1 using System;
2 using System.Collections.Generic;
3 using System.Linq;
4 using System.Text;
5 using System.IO;
6
7 namespace ConsoleApplication3
8 {
9 class Program
10 {
11 static void Main(string[] args)
12 {
13 var Items = new List<SortItem>();
14
15 Items.AddRange(new SortItem[] {
16 new SortItem(){ GroupId = 1, Id=1, Count = 1, Weight = 100 },
17 new SortItem(){ GroupId = 1, Id=1, Count = 2, Weight = null },
18 new SortItem(){ GroupId = 1, Id =2, Count = 3, Weight = 300 },
19 new SortItem(){ GroupId = 2, Id=1, Count = 2, Weight = 200 },
20 new SortItem(){ GroupId = 2, Id=2, Count = 2, Weight = 200 },
21 new SortItem(){ GroupId = 2, Id=3, Count = 4, Weight = null },
22 new SortItem(){ GroupId = 3, Id=3 ,Count = 2, Weight = null },
23 new SortItem(){ GroupId = 1, Id =2, Count = 6, Weight = 300 },
24 });
25
26 var data = (from a in Items
27 group a by new { a.GroupId, a.Id } into b
28 orderby new ComparerItem() { GroupId = b.Key.GroupId, Id = b.Key.Id } descending
29 select new
30 {
31 GroupId = b.Key.GroupId,
32 Id = b.Key.Id,
33 Count = b.Sum(c => c.Count),
34 Weight = b.Sum(c => c.Weight)
35 }).OrderBy(t => t.GroupId).ThenBy(t => t.Id);
36
37
38 foreach (var anItem in data)
39 Console.WriteLine("GroupId:{0} Id:{1} Count:{2} Weight:{3}", anItem.GroupId, anItem.Id, anItem.Count, anItem.Weight);
40
41 Console.ReadKey();
42 }
43
44 class ComparerItem : IComparable
45 {
46 public int GroupId { get; set; }
47 public int Id { get; set; }
48
49 public int CompareTo(object obj)
50 {
51 var tem = (ComparerItem)obj;
52
53 if (this.GroupId == tem.GroupId)
54 {
55 if (this.Id > tem.Id) return 1;
56 else if (this.Id < tem.Id) return -1;
57
58 return 0;
59 }
60 else if (this.GroupId > tem.GroupId)
61 {
62 return 1;
63 }
64
65 return -1;
66 }
67 }
68
69
70 class SortItem
71 {
72 public int GroupId { get; set; }
73 public int Id { get; set; }
74 public int Count { get; set; }
75 public int? Weight { get; set; }
76 }
77 }
78 }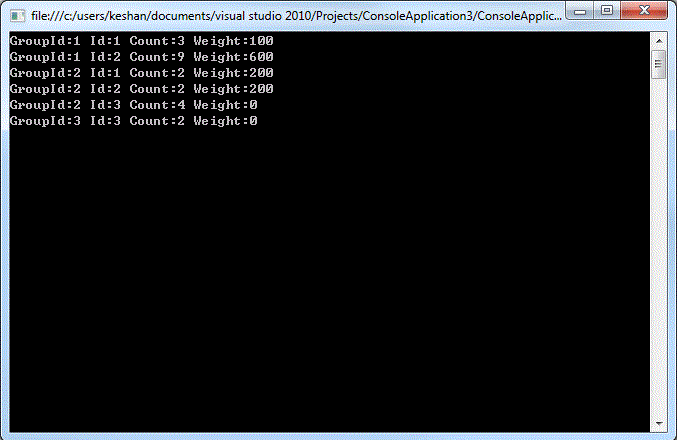
2 using System.Collections.Generic;
3 using System.Linq;
4 using System.Text;
5 using System.IO;
6
7 namespace ConsoleApplication3
8 {
9 class Program
10 {
11 static void Main(string[] args)
12 {
13 var Items = new List<SortItem>();
14
15 Items.AddRange(new SortItem[] {
16 new SortItem(){ GroupId = 1, Id=1, Count = 1, Weight = 100 },
17 new SortItem(){ GroupId = 1, Id=1, Count = 2, Weight = null },
18 new SortItem(){ GroupId = 1, Id =2, Count = 3, Weight = 300 },
19 new SortItem(){ GroupId = 2, Id=1, Count = 2, Weight = 200 },
20 new SortItem(){ GroupId = 2, Id=2, Count = 2, Weight = 200 },
21 new SortItem(){ GroupId = 2, Id=3, Count = 4, Weight = null },
22 new SortItem(){ GroupId = 3, Id=3 ,Count = 2, Weight = null },
23 new SortItem(){ GroupId = 1, Id =2, Count = 6, Weight = 300 },
24 });
25
26 var data = (from a in Items
27 group a by new { a.GroupId, a.Id } into b
28 orderby new ComparerItem() { GroupId = b.Key.GroupId, Id = b.Key.Id } descending
29 select new
30 {
31 GroupId = b.Key.GroupId,
32 Id = b.Key.Id,
33 Count = b.Sum(c => c.Count),
34 Weight = b.Sum(c => c.Weight)
35 }).OrderBy(t => t.GroupId).ThenBy(t => t.Id);
36
37
38 foreach (var anItem in data)
39 Console.WriteLine("GroupId:{0} Id:{1} Count:{2} Weight:{3}", anItem.GroupId, anItem.Id, anItem.Count, anItem.Weight);
40
41 Console.ReadKey();
42 }
43
44 class ComparerItem : IComparable
45 {
46 public int GroupId { get; set; }
47 public int Id { get; set; }
48
49 public int CompareTo(object obj)
50 {
51 var tem = (ComparerItem)obj;
52
53 if (this.GroupId == tem.GroupId)
54 {
55 if (this.Id > tem.Id) return 1;
56 else if (this.Id < tem.Id) return -1;
57
58 return 0;
59 }
60 else if (this.GroupId > tem.GroupId)
61 {
62 return 1;
63 }
64
65 return -1;
66 }
67 }
68
69
70 class SortItem
71 {
72 public int GroupId { get; set; }
73 public int Id { get; set; }
74 public int Count { get; set; }
75 public int? Weight { get; set; }
76 }
77 }
78 }
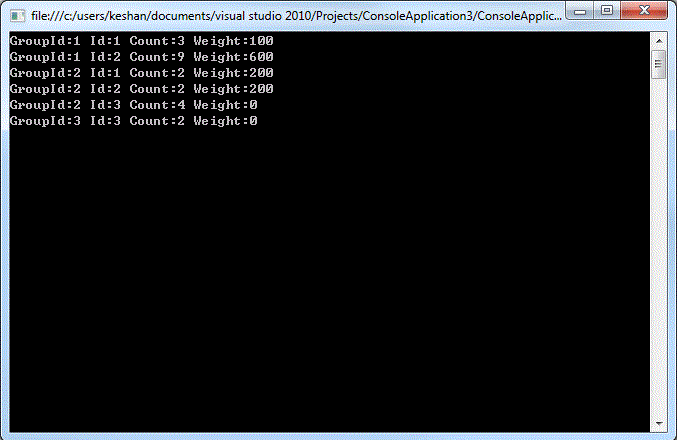