自己写练手项目的时候常常会遇到一个问题,没有后台接口,获取数据总是很麻烦,于是在网上找了下,发现一个挺好用的模拟后台接口数据的工具:mockjs.现在把自己在项目中使用的方法贴出来
先看下项目的目录,这是用vue-cli生成的一个vue项目,主要是需要配置axios和写接口数据mock.js
首先需要安装axios和mockjs
npm i axios mockjs --save
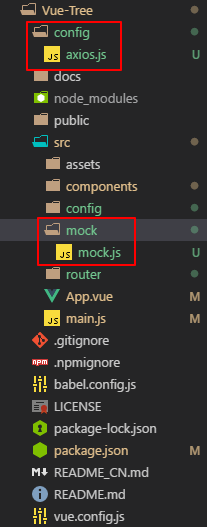
在项目中新建一个config目录,与src同级,配置axios
./config/axios.js
import axios from 'axios' axios.defaults.headers.post['Content-Type'] = 'application/x-www-form-urlencoded' // 请求拦截器 axios.interceptors.request.use(function(config) { return config }, function(error) { return Promise.reject(error) }) // 响应拦截器 axios.interceptors.response.use(function(response) { return response }, function(error) { return Promise.reject(error) })
在src文件中新建一个mock目录.定义接口数据
./src/mock/mock.js
mockjs的文档提供了很多生成随机数据的字段,使用Random关键字+字段方法名就可以,具体需要什么数据可以查看官网文档http://mockjs.com/examples.html#Random.zip()
当然你也可以完全自己写数据
import Mock from 'mockjs' const Random = Mock.Random // mock需要给三个参数,url(与axios请求是传的url一致,我这个是本地启动的项目就直接用本地域名了) // 请求类型: get post...其他看文档 // 数据处理函数,函数需要return数据 Mock.mock('http://localhost:8081/test/city', 'get', () => { let citys = [] for (let i = 0; i < 10; i++) { let obj = { id: i+1, city: Random.city(), color: Random.color() } citys.push(obj) } return {cityList: citys} }) // post请求,带参数,参数会在data中返回,会返回url,type,body三个参数,可以把data打印出来看看 Mock.mock('http://localhost:8081/test/cityInfo', 'post', (data) => { // 请求传过来的参数在body中,传回的是json字符串,需要转义一下 const info= JSON.parse(data.body) return { img: Random.image('200x100', '#4A7BF7', info.name) } })
在main.js文件中引入mock和axios
./main.js
import Vue from 'vue' import App from './App.vue' import VueRouter from 'vue-router' import routes from './router' import './mock/mock.js' import axios from 'axios' import '../config/axios' // 将axios挂载到Vue实例,在组件中可以直接使用 Vue.prototype.$axios = axios Vue.config.productionTip = false Vue.use(VueRouter) const router = new VueRouter({ routes, strict: process.env.NODE_ENV !== 'production' }) new Vue({ router, render: h => h(App) }).$mount('#app')
在vue组件中调用接口渲染页面
<template> <div> test <button @click="clickMe">获取城市</button> <ul class="city_container"> <li class="city_item" v-for="item in cityList" :key="item.id" @click="getCityInfo(item.city)"> <a href="javascript:void(0)" :style="{color: item.color}">{{item.city}}</a> </li> </ul> <img :src="img" alt=""> </div> </template> <script> export default { name: 'test', components: { }, data() { return { cityList: [], img: '' } }, methods: { clickMe () {
// 这里请求的地址要和mock中定义的请求地址一致 this.$axios.get('http://localhost:8081/test/city').then(res => { console.log(77, res) if (res.data) { this.cityList = res.data.cityList } }) }, getCityInfo (name) { this.$axios.post('http://localhost:8081/test/cityInfo', { name: name }).then(res => { console.log(88, res) if (res.data) { this.img = res.data.img } }) } } } </script> <style scoped> .city_item { list-style: none; float: left; border: 1px solid orange; auto; height: 50px; line-height: 50px; padding: 0 5px; border-right: none; } .city_container :last-of-type { border-right: 1px solid orange; } .city_container .city_item a { text-decoration: none; border: none; } </style>
页面效果:
获取随机城市
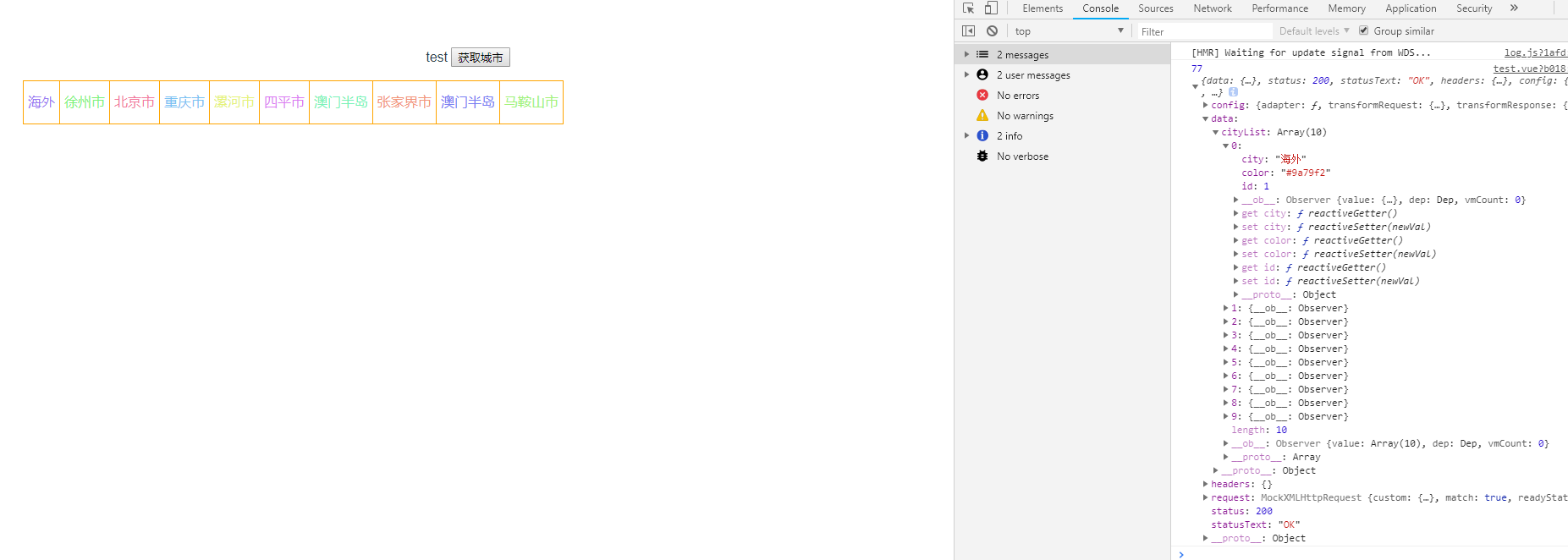
点击城市获取图片
