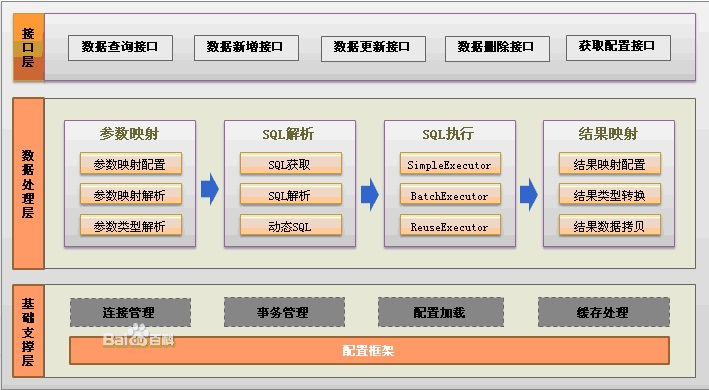
private TeacherEntity teacherEntity;
<resultMap type="ClassEntity" id="classResultMap"> <id property="classID" column="CLASS_ID" /> <result property="className" column="CLASS_NAME" /> <result property="classYear" column="CLASS_YEAR" /> <association property="teacherEntity" column="TEACHER_ID" select="getTeacher"/> </resultMap> <select id="getClassByID" parameterType="String" resultMap="classResultMap"> SELECT * FROM CLASS_TBL CT WHERE CT.CLASS_ID = #{classID}; </select>
TeacherMapper.xml文件部分内容:
<resultMap type="TeacherEntity" id="teacherResultMap"> <id property="teacherID" column="TEACHER_ID" /> <result property="teacherName" column="TEACHER_NAME" /> <result property="teacherSex" column="TEACHER_SEX" /> <result property="teacherBirthday" column="TEACHER_BIRTHDAY"/> <result property="workDate" column="WORK_DATE"/> <result property="professional" column="PROFESSIONAL"/> </resultMap> <select id="getTeacher" parameterType="String" resultMap="teacherResultMap"> SELECT * FROM TEACHER_TBL TT WHERE TT.TEACHER_ID = #{teacherID} </select>
<resultMap type="ClassEntity" id="classResultMap"> <id property="classID" column="CLASS_ID" /> <result property="className" column="CLASS_NAME" /> <result property="classYear" column="CLASS_YEAR" /> <association property="teacherEntity" column="TEACHER_ID" resultMap="teacherResultMap"/> </resultMap> <select id="getClassAndTeacher" parameterType="String" resultMap="classResultMap"> SELECT * FROM CLASS_TBL CT LEFT JOIN TEACHER_TBL TT ON CT.TEACHER_ID = TT.TEACHER_ID WHERE CT.CLASS_ID = #{classID}; </select>
3.5 collection聚集
private List<StudentEntity> studentList;
3.5.1使用select实现聚集
<resultMap type="ClassEntity" id="classResultMap"> <id property="classID" column="CLASS_ID" /> <result property="className" column="CLASS_NAME" /> <result property="classYear" column="CLASS_YEAR" /> <association property="teacherEntity" column="TEACHER_ID" select="getTeacher"/> <collection property="studentList" column="CLASS_ID" javaType="ArrayList" ofType="StudentEntity" select="getStudentByClassID"/> </resultMap> <select id="getClassByID" parameterType="String" resultMap="classResultMap"> SELECT * FROM CLASS_TBL CT WHERE CT.CLASS_ID = #{classID}; </select>
StudentMapper.xml文件部分内容:
<!-- java属性,数据库表字段之间的映射定义 --> <resultMap type="StudentEntity" id="studentResultMap"> <id property="studentID" column="STUDENT_ID" /> <result property="studentName" column="STUDENT_NAME" /> <result property="studentSex" column="STUDENT_SEX" /> <result property="studentBirthday" column="STUDENT_BIRTHDAY" /> </resultMap> <!-- 查询学生list,根据班级id --> <select id="getStudentByClassID" parameterType="String" resultMap="studentResultMap"> <include refid="selectStudentAll" /> WHERE ST.CLASS_ID = #{classID} </select>
<resultMap type="ClassEntity" id="classResultMap"> <id property="classID" column="CLASS_ID" /> <result property="className" column="CLASS_NAME" /> <result property="classYear" column="CLASS_YEAR" /> <association property="teacherEntity" column="TEACHER_ID" resultMap="teacherResultMap"/> <collection property="studentList" column="CLASS_ID" javaType="ArrayList" ofType="StudentEntity" resultMap="studentResultMap"/> </resultMap> <select id="getClassAndTeacherStudent" parameterType="String" resultMap="classResultMap"> SELECT * FROM CLASS_TBL CT LEFT JOIN STUDENT_TBL ST ON CT.CLASS_ID = ST.CLASS_ID LEFT JOIN TEACHER_TBL TT ON CT.TEACHER_ID = TT.TEACHER_ID WHERE CT.CLASS_ID = #{classID}; </select>
基础知识参考 http://www.mybatis.org/mybatis-3/zh/getting-started.html
MyBatis中使用#和$书写占位符有什么区别?
答:#将传入的数据都当成一个字符串,会对传入的数据自动加上引号;$将传入的数据直接显示生成在SQL中。注意:使用$占位符可能会导致SQL注射攻击,能用#的地方就不要使用$,写order by子句的时候应该用$而不是#
解释一下MyBatis中命名空间(namespace)的作用。
答:在大型项目中,可能存在大量的SQL语句,这时候为每个SQL语句起一个唯一的标识(ID)就变得并不容易了。为了解决这个问题,在MyBatis中,可以为每个映射文件起一个唯一的命名空间,这样定义在这个映射文件中的每个SQL语句就成了定义在这个命名空间中的一个ID。只要我们能够保证每个命名空间中这个ID是唯一的,即使在不同映射文件中的语句ID相同,也不会再产生冲突了。
145、MyBatis中的动态SQL是什么意思?
答:对于一些复杂的查询,我们可能会指定多个查询条件,但是这些条件可能存在也可能不存在,例如在58同城上面找房子,我们可能会指定面积、楼层和所在位置来查找房源,也可能会指定面积、价格、户型和所在位置来查找房源,此时就需要根据用户指定的条件动态生成SQL语句。如果不使用持久层框架我们可能需要自己拼装SQL语句,还好MyBatis提供了动态SQL的功能来解决这个问题。MyBatis中用于实现动态SQL的元素主要有:
- if
- choose / when / otherwise
- trim
- where
- set
- foreach
下面是映射文件的片段。
<select id="foo" parameterType="Blog" resultType="Blog">
select * from t_blog where 1 = 1
<if test="title != null">
and title = #{title}
</if>
<if test="content != null">
and content = #{content}
</if>
<if test="owner != null">
and owner = #{owner}
</if>
</select>
当然也可以像下面这些书写。
<select id="foo" parameterType="Blog" resultType="Blog">
select * from t_blog where 1 = 1
<choose>
<when test="title != null">
and title = #{title}
</when>
<when test="content != null">
and content = #{content}
</when>
<otherwise>
and owner = "owner1"
</otherwise>
</choose>
</select>
再看看下面这个例子。
<select id="bar" resultType="Blog">
select * from t_blog where id in
<foreach collection="array" index="index"
item="item" open="(" separator="," close=")">
#{item}
</foreach>
</select>