学习怎样创建对象是理解面向对象编程的第一步,第二步是理解继承。
JS的继承方式与传统的面向对象编程语言不同,继承可以发生对象之间,这种继承的机制是我们已经熟悉的一种机制:原型
一.原型链接和Object.prototype
js内置的继承方式被称为原型链接或原型继承
原型链:对象会继承自己原型对象中的属性,而这个原型会继续继承自己的原型,依此类推
所有的对象都继承 Object.prototype
var book = {
title:'黑客与画家'
};
var prototype = Object.getPrototypeOf(book);
console.log(prototype === Object.prototype)//true
从Object.prototype中继承的方法
.hasOwnProperty() 判断对象中有没有某个属性
.propertyIsEnumerable() 判断对象是否可枚举
.isPrototypeOf() 判断一个对象是否是另一个对象的原型
.valueOf() 返回对象值的表示形式
.toString() 返回对象的字符串表示形式
.toLocaleString() 返回对象的本地保存形式
valueOf() 当我们操作对象时就会被调用。默认情况下会返回对象实例。对于字符串,布尔值和数字类型的值,首先会使用原始包装器包装成对象,在调用方法。
var now = new Date(); var earlier = new Date(2010,3,28) console.log(now > earlier) //true console.log(now.toString()) //Fri Sep 15 2017 19:44:20 GMT+0800 (中国标准时间) console.log(now.toLocaleDateString()) //2017/9/15
二. 对象继承
最简单的继承方式是对象间的继承。我们所需要做的就是指定新创建的对象的原型指向哪个对象。Object字面量的形式创建的对象默认将__proto__属性指向了Object.prototype,但是我们可以通过Object.create()方法将__proto__属性指向其它对象
var person1 = {
name:'李三婆',
sayName:function(){
console.log(this.name);
}
};
var person2 = Object.create(person1,{
name:{
value:'刘老二',
enumerable:true,
}
})
person1.sayName() //李三婆
person2.sayName() //刘老二
console.log(person2.hasOwnProperty('sayName'))//false
console.log(person1.hasOwnProperty('sayName'))//true
console.log(person1.isPrototypeOf(person2))//true
三.构造函数继承
//1.简单示例:
var pander = {
name:'李四',
constructor:Persion
}
function Persion(name){
this.name = name
}
Persion.prototype = pander
var person1 = new Persion()
console.log(pander.isPrototypeOf(person1))//true
console.log(person1)
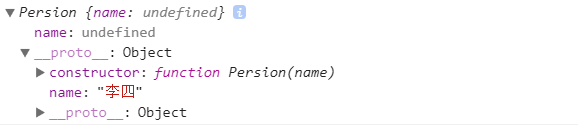
//2.由于prototype属性是可写的,因此通过复写它我们可以改变原型链。例如:
function Rectangle(length,width){
this.length = length;
this.width = width;
}
Rectangle.prototype.getArea = function(){
return this.length * this.width;
}
Rectangle.prototype.toString = function(){
return '[Rectangle '+this.length+' x '+this.width+']'
}
Rectangle.prototype.getBoder = function(){
return 4;
}
// 继承 Rectangle
function Square(size){
Rectangle.call(this, size,size)
}
Square.prototype = new Rectangle;
Rectangle.prototype.constructor = Rectangle;
Square.prototype.toString = function(){
var text = Rectangle.prototype.toString.call(this);
return text.replace('Rectangle','Square')
}
Square.prototype.getBoder = function(){
return 'Square边数:' + Rectangle.prototype.getBoder();
}
var square = new Square(20)
//console.log(square.getArea()) //400
//console.log(square.toString()) //[Square 20 x 20]
//console.log(square.getBoder()) //Square边数:4
在上面的代码,使用Square.prototype.toString()和call()方法一起调用Rectangle.prototype.toString()。
这个方法只需要在返回结果之前将Rectangle替换成Square就可以了。