多系统(异构系统)进行交互时,一种良好的方式便是调用Web Service,本示例基于Apache组织的CXF
环境:
Eclipse
JDK6
Tomcat6
CXF2.6.1
Spring3
示例项目结构图:
![]()
![]()
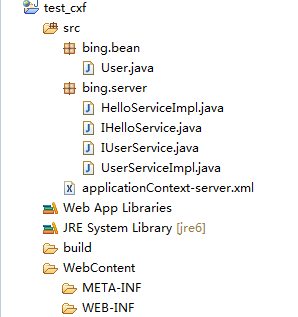
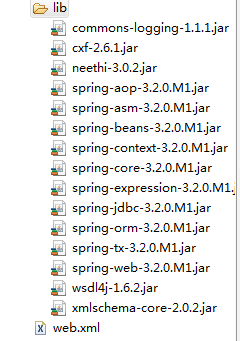
![]()
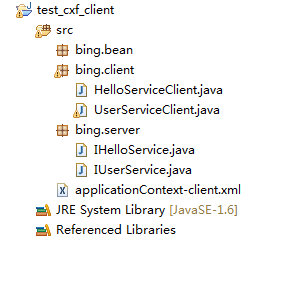
IHelloService.java
HelloServiceImpl.java
HelloServiceClient.java
applicationContext-server.xml

<?xml version="1.0" encoding="UTF-8"?>

<beans xmlns="http://www.springframework.org/schema/beans"

xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"

xmlns:jaxws="http://cxf.apache.org/jaxws"

xsi:schemaLocation="http://www.springframework.org/schema/beans

http://www.springframework.org/schema/beans/spring-beans.xsd

http://cxf.apache.org/jaxws http://cxf.apache.org/schemas/jaxws.xsd">

<!--

***注意***

手动添加的内容:

xmlns:jaxws="http://cxf.apache.org/jaxws"

http://cxf.apache.org/jaxws http://cxf.apache.org/schemas/jaxws.xsd"

-->

<import resource="classpath:META-INF/cxf/cxf.xml" />

<import resource="classpath:META-INF/cxf/cxf-extension-soap.xml" />

<import resource="classpath:META-INF/cxf/cxf-servlet.xml" />


<jaxws:endpoint id="helloService" implementor="bing.server.HelloServiceImpl" address="/helloService" />

</beans>

applicationContext-client.xml

<?xml version="1.0" encoding="UTF-8"?>

<beans xmlns="http://www.springframework.org/schema/beans"

xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"

xmlns:jaxws="http://cxf.apache.org/jaxws"

xsi:schemaLocation="http://www.springframework.org/schema/beans

http://www.springframework.org/schema/beans/spring-beans.xsd

http://cxf.apache.org/jaxws http://cxf.apache.org/schemas/jaxws.xsd">

<!--

***注意***

手动添加的内容:

xmlns:jaxws="http://cxf.apache.org/jaxws"

http://cxf.apache.org/jaxws http://cxf.apache.org/schemas/jaxws.xsd"

-->

<import resource="classpath:META-INF/cxf/cxf.xml" />

<import resource="classpath:META-INF/cxf/cxf-extension-soap.xml" />

<import resource="classpath:META-INF/cxf/cxf-servlet.xml" />


<bean id="client" class="bing.server.IHelloService" factory-bean="clientFactory" factory-method="create" />


<bean id="clientFactory" class="org.apache.cxf.jaxws.JaxWsProxyFactoryBean">

<property name="serviceClass" value="bing.server.IHelloService" />

<property name="address" value="http://localhost:8080/CXFDemo/ws/helloService" />

</bean>

</beans>

web.xml

<?xml version="1.0" encoding="UTF-8"?>

<web-app version="3.0"

xmlns="http://java.sun.com/xml/ns/javaee"

xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"

xsi:schemaLocation="http://java.sun.com/xml/ns/javaee

http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd">

<display-name>CXFDemo</display-name>

<context-param>

<param-name>contextConfigLocation</param-name>

<param-value>classpath:applicationContext-server.xml</param-value>

</context-param>

<listener>

<listener-class>org.springframework.web.context.ContextLoaderListener</listener-class>

</listener>

<servlet>

<servlet-name>CXFServlet</servlet-name>

<display-name>CXFServlet</display-name>

<servlet-class>org.apache.cxf.transport.servlet.CXFServlet</servlet-class>

<load-on-startup>1</load-on-startup>

</servlet>

<servlet-mapping>

<servlet-name>CXFServlet</servlet-name>

<url-pattern>/ws/*</url-pattern>

</servlet-mapping>

<welcome-file-list>

<welcome-file>index.jsp</welcome-file>

</welcome-file-list>

</web-app>