Going Home
Time Limit: 10000/5000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Others)
Total Submission(s): 6478 Accepted Submission(s): 3411
Problem Description
On a grid map there are n little men and n houses. In each unit time, every little man can move one unit step, either horizontally, or vertically, to an adjacent point. For each little man, you need to pay a $1 travel fee for every step he moves, until he enters a house. The task is complicated with the restriction that each house can accommodate only one little man.
Your task is to compute the minimum amount of money you need to pay in order to send these n little men into those n different houses. The input is a map of the scenario, a '.' means an empty space, an 'H' represents a house on that point, and am 'm' indicates there is a little man on that point.
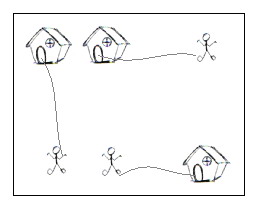
You can think of each point on the grid map as a quite large square, so it can hold n little men at the same time; also, it is okay if a little man steps on a grid with a house without entering that house.
Your task is to compute the minimum amount of money you need to pay in order to send these n little men into those n different houses. The input is a map of the scenario, a '.' means an empty space, an 'H' represents a house on that point, and am 'm' indicates there is a little man on that point.
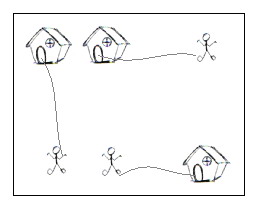
You can think of each point on the grid map as a quite large square, so it can hold n little men at the same time; also, it is okay if a little man steps on a grid with a house without entering that house.
Input
There are one or more test cases in the input. Each case starts with a line giving two integers N and M, where N is the number of rows of the map, and M is the number of columns. The rest of the input will be N lines describing the map. You may assume both N and M are between 2 and 100, inclusive. There will be the same number of 'H's and 'm's on the map; and there will be at most 100 houses. Input will terminate with 0 0 for N and M.
Output
For each test case, output one line with the single integer, which is the minimum amount, in dollars, you need to pay.
Sample Input
2 2
.m
H.
5 5
HH..m
.....
.....
.....
mm..H
7 8
...H....
...H....
...H....
mmmHmmmm
...H....
...H....
...H....
0 0
Sample Output
2
10
28
题意 n个人 n个房子 在N*M个方格 人移动一格要花费 1(只能水平竖直四个方向) 问n个人走到n个房子的最小花费是多少(一个房子只能一个人待)
解析 这道题可以转化成费用流来解决,n个源点n个汇点 最大流为n的最小费用 ,我们直接建立一个超源点0,一个超汇点n*m+1 然后和源点汇点相连 容量1 费用0
注意 其他边的费用为1 但是容量要设为inf 因为走过之后还可以走.
也可以用二分图最大全匹配写 还没get这项技能。。。
代码一 // 一比二快100ms。
1 #include<bits/stdc++.h> 2 using namespace std; 3 const int maxn=1e4+20,mod=1e9+7,inf=0x3f3f3f3f; 4 typedef long long ll; 5 #define pb push_back 6 #define mp make_pair 7 #define X first 8 #define Y second 9 #define all(a) (a).begin(), (a).end() 10 #define fillchar(a, x) memset(a, x, sizeof(a)) 11 #define huan printf(" "); 12 #define debug(a,b) cout<<a<<" "<<b<<" "; 13 int dir[4][2]={{1,0},{-1,0},{0,-1},{0,1}}; 14 char a[maxn][maxn]; 15 struct MCMF { 16 struct Edge { 17 int from, to, cap, cost; 18 Edge(int u, int v, int w, int c): from(u), to(v), cap(w), cost(c) {} 19 }; 20 int n, s, t; 21 vector<Edge> edges; 22 vector<int> G[maxn]; 23 int inq[maxn], d[maxn], p[maxn], a[maxn]; 24 25 void init(int n) { 26 this->n = n; 27 for (int i = 0; i <= n; i ++) G[i].clear(); 28 edges.clear(); 29 } 30 void addedge(int from, int to, int cap, int cost) { 31 edges.push_back(Edge(from, to, cap, cost)); 32 edges.push_back(Edge(to, from, 0, -cost)); 33 int m = edges.size(); 34 G[from].push_back(m - 2); 35 G[to].push_back(m - 1); 36 } 37 bool BellmanFord(int s, int t, int &flow, int &cost) { 38 for (int i = 0; i <= n; i ++) d[i] = inf; 39 memset(inq, 0, sizeof(inq)); 40 d[s] = 0; inq[s] = 1; p[s] = 0; a[s] = inf; 41 42 queue<int> Q; 43 Q.push(s); 44 while (!Q.empty()) { 45 int u = Q.front(); Q.pop(); 46 inq[u] = 0; 47 for (int i = 0; i < G[u].size(); i ++) { 48 Edge &e = edges[G[u][i]]; 49 if (e.cap && d[e.to] > d[u] + e.cost) { 50 d[e.to] = d[u] + e.cost; 51 p[e.to] = G[u][i]; 52 a[e.to] = min(a[u], e.cap); 53 if (!inq[e.to]) { 54 Q.push(e.to); 55 inq[e.to] = 1; 56 } 57 } 58 } 59 } 60 if (d[t] == inf) return false; 61 flow += a[t]; 62 cost += d[t] * a[t]; 63 int u = t; 64 while (u != s) { 65 edges[p[u]].cap -= a[t]; 66 edges[p[u] ^ 1].cap += a[t]; 67 u = edges[p[u]].from; 68 } 69 return true; 70 } 71 int solve(int s, int t) { 72 int flow = 0, cost = 0; 73 while (BellmanFord(s, t, flow, cost)); 74 return cost; 75 } 76 }solver;; 77 void build(int n,int m) 78 { 79 vector<int> ss,tt; 80 for(int i=1;i<=n;i++) 81 { 82 scanf("%s",a[i]+1); 83 } 84 for(int i=1;i<=n;i++) 85 { 86 for(int j=1;j<=m;j++) 87 { 88 int temp=(i-1)*m+j; 89 if(a[i][j]=='m') 90 ss.push_back(temp); 91 else if(a[i][j]=='H') 92 tt.push_back(temp); 93 for(int k=0;k<4;k++) 94 { 95 int x=i+dir[k][0]; 96 int y=j+dir[k][1]; 97 if(x>=1&&x<=n&&y>=1&&y<=m) 98 { 99 int temp2=(x-1)*m+y; 100 solver.addedge(temp,temp2,inf,1); 101 // cout<<i<<" "<<j<<" "<<temp<<" "<<temp2<<endl; 102 } 103 } 104 } 105 } 106 for(int i=0;i<ss.size();i++) 107 solver.addedge(0,ss[i],1,0); 108 for(int i=0;i<tt.size();i++) 109 solver.addedge(tt[i],n*m+1,1,0); 110 } 111 int main() 112 { 113 int n,m; 114 while(~scanf("%d%d",&n,&m)&&n&&m) 115 { 116 solver.init(n*m+1); 117 build(n,m); 118 int maxflow; 119 maxflow=solver.solve(0,n*m+1); 120 printf("%d ",maxflow); 121 } 122 }
代码二
1 #include<iostream> 2 #include<stdio.h> 3 #include<string.h> 4 #include<stdlib.h> 5 #include<vector> 6 #include<queue> 7 using namespace std; 8 const int maxn=2e4+20,mod=1e9+7,inf=0x3f3f3f3f; 9 struct edge 10 { 11 int to,next,cap,flow,cost; 12 } edge[maxn*100]; 13 int head[maxn],tol; 14 int pre[maxn],dis[maxn]; 15 bool vis[maxn]; 16 int N; 17 char a[maxn][maxn]; 18 void init(int n) 19 { 20 N=n,tol=0; 21 memset(head,-1,sizeof(head)); 22 } 23 void addedge(int u,int v,int cap,int cost) 24 { 25 edge[tol].to=v; 26 edge[tol].cap=cap; 27 edge[tol].flow=0; 28 edge[tol].cost=cost; 29 edge[tol].next=head[u]; 30 head[u]=tol++; 31 edge[tol].to=u; 32 edge[tol].cap=0; 33 edge[tol].flow=0; 34 edge[tol].cost=-cost; 35 edge[tol].next=head[v]; 36 head[v]=tol++; 37 } 38 bool spfa(int s,int t) 39 { 40 queue<int> q; 41 for(int i=0; i<=N; i++) 42 { 43 dis[i]=inf; 44 vis[i]=false; 45 pre[i]=-1; 46 } 47 dis[s]=0; 48 vis[s]=true; 49 q.push(s); 50 while(!q.empty()) 51 { 52 int u=q.front(); 53 q.pop(); 54 vis[u]=false; 55 for(int i=head[u]; i!=-1; i=edge[i].next) 56 { 57 int v=edge[i].to; 58 if(edge[i].cap>edge[i].flow&&dis[v]>dis[u]+edge[i].cost) 59 { 60 dis[v]=dis[u]+edge[i].cost; 61 pre[v]=i; 62 if(!vis[v]) 63 { 64 vis[v]=true; 65 q.push(v); 66 } 67 } 68 } 69 } 70 if(pre[t]==-1) return false; 71 else return true; 72 } 73 int mincostflow(int s,int t,int &cost) 74 { 75 int flow=0; 76 cost=0; 77 while(spfa(s,t)) 78 { 79 int Min=inf; 80 for(int i=pre[t]; i!=-1; i=pre[edge[i^1].to]) 81 { 82 if(Min>edge[i].cap-edge[i].flow) 83 Min=edge[i].cap-edge[i].flow; 84 } 85 for(int i=pre[t]; i!=-1; i=pre[edge[i^1].to]) 86 { 87 edge[i].flow+=Min; 88 edge[i^1].flow-=Min; 89 cost+=edge[i].cost*Min; 90 } 91 flow+=Min; 92 } 93 return flow; 94 } 95 int dir[4][2]={{1,0},{-1,0},{0,-1},{0,1}}; 96 void build(int n,int m) 97 { 98 vector<int> ss,tt; 99 for(int i=1;i<=n;i++) 100 { 101 scanf("%s",a[i]+1); 102 } 103 for(int i=1;i<=n;i++) 104 { 105 for(int j=1;j<=m;j++) 106 { 107 int temp=(i-1)*m+j; 108 if(a[i][j]=='m') 109 ss.push_back(temp); 110 else if(a[i][j]=='H') 111 tt.push_back(temp); 112 for(int k=0;k<4;k++) 113 { 114 int x=i+dir[k][0]; 115 int y=j+dir[k][1]; 116 if(x>=1&&x<=n&&y>=1&&y<=m) 117 { 118 int temp2=(x-1)*m+y; 119 addedge(temp,temp2,inf,1); 120 // cout<<i<<" "<<j<<" "<<temp<<" "<<temp2<<endl; 121 } 122 } 123 } 124 } 125 for(int i=0;i<ss.size();i++) 126 addedge(0,ss[i],1,0); 127 for(int i=0;i<tt.size();i++) 128 addedge(tt[i],n*m+1,1,0); 129 } 130 int main() 131 { 132 int n,m; 133 while(~scanf("%d%d",&n,&m)&&n&&m) 134 { 135 init(n*m+1); 136 build(n,m); 137 int ans,maxflow; 138 maxflow=mincostflow(0,n*m+1,ans); 139 printf("%d ",ans); 140 } 141 }