Radar Installation
Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions: 76641 | Accepted: 17158 |
Description
Assume the coasting is an infinite straight line. Land is in one side of coasting, sea in the other. Each small island is a point locating in the sea side. And any radar installation, locating on the coasting, can only cover d distance, so an island in the sea can be covered by a radius installation, if the distance between them is at most d.
We use Cartesian coordinate system, defining the coasting is the x-axis. The sea side is above x-axis, and the land side below. Given the position of each island in the sea, and given the distance of the coverage of the radar installation, your task is to write a program to find the minimal number of radar installations to cover all the islands. Note that the position of an island is represented by its x-y coordinates.
Figure A Sample Input of Radar Installations
We use Cartesian coordinate system, defining the coasting is the x-axis. The sea side is above x-axis, and the land side below. Given the position of each island in the sea, and given the distance of the coverage of the radar installation, your task is to write a program to find the minimal number of radar installations to cover all the islands. Note that the position of an island is represented by its x-y coordinates.
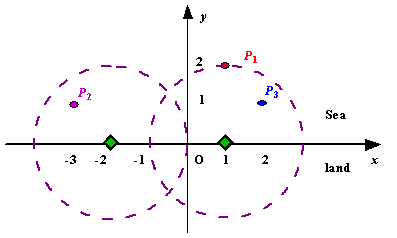
Figure A Sample Input of Radar Installations
Input
The input consists of several test cases. The first line of each case contains two integers n (1<=n<=1000) and d, where n is the number of islands in the sea and d is the distance of coverage of the radar installation. This is followed by n lines each containing two integers representing the coordinate of the position of each island. Then a blank line follows to separate the cases.
The input is terminated by a line containing pair of zeros
The input is terminated by a line containing pair of zeros
Output
For each test case output one line consisting of the test case number followed by the minimal number of radar installations needed. "-1" installation means no solution for that case.
Sample Input
3 2
1 2
-3 1
2 1
1 2
0 2
0 0
Sample Output
Case 1: 2
Case 2: 1
题目大意:求最小的雷达个数能够覆盖所有的岛屿,n为岛屿的个数,d时每个雷达能够覆盖的范围
思路:贪心,我们先对所有的岛屿按照x坐标排序,从左向右依次计算雷达在x轴的区间能够覆盖该岛屿。
如果发现区间有重叠,则更新区间范围,因为要同时满足覆盖两个岛屿,因此更新的区间就是重叠的区间
即区间左端点为两个大的一个,右端点为两个小的一个,如果不重叠则需要增加新的雷达.
代码如下:
#include <iostream> #include<cstdio> #include<cmath> #include<algorithm> #include<cstring> using namespace std; const int maxs = 1000+5; const int INF = 0x3f3f3f3f; struct Node { int x,y; }island[maxs];//岛屿 struct Area { double x1,x2;//能够覆盖岛屿的雷达的左右两端点 }radar[maxs]; int n,d; bool cmp(Node a,Node b) { return a.x<b.x; } int main() { //freopen("in.txt","r",stdin); int t = 1; while(scanf("%d%d",&n,&d)!=EOF&&n) { int ans = 0; memset(radar,0,sizeof(radar)); radar[0].x1=radar[0].x2=-INF; for(int i=1;i<=n;i++) scanf("%d%d",&island[i].x,&island[i].y); sort(island+1,island+1+n,cmp); for(int i=1;i<=n;i++) { if(d<0||d<island[i].y||island[i].y<0) { ans = -1; break; } double x = sqrt(d*d-island[i].y*island[i].y); double x1 = island[i].x-x,x2=island[i].x+x; if(x1>radar[ans].x2)//说明需要加一个雷达 { ans++; radar[ans].x1 = x1; radar[ans].x2 = x2; } else { //更正共用雷达的区间 radar[ans].x1 = max(radar[ans].x1,x1); radar[ans].x2 = min(radar[ans].x2,x2); } } getchar(); printf("Case %d: %d ",t++,ans); } }