题目如下:
方法一:动态编程解题思路:
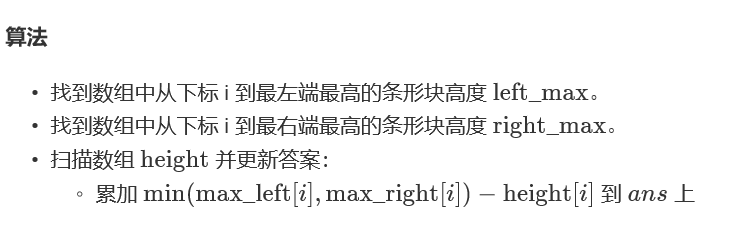
代码如下:
int trap(vector<int>& height) { if(height == null) return 0; int ans = 0; int size = height.size(); vector<int> left_max(size), right_max(size); left_max[0] = height[0]; for (int i = 1; i < size; i++) { left_max[i] = max(height[i], left_max[i - 1]); } right_max[size - 1] = height[size - 1]; for (int i = size - 2; i >= 0; i--) { right_max[i] = max(height[i], right_max[i + 1]); } for (int i = 1; i < size - 1; i++) { ans += min(left_max[i], right_max[i]) - height[i]; } return ans; }
方法二:双指针解题思路:
代码:
class Solution: def trap(self, height): """ :type height: List[int] :rtype: int """ left = 0 right = len(height) - 1 res = 0 left_max = 0 right_max = len(height) - 1 while left < right: if height[left] > height[right]: if height[right_max] < height[right]: right_max = right right = right - 1 else: res += height[right_max] - height[right] right = right - 1 if height[left] <= height[right]: if height[left_max] < height[left]: left_max = left left = left + 1 else: res += height[left_max] - height[left] left = left + 1 return res