关于界面布局约束的方法有很多,纯代码布局,可以使用官方原生布局(很繁琐)、VFL、Masonary第三方等,在xib或者storyboard中也可以使用Autolayout的界面约束进行布局约束。
我今天要着重记录的是VFL 的布局方式,以前看过相关的博客文档,试着写过,但是因为约束的那串字符串有点复杂也就浅尝辄止,最近要写项目,突然发现VFL布局的奇妙之处,所以在此记录一下VFL的使用。
使用VFL进行约束时你完全不需要对view进行frame设置,所有的布局信息全都融合在一串字符串里面,对于纯代码布局来说,还是一种不错的布局方式。
有点喜欢这种不需要添加frame的约束方式了。
注意点:
/* * *VFL 使用: *非scrollView:约束一个view需要上下左右边界四个条件就可以进行约束了 *scrollView:约束一个scrollView的subview不仅仅需要上下左右四个边界,你还必须对subview的高度和跨度 *进行约束,因为VFL中会根据高度和宽度进行contentsize的自动适配 * */
//VFL 字符串带变量: NSString *toolbarVfV = [NSString stringWithFormat:@"V:[toolbarView(%f)]-(-%f)-|",ePayWapViewToolbarHeight,ePayWapViewToolbarHeight];
本例测试实现:vertical方向布局四个View,同时在其中一个view中实现水平布局(有点类似于前端以及安卓中的先大再小的布局方式)
,进入正题直接上Demo:
/*
*
*vertical方向布局
*
*/
-(void)setupVerticalLayout{
//
UIView *blueView=[[UIView alloc]init];
blueView.backgroundColor=Color_1;
//
UIView *redView=[[UIView alloc]init];
redView.backgroundColor=Color_5;
//
UIView *yellowView=[[UIView alloc]init];
yellowView.backgroundColor=Color_4;
//
UIView *grayView=[[UIView alloc]init];
grayView.backgroundColor=Color_3;
//使用VFL时一定要加上这几句代码:
[blueView setTranslatesAutoresizingMaskIntoConstraints:NO];
[redView setTranslatesAutoresizingMaskIntoConstraints:NO];
[yellowView setTranslatesAutoresizingMaskIntoConstraints:NO];
[grayView setTranslatesAutoresizingMaskIntoConstraints:NO];
[self.view addSubview:blueView];
[self.view addSubview:redView];
[self.view addSubview:yellowView];
[self.view addSubview:grayView];
NSDictionary *views=NSDictionaryOfVariableBindings(blueView,redView,yellowView,grayView);
NSDictionary *metrics=@{@"margin":@10};
//vertical 方向约束
[self.view addConstraints:[NSLayoutConstraint constraintsWithVisualFormat:@"V:|-20-[blueView(==100)]-margin-[redView(==150)]-margin-[yellowView(==100)]-margin-[grayView]-margin-|" options:0 metrics:metrics views:views]];
//blueView 的horizon方向
[self.view addConstraints:[NSLayoutConstraint constraintsWithVisualFormat:@"H:|-margin-[blueView]-margin-|" options:0 metrics:metrics views:views]];
//redView 的horizon方向
[self.view addConstraints:[NSLayoutConstraint constraintsWithVisualFormat:@"H:|-margin-[redView]-margin-|" options:0 metrics:metrics views:views]];
//yellowView 的horizon方向
[self.view addConstraints:[NSLayoutConstraint constraintsWithVisualFormat:@"H:|-margin-[yellowView]-margin-|" options:0 metrics:metrics views:views]];
//grayView 的horizon方向 [self.view addConstraints:[NSLayoutConstraint constraintsWithVisualFormat:@"H:|-margin-[grayView]-margin-|" options:0 metrics:metrics views:views]]; [self setupHorisonalLayout:redView];}
*
*vertical方向布局
*
*/
-(void)setupVerticalLayout{
//
UIView *blueView=[[UIView alloc]init];
blueView.backgroundColor=Color_1;
//
UIView *redView=[[UIView alloc]init];
redView.backgroundColor=Color_5;
//
UIView *yellowView=[[UIView alloc]init];
yellowView.backgroundColor=Color_4;
//
UIView *grayView=[[UIView alloc]init];
grayView.backgroundColor=Color_3;
//使用VFL时一定要加上这几句代码:
[blueView setTranslatesAutoresizingMaskIntoConstraints:NO];
[redView setTranslatesAutoresizingMaskIntoConstraints:NO];
[yellowView setTranslatesAutoresizingMaskIntoConstraints:NO];
[grayView setTranslatesAutoresizingMaskIntoConstraints:NO];
[self.view addSubview:blueView];
[self.view addSubview:redView];
[self.view addSubview:yellowView];
[self.view addSubview:grayView];
NSDictionary *views=NSDictionaryOfVariableBindings(blueView,redView,yellowView,grayView);
NSDictionary *metrics=@{@"margin":@10};
//vertical 方向约束
[self.view addConstraints:[NSLayoutConstraint constraintsWithVisualFormat:@"V:|-20-[blueView(==100)]-margin-[redView(==150)]-margin-[yellowView(==100)]-margin-[grayView]-margin-|" options:0 metrics:metrics views:views]];
//blueView 的horizon方向
[self.view addConstraints:[NSLayoutConstraint constraintsWithVisualFormat:@"H:|-margin-[blueView]-margin-|" options:0 metrics:metrics views:views]];
//redView 的horizon方向
[self.view addConstraints:[NSLayoutConstraint constraintsWithVisualFormat:@"H:|-margin-[redView]-margin-|" options:0 metrics:metrics views:views]];
//yellowView 的horizon方向
[self.view addConstraints:[NSLayoutConstraint constraintsWithVisualFormat:@"H:|-margin-[yellowView]-margin-|" options:0 metrics:metrics views:views]];
//grayView 的horizon方向 [self.view addConstraints:[NSLayoutConstraint constraintsWithVisualFormat:@"H:|-margin-[grayView]-margin-|" options:0 metrics:metrics views:views]]; [self setupHorisonalLayout:redView];}
上面的Demo实现了是个view的vertical方向的布局约束,
下面实现四个horizon方向的view的布局:
/*
*
*horizon方向布局
*
*/
-(void)setupHorisonalLayout:(UIView*)containtView{
//
UIView *blueView=[[UIView alloc]init];
blueView.backgroundColor=Color_1;
//
UIView *redView=[[UIView alloc]init];
redView.backgroundColor=Color_2;
//
UIView *yellowView=[[UIView alloc]init];
yellowView.backgroundColor=Color_3;
//
UIView *grayView=[[UIView alloc]init];
grayView.backgroundColor=Color_4;
[blueView setTranslatesAutoresizingMaskIntoConstraints:NO];
[redView setTranslatesAutoresizingMaskIntoConstraints:NO];
[yellowView setTranslatesAutoresizingMaskIntoConstraints:NO];
[grayView setTranslatesAutoresizingMaskIntoConstraints:NO];
[containtView addSubview:blueView];
[containtView addSubview:redView];
[containtView addSubview:yellowView];
[containtView addSubview:grayView];
NSDictionary *views=NSDictionaryOfVariableBindings(blueView,redView,yellowView,grayView);
NSDictionary *metrics=@{@"margin":@20};
//水平方向约束
[containtView addConstraints:[NSLayoutConstraint constraintsWithVisualFormat:@"H:|-margin-[blueView]-margin-[redView(blueView)]-margin-[yellowView(redView)]-margin-[grayView(yellowView)]-margin-|" options:0 metrics:metrics views:views]];
//blueView 的竖直方向
[containtView addConstraints:[NSLayoutConstraint constraintsWithVisualFormat:@"V:|-20-[blueView(==30)]" options:0 metrics:nil views:views]];
//redView 的竖直方向
[containtView addConstraints:[NSLayoutConstraint constraintsWithVisualFormat:@"V:|-20-[redView(blueView)]" options:0 metrics:nil views:views]];
//yellowView 的竖直方向
[containtView addConstraints:[NSLayoutConstraint constraintsWithVisualFormat:@"V:|-20-[yellowView(blueView)]" options:0 metrics:nil views:views]];
//grayView 的竖直方向
[containtView addConstraints:[NSLayoutConstraint constraintsWithVisualFormat:@"V:|-20-[grayView(blueView)]" options:0 metrics:nil views:views]];
}
*
*horizon方向布局
*
*/
-(void)setupHorisonalLayout:(UIView*)containtView{
//
UIView *blueView=[[UIView alloc]init];
blueView.backgroundColor=Color_1;
//
UIView *redView=[[UIView alloc]init];
redView.backgroundColor=Color_2;
//
UIView *yellowView=[[UIView alloc]init];
yellowView.backgroundColor=Color_3;
//
UIView *grayView=[[UIView alloc]init];
grayView.backgroundColor=Color_4;
[blueView setTranslatesAutoresizingMaskIntoConstraints:NO];
[redView setTranslatesAutoresizingMaskIntoConstraints:NO];
[yellowView setTranslatesAutoresizingMaskIntoConstraints:NO];
[grayView setTranslatesAutoresizingMaskIntoConstraints:NO];
[containtView addSubview:blueView];
[containtView addSubview:redView];
[containtView addSubview:yellowView];
[containtView addSubview:grayView];
NSDictionary *views=NSDictionaryOfVariableBindings(blueView,redView,yellowView,grayView);
NSDictionary *metrics=@{@"margin":@20};
//水平方向约束
[containtView addConstraints:[NSLayoutConstraint constraintsWithVisualFormat:@"H:|-margin-[blueView]-margin-[redView(blueView)]-margin-[yellowView(redView)]-margin-[grayView(yellowView)]-margin-|" options:0 metrics:metrics views:views]];
//blueView 的竖直方向
[containtView addConstraints:[NSLayoutConstraint constraintsWithVisualFormat:@"V:|-20-[blueView(==30)]" options:0 metrics:nil views:views]];
//redView 的竖直方向
[containtView addConstraints:[NSLayoutConstraint constraintsWithVisualFormat:@"V:|-20-[redView(blueView)]" options:0 metrics:nil views:views]];
//yellowView 的竖直方向
[containtView addConstraints:[NSLayoutConstraint constraintsWithVisualFormat:@"V:|-20-[yellowView(blueView)]" options:0 metrics:nil views:views]];
//grayView 的竖直方向
[containtView addConstraints:[NSLayoutConstraint constraintsWithVisualFormat:@"V:|-20-[grayView(blueView)]" options:0 metrics:nil views:views]];
}
最终实现的页面是这样的:
注意:
使用VFL布局时一定要添加下面这行代码:
[blueView setTranslatesAutoresizingMaskIntoConstraints:NO];
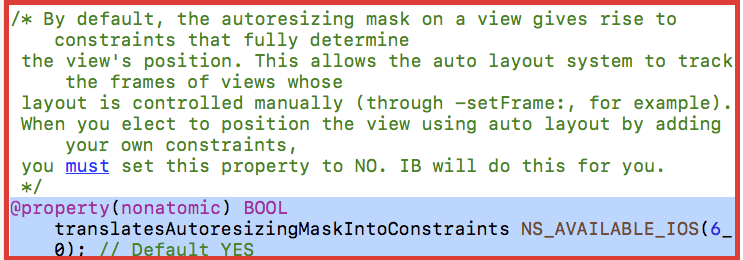
这是官方对这个方法的注解,我的理解是:
这个参数默认时为yes,autoresizing会自动为你生成一组决定view位置的约束(也就是说默认情况下你添加layout约束是无效的),此时使用的不是Autolayout布局约束而是autoresizing
Autoresizing:
已经是比较过时的设置适配方法了,而且有很大的缺陷,只能设置父子控件之间的约束,不能设置兄弟控件之间的约束。所以在这里我们最好不要再开发中应用。
AutoLayout:
最流行的适配方式,苹果积极推荐,非常强大好用的适配方法。对提升开发中的效率有很大的帮助。
autolayout 和Autoresizing 是会相互冲突的
Autoresizing:
已经是比较过时的设置适配方法了,而且有很大的缺陷,只能设置父子控件之间的约束,不能设置兄弟控件之间的约束。所以在这里我们最好不要再开发中应用。
AutoLayout:
最流行的适配方式,苹果积极推荐,非常强大好用的适配方法。对提升开发中的效率有很大的帮助。
autolayout 和Autoresizing 是会相互冲突的