1、介绍
所谓程序集级别的依赖注入是指接口和实现的依赖不使用配置文件或硬代码实现(builder.RegisterType<UserInfoService>().As<IUserInfoService>();),而是通过名称约定实现依赖注入
2、项目接口及dll
2.1 项目结构(创建MVC3项目)

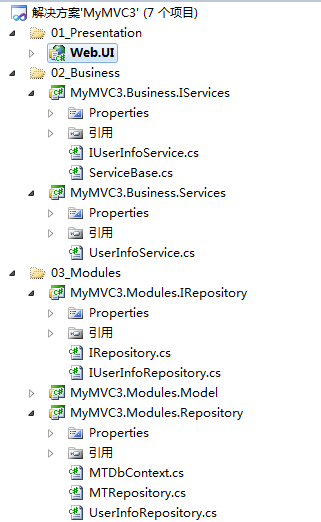
2.2 UI层需引入的dll(使用nuget引入)
2.2.1 Autofac
2.2.2 Autofac.Integration.Mvc
2.2.3 MyMVC3.Business.IServices
2.2.4 MyMVC3.Modules.IRepository
2.2.5 MyMVC3.Modules.Model
3、依赖注入配置代码(在Global.asax的Application_Start方法中调用)
/// <summary> /// 使用AutoFac实现依赖注入 /// </summary> private void autoFac() { var builder = new ContainerBuilder(); SetupResolveRules(builder); //注入 builder.RegisterControllers(Assembly.GetExecutingAssembly()); //注入所有Controller var container = builder.Build(); DependencyResolver.SetResolver(new AutofacDependencyResolver(container)); }
private void SetupResolveRules(ContainerBuilder builder) { //UI项目只用引用service和repository的接口,不用引用实现的dll。 //如需加载实现的程序集,将dll拷贝到bin目录下即可,不用引用dll var IServices = Assembly.Load("MyMVC3.Business.IServices"); var Services = Assembly.Load("MyMVC3.Business.Services"); var IRepository = Assembly.Load("MyMVC3.Modules.IRepository"); var Repository = Assembly.Load("MyMVC3.Modules.Repository"); //根据名称约定(服务层的接口和实现均以Service结尾),实现服务接口和服务实现的依赖 builder.RegisterAssemblyTypes(IServices, Services) .Where(t => t.Name.EndsWith("Service")) .AsImplementedInterfaces(); //根据名称约定(数据访问层的接口和实现均以Repository结尾),实现数据访问接口和数据访问实现的依赖 builder.RegisterAssemblyTypes(IRepository, Repository) .Where(t => t.Name.EndsWith("Repository")) .AsImplementedInterfaces();
//或者
Assembly serviceAss = Assembly.Load("Elight.Service");
Type[] serviceTypes = serviceAss.GetTypes();
builder.RegisterTypes(serviceTypes).Where(t => t.Name.EndsWith("Service")).AsImplementedInterfaces();//表示注册的类型,以接口的方式注册
Assembly repositoryAss = Assembly.Load("Elight.Repository");
Type[] repositoryTypes = repositoryAss.GetTypes();
builder.RegisterTypes(repositoryTypes).Where(t => t.Name.EndsWith("Repository")).AsImplementedInterfaces();
}
Assembly.LoadFile只载入相应的dll文件,比如Assembly.LoadFile("a.dll"),则载入a.dll,假如a.dll中引用了b.dll的话,b.dll并不会被载入。
Assembly.LoadFrom则不一样,它会载入dll文件及其引用的其他dll,比如上面的例子,b.dll也会被载入。
4、各层级间配置构造函数注入
4.1 UserInfoService
private IUserInfoRepository productRepository; public UserInfoService(IUserInfoRepository productRepository) { this.productRepository = productRepository; this.AddDisposableObject(productRepository); }
4.2 UserInfoService
public IUserInfoService userService; public HomeController(IUserInfoService userService) { this.userService = userService; this.AddDisposableObject(userService); }