15.1 查询表达式的概念
private static void ShowContextualKeywords1()
{
IEnumerable<string> selection = from word in Keywords
where !word.Contains('*')
select word;
foreach (string keyword in selection)
{
Console.Write(" " + keyword);
}
}
private static string[] Keywords = {
"abstract", "add*", "alias*", "as", "ascending*", "base",
"bool", "break", "by*", "byte", "case", "catch", "char",
"checked", "class", "const", "continue", "decimal",
"default", "delegate", "descending*", "do", "double",
"dynamic*", "else", "enum", "event", "equals*",
"explicit", "extern", "false", "finally", "fixed",
"from*", "float", "for", "foreach", "get*", "global*",
"group*", "goto", "if", "implicit", "in", "int",
"into*", "interface", "internal", "is", "lock", "long",
"join*", "let*", "namespace", "new", "null", "object",
"on*", "operator", "orderby*", "out", "override",
"params", "partial*", "private", "protected", "public",
"readonly", "ref", "remove*", "return", "sbyte", "sealed",
"select*", "set*", "short", "sizeof", "stackalloc",
"static", "string", "struct", "switch", "this", "throw",
"true", "try", "typeof", "uint", "ulong", "unchecked",
"unsafe", "ushort", "using", "value*", "var*", "virtual",
"void", "volatile", "where*", "while", "yield*"
};
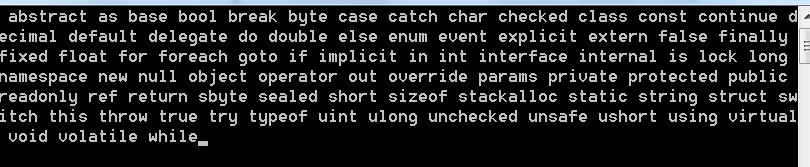
15.1.1 投射
IEnumerbale<T>
或 IQueryable<T>
集合。T数据类型是从select或者groupby子句推导。select word
推导的,因为word是一个字符串。word数据类型是由from
子句所指定的IEnumerbale<T>集合的类型参数(这里是Keywords)。由于Keywords是一个string数组,它实现了IEnumerbale<T>
,所以word是一个字符串。
public static void Main()
{
List1(Directory.GetCurrentDirectory(),"*");
}
static void List1(string rootDirectory, string searchPattern)
{
IEnumerable<FileInfo> files =
from fileName in Directory.GetFiles(
rootDirectory, searchPattern)
select new FileInfo(fileName);
foreach (FileInfo file in files)
{
Console.WriteLine(".{0} ({1})",
file.Name, file.LastWriteTime);
}
}

IEnumerable<FileInfo>
,而不是System.IO.Directory.GetFiles()
返回的IEnumerables<string>
数据类型。
var files =
from fileName in Directory.GetFiles(
rootDirectory, searchPattern)
select new FileInfo(fileName);
15.1.2 筛选
IEnumerable<string> selection = from word in Keywords
where !word.Contains('*')
select word;
15.1.3 排序
IEnumerable<string> fileNames =
from fileName in Directory.GetFiles(
rootDirectory, searchPattern)
orderby (new FileInfo(fileName)).Length descending,
fileName
select fileName;
15.1.4 let子句
public static void Main()
{
ListByFileSize2(Directory.GetCurrentDirectory(), "*");
}
static void ListByFileSize2(
string rootDirectory, string searchPattern)
{
IEnumerable<FileInfo> files =
from fileName in Directory.GetFiles(
rootDirectory, searchPattern)
orderby new FileInfo(fileName).Length, fileName
select new FileInfo(fileName);
foreach (FileInfo file in files)
{
// As simplification, current directory is
// assumed to be a subdirectory of
// rootDirectory
string relativePath = file.FullName.Substring(
Environment.CurrentDirectory.Length);
Console.WriteLine(".{0}({1})",
relativePath, file.Length);
}
}
IEnumerable<FileInfo> files =
from fileName in Directory.GetFiles(
rootDirectory, searchPattern)
let file = new FileInfo(fileName)
orderby file.Length, fileName
select file;
let子句引入了一个新的范围变量
它容纳的表达式值可以在查询表达式剩余部分使用
可以添加任意数量的let表达式,只需要它们每一个作为一个附加的子句
放在第一个from子句之后,最后一个select/group by子句之前,加入查询即可
15.1.5 分组
private static void GroupKeywords1()
{
IEnumerable<IGrouping<bool, string>> selection =
from word in keyWords
group word by word.Contains('*');
foreach (IGrouping<bool, string> wordGroup
in selection)
{
Console.WriteLine(Environment.NewLine + "{0}:",
wordGroup.Key ?
"Contextual Keywords" : "Keywords");
foreach (string keyword in wordGroup)
{
Console.Write(" " +
(wordGroup.Key ?
keyword.Replace("*", null) : keyword));
}
}
}
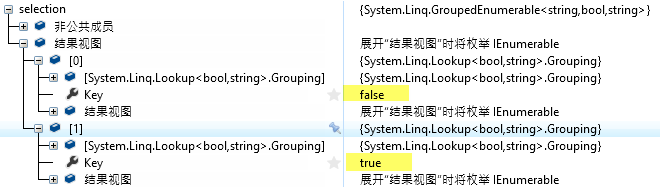
结果


private static void GroupKeywords1()
{
IEnumerable<IGrouping<bool, string>> keywordGroups =
from word in keyWords
group word by word.Contains('*');
var selection = from groups in keywordGroups
select new
{
IsContextualKeyword = groups.Key,
Items = groups
};
foreach (var wordGroup in selection)
{
Console.WriteLine(Environment.NewLine + "{0}:",
wordGroup.IsContextualKeyword ?
"Contextual Keywords" : "Keywords");
foreach (var keyword in wordGroup.Items)
{
Console.Write(" " +
keyword.Replace("*", null));
}
}
}

15.1.6 使用into进行查询延续
var selection =
from word in keyWords
group word by word.Contains('*')
into groups
select new
{
IsContextualKeyword = groups.Key,
Items = groups
};
15.1.7 用多个from 子句“平整”序列的序列
var selection = from word in keyWords
from character in word
select character;
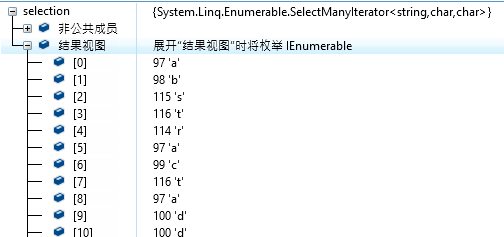
var numbers = new[] {1, 2, 3};
var product = from word in keyWords
from number in numbers
select new {word, number};
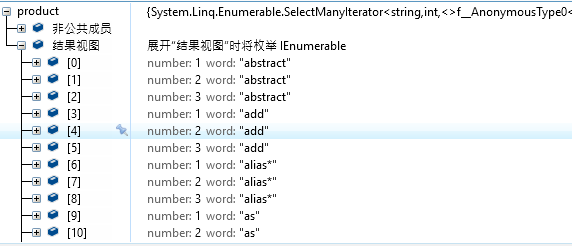
主题:不重复的成员
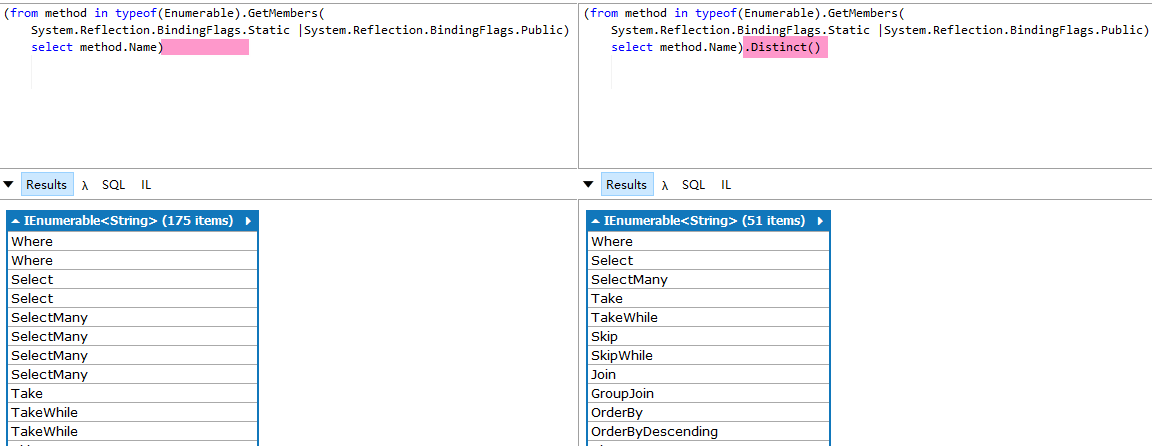
IEnumerable<string> selection = from word in Keywords
where !word.Contains('*')
select word;
IEnumerable<string> selection =
keyWords.Where(word => word.Contains('*'));