什么是函数?
函数是组织好的,可重复使用的,用来实现单一,或相关联功能的代码段
1.函数命名必须要以字母开头
>>> def a2_33():
... pass#pass表示不做任何操作,只是占位
...
>>> a2_33()#函数调用函数名加括号
>>>
练习:统计字母的个数
>>> def count_letter(s):
... result = 0
... for i in s:
... if i >='a' and i <='z':
... result +=1
... return result
...
>>> count_letter("1a2Drty")
4
练习:统计一句话里有多少个数字
>>> def f(s):
... result =0
... for i in s:
... if i >='0' and i <= '9':
... result +=1
... return result
...
>>> f("1a2b3c4d")
4
函数的传参
>>> def add(a,b):
... return a+b
...
>>> add(1,2)
3
def add(a,b):
if isinstance(a,(int,float,complex)) and isinstance(b,(int,float,complex)):#判断参数类型
return a+b# return有短路功能,所以不用写else
print("one of two parameters is not a number type!")
return None
print(add("a",2))
全局变量和局部变量
>>> n = 1#在函数外部定义的变量叫全局变量
>>> def func():
... n = 2#在函数内部定义的变量,叫局部变量,出了这个函数,这个局部变量就不生效了
... return n
...
>>> func()
2
>>> print(n)
1
>>> func =10
>>> func()
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: 'int' object is not callable#不能重新给一个函数去赋值 否则会报错
>>>
必填参数:不可少
可选参数:当有默认值的时候不填也会使用默认值,无默认值时会使用传递的值,默认值必须放在非默认值后面
>>> def add(a,b=100):
... return a+b
...
>>> add(1)#使用默认值
101
>>>
>>> add(1,11)#传值
12
>>>
>>> add(b=1,a=11)#带参数名传值
12
>>>
>>> def add(a=100,b):
... return a+b
...
File "<stdin>", line 1
SyntaxError: non-default argument follows default argument#默认值放在了非默认值前面,报错
返回多个参数
>>> def times_ten_bigger(a,b):
... return a*10,b*10
...
>>> a,b=times_ten_bigger(1,2)
>>> print(a)
10
>>> print(b)
20
>>>
参数命名的方式传值
return a*10,b*10,c*10
print(times_ten_bigger(c=5,b=1,a=2))
>>> n=1
>>> def func():
... return n+1
...
>>> func()
2
>>>
2 可以这样写
>>> def func():
... n=2
... return n
...
>>> func()
2
>>>
>>> def func():
... n+=1
... return n#不知道返回的是哪一个n
...
>>> func()
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "<stdin>", line 2, in func
UnboundLocalError: local variable 'n' referenced before assignment
3可以这样写 改为全局变量
>>> n=1
>>> def func():
... global n
... n+=1
... return n
...
>>> func()
2
>>> n
2
>>>
4 可以这样写
>>> n =1
>>> def func(a):
... a=a+1
... return a
...
>>> func(n)#n被当作一个参数传入
2
>>> n
1
>>>
n =[]
def func(a):
a.append(1)
print(n)
def func(a):
a=a+"d"
print(func(n))
print(n)
如果你传入的参数是个变量a,这个变量是可变类型
(list、dict、set)
那么函数内部对于这个参数的所有操作结果都会影响
外部的参数值
原则2:
如果你传入的参数是个变量a,这个变量是不可变类型()
(字符串、整数、小数)
那么 函数内部对于这个参数的所有操作结果都不会影响外部变量
可变参数,*c,存储为元祖
练习:
result =a+b
for i in c:
result +=i
for i in d.values():
result +=i
return result
print(add(1,2,3,4,c=5,d=6,e=7))
函数缺省值
>>> def add(a,b=100):
... return a+b
...
>>> print(add(10))
110
>>>
函数传入的参数为不可变的,对外部的变量没有影响
按值传。(传的不是变量对应的内存地址)
函数传入的参数为可变的,对外部的变量就会有影响
按引用传。(传的是变量对于的内存地址)
>>> a=100
>>> def f(a):
... a+=1
... return a
...
>>> f(a)
101
>>> a =[]
>>> def f(a):
... a.append(1)
... return a
...
>>> f(a)
[1]
>>> a
[1]
>>>
全局变量
>>> a =100
>>> def f():
... global a
... a+=1
... return a
...
>>> a
100
>>> f()
101
>>>
可变参数传递
>>> def f(a,b,*arg):
... print(type(arg))
... for i in arg:
... print(i)
...
>>> f(1,2,3)
<class 'tuple'>
3
>>> f(1,2,3,4)
<class 'tuple'>
3
4
>>> f(1,2,3,4,5,6,7)
<class 'tuple'>
3
4
5
6
7
>>>
练习:
写一个函数,使用可变参数计算函数所有参数之和
... result =0
... result =a+b
... for i in arg:
... result +=i
... return result
...
>>> f(1,2,3,4,5)
15
... for i in arg:
... print(i)
...
>>> f(1,2,3,4,5)
1
2
3
4
5
>>>
... print(type(kw))
... for k,v in kw.items():
... print(k,v)
...
>>> f(a=1,b=2,c=3)
<class 'dict'>
c 3
>>> f(1,2,a=3,b=4,c=5)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: f() got multiple values for argument 'a' #传参重复,a已经存在
>>> f(1,2,c=3,d=4,e=5)
<class 'dict'>
c 3
d 4
e 5
>>>
... print(a,b)
...
>>> func(b=1,a=2)
2 1
>>>
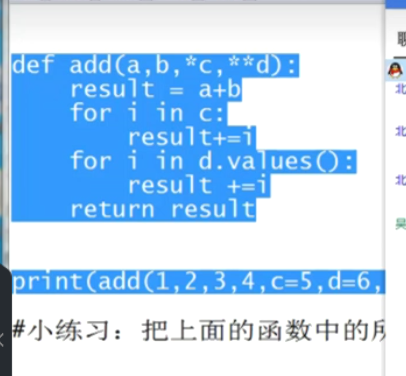