本软件是基于大学寝室局域网聊天的思路。c#源代码如下:

1 using System; 2 using System.Drawing; 3 using System.Collections; 4 using System.ComponentModel; 5 using System.Windows.Forms; 6 using System.Data; 7 using System.Threading; 8 using System.Net.Sockets; 9 using System.IO; 10 //Download by http://www.codefans.net 11 namespace SimpleChat 12 { 13 /// <summary> 14 /// Form1 的摘要说明。 15 /// </summary> 16 public class MainForm : System.Windows.Forms.Form 17 { 18 /// <summary> 19 /// 必需的设计器变量。 20 /// </summary> 21 private System.ComponentModel.Container components = null; 22 private Thread Listener; 23 private System.Windows.Forms.Label label1; 24 private System.Windows.Forms.RichTextBox richTextBox1; 25 private System.Windows.Forms.Label label2; 26 private System.Windows.Forms.Button button1; 27 private System.Windows.Forms.RichTextBox richTextBox2; 28 private System.Windows.Forms.Label label3; 29 private System.Windows.Forms.GroupBox groupBox1; 30 private System.Windows.Forms.TextBox IP; 31 private System.Windows.Forms.Label label4; 32 private System.Windows.Forms.TextBox NickName; 33 private System.Windows.Forms.GroupBox groupBox2; 34 private System.Windows.Forms.Splitter splitter1; 35 private System.Windows.Forms.GroupBox groupBox3; 36 private System.Windows.Forms.Splitter splitter2; 37 private System.Windows.Forms.Button button2; 38 private TcpListener TcpListener; 39 private Label label5; 40 private ComboBox comboBox1; 41 private Label label6; 42 private ComboBox comboBox2; 43 private Thread sends; 44 45 public MainForm() 46 { 47 // 48 // Windows 窗体设计器支持所必需的 49 // 50 InitializeComponent(); 51 52 // 53 // TODO: 在 InitializeComponent 调用后添加任何构造函数代码 54 // 55 } 56 57 /// <summary> 58 /// 清理所有正在使用的资源。 59 /// </summary> 60 protected override void Dispose( bool disposing ) 61 { 62 if( disposing ) 63 { 64 if (components != null) 65 { 66 components.Dispose(); 67 } 68 } 69 base.Dispose( disposing ); 70 } 71 72 #region Windows 窗体设计器生成的代码 73 /// <summary> 74 /// 设计器支持所需的方法 - 不要使用代码编辑器修改 75 /// 此方法的内容。 76 /// </summary> 77 private void InitializeComponent() 78 { 79 System.ComponentModel.ComponentResourceManager resources = new System.ComponentModel.ComponentResourceManager(typeof(MainForm)); 80 this.label1 = new System.Windows.Forms.Label(); 81 this.richTextBox1 = new System.Windows.Forms.RichTextBox(); 82 this.label2 = new System.Windows.Forms.Label(); 83 this.button1 = new System.Windows.Forms.Button(); 84 this.richTextBox2 = new System.Windows.Forms.RichTextBox(); 85 this.label3 = new System.Windows.Forms.Label(); 86 this.groupBox1 = new System.Windows.Forms.GroupBox(); 87 this.NickName = new System.Windows.Forms.TextBox(); 88 this.label4 = new System.Windows.Forms.Label(); 89 this.IP = new System.Windows.Forms.TextBox(); 90 this.groupBox2 = new System.Windows.Forms.GroupBox(); 91 this.splitter1 = new System.Windows.Forms.Splitter(); 92 this.groupBox3 = new System.Windows.Forms.GroupBox(); 93 this.label5 = new System.Windows.Forms.Label(); 94 this.comboBox1 = new System.Windows.Forms.ComboBox(); 95 this.splitter2 = new System.Windows.Forms.Splitter(); 96 this.button2 = new System.Windows.Forms.Button(); 97 this.label6 = new System.Windows.Forms.Label(); 98 this.comboBox2 = new System.Windows.Forms.ComboBox(); 99 this.groupBox1.SuspendLayout(); 100 this.groupBox2.SuspendLayout(); 101 this.groupBox3.SuspendLayout(); 102 this.SuspendLayout(); 103 // 104 // label1 105 // 106 this.label1.Location = new System.Drawing.Point(8, 17); 107 this.label1.Name = "label1"; 108 this.label1.Size = new System.Drawing.Size(80, 15); 109 this.label1.TabIndex = 0; 110 this.label1.Text = "对方IP地址:"; 111 // 112 // richTextBox1 113 // 114 this.richTextBox1.BackColor = System.Drawing.SystemColors.HighlightText; 115 this.richTextBox1.Dock = System.Windows.Forms.DockStyle.Fill; 116 this.richTextBox1.ForeColor = System.Drawing.Color.FromArgb(((int)(((byte)(0)))), ((int)(((byte)(192)))), ((int)(((byte)(0))))); 117 this.richTextBox1.Location = new System.Drawing.Point(3, 39); 118 this.richTextBox1.Name = "richTextBox1"; 119 this.richTextBox1.Size = new System.Drawing.Size(478, 110); 120 this.richTextBox1.TabIndex = 1; 121 this.richTextBox1.Text = ""; 122 // 123 // label2 124 // 125 this.label2.Dock = System.Windows.Forms.DockStyle.Top; 126 this.label2.Location = new System.Drawing.Point(3, 17); 127 this.label2.Name = "label2"; 128 this.label2.Size = new System.Drawing.Size(478, 19); 129 this.label2.TabIndex = 2; 130 this.label2.Text = "输入框:"; 131 // 132 // button1 133 // 134 this.button1.Location = new System.Drawing.Point(0, 352); 135 this.button1.Name = "button1"; 136 this.button1.Size = new System.Drawing.Size(75, 23); 137 this.button1.TabIndex = 3; 138 this.button1.Text = "发送"; 139 this.button1.Click += new System.EventHandler(this.button1_Click); 140 // 141 // richTextBox2 142 // 143 this.richTextBox2.BackColor = System.Drawing.SystemColors.HighlightText; 144 this.richTextBox2.Dock = System.Windows.Forms.DockStyle.Fill; 145 this.richTextBox2.ForeColor = System.Drawing.Color.FromArgb(((int)(((byte)(192)))), ((int)(((byte)(0)))), ((int)(((byte)(192))))); 146 this.richTextBox2.Location = new System.Drawing.Point(3, 36); 147 this.richTextBox2.Name = "richTextBox2"; 148 this.richTextBox2.Size = new System.Drawing.Size(478, 121); 149 this.richTextBox2.TabIndex = 4; 150 this.richTextBox2.Text = ""; 151 // 152 // label3 153 // 154 this.label3.Dock = System.Windows.Forms.DockStyle.Top; 155 this.label3.Location = new System.Drawing.Point(3, 17); 156 this.label3.Name = "label3"; 157 this.label3.Size = new System.Drawing.Size(478, 16); 158 this.label3.TabIndex = 5; 159 this.label3.Text = "聊天信息:"; 160 // 161 // groupBox1 162 // 163 this.groupBox1.Controls.Add(this.NickName); 164 this.groupBox1.Controls.Add(this.label4); 165 this.groupBox1.Controls.Add(this.IP); 166 this.groupBox1.Controls.Add(this.label1); 167 this.groupBox1.Dock = System.Windows.Forms.DockStyle.Top; 168 this.groupBox1.Location = new System.Drawing.Point(0, 0); 169 this.groupBox1.Name = "groupBox1"; 170 this.groupBox1.Size = new System.Drawing.Size(484, 40); 171 this.groupBox1.TabIndex = 6; 172 this.groupBox1.TabStop = false; 173 // 174 // NickName 175 // 176 this.NickName.Location = new System.Drawing.Point(308, 13); 177 this.NickName.Name = "NickName"; 178 this.NickName.Size = new System.Drawing.Size(110, 21); 179 this.NickName.TabIndex = 3; 180 // 181 // label4 182 // 183 this.label4.Location = new System.Drawing.Point(247, 16); 184 this.label4.Name = "label4"; 185 this.label4.Size = new System.Drawing.Size(70, 18); 186 this.label4.TabIndex = 2; 187 this.label4.Text = "你的昵称:"; 188 // 189 // IP 190 // 191 this.IP.Location = new System.Drawing.Point(85, 14); 192 this.IP.Name = "IP"; 193 this.IP.Size = new System.Drawing.Size(100, 21); 194 this.IP.TabIndex = 1; 195 // 196 // groupBox2 197 // 198 this.groupBox2.Controls.Add(this.richTextBox2); 199 this.groupBox2.Controls.Add(this.splitter1); 200 this.groupBox2.Controls.Add(this.label3); 201 this.groupBox2.Dock = System.Windows.Forms.DockStyle.Top; 202 this.groupBox2.Location = new System.Drawing.Point(0, 40); 203 this.groupBox2.Name = "groupBox2"; 204 this.groupBox2.Size = new System.Drawing.Size(484, 160); 205 this.groupBox2.TabIndex = 7; 206 this.groupBox2.TabStop = false; 207 // 208 // splitter1 209 // 210 this.splitter1.Dock = System.Windows.Forms.DockStyle.Top; 211 this.splitter1.Location = new System.Drawing.Point(3, 33); 212 this.splitter1.Name = "splitter1"; 213 this.splitter1.Size = new System.Drawing.Size(478, 3); 214 this.splitter1.TabIndex = 6; 215 this.splitter1.TabStop = false; 216 // 217 // groupBox3 218 // 219 this.groupBox3.Controls.Add(this.label5); 220 this.groupBox3.Controls.Add(this.comboBox1); 221 this.groupBox3.Controls.Add(this.richTextBox1); 222 this.groupBox3.Controls.Add(this.splitter2); 223 this.groupBox3.Controls.Add(this.label2); 224 this.groupBox3.Dock = System.Windows.Forms.DockStyle.Top; 225 this.groupBox3.Location = new System.Drawing.Point(0, 200); 226 this.groupBox3.Name = "groupBox3"; 227 this.groupBox3.Size = new System.Drawing.Size(484, 152); 228 this.groupBox3.TabIndex = 8; 229 this.groupBox3.TabStop = false; 230 // 231 // label5 232 // 233 this.label5.AutoSize = true; 234 this.label5.Location = new System.Drawing.Point(139, 17); 235 this.label5.Name = "label5"; 236 this.label5.Size = new System.Drawing.Size(65, 12); 237 this.label5.TabIndex = 5; 238 this.label5.Text = "常用短语:"; 239 // 240 // comboBox1 241 // 242 this.comboBox1.BackColor = System.Drawing.Color.FromArgb(((int)(((byte)(255)))), ((int)(((byte)(255)))), ((int)(((byte)(192))))); 243 this.comboBox1.Font = new System.Drawing.Font("华文新魏", 8.999999F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((byte)(134))); 244 this.comboBox1.ForeColor = System.Drawing.Color.FromArgb(((int)(((byte)(192)))), ((int)(((byte)(0)))), ((int)(((byte)(192))))); 245 this.comboBox1.FormattingEnabled = true; 246 this.comboBox1.Items.AddRange(new object[] { 247 "你好,逗比 >=<", 248 "我在忙,等会回复你。", 249 "额心情不好,表惹我!!!", 250 "F-U-C-K !", 251 "贱人,且行且珍惜。。。。", 252 "因为你长的太丑,系统拒绝发送你的信息^-^", 253 "不是我不说话,我一说话就把我的智商暴露了。~(*^__^*) 嘻嘻…"}); 254 this.comboBox1.Location = new System.Drawing.Point(222, 15); 255 this.comboBox1.Name = "comboBox1"; 256 this.comboBox1.Size = new System.Drawing.Size(211, 20); 257 this.comboBox1.TabIndex = 4; 258 this.comboBox1.SelectedIndexChanged += new System.EventHandler(this.comboBox1_SelectedIndexChanged); 259 // 260 // splitter2 261 // 262 this.splitter2.Dock = System.Windows.Forms.DockStyle.Top; 263 this.splitter2.Location = new System.Drawing.Point(3, 36); 264 this.splitter2.Name = "splitter2"; 265 this.splitter2.Size = new System.Drawing.Size(478, 3); 266 this.splitter2.TabIndex = 3; 267 this.splitter2.TabStop = false; 268 // 269 // button2 270 // 271 this.button2.Location = new System.Drawing.Point(397, 354); 272 this.button2.Name = "button2"; 273 this.button2.Size = new System.Drawing.Size(75, 23); 274 this.button2.TabIndex = 9; 275 this.button2.Text = "关于"; 276 this.button2.Click += new System.EventHandler(this.button2_Click); 277 // 278 // label6 279 // 280 this.label6.AutoSize = true; 281 this.label6.Location = new System.Drawing.Point(136, 357); 282 this.label6.Name = "label6"; 283 this.label6.Size = new System.Drawing.Size(65, 12); 284 this.label6.TabIndex = 10; 285 this.label6.Text = "常用表情:"; 286 // 287 // comboBox2 288 // 289 this.comboBox2.BackColor = System.Drawing.Color.FromArgb(((int)(((byte)(255)))), ((int)(((byte)(224)))), ((int)(((byte)(192))))); 290 this.comboBox2.ForeColor = System.Drawing.Color.FromArgb(((int)(((byte)(192)))), ((int)(((byte)(0)))), ((int)(((byte)(192))))); 291 this.comboBox2.FormattingEnabled = true; 292 this.comboBox2.Items.AddRange(new object[] { 293 "\^o^/ 欢呼", 294 "", 295 "*^?^* 笑,打", 296 "", 297 "::>_<:: 哭 ", 298 "", 299 "@x@ 生气", 300 "", 301 "( ^_^ )? 什麼意思??", 302 "", 303 "O_o 惊讶~~~", 304 "", 305 "^◎- 爱你呦~~", 306 "", 307 "得意 <( ̄︶ ̄)>", 308 "", 309 " 乾杯 []~( ̄▽ ̄)~*", 310 "", 311 "滿足 ( ̄ˇ ̄) ", 312 "", 313 "沒睡醒 ( ̄﹏ ̄) ", 314 "", 315 "狡猾(‵﹏′) ", 316 "", 317 "被打一巴掌 ( ̄ε(# ̄)", 318 " ", 319 "無言 ( ̄. ̄) ", 320 "", 321 "無奈 ╮( ̄▽ ̄)╭ ", 322 "", 323 "裝傻 ( ̄▽ ̄)~* ", 324 "", 325 "驚訝 (⊙ˍ⊙)", 326 "", 327 "發現( ̄. ̄)+ ", 328 " ", 329 "驚嚇 Σ( ° △ °|||)︴ ", 330 "", 331 "冷 ( ̄▽ ̄)" ", 332 "", 333 "沒辦法 ╮(╯▽╰)╭ ", 334 "", 335 "貓咪臉 (= ̄ω ̄=) ", 336 "", 337 "疑惑 ( ̄3 ̄)a ", 338 "", 339 "阿達 ( ̄0  ̄)y ", 340 "", 341 "重創 (。_。) ", 342 "", 343 "不 (>﹏<) ", 344 "", 345 "懷疑 (→_→) ", 346 "", 347 "睏 ( ̄o ̄) . z Z ", 348 "", 349 "崇拜 m( _ _ )m ", 350 "", 351 "我想想 (ˇˍˇ) ", 352 "", 353 "生氣 <( ̄ ﹌  ̄)> "}); 354 this.comboBox2.Location = new System.Drawing.Point(207, 354); 355 this.comboBox2.Name = "comboBox2"; 356 this.comboBox2.Size = new System.Drawing.Size(125, 20); 357 this.comboBox2.TabIndex = 11; 358 this.comboBox2.SelectedIndexChanged += new System.EventHandler(this.comboBox2_SelectedIndexChanged); 359 // 360 // MainForm 361 // 362 this.AutoScaleBaseSize = new System.Drawing.Size(6, 14); 363 this.BackColor = System.Drawing.Color.FromArgb(((int)(((byte)(192)))), ((int)(((byte)(192)))), ((int)(((byte)(0))))); 364 this.ClientSize = new System.Drawing.Size(484, 377); 365 this.Controls.Add(this.comboBox2); 366 this.Controls.Add(this.label6); 367 this.Controls.Add(this.button2); 368 this.Controls.Add(this.groupBox3); 369 this.Controls.Add(this.groupBox2); 370 this.Controls.Add(this.groupBox1); 371 this.Controls.Add(this.button1); 372 this.Icon = ((System.Drawing.Icon)(resources.GetObject("$this.Icon"))); 373 this.MaximumSize = new System.Drawing.Size(500, 416); 374 this.Name = "MainForm"; 375 this.RightToLeftLayout = true; 376 this.Text = "HPU-计算机11-4 局域网聊天"; 377 this.Closed += new System.EventHandler(this.MainForm_Closed); 378 this.Load += new System.EventHandler(this.MainForm_Load); 379 this.groupBox1.ResumeLayout(false); 380 this.groupBox1.PerformLayout(); 381 this.groupBox2.ResumeLayout(false); 382 this.groupBox3.ResumeLayout(false); 383 this.groupBox3.PerformLayout(); 384 this.ResumeLayout(false); 385 this.PerformLayout(); 386 387 } 388 #endregion 389 390 /// <summary> 391 /// 应用程序的主入口点。 392 /// </summary> 393 [STAThread] 394 static void Main() 395 { 396 Application.Run(new MainForm()); 397 } 398 private void StartListen() 399 { 400 this.TcpListener = new TcpListener(19808); 401 this.TcpListener.Start(); 402 while( true ) 403 { 404 TcpClient TcpClient = this.TcpListener.AcceptTcpClient(); 405 NetworkStream MyStream = TcpClient.GetStream(); 406 byte [] bytes = new byte[2048]; 407 int bytesRead = MyStream.Read(bytes,0,bytes.Length); 408 string message = System.Text.Encoding.UTF8.GetString(bytes,0,bytesRead); 409 this.richTextBox2.Text += message + " "; 410 } 411 } 412 413 private void MainForm_Load(object sender, System.EventArgs e) 414 { 415 this.Listener = new Thread(new ThreadStart(StartListen)); 416 this.Listener.Start(); 417 } 418 419 private void MainForm_Closed(object sender, System.EventArgs e) 420 { 421 if ( this.Listener != null ) 422 this.Listener.Abort(); 423 if ( this.TcpListener != null ) 424 this.TcpListener.Stop(); 425 } 426 427 private void button1_Click(object sender, System.EventArgs e) 428 { 429 if ( sends == null || !sends.IsAlive) 430 { 431 sends = new Thread(new ThreadStart(send)); 432 sends.Start(); 433 } 434 else 435 MessageBox.Show("发送过快!","提示",MessageBoxButtons.OK,MessageBoxIcon.Information); 436 } 437 438 private void button2_Click(object sender, System.EventArgs e) 439 { 440 MessageBox.Show("制作:HPU 计算机11-4 魏春生 输入对方IP地址,只要双方都打开本程序即可聊天... HpuChat v1.0"); 441 } 442 443 private void send() 444 { 445 if (this.IP.Text.Length < 7 ) 446 { 447 MessageBox.Show("IP地址错误!","错误信息:",MessageBoxButtons.OK,MessageBoxIcon.Information); 448 return; 449 } 450 if ( this.richTextBox1.Text.Length < 1 ) 451 { 452 return; 453 } 454 try 455 { 456 string Message = this.NickName.Text + ":" + " " + DateTime.Now.ToString() + " " + " " + this.richTextBox1.Text + " "; 457 TcpClient TcpClient = new TcpClient(this.IP.Text, 19808); 458 NetworkStream tcpStream = TcpClient.GetStream(); 459 StreamWriter stream = new StreamWriter(tcpStream); 460 stream.Flush(); 461 stream.Write(Message); 462 stream.Close(); 463 TcpClient.Close(); 464 this.richTextBox2.AppendText( Message + " "); 465 richTextBox1.Text = string.Empty; 466 } 467 catch ( Exception Err) 468 { 469 MessageBox.Show(Err.Message,"错误信息:",MessageBoxButtons.OK,MessageBoxIcon.Information); 470 } 471 finally 472 { 473 sends.Abort(); 474 } 475 476 } 477 478 private void comboBox1_SelectedIndexChanged(object sender, EventArgs e) 479 { 480 this.richTextBox1.Text = comboBox1.SelectedItem.ToString(); 481 } 482 483 private void comboBox2_SelectedIndexChanged(object sender, EventArgs e) 484 { 485 this.richTextBox1.Text = comboBox2.SelectedItem.ToString(); 486 } 487 } 488 }
功能比较简单。只能文本聊天,没有表情。有待改善。
软件截图::::::
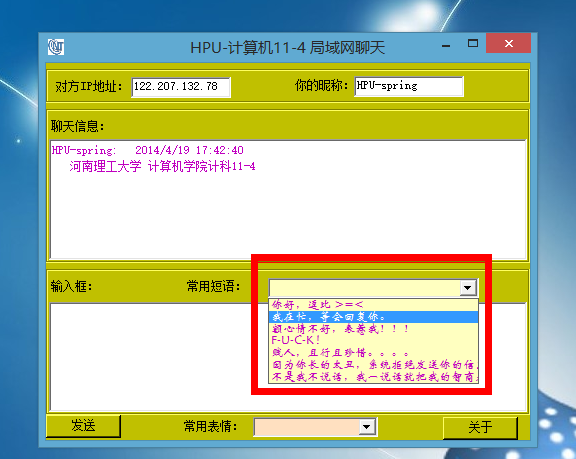
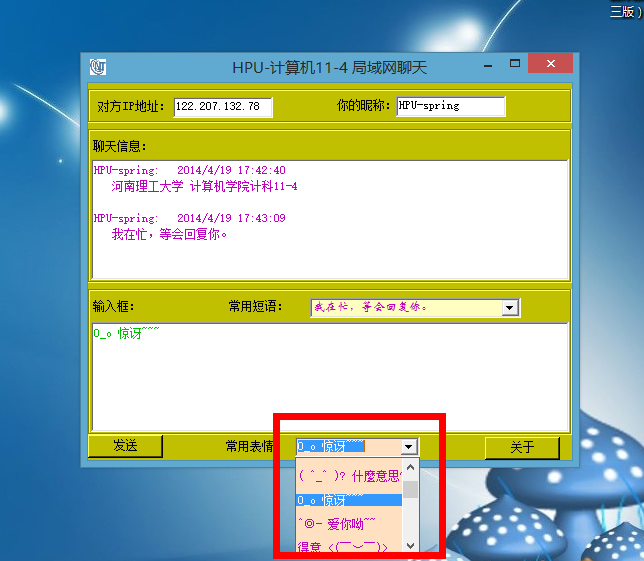
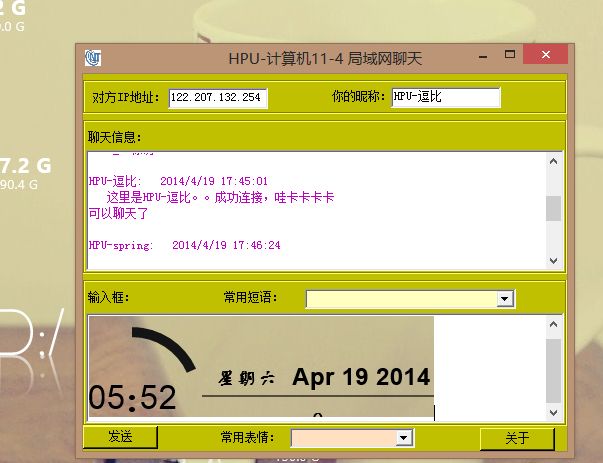
使用说明书地址::