configparser模块
config:配置,parser:解析。字面意思理解configparser模块就是配置文件的解析模块。
来看一个好多软件的常见文档格式如下:
[DEFAULT] # 标题性的东西, # 下面四组键值对是关于[DEFAULT]的介绍 ServerAliveInterval = 45 Compression = yes CompressionLevel = 9 ForwardX11 = yes [bitbucket.org] User = hg [topsecret.server.com] Port = 50022 ForwardX11 = no
- 用Python生成配置文件
如果想用python生成一个这样的文档怎么做呢?
import configparser config = configparser.ConfigParser() # 实例出来一个对象,相当于创建了一个空字典config={} # ["DEFAULT"] 相当于字典config的键,{}里的内容是键["DEFAULT"]的值。 config["DEFAULT"] = {'ServerAliveInterval': '45', 'Compression': 'yes', 'CompressionLevel': '9'} # 把写好的对象写到一个文件里 # 把句柄configfile作为内容写入到xample.ini文件里。 with open('example.ini', 'w') as configfile: config.write(configfile) # 通过config对象,调用write方法,把句柄configfile写入到example.ini文件里。
执行后example.ini文件的内容如下:
文件内容与最开始的文件结构一致了,这样一个简单的配置文件就通过Python生成好了。
再来通过Python给生成还有更多块的配置文件
import configparser config = configparser.ConfigParser() # 实例出来一个对象,相当于创建了一个空字典config={} # ["DEFAULT"] 相当于字典config的键,{}里的内容是键["DEFAULT"]的值。 # 相当于创建了一个[DEFAULT]块(指的是在配置文件里的块哦) config["DEFAULT"] = {'ServerAliveInterval': '45', 'Compression': 'yes', 'CompressionLevel': '9'} config['DEFAULT']['ForwardX11'] = 'yes' # 也可以这样给字典里新增键值对 # 这个写法虽然与上面不一样,但是原理是一样的 # 首先config的键['bitbucket.org'] 对应一个空字典{} # 这就相当于又创建了一个块[bitbucket.org](在配置文件里哦) config['bitbucket.org'] = {} # 给空字典{}里增加了一个键值对 config['bitbucket.org']['User'] = 'hg' # 同理又创建了一个块[topsecret.server.com] config['topsecret.server.com'] = {} topsecret = config['topsecret.server.com'] # 然后空字典{}里给它填值 topsecret['Host Port'] = '50022' # mutates the parser topsecret['ForwardX11'] = 'no' # same here # 把写好的对象写到一个文件里 # 把句柄configfile作为内容写入到xample.ini文件里。 with open('example.ini', 'w') as configfile: config.write(configfile) # 通过config对象,调用write方法,把句柄configfile写入到example.ini文件里。
生成后的配置文件内容

# 无注释版代码 import configparser config = configparser.ConfigParser() config["DEFAULT"] = {'ServerAliveInterval': '45', 'Compression': 'yes', 'CompressionLevel': '9'} config['DEFAULT']['ForwardX11'] = 'yes' config['bitbucket.org'] = {} config['bitbucket.org']['User'] = 'hg' config['topsecret.server.com'] = {} topsecret = config['topsecret.server.com'] topsecret['Host Port'] = '50022' topsecret['ForwardX11'] = 'no' with open('example.ini', 'w') as configfile: config.write(configfile)
- 处理配置文件
配置文件生成了,那如何使用呢?一般对文件的使用或处理就是增、删、改、查,来看看吧!
-
- 查
import configparser # 1. 不管是生成文件还是处理文件,都得先通过configparser.ConfigParser()生成一个对象config config = configparser.ConfigParser() # 2. 生成的对象要跟将要处理的文件进行关联后,才能处理 config.read("example.ini") # 一. 查 # # 1. 可以查出有哪些块名 print(config.sections()) # ['bitbucket.org', 'topsecret.server.com'] ''' 1. sections是块的意思; 2. 只会打印出[DEFAULT]以外的块名 3. 得到块名,就可以进行判断操作 ''' # 比如,可以判断用户输入的那部分有没有在配置文件里。 # 直接判断是不是在config,其实也是判断是否在sections里,所以下面两个写法效果一样。 print("bytebong.com" in config) # False print("bytebong.com" in config.sections()) # False print("bitbucket.org" in config) # True 判断块名是否在config # 2. 可以查出块下有什么键值对 # 注意,这个查询不区分大小写哦,配置文件里是小写,这里写大写的USER,照样可以取到正确的值 print(config["bitbucket.org"]["USER"]) # hg # 3. 查询DEFAULT下面某个键的值 print(config['DEFAULT']['serveraliveinterval']) # 45 # 4. 既然是一个字典,当然也可以进行遍历 for key in config['topsecret.server.com']: print(key) # 结果 # host port # forwardx11 # serveraliveinterval # compression # compressionlevel ''' 注意,这里有个问题,遍历的只是[topsecret.server.com]块下的键,为什么[DEFAULT]下面的键也出来了呢? [答] 这就是[DEFAULT]的一个特点,不管你遍历谁,它都会跟着被遍历出来。 那如果你说,那我不想遍历出[DEFAULT]的内容怎么办? [答] 不想遍历出,那你就别用[DEFAULT]命名,[DEFAULT]就是这么关键。 [DEFAULT]的作用: 是文件信息,无论你用到那一部分,[DEFAULT]都必须需要跟着出现的配置信息,是非有不可的场景。 举例: [DEFAULT]就相当于一个公用的,比如你想给客户展示的所有信息,都必须带着公司的名字,这些所有信息可能存在于不同的 配置文件,或者块下面,既然都得带着公司名字,就没必要每个配置文件或者块都写一遍, 就可以把公司信息提出了单独写在 [DEFAULT]里,这样无论什么时候都可以取到公司名字了。 ''' # 4. options():提取出配置文件里块名下的键,然后把结果放在列表里。 # 上面遍历取到的结果都是单独的显示在一行上 print(config.options("topsecret.server.com")) # ['host port', 'forwardx11', 'serveraliveinterval', 'compression', 'compressionlevel']' # 5. items():把取到的键和值结果组成一个元组显示 print(config.items("bitbucket.org")) # [('serveraliveinterval', '45'), ('compression', 'yes'), ('compressionlevel', '9'), ('forwardx11', 'yes'), ('user', 'hg')] # 6. get(): 连续去去值的功能 print(config.get("bitbucket.org", "compressionlevel")) # 9 ''' get()方法的工作原理: 第一步:先得到"bitbucket.org"下的值,它的值是一个字典A 第二步:再取这个字典A里的值 注意,从文件里看,bitbucket.org下面只有一个字典,键值对是user = hg,为什么这里可以取到compressionlevel的值呢? [答] 这个其实上面已经说过了, 因为compressionlevel是在[DEFAULT]下面的,所以无论在其他哪个块下,都可以提取到[DEFAULT] 下的信息 '''
上面的查操作仅仅是从配置文件里查询内容,没有对文件进行任何修改,而下面介绍的增,删,改都对文件进行了修改,所以操作完成后,都有一个共同的
语句,必须把最终结果写入配置文件
-
- 增
import configparser config = configparser.ConfigParser() config.read("example.ini") # 1. add_section():增加一个块 config.add_section("yuan") config.write(open("i.cfg", 'w')) ''' 上面先从config里读取了文件 下面再增加一个块并写进了一个新的文件 所以,新的文件i.cfg里有四个块 '''
# 2. set(): 给块下面增加值 import configparser config = configparser.ConfigParser() config.read("example.ini") config.add_section("yuan") # 给块里加值
# 第一个参数是块; 第二个参数是块下面的键; 第三个参数是键对应的值 config.set("yuan","key1","value1") config.set("yuan","key2","value2") config.write(open("i.cfg", 'w'))
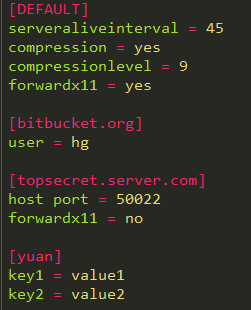
-
- 删
上面已经生成了i.cfg文件,就直接用它吧 import configparser config = configparser.ConfigParser() config.read("i.cfg") # 1. remove_section(): 删除块 config.remove_section("topsecret.server.com") # 会把该块下的内容全部删除 # 2. 删除块下的键值对 config.remove_option("yuan", "key1") config.write(open("i.cfg", 'w'))
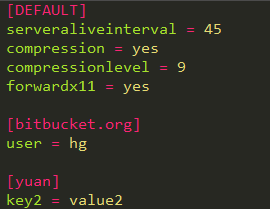
小技巧
config.write(open("i.cfg", 'w')) 这里的open()没有让它等于一个文件句柄 f,所以是不需要进行close的。 建议以后都这样写,这种写法非常好的。 直接在一个方法里面写个open()代替f的话,是不需要对它进行close的。
-
- 改
# 修改其实就是set()方法实现 # set可以新增键值对,也可以更新键值对的值 import configparser config = configparser.ConfigParser() config.read("i.cfg") config.set("yuan","key2","value3") config.write(open("i.cfg", 'w'))
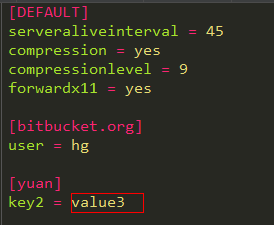