/**
* 以程序为主体
* 以文件为目的地,进行输入输出,修改,删除等操作
* out write为输出信息,也是向文件中写入东西的意思。 将信息存入目的地
* in read为输入信息,读取文件内容,然后展示在我们控制台。 从目的地获得信息
*/
创建一个新txt文件

/** * 创建一个新文件 */ @Test public void test1() { File f = new File("D:\hello.txt"); try { f.createNewFile(); } catch (IOException e) { e.printStackTrace(); } }
File的两个常量,有的在os不同的时候是不一样的。

@Test public void test2() { System.out.println(File.separator); System.out.println(File.pathSeparator); }
增强代码可用性

@Test public void test3() { String fileName = "D:" + File.separator + "hello1.txt"; File f = new File(fileName); try { f.createNewFile(); } catch (IOException e) { e.printStackTrace(); } }
删除一个txt文件

@Test public void test4() { String fileName = "D:" + File.separator + "hello.txt"; File f = new File(fileName); if (f.exists()) { f.delete(); } else { System.out.println(fileName + "改文件不存在"); } }
列出指定目录全部文件,包括隐藏文件

@Test public void test5() { String fileName = "D:" + File.separator; File f = new File(fileName); String[] strings = f.list(); for (int i = 0; i < strings.length; i++) { System.out.println(strings[i]); } System.out.println(strings.length); }
列出指定目录的所有文件,包含隐含文件,列出的方式是
* 展现所有目录的完整路径

@Test public void test6() { String fileName = "D:" + File.separator; File f = new File(fileName); File[] files = f.listFiles(); for (int i = 0; i < files.length; i++) { System.out.println(files[i]); } System.out.println(files.length); }
判断指定路径是不是真实路径

@Test public void test7() { String fileName = "D:" + File.separator; File f = new File(fileName); if (f.isDirectory()) { System.out.println(fileName + "是真实路径"); } else { System.out.println(fileName + "不是真实路径"); } }
打印出指定目录的全部内容,完整路径。-----递归

@Test public void test8() { // String fileName = "D:"+File.separator; String fileName = "D:\640808\shenshaonian\Program Files-fan"; File f = new File(fileName); printf(f); } public static void printf(File f) { if (null != f) { if (f.isDirectory()) { File[] files = f.listFiles(); if (null != files) { for (int i = 0; i < files.length; i++) { printf(files[i]); } } } else { System.out.println(f); } } }
使用RandomAccessFile写入文件,结果发现是乱码

public void test9() throws IOException { String fileName = "D:" + File.separator + "hello.txt"; File f = new File(fileName); RandomAccessFile demo = new RandomAccessFile(f, "rw"); demo.writeBytes("randomAccessFile写入"); demo.writeInt(12); demo.writeBoolean(true); demo.writeChar('A'); demo.writeFloat(1.21f); demo.writeDouble(12.123); demo.close(); }
原因https://www.cnblogs.com/kakaisgood/p/8857885.html
按字节序列将该字符串写入该文件。该字符串中的每个字符均按顺序写出,并丢弃其高八位。写入从文件指针的当前位置开始
问题出在writeBytes(String s)这个方法上。
JAVA中的char是16位的,一个char存储一个中文字符,直接用writeBytes方法转换会变为8位,直接导致高8位丢失。从而导致中文乱码。
乱码的原因和解决方案

public void test9() throws IOException { String fileName = "D:" + File.separator + "hello.txt"; File f = new File(fileName); RandomAccessFile demo = new RandomAccessFile(f, "rw"); // String s = "randomAccessFile写入"; // demo.write(s.getBytes()); // demo.write("randomAccessFile写入fdasf".getBytes()); // int i = 12; // String ii = Integer.valueOf(i).toString(); // demo.write(ii.getBytes()); double db = 12.123; demo.write(Double.valueOf(db).toString().getBytes()); // demo.writeBytes("randomAccessFile写入"); // JAVA中的char是16位的,一个char存储一个中文字符,直接用writeBytes方法转换会变为8位, // 直接导致高8位丢失。从而导致中文乱码。 // 解决方法: // 现转换为字节组,再write写入流。 // demo.writeInt(12);int使用的是一个4个字节保存的,想显示出来必须转换为字符串。。。 // demo.writeBoolean(true); 占8字节,写进去是什么都没显示的,可读取出来,代码如下 // demo.writeChar('A'); // demo.writeFloat(1.21f);4字节,转string,按字节写入 // demo.writeDouble(12.123);,8字节 demo.close(); // //写入写入boolean // boolean b = true; // // create a new RandomAccessFile with filename test // RandomAccessFile raf = new RandomAccessFile("d:/test11.txt", "rw"); // // write a boolean // raf.writeBoolean(false); // // set the file pointer at 0 position // raf.seek(0); // // print the boolean // System.out.println("" + raf.readBoolean()); // // write a boolean // raf.writeBoolean(b); // // set the file pointer at position 1 // raf.seek(1); // // print the boolean // System.out.println("" + raf.readBoolean()); }
FileOutputStream向文件中写入字符串,结果 正常显示

@Test public void test10() throws IOException { String fileName = "D:" + File.separator + "hello.txt"; File f = new File(fileName); OutputStream out = new FileOutputStream(f); String str = "你好啊 字节流"; byte[] b = str.getBytes(); out.write(b); out.close(); }
一个字节一个字节的写入FileOutputStream

@Test public void test11() throws IOException { String fileName = "D:" + File.separator + "hello.txt"; File f = new File(fileName); OutputStream out = new FileOutputStream(f); String str = "nihao 我一个一个字节的写入"; byte[] b = str.getBytes(); for (int i = 0; i < b.length; i++) { out.write(b[i]); } out.close(); }
字节流,向文件中追加新的内容 true FileOutputStream

@Test public void test12() throws IOException { String fileName = "D:" + File.separator + "hello.txt"; File f = new File(fileName); OutputStream out = new FileOutputStream(f, true); String str = "append Roolen"; String str2 = " 换行"; byte[] b1 = str.getBytes(); byte[] b2 = str2.getBytes(); for (int i = 0; i < b1.length; i++) { out.write(b1[i]); } for (int i = 0; i < b2.length; i++) { out.write(b2[i]); } out.close(); }
关于 https://www.cnblogs.com/thinkingthigh/p/7642680.html
Windows系统里面,每行结尾是“<回车><换行>”,即“ ”;
字节流 读取文件内容FileInputStream

@Test public void test13() throws IOException { String fileName = "D:" + File.separator + "hello.txt"; File f = new File(fileName); InputStream in = new FileInputStream(f); byte[] b = new byte[1024]; int len = in.read(b); in.close(); System.out.println(new String(b)); System.out.println(len); }
1024字节,1024byte,
1kb
1byte= 8bit
关于位,字节,字,
https://blog.csdn.net/m0_37526672/article/details/80273098
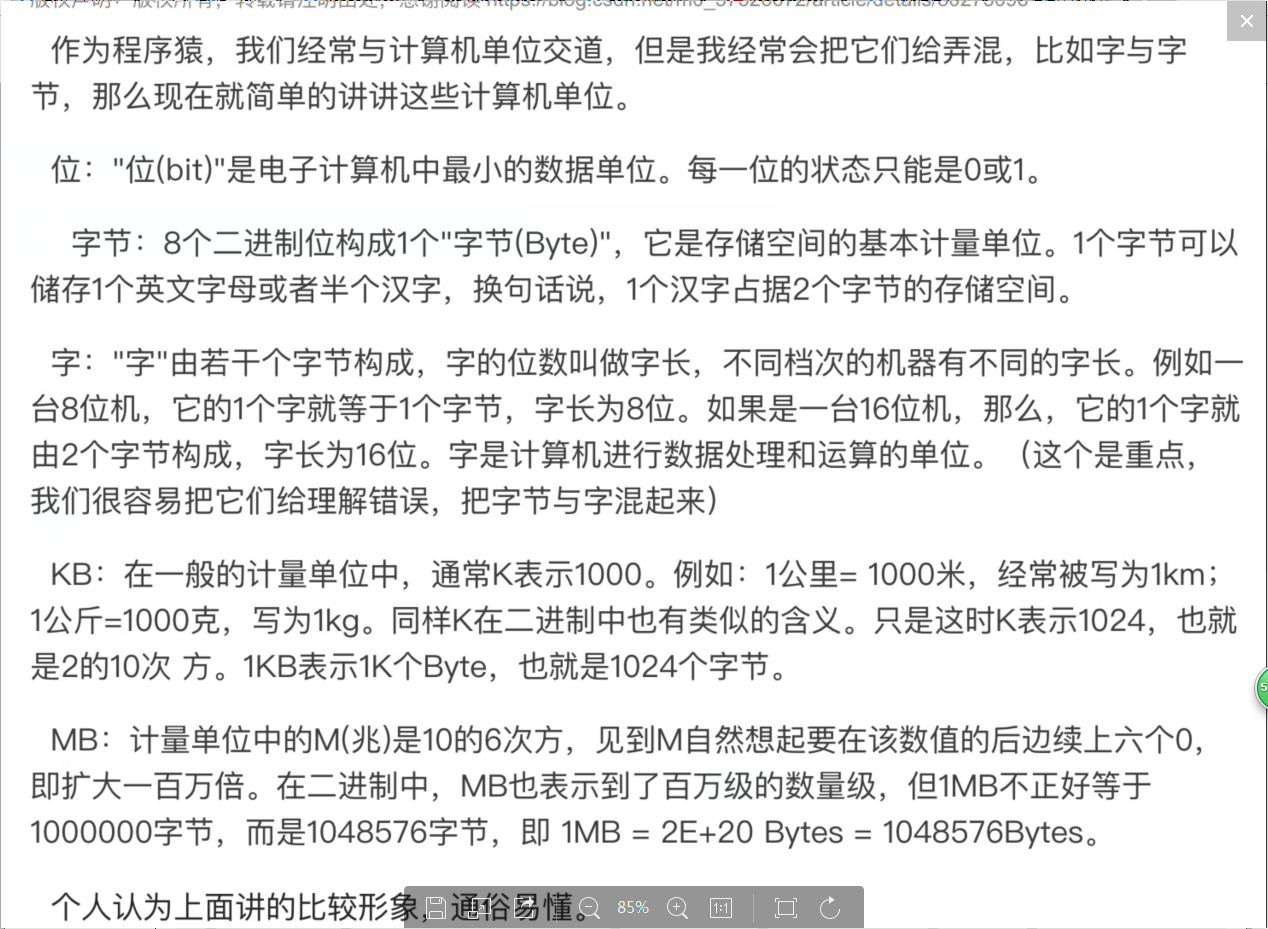
和上面的对比 主要是(new String(b,0,len));FileInputStream
* 这个emm 什么读取出来会有大量空格,。, 反正拿到的长度是一样的

@Test public void test14() throws IOException { String fileName = "D:" + File.separator + "hello.txt"; File f = new File(fileName); InputStream in = new FileInputStream(f); byte[] b = new byte[1024]; int len = in.read(b); in.close(); System.out.println("读入长度为:" + len); System.out.println(new String(b, 0, len)); }
这个我也没去查,就是new String(b,0,len)
节省空间,,,是不是真的节省了??FileInputStream

@Test public void test15() throws IOException { String fileName = "D:" + File.separator + "Hello.txt"; File f = new File(fileName); InputStream in = new FileInputStream(f); byte[] b = new byte[(int) f.length()]; in.read(b); System.out.println("文件长度为:" + f.length()); in.close(); System.out.println(new String(b)); System.out.println(b.length); }
将上面的改为一个一个读,FileInputStream,对比一个一个写

@Test public void test16() throws IOException { String fileName = "D:" + File.separator + "hello.txt"; File f = new File(fileName); InputStream in = new FileInputStream(f); byte[] b = new byte[(int) f.length()]; for (int i = 0; i < b.length; i++) { b[i] = (byte) in.read(); } in.close(); System.out.println(new String(b)); }
这个说明了,文件读完的时候会返回-1 FileInputStream

@Test public void test17() throws IOException { File f = new File(new String("D:" + File.separator + "hello.txt")); InputStream in = new FileInputStream(f); byte[] b = new byte[1024]; int count = 0; int temp = 0; while ((temp = in.read()) != -1) { b[count++] = (byte) temp; } in.close(); System.out.println(new String(b)); }
使用字符流, 字符的拼接 FileWriter 总是file--write--output,这就是程序,相对文件的命名方法,这个程序是向文件write,output,往文件写东西,额程序这个时候是
信息输出的

@Test public void test18() throws IOException { String fileName = "D:" + File.separator + "hello.txt"; File f = new File(fileName); // Writer out = new FileWriter(f); // String str = "hello"; Writer out = new FileWriter(f, true); String str = "append hello"; out.write(str); out.close(); }
而源码jdk8中
public FileWriter(File file, boolean append) throws IOException {
super(new FileOutputStream(file, append));
}
实际上就是掉这个fileoutputstream
字符流
* 从字符串中读取内容 FileReader ---------fileinputstream

@Test public void test19() throws IOException { File f = new File(new String("D:" + File.separator + "hello.txt")); char[] ch = new char[100]; Reader reader = new FileReader(f); int count = reader.read(ch); reader.close(); System.out.println(count); System.out.println(new String(ch, 0, count)); }
这么傻逼的循环,你前面这个char还不是设置的有大小的,文件大了还不是废了

@Test public void test20() throws IOException { File f = new File(new String("D:" + File.separator + "hello.txt")); char[] ch = new char[10]; Reader reader = new FileReader(f); int temp = 0; int count = 0; while ((temp = reader.read()) != -1) { ch[count++] = (char) temp; } reader.close(); System.out.println(new String(ch, 0, count)); }