --------{{ value }}</div>
</div>
<script src="./vue/dist/vue.js"></script>
<script>
new Vue({
el:"#app",
data(){
return {
data:{
status:"ok",
users:[
{id:1,name:"aaa",age:10},
{id:2,name:"bbb",age:20},
{id:3,name:"ccc",age:30},
]
},
person:{
name:"ddd"
}
}
},
methods:{}
})
</script>
</body>
</html>
5. vue中使用ajax
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>vue-ajax</title>
<style>
span.active {
color: red;
}
</style>
</head>
<body>
<div id="app">
<div>
<span @click="handlerClick(index,category.id)" v-for="(category,index) in categoryList" :key="category.id"
:class="{active:currentIndex == index}">{{ category.name }}</span>
</div>
</div>
<script src="vue/dist/vue.js"></script>
<script src="vue/dist/jquery.js"></script>
<script>
let vm = new Vue({
el: "#app",
data() {
return {
categoryList: [],
currentIndex: 0
}
},
methods: {
handlerClick(i, id) {
this.currentIndex = i;
$.ajax({
url: `https://www.luffycity.com/api/v1/courses/?sub_category=${id}`,
type: "get",
success: (data) => {
var data = data.data;
console.log(data);
}
})
}
},
created() {
$.ajax({
url: "https://www.luffycity.com/api/v1/course_sub/category/list/",
type: "get",
success: (data) => {
if (data.error_no === 0) {
var data = data.data;
let obj = {
id: 0,
name: "全部",
category: 0
};
this.categoryList = data;
this.categoryList.unshift(obj)
}
},
error: function (err) {
console.log(err)
}
})
}
})
</script>
</body>
</html>
6. 音乐播放器
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>音乐播放器</title>
<style>
ul li.active {
background-color: #f0ad4e;
}
</style>
</head>
<body>
<div id="music">
<!-- @ended 播放完成会自动调用该方法 -->
<audio :src="musicData[currentIndex].songSrc" controls autoplay @ended = "nextHandler"></audio>
<ul>
<li @click = "songHandler(index)" v-for = "(item,index) in musicData" :key = "item.id" :class = "{active:index === currentIndex}">
<h2>歌手: {{ item.author }}</h2>
<p>歌名: {{ item.name }}</p>
</li>
<button @click = "prevHandler">上一首</button>
<button @click = "nextHandler">下一首</button>
<button @click = "suiji">随机播放</button>
<button @click = "xunhuan">单曲循环</button>
</ul>
</div>
<script src="vue/dist/vue.js"></script>
<script>
var musicData = [
{
id: 1,
name: "于荣光-少林英雄",
author: "于荣光",
songSrc: "./static/于荣光 - 少林英雄.mp3"
},
{
id: 2,
name: "Joel Adams - Please Dont Go",
author: "Joel Adams",
songSrc: "./static/Joel Adams - Please Dont Go.mp3"
},
{
id: 3,
name: "MKJ - Time",
author: "MKJ",
songSrc: "./static/MKJ - Time.mp3"
},
{
id: 4,
name: "Russ - Psycho (Pt. 2)",
author: "Russ",
songSrc: "./static/Russ - Psycho (Pt. 2).mp3"
},
];
new Vue({
el: "#music",
data() {
return {
musicData: [],
currentIndex: 0
}
},
methods: {
songHandler(i) {
this.currentIndex = i;
},
nextHandler() {
this.currentIndex++;
},
prevHandler() {
this.currentIndex--;
},
suiji(){
this.currentIndex = Math.ceil(Math.random()*5);
},
xunhuan(){
this.currentIndex = this.currentIndex
}
},
created() {
this.musicData = musicData
}
})
</script>
</body>
</html>
7. 计算属性
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>计算属性</title>
</head>
<body>
<div id="app">
<p>{{ msg }}</p>
<p>{{ myMsg }}</p>
<button @click = "clickHandler">修改</button>
</div>
<script src="./vue/dist/vue.js"></script>
<script>
let vm = new Vue({
el:"#app",
data(){
return {
msg:"alex",
age:18
}
},
created(){
setInterval(() => {
})
},
methods:{
clickHandler(){
this.msg = "wusir";
this.age = 20;
},
},
computed:{
myMsg:function () {
return `我的名字叫${this.msg},年龄是${this.age}`;
}
}
})
</script>
</body>
</html>