1、不加static可以用类名.成员名或者对象名.成员名调用。
1 public class SquareInt { 2 3 public static void main(String[] args) { 4 int result; 5 6 for (int x = 1; x <= 10; x++) { 7 result = square(x); 8 // Math库中也提供了求平方数的方法 9 // result=(int)Math.pow(x,2); 10 System.out.println("The square of " + x + " is " + result + " "); 11 } 12 } 13 14 // 自定义求平方数的静态方法 15 public static int square(int y) { 16 return y * y; 17 } 18 }
2、编写一个方法,使用纯随机数发生器算法生成指定数目(比如1000个)的随机整数。
1 import javax.swing.JOptionPane; 2 3 4 public class Testseed { 5 public static void main( String args[] ) 6 { 7 int value; 8 String output = ""; 9 10 for ( int i = 1; i <= 100; i++ ) { 11 value = 1 + (int) ( Math.random() * 100 ); 12 output += value + " "; 13 14 if ( i % 10== 0 ) 15 output += " "; 16 } 17 18 JOptionPane.showMessageDialog( null, output, 19 "20 Random Numbers from 1 to 6", 20 JOptionPane.INFORMATION_MESSAGE ); 21 22 System.exit( 0 ); 23 } 24 }
3.请看以下代码,你发现了什么特殊之处吗?
1 public class MethodOverload { 2 3 public static void main(String[] args) { 4 System.out.println("The square of integer 7 is " + square(7)); 5 System.out.println(" The square of double 7.5 is " + square(7.5)); 6 } 7 8 public static int square(int x) { 9 return x * x; 10 } 11 12 public static double square(double y) { 13 return y * y; 14 } 15 }
[特殊之处]
自定义了两个方法,int和double型,输出时int 自动调用int型的方法,double调用double型的方法,与自定义方法的顺序无关。
4、使用计算机计算组合数:
1 import java.util.Scanner; 2 public class Zuheshu1 { 3 public static void main(String[]args){ 4 System.out.println("输入组合数的n和k:"); 5 Scanner in1=new Scanner(System.in); 6 int n=in1.nextInt(); 7 Scanner in2=new Scanner(System.in); 8 int k=in2.nextInt(); 9 int result=jiechen(n)/(jiechen(k)*jiechen(n-k)); 10 System.out.println("结果为:"+result); 11 in1.close(); 12 in2.close(); 13 } 14 public static int jiechen(int n) 15 { 16 int jieguo=1; 17 if(n<0) 18 { 19 System.out.println("输入非法!"); 20 } 21 else if(n==0||n==1) 22 { 23 jieguo=1; 24 } 25 else 26 { 27 jieguo=jiechen(n-1)*n; 28 } 29 return jieguo; 30 31 } 32 } 33 递推: 34 package Zuheshu2; 35 36 import java.util.Scanner; 37 38 public class Zuheshu2 { 39 public static void main(String[]args){ 40 System.out.println("输入组合数的n和k:"); 41 Scanner in1=new Scanner(System.in); 42 int n=in1.nextInt(); 43 Scanner in2=new Scanner(System.in); 44 int k=in2.nextInt(); 45 System.out.println("结果为:"+jieguo(n,k)); 46 in1.close(); 47 in2.close(); 48 } 49 public static int jieguo(int n,int m) 50 { 51 if(m==0||n==m) 52 return 1; 53 int s=Math.min(m, n-m); 54 int f=1,f1=0; 55 for(int i=1;i<=s;i++) 56 { 57 f1=f*(n-i+1)/(i); 58 f=f1; 59 } 60 return f1; 61 } 62 } 63 递归: 64 import java.util.Scanner; 65 66 public class Zuheshu2 { 67 public static void main(String[]args){ 68 System.out.println("输入组合数的n和k:"); 69 Scanner in1=new Scanner(System.in); 70 int n=in1.nextInt(); 71 Scanner in2=new Scanner(System.in); 72 int k=in2.nextInt(); 73 System.out.println("结果为:"+jieguo(n,k)); 74 in1.close(); 75 in2.close(); 76 } 77 public static int jieguo(int m,int n) 78 { 79 if(m<0||n<0||m<n) 80 return 0; 81 if(m==n) 82 return 1; 83 if(n==1) 84 return m; 85 return jieguo(m-1,n)+jieguo(m-1,n-1); 86 } 87 }
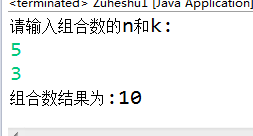
5.用Java实现递归编程解决汉诺塔问题。
1 public class TowersOfHanoi 2 { 3 public static void solveTowers( int disks, int sourcePeg, 4 int destinationPeg, int tempPeg ) 5 { 6 if ( disks == 1 ) 7 { 8 System.out.printf( " %d --> %d", sourcePeg, destinationPeg ); 9 return; 10 } 11 solveTowers( disks - 1, sourcePeg, tempPeg, destinationPeg ); 12 System.out.printf( " %d --> %d", sourcePeg, destinationPeg ); 13 solveTowers( disks - 1, tempPeg, destinationPeg, sourcePeg ); 14 } 15 public static void main( String[] args ) 16 { 17 int startPeg = 1; 18 int endPeg = 3; 19 int tempPeg = 2; 20 int totalDisks = 3; 21 solveTowers( totalDisks, startPeg, endPeg, tempPeg ); 22 } 23 }
6.回文数
1 import java.util.*; 2 public class Palindrome { 3 public static void main(String[]args){ 4 //从键盘上输入一个字符串str 5 String str=""; 6 System.out.println("请输入一个字符串:"); 7 Scanner in=new Scanner(System.in); 8 str=in.nextLine(); 9 //根据字符串创建一个字符缓存类对象sb 10 StringBuffer sb=new StringBuffer(str); 11 //将字符缓存中的内容倒置 12 sb.reverse(); 13 //计算出str与sb中对应位置字符相同的个数n 14 int n=0; 15 for(int i=0;i<str.length();i++){ 16 if(str.charAt(i)==sb.charAt(i)) 17 n++; 18 } 19 //如果所有字符都相等,即n的值等于str的长度,则str就是回文。 20 if(n==str.length()) 21 System.out.println(str+"是回文!"); 22 else 23 System.out.println(str+"不是回文!"); 24 } 25 }
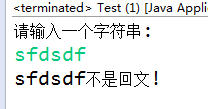
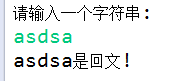